Why Rushing Through Basics Isn’t Building Your Foundation in Programming
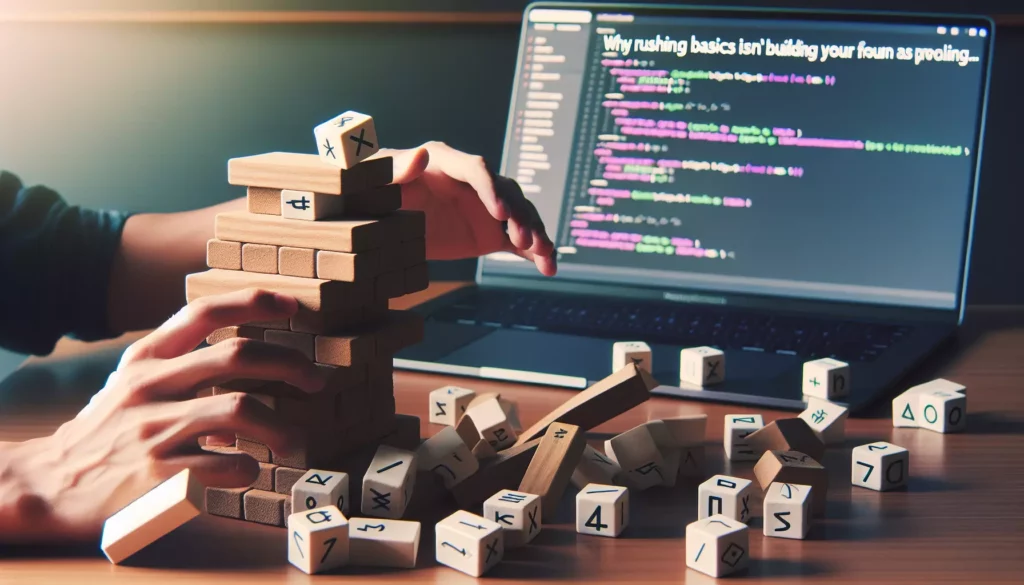
In the fast-paced world of tech, there’s an understandable eagerness to quickly master programming and land that dream job at a prestigious company. Many aspiring developers, fueled by success stories of self-taught programmers and bootcamp graduates securing six-figure salaries, are tempted to take shortcuts. They skim through fundamentals, memorize solutions to common interview problems, and rush toward advanced concepts without truly understanding the basics.
But here’s the reality: rushing through programming fundamentals is like building a skyscraper on a foundation of sand. It might look impressive initially, but it won’t stand the test of time.
In this article, we’ll explore why taking the time to thoroughly master programming basics is not just beneficial but essential for long-term success in tech. We’ll examine the pitfalls of the “rush approach” and provide strategies for building a rock-solid foundation that will support your entire career.
The Illusion of Progress: Why Speed Isn’t Always Your Friend
The tech industry moves at lightning speed, and there’s constant pressure to keep up. New frameworks emerge seemingly overnight, languages evolve rapidly, and job descriptions list increasingly demanding requirements. This environment creates what I call the “illusion of progress” – the belief that moving quickly through learning material equates to actual skill development.
When you rush through basics, you might experience:
- Surface-level understanding: You recognize concepts but struggle to apply them in new contexts
- Knowledge gaps: Critical foundational elements are missed entirely
- Fragile comprehension: Your understanding crumbles when faced with slightly modified problems
- Dependency on examples: You can only solve problems that closely resemble ones you’ve seen before
Consider this real-world example: A student learning arrays might quickly memorize how to perform common operations like insertion and deletion. They complete practice problems successfully and move on to more advanced data structures. Months later, when asked to optimize an algorithm using array manipulation techniques, they struggle because they never truly understood how arrays work in memory or the performance implications of different operations.
This scenario plays out constantly in technical interviews and on the job. The surface-level knowledge that seemed sufficient during learning proves inadequate when faced with real-world challenges.
The Hidden Cost of Cutting Corners
When you bypass thorough learning of fundamentals, you incur a debt – not financial, but intellectual. This “knowledge debt” compounds over time, creating increasingly significant obstacles to your progress.
Technical Debt in Your Learning
Software developers are familiar with the concept of technical debt – cutting corners in code implementation to save time now, only to pay a much higher price later in maintenance and bug fixes. The same principle applies to learning programming.
Skipping fundamentals creates learning debt that manifests in several ways:
- Debugging difficulties: Without strong fundamentals, tracking down bugs becomes exponentially harder
- Inability to learn independently: You become dependent on tutorials and step-by-step guides
- Impostor syndrome: The awareness of your shaky foundation contributes to feelings of inadequacy
- Career ceiling: Advancement beyond junior roles becomes difficult without solid fundamentals
A junior developer I mentored once confessed that he had “faked” his way through learning JavaScript. He could build simple applications by copying patterns from tutorials but didn’t understand closures, prototypal inheritance, or asynchronous programming. When tasked with debugging a complex production issue, he spent days struggling with concepts his peers grasped quickly. What seemed like a time-saving approach to learning ultimately cost him weeks of productivity and considerable professional embarrassment.
The Interview Wall
Perhaps nowhere is a weak foundation more exposed than in technical interviews, especially at competitive companies. While memorizing solutions to common LeetCode problems might help you pass some interviews, companies with rigorous processes will quickly identify candidates with superficial understanding.
Technical interviews typically assess:
- Your ability to apply concepts to new problems
- How you think through challenges, not just whether you reach the correct answer
- Your understanding of why certain approaches are more efficient than others
- Your capability to optimize solutions based on constraints
These assessments are nearly impossible to fake. Interviewers at companies like Google, Amazon, and Facebook are trained to probe beyond memorized solutions and evaluate fundamental understanding.
The Fundamentals You Can’t Afford to Rush
So what exactly constitutes the “fundamentals” that deserve your thorough attention? While the specifics might vary by your chosen specialization, certain core concepts form the bedrock of programming knowledge.
1. Data Structures and Their Operations
Understanding how data is organized and manipulated is critical to efficient programming. Key structures include:
- Arrays and Strings: How they’re stored in memory, indexing, and time complexity of operations
- Linked Lists: Singly and doubly linked, insertion and deletion operations
- Stacks and Queues: LIFO vs. FIFO principles and implementation approaches
- Trees and Graphs: Traversal methods, representation techniques, and common algorithms
- Hash Tables: Collision resolution, load factors, and efficient key selection
For each structure, you should understand:
- The time and space complexity of common operations
- When to use each structure (and when not to)
- How to implement the structure from scratch
- Common pitfalls and edge cases
Consider this simple example of array operations:
// JavaScript example of array operations
let array = [1, 2, 3, 4, 5];
// Accessing elements - O(1) time complexity
let thirdElement = array[2]; // Returns 3
// Inserting at the end - Amortized O(1)
array.push(6); // Array becomes [1, 2, 3, 4, 5, 6]
// Inserting at the beginning - O(n) because all elements must shift
array.unshift(0); // Array becomes [0, 1, 2, 3, 4, 5, 6]
// Removing from middle - O(n) due to shifting
array.splice(3, 1); // Removes element at index 3
Understanding why insertion at the beginning of an array is O(n) while insertion at the end is amortized O(1) requires knowledge of how arrays work in memory. This level of understanding is what separates surface-level knowledge from true mastery.
2. Algorithmic Thinking and Problem-Solving Patterns
Beyond specific algorithms, understanding problem-solving approaches is crucial:
- Recursion and how it relates to the call stack
- Divide and conquer strategies
- Dynamic programming and memoization
- Greedy algorithms and when they’re appropriate
- Backtracking for combinatorial problems
These approaches form the foundation of algorithmic thinking. Consider recursion, which is often rushed through by beginners:
// Simple recursive function to calculate factorial
function factorial(n) {
// Base case
if (n <= 1) {
return 1;
}
// Recursive case
return n * factorial(n - 1);
}
Understanding this function requires grasping:
- How the call stack manages recursive calls
- The importance of the base case
- How values are returned up the call chain
- The potential for stack overflow with large inputs
Many developers memorize recursive patterns without truly understanding how recursion works, leading to difficulty when they encounter complex recursive problems.
3. Language Fundamentals and Programming Paradigms
Each programming language has its own philosophy and features:
- Type systems: Static vs. dynamic, strong vs. weak
- Memory management: Garbage collection, manual memory allocation
- Execution models: Interpreted vs. compiled, JIT compilation
- Paradigms: Object-oriented, functional, procedural
Take JavaScript's closure concept, for example:
function createCounter() {
let count = 0;
return function() {
count++;
return count;
};
}
const counter = createCounter();
console.log(counter()); // 1
console.log(counter()); // 2
A developer who rushed through JavaScript basics might use closures without understanding that the inner function maintains access to the outer function's scope even after the outer function has completed execution. This fundamental concept underpins many JavaScript patterns and libraries.
4. System Design and Architecture Principles
As you advance, understanding how systems fit together becomes critical:
- Client-server architecture
- API design principles
- Database fundamentals: Relational vs. NoSQL, indexing, normalization
- Caching strategies
- Concurrency and parallelism
These concepts form the backbone of modern application development and are difficult to grasp without strong fundamentals in programming basics.
Signs You're Rushing Through Fundamentals
How do you know if you're guilty of rushing? Watch for these warning signs:
The Copy-Paste Syndrome
If you frequently find yourself copying code without fully understanding how it works, you're likely rushing. While reference implementations can be valuable learning tools, they should be starting points for understanding, not shortcuts to avoid learning.
Challenge yourself: After using reference code, close it and try to reimplement the solution from memory, explaining each line's purpose as you write it.
Tutorial Dependency
If you can only solve problems with step-by-step guidance, your foundation may be weak. Many developers get stuck in "tutorial hell," where they can follow along with guided examples but struggle to apply concepts independently.
Break this cycle by forcing yourself to work through problems without guidance, even if it takes significantly longer. The struggle is where genuine learning happens.
The "I'll Learn It Later" Mindset
Postponing understanding of core concepts creates a dangerous accumulation of knowledge gaps. If you frequently tell yourself "I don't need to understand how this works right now," you're setting yourself up for future difficulties.
Instead, adopt the mindset that understanding fundamentals is non-negotiable. When you encounter a concept you don't fully grasp, make it a priority to learn it thoroughly before moving on.
Difficulty Explaining Concepts
Richard Feynman famously said, "If you can't explain it simply, you don't understand it well enough." If you struggle to explain basic programming concepts to others, it's a strong indicator that your understanding is superficial.
Practice explaining concepts in simple terms, as if teaching someone with no programming background. This exercise quickly reveals gaps in your understanding.
Building a Solid Foundation: Strategies That Work
Now that we understand the importance of fundamentals and how to identify when we're rushing, let's explore effective strategies for building a solid foundation.
The Deliberate Practice Approach
Psychologist K. Anders Ericsson's research on expertise development highlights the importance of deliberate practice – focused, structured practice designed to improve performance. For programming, this means:
- Setting specific learning goals for each study session
- Focusing on areas of weakness rather than practicing what you already know
- Seeking immediate feedback on your solutions
- Reflecting on your learning process to identify improvement opportunities
For example, if you're learning about binary search trees, your deliberate practice might include:
- Implementing a BST from scratch without reference
- Adding insertion, deletion, and traversal methods
- Testing your implementation with edge cases (empty trees, single nodes, unbalanced trees)
- Analyzing the time complexity of your operations
- Comparing your implementation to standard libraries
This approach is more time-intensive than simply reading about BSTs or copying an implementation, but it builds deeper understanding and retention.
The Implementation Challenge
One of the most effective ways to solidify your understanding of programming concepts is to implement them yourself, from scratch. This approach forces you to grapple with the details that tutorials often gloss over.
Consider challenging yourself to implement:
- Common data structures (linked lists, hash tables, trees)
- Sorting algorithms (quicksort, mergesort, heapsort)
- A simple interpreter or compiler
- A basic database engine
- A simplified version of a framework or library you use regularly
These projects won't produce code you'll use in production, but they'll dramatically deepen your understanding of how these systems work.
For example, implementing a hash table requires understanding:
class HashTable {
constructor(size = 53) {
this.keyMap = new Array(size);
}
_hash(key) {
let total = 0;
const WEIRD_PRIME = 31;
for (let i = 0; i < Math.min(key.length, 100); i++) {
const char = key[i];
const value = char.charCodeAt(0) - 96;
total = (total * WEIRD_PRIME + value) % this.keyMap.length;
}
return total;
}
set(key, value) {
const index = this._hash(key);
if (!this.keyMap[index]) {
this.keyMap[index] = [];
}
this.keyMap[index].push([key, value]);
}
get(key) {
const index = this._hash(key);
if (!this.keyMap[index]) return undefined;
for (let i = 0; i < this.keyMap[index].length; i++) {
if (this.keyMap[index][i][0] === key) {
return this.keyMap[index][i][1];
}
}
return undefined;
}
}
Working through this implementation requires understanding hash functions, collision resolution, and the performance implications of different approaches – knowledge that's difficult to gain by simply using a built-in Map or object.
The Feynman Technique
Named after physicist Richard Feynman, this learning technique involves explaining concepts in simple terms, as if teaching a child. The process has four steps:
- Choose a concept you want to understand
- Explain it in simple terms, as if teaching someone unfamiliar with the subject
- Identify gaps in your explanation where you struggle or resort to complex terminology
- Review and simplify until you can explain the concept clearly and completely
This technique is particularly effective for programming concepts because it forces you to break down complex ideas into fundamental components. Try explaining concepts like recursion, closures, or asynchronous programming without using technical jargon – the exercise will quickly reveal whether your understanding is superficial or deep.
Spaced Repetition and Retrieval Practice
Cognitive science research shows that spacing out your learning and actively retrieving information leads to better long-term retention than cramming or passive review.
Implement spaced repetition by:
- Reviewing concepts at increasing intervals (1 day, 3 days, 1 week, 2 weeks, etc.)
- Using flashcard systems like Anki for programming concepts
- Regularly revisiting previously completed problems without looking at your solution
Combine this with retrieval practice:
- After learning a concept, close all references and write an explanation
- Implement solutions from memory, then compare with reference implementations
- Teach concepts to others without preparation
These techniques are more effortful than simply rereading notes or tutorials, but they lead to much stronger retention and understanding.
The Long Game: Why Patience Pays Off
Building a solid foundation takes time – often significantly more time than rushing through concepts. This investment might seem excessive, especially when you see peers apparently progressing faster or hear stories of developers landing jobs after short bootcamps.
However, programming careers are marathons, not sprints. The time invested in building strong fundamentals pays dividends throughout your career in several ways:
Accelerated Learning of New Technologies
When you understand programming fundamentals deeply, learning new languages, frameworks, and tools becomes much easier. You recognize patterns, understand the underlying principles, and can focus on the unique aspects of new technologies rather than struggling with basic concepts.
For example, a developer with a strong understanding of programming paradigms can learn a new language like Rust or Elixir much faster than someone who only knows JavaScript syntax without understanding core concepts like memory management or concurrency.
Problem-Solving Versatility
Strong fundamentals enable you to approach problems from multiple angles and adapt when your first approach fails. This versatility is invaluable in both technical interviews and on-the-job problem solving.
Consider a developer tasked with optimizing a slow database query. Someone with a solid understanding of database fundamentals, indexing strategies, and query execution plans can systematically analyze and improve the query. A developer who rushed through database basics might try random optimizations or resort to brute-force approaches that don't address the root cause.
Career Longevity and Advancement
The tech industry changes rapidly, but fundamental principles remain relatively stable. Developers with strong foundations can adapt to industry shifts, while those who rely on surface-level knowledge of specific technologies often struggle when those technologies fall out of favor.
Additionally, advancement to senior and leadership roles typically requires deep technical understanding. Technical architects, engineering managers, and CTOs need to make decisions based on fundamental principles rather than trending technologies.
Finding Balance: Pragmatic Learning Without Rushing
While I've emphasized the importance of not rushing through fundamentals, it's also important to find a balance. Perfectionism can be as problematic as rushing, leading to analysis paralysis and never feeling "ready" to advance.
Here are some guidelines for finding that balance:
Understand vs. Memorize
Focus on understanding principles rather than memorizing implementations. You don't need to memorize the exact syntax of every method in a language's standard library, but you should understand the underlying concepts.
For example, you don't need to memorize every array method in JavaScript, but you should understand:
- The difference between methods that mutate arrays and those that return new arrays
- How higher-order functions like map, filter, and reduce work
- The time complexity implications of different array operations
Depth in Core Concepts, Breadth in Applications
Develop deep understanding of core programming concepts, but allow yourself to explore applications more broadly. This approach provides both the foundation you need and exposure to different domains.
For example, you might deeply learn:
- Data structures and algorithms
- Core language features
- Design patterns
- System design principles
While exploring applications like:
- Web development
- Mobile app development
- Data science
- Game development
This balanced approach prevents both the fragility of rushing and the stagnation of perfectionism.
Build Projects While Learning
Apply concepts to projects as you learn them, rather than waiting until you've "mastered" a topic. Projects provide context for abstract concepts and help identify areas where your understanding needs improvement.
For example, while learning about databases, you might build a simple application that:
- Creates and manipulates database tables
- Implements different types of relationships
- Optimizes queries with indexes
- Handles transactions and concurrency
This project-based approach maintains momentum while ensuring you're building practical skills alongside theoretical knowledge.
Conclusion: The Sustainable Path to Programming Mastery
In a field that moves as quickly as software development, the temptation to rush through fundamentals is strong. The pressure to keep up with peers, master the latest frameworks, and prepare for interviews can push us toward shortcuts and surface-level learning.
However, as we've explored in this article, rushing through basics creates an illusion of progress while undermining long-term success. The developers who thrive throughout their careers are those who invest time in building strong foundations – who understand not just how to use programming tools, but why they work the way they do.
Building this foundation requires patience, deliberate practice, and sometimes slowing down to ensure you truly understand concepts before moving on. It means embracing the struggle of learning rather than seeking the path of least resistance.
The good news is that this approach is sustainable and rewarding. Each fundamental concept you truly master makes learning the next concept easier. Over time, your learning accelerates not because you're rushing, but because you've built the mental models necessary to assimilate new information effectively.
So the next time you're tempted to skim through a complex programming concept or copy-paste code without understanding it, remember: in programming, as in architecture, the quality of the foundation determines how high you can ultimately build. Take the time to build yours properly.
Your future self – debugging a complex issue, designing a system, or interviewing for that dream job – will thank you for the solid foundation you're building today.