Why Revisiting Old Problems Can Help You Master New Coding Challenges
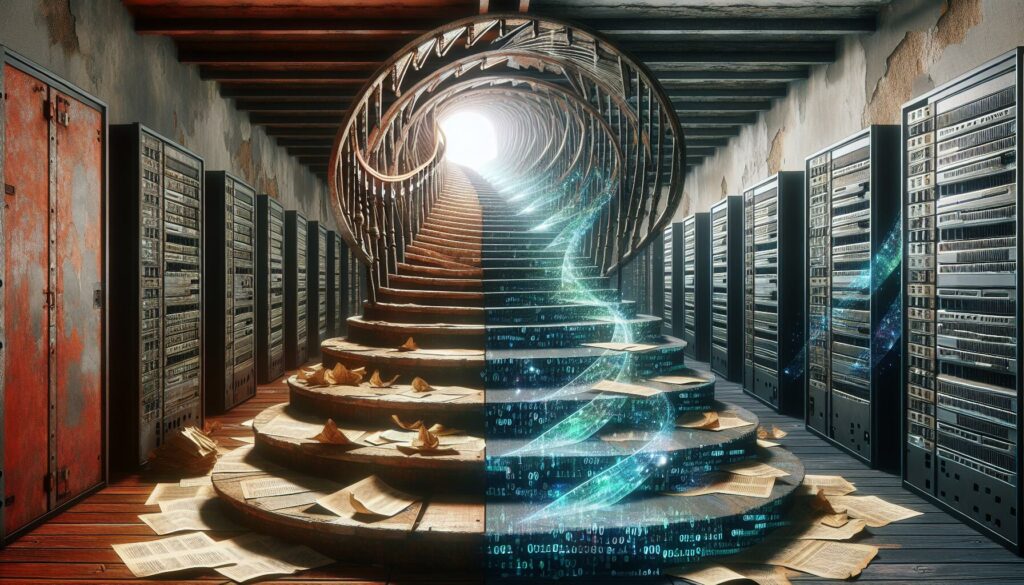
In the ever-evolving world of programming, it’s easy to get caught up in the pursuit of new technologies, frameworks, and cutting-edge techniques. However, there’s a powerful learning strategy that often goes overlooked: revisiting old coding problems. This practice, sometimes referred to as “deliberate practice” in the realm of skill acquisition, can be a game-changer for programmers at all levels, from beginners to seasoned professionals. In this article, we’ll explore why going back to previously solved problems can significantly enhance your coding skills and prepare you for new challenges, particularly in the context of technical interviews and algorithmic problem-solving.
The Power of Repetition in Learning
Before we dive into the specific benefits of revisiting old coding problems, it’s important to understand the fundamental principle behind this practice: the power of repetition in learning. Cognitive science has long established that repetition is a key factor in memory consolidation and skill development. When we revisit a concept or problem multiple times, we strengthen the neural pathways associated with that knowledge, making it easier to recall and apply in the future.
In the context of coding, this means that each time you revisit a problem, you’re not just solving it again—you’re reinforcing your understanding of the underlying concepts, algorithms, and problem-solving strategies. This repetition builds a stronger foundation for tackling new, more complex challenges in the future.
Deepening Your Understanding of Fundamental Concepts
One of the primary benefits of revisiting old coding problems is the opportunity to deepen your understanding of fundamental programming concepts. When you first encounter a problem, you might focus primarily on finding a solution that works. However, when you return to the same problem later, you have the chance to approach it with a fresh perspective and a more advanced skill set.
For example, consider a classic problem like implementing a binary search algorithm. The first time you solve this, you might be focused on getting the basic logic right:
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
However, when revisiting this problem, you might start to consider more nuanced aspects:
- How does the choice of
(left + right) // 2
for the midpoint calculation affect the algorithm’s performance for very large arrays? - Are there edge cases you didn’t consider in your initial implementation?
- How can you modify the algorithm to handle duplicate elements or return the first/last occurrence of the target?
By exploring these questions, you’re not just rehashing old ground—you’re building a more robust and nuanced understanding of the binary search algorithm and its applications.
Improving Problem-Solving Efficiency
Another significant benefit of revisiting old problems is the improvement in problem-solving efficiency. When you encounter a problem for the second or third time, you’re likely to solve it more quickly and with greater ease. This efficiency gain isn’t just about memorizing solutions; it’s about developing a more intuitive understanding of problem-solving patterns and strategies.
As you revisit problems, you’ll start to recognize common patterns and techniques that can be applied across various challenges. For instance, the two-pointer technique used in array manipulation problems or the sliding window approach for substring problems become second nature. This pattern recognition is invaluable when facing new challenges, as it allows you to quickly identify familiar elements and adapt known strategies to novel situations.
Enhancing Code Quality and Optimization
Revisiting old problems provides an excellent opportunity to focus on code quality and optimization. When you first solve a problem, your primary goal is often to find a working solution. However, when you return to it later, you can shift your focus to improving the efficiency, readability, and maintainability of your code.
Consider this initial solution to the “Two Sum” problem:
def two_sum(nums, target):
for i in range(len(nums)):
for j in range(i+1, len(nums)):
if nums[i] + nums[j] == target:
return [i, j]
return []
While this brute-force approach works, revisiting the problem might lead you to a more optimized solution using a hash table:
def two_sum_optimized(nums, target):
num_dict = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_dict:
return [num_dict[complement], i]
num_dict[num] = i
return []
This process of refining and optimizing solutions not only improves your coding skills but also prepares you for real-world scenarios where code efficiency and scalability are crucial.
Preparing for Technical Interviews
For many programmers, especially those aiming for positions at major tech companies (often referred to as FAANG: Facebook, Amazon, Apple, Netflix, Google), technical interviews are a significant hurdle. These interviews often focus on algorithmic problem-solving and coding efficiency. Revisiting old problems is an excellent way to prepare for these high-pressure situations.
By regularly revisiting a diverse set of problems, you build confidence in your ability to tackle various challenges. You become more comfortable with common data structures and algorithms, which are frequently the focus of technical interviews. Moreover, the practice of explaining your thought process and discussing alternative approaches—which you naturally do when revisiting problems—directly translates to the skills needed in a technical interview setting.
Tracking Progress and Identifying Areas for Improvement
Revisiting old problems serves as a tangible way to track your progress as a programmer. By comparing your current solutions to those you wrote weeks, months, or even years ago, you can see how your coding style, efficiency, and problem-solving approach have evolved.
This practice also helps identify areas where you may still need improvement. For instance, you might notice that while your algorithmic thinking has improved, your code readability could use some work. Or perhaps you’ve become more efficient with array manipulations but still struggle with tree-based problems. These insights are invaluable for directing your future learning efforts.
Implementing a Systematic Review Process
To maximize the benefits of revisiting old problems, it’s helpful to implement a systematic review process. Here’s a suggested approach:
- Keep a Problem Log: Maintain a list of problems you’ve solved, along with brief notes on the approaches you used.
- Set Regular Review Intervals: Schedule time to revisit problems after varying intervals (e.g., 1 week, 1 month, 3 months).
- Solve from Scratch: When revisiting a problem, try to solve it from scratch before looking at your old solution.
- Compare and Analyze: After solving, compare your new solution with the old one. Analyze the differences in approach, efficiency, and code quality.
- Explore Variations: Once you’ve revisited the original problem, consider variations or related problems to further extend your understanding.
Leveraging Tools and Platforms
Several coding platforms and tools can support your practice of revisiting old problems. Platforms like AlgoCademy, LeetCode, and HackerRank offer features that allow you to revisit problems you’ve previously solved. These platforms often provide:
- A history of your submissions, allowing you to compare your solutions over time
- Discussion forums where you can explore different approaches to the same problem
- Performance metrics to help you track improvements in runtime and memory usage
- AI-powered suggestions for related problems or areas of improvement
Utilizing these features can enhance your review process and provide additional insights into your progress and areas for improvement.
Balancing Old and New: A Holistic Approach to Skill Development
While the benefits of revisiting old problems are clear, it’s important to strike a balance between reviewing familiar content and tackling new challenges. A holistic approach to skill development in programming should include:
- Regular Problem Review: Set aside time to revisit and refine solutions to problems you’ve previously solved.
- New Problem Exploration: Continuously challenge yourself with new problems to expand your skill set.
- Concept Deep Dives: Periodically focus on deep dives into specific concepts or algorithms to enhance your theoretical understanding.
- Real-World Application: Apply your skills to personal projects or open-source contributions to gain practical experience.
- Peer Learning: Engage in code reviews, pair programming, or discussion forums to learn from and teach others.
By combining these elements, you create a comprehensive learning strategy that not only reinforces your existing knowledge but also continually pushes you to grow as a programmer.
Overcoming the “I Already Know This” Mindset
One challenge you might face when revisiting old problems is the feeling that you’re wasting time on something you already know. It’s important to overcome this mindset and recognize that true mastery comes from repeated exposure and practice.
Consider the following strategies to maintain motivation when revisiting familiar problems:
- Set New Goals: Instead of just solving the problem, set specific goals like improving runtime efficiency, reducing space complexity, or writing more readable code.
- Teach the Concept: Pretend you’re explaining the problem and solution to someone else. This often reveals gaps in your understanding or leads to new insights.
- Explore Edge Cases: Challenge yourself to identify and handle edge cases you might have overlooked in your initial solution.
- Implement in a Different Language: If you originally solved the problem in Python, try implementing it in JavaScript or C++. This reinforces the conceptual understanding while also improving your polyglot programming skills.
The Role of Spaced Repetition in Coding Practice
The concept of spaced repetition, a learning technique that involves reviewing material at gradually increasing intervals, can be particularly effective when applied to coding practice. This method aligns well with the idea of revisiting old problems and can significantly enhance long-term retention of programming concepts and problem-solving strategies.
Here’s how you might apply spaced repetition to your coding practice:
- Solve a new problem.
- Review and attempt to solve it again after 1 day.
- If successful, review again after 3 days.
- Then after a week, two weeks, a month, and so on.
This approach ensures that you’re not just cramming information for short-term recall but building lasting understanding and skill.
Connecting the Dots: How Old Problems Inform New Solutions
As you revisit old problems and encounter new challenges, you’ll start to notice connections between different types of problems. These connections are crucial for developing a robust problem-solving toolkit. For example, understanding how to implement depth-first search (DFS) in a binary tree problem can help you tackle graph traversal issues in network analysis.
Consider keeping a “connection journal” where you note these insights. For instance:
"The two-pointer technique I used in the 'Remove Duplicates from Sorted Array' problem
was useful in solving the 'Container With Most Water' challenge. Both involve
efficiently traversing an array while maintaining two pointers."
These observations will help you build a mental map of problem-solving strategies, making it easier to approach new challenges with confidence.
The Impact on Real-World Programming Skills
While much of our discussion has focused on algorithmic problem-solving and interview preparation, the practice of revisiting old problems has significant benefits for real-world programming skills as well. In professional software development, you often encounter situations where you need to revisit and refactor old code, debug complex systems, or optimize existing solutions.
The skills you develop through regular review and refinement of coding problems translate directly to these real-world scenarios:
- Code Refactoring: The practice of revisiting and optimizing old solutions mirrors the process of refactoring legacy code in professional settings.
- Debugging: The deep understanding you gain from repeatedly analyzing problems helps in identifying and fixing bugs in complex systems.
- System Design: As you explore more efficient solutions to old problems, you develop insights that can inform better system design decisions.
- Code Review: The critical eye you develop for your own code enhances your ability to provide constructive feedback in code reviews.
Cultivating a Growth Mindset Through Continuous Review
Perhaps one of the most valuable outcomes of regularly revisiting old problems is the cultivation of a growth mindset. This psychological approach, popularized by psychologist Carol Dweck, emphasizes the belief that abilities and intelligence can be developed through dedication and hard work.
In the context of coding, a growth mindset manifests as:
- Viewing challenges as opportunities for learning rather than tests of ability
- Embracing the process of improvement rather than focusing solely on outcomes
- Recognizing that struggle and effort are part of the learning process, not indicators of lack of talent
By consistently returning to old problems and seeing your progress over time, you reinforce the idea that your coding abilities are not fixed but can continuously improve with practice and persistence.
Conclusion: Embracing the Journey of Continuous Improvement
In the fast-paced world of technology, where new languages, frameworks, and paradigms emerge constantly, the practice of revisiting old coding problems might seem counterintuitive. However, as we’ve explored in this article, this practice is a powerful tool for deepening your understanding, improving your problem-solving skills, and preparing for both technical interviews and real-world programming challenges.
By incorporating regular review into your coding practice, you’re not just preparing for specific problems or interviews—you’re building a robust foundation of knowledge and skills that will serve you throughout your programming career. You’re developing the ability to see connections between different types of problems, to approach challenges with confidence and creativity, and to continuously refine and optimize your solutions.
Remember, mastery in programming, as in any field, is not a destination but a journey. Each time you revisit an old problem, you’re not just retracing your steps—you’re carving a deeper, more insightful path forward. Embrace this process of continuous improvement, and you’ll find that not only do you become better equipped to handle new coding challenges, but you also cultivate a mindset of growth and lifelong learning that will serve you well in all aspects of your career.
So, the next time you’re tempted to always seek out new problems, remember the value in revisiting the old. Your future self—whether in a high-stakes interview or tackling a complex project at work—will thank you for the deep, nuanced understanding you’ve developed through this practice of deliberate, thoughtful review.