Why Reading Documentation is Key to Mastering Syntax
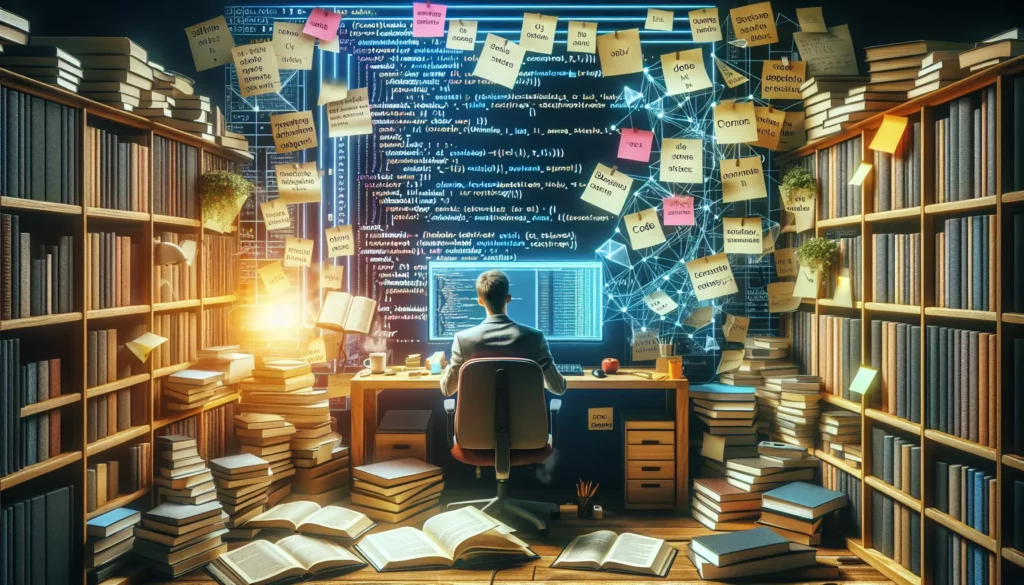
In the vast landscape of coding education and programming skills development, one often overlooked yet crucial aspect is the ability to read and understand documentation. As aspiring programmers progress from beginner-level coding to preparing for technical interviews at major tech companies, the importance of documentation becomes increasingly apparent. This article will explore why reading documentation is essential for mastering syntax and how it contributes to overall programming proficiency.
The Foundation of Programming Knowledge
Documentation serves as the foundation of programming knowledge. It is the primary source of information for understanding the intricacies of a programming language, framework, or library. While interactive coding tutorials and AI-powered assistance tools like those offered by AlgoCademy are invaluable for learning, documentation provides a comprehensive and authoritative reference that these resources often build upon.
Accurate and Up-to-Date Information
One of the key advantages of official documentation is its accuracy and currency. Programming languages and frameworks evolve rapidly, and documentation is typically updated to reflect the latest changes, features, and best practices. By regularly consulting documentation, developers can stay informed about new syntax, deprecated methods, and optimal coding patterns.
Comprehensive Coverage
Documentation often covers a wide range of topics, from basic syntax to advanced features. This comprehensive coverage allows developers to explore the full capabilities of a language or framework, discovering features they may not have encountered in tutorials or coding exercises.
Enhancing Problem-Solving Skills
Reading documentation is not just about memorizing syntax; it’s about developing problem-solving skills. As developers encounter challenges in their coding projects, documentation becomes a valuable resource for finding solutions and understanding the underlying concepts.
Learning to Navigate Complex Information
Documentation often contains a wealth of information, and learning to navigate it efficiently is a skill in itself. This skill translates directly to improved problem-solving abilities, as developers become adept at quickly locating relevant information and applying it to their specific coding challenges.
Understanding Context and Best Practices
Documentation often provides context for when and how to use certain features or methods. This context is crucial for developing a deeper understanding of programming concepts and best practices. By reading documentation, developers learn not just the “how” but also the “why” behind certain syntactical choices.
Preparing for Technical Interviews
For those aspiring to work at major tech companies, often referred to as FAANG (Facebook, Amazon, Apple, Netflix, Google), a solid grasp of documentation reading skills can be a significant advantage during technical interviews.
Demonstrating In-Depth Knowledge
Interviewers often appreciate candidates who can discuss language features and syntax with depth and precision. This level of knowledge is often best acquired through careful study of official documentation, allowing candidates to speak confidently about various programming concepts.
Adapting to New Technologies
Technical interviews may involve working with unfamiliar libraries or frameworks. The ability to quickly read and understand documentation becomes crucial in these situations, demonstrating adaptability and learning agility to potential employers.
Practical Applications of Documentation Reading
To illustrate the practical benefits of reading documentation, let’s consider a few examples of how it can enhance coding skills and syntax mastery.
Example 1: Understanding Function Parameters
Consider a Python function from the random
module:
import random
random_number = random.randint(1, 10)
While this code might seem straightforward, reading the documentation for random.randint()
reveals important details:
random.randint(a, b)
Return a random integer N such that a <= N <= b. Alias for randrange(a, b+1).
This documentation clarifies that the upper bound is inclusive, which might not be immediately apparent from the function name alone. Understanding these nuances is crucial for writing accurate and predictable code.
Example 2: Exploring Advanced Features
Documentation often reveals advanced features that can significantly improve code efficiency. For instance, consider Python’s list comprehensions:
squares = [x**2 for x in range(10)]
While many tutorials cover basic list comprehensions, the official Python documentation delves into more complex uses, such as nested list comprehensions:
matrix = [
[1, 2, 3, 4],
[5, 6, 7, 8],
[9, 10, 11, 12],
]
transposed = [[row[i] for row in matrix] for i in range(4)]
Understanding these advanced features through documentation study can lead to more elegant and efficient code solutions.
Example 3: Debugging with Documentation
When encountering errors, documentation can be a valuable debugging tool. Consider this JavaScript example:
let numbers = [1, 2, 3, 4, 5];
let sum = numbers.reduce((a, b) => a + b);
console.log(sum); // Output: 15
let emptyArray = [];
let emptySum = emptyArray.reduce((a, b) => a + b);
// Throws: TypeError: Reduce of empty array with no initial value
The error in the second reduce operation might be puzzling at first. However, consulting the MDN Web Docs for Array.prototype.reduce()
reveals:
If the array is empty and no initialValue is provided, a TypeError will be thrown.
This information helps in understanding the error and how to fix it by providing an initial value:
let emptySum = emptyArray.reduce((a, b) => a + b, 0); // Now works correctly
Strategies for Effective Documentation Reading
To maximize the benefits of reading documentation, consider the following strategies:
1. Start with the Basics
Begin by familiarizing yourself with the structure and organization of the documentation. Most documentation follows a logical structure, often starting with installation and basic concepts before moving on to more advanced topics.
2. Use the Search Function
Most online documentation includes a search feature. Learn to use it effectively to quickly find specific information about functions, methods, or concepts you’re interested in.
3. Read Examples and Code Snippets
Pay close attention to the examples and code snippets provided in the documentation. These often demonstrate practical applications of the concepts being discussed and can provide valuable insights into best practices.
4. Explore Related Topics
Don’t limit yourself to just the specific function or method you’re looking up. Take time to explore related topics and concepts. This broader understanding can lead to more creative and efficient coding solutions.
5. Practice Implementation
After reading about a new concept or feature in the documentation, try implementing it in your own code. This hands-on practice helps reinforce your understanding and reveals any areas that might need further clarification.
6. Keep Documentation Handy
Bookmark official documentation pages for quick reference. Many integrated development environments (IDEs) also offer plugins that provide easy access to documentation directly within the coding environment.
Overcoming Common Challenges in Reading Documentation
While documentation is an invaluable resource, it can sometimes be challenging to navigate, especially for beginners. Here are some common challenges and how to overcome them:
Challenge 1: Technical Jargon
Documentation often uses technical terms that may be unfamiliar to newcomers.
Solution: Keep a glossary of terms handy and gradually build your technical vocabulary. Don’t hesitate to look up unfamiliar terms, as understanding them will enhance your overall comprehension of programming concepts.
Challenge 2: Overwhelming Amount of Information
Comprehensive documentation can sometimes feel overwhelming, especially for large frameworks or libraries.
Solution: Start with the “Getting Started” or introduction sections to gain a broad overview. Then, focus on specific areas relevant to your current learning goals or project needs. Gradually explore other sections as your knowledge expands.
Challenge 3: Lack of Context
Sometimes, documentation may assume prior knowledge, making it difficult to understand certain concepts in isolation.
Solution: Use a combination of resources. While documentation provides detailed information, tutorials and courses like those offered by AlgoCademy can provide the necessary context and practical applications to help you better understand the documentation.
The Role of Documentation in Different Programming Paradigms
Documentation plays a crucial role across various programming paradigms, each with its unique focus and requirements.
Object-Oriented Programming (OOP)
In OOP, documentation is essential for understanding class hierarchies, inheritance, and method interactions. It often includes detailed descriptions of class methods, properties, and their usage.
Example from Python’s datetime
module documentation:
class datetime(date):
"""A combination of a date and a time. Attributes: year, month, day, hour, minute, second, microsecond, and tzinfo."""
def now(cls, tz=None):
"""Return the current local date and time."""
# Implementation details...
This documentation succinctly describes the datetime
class and its now()
method, providing crucial information for developers working with date and time in Python.
Functional Programming
In functional programming, documentation often focuses on describing pure functions, their inputs, outputs, and any side effects. It may also cover concepts like higher-order functions and immutability.
Example from JavaScript’s Array.prototype.map() documentation:
The map() method creates a new array populated with the results of calling a provided function on every element in the calling array.
const array1 = [1, 4, 9, 16];
// Pass a function to map
const map1 = array1.map(x => x * 2);
console.log(map1);
// Expected output: Array [2, 8, 18, 32]
This documentation clearly explains the purpose and behavior of the map()
function, which is crucial in functional programming paradigms.
Documentation and API Integration
As developers progress in their careers, they often work with various APIs (Application Programming Interfaces). Understanding how to read and interpret API documentation becomes a critical skill.
RESTful API Documentation
RESTful API documentation typically includes:
- Endpoint descriptions
- Request methods (GET, POST, PUT, DELETE, etc.)
- Request parameters and headers
- Response formats and status codes
- Authentication requirements
Example of a typical API documentation entry:
GET /api/users/{id}
Description: Retrieves a user by their ID.
Parameters:
- id (path, required): The unique identifier of the user.
Response:
200 OK
{
"id": 1,
"name": "John Doe",
"email": "john@example.com"
}
404 Not Found
{
"error": "User not found"
}
This documentation provides all the necessary information for a developer to understand how to use this specific API endpoint, including the expected request format and possible responses.
The Future of Documentation in Programming
As programming languages and development practices evolve, so too does the nature of documentation. Several trends are shaping the future of documentation in the programming world:
1. Interactive Documentation
Many modern documentation platforms now include interactive elements, allowing developers to test code snippets directly within the documentation. This hands-on approach enhances learning and understanding.
2. AI-Assisted Documentation
Artificial Intelligence is being increasingly used to generate and maintain documentation. AI can help keep documentation up-to-date, suggest improvements, and even provide contextual help based on a developer’s coding patterns.
3. Community-Driven Documentation
Open-source projects often rely on community contributions to improve and expand their documentation. This collaborative approach ensures that documentation remains relevant and comprehensive.
4. Video and Multimedia Integration
As learning styles diversify, documentation is beginning to incorporate more video tutorials, interactive diagrams, and other multimedia elements to cater to different preferences in information consumption.
Conclusion: The Ongoing Importance of Documentation
In the journey from beginner-level coding to preparing for technical interviews at major tech companies, the ability to read and understand documentation remains a critical skill. It forms the backbone of deep programming knowledge, enhances problem-solving abilities, and prepares developers for the challenges of professional software development.
As platforms like AlgoCademy continue to innovate in coding education, providing interactive tutorials and AI-powered assistance, they complement rather than replace the need for proficient documentation reading skills. The combination of structured learning environments and the ability to dive deep into official documentation creates well-rounded developers capable of tackling complex coding challenges.
Remember, mastering syntax is not just about memorizing code structures; it’s about understanding the underlying principles, best practices, and the context in which different syntactical elements should be used. Documentation provides this crucial context, making it an indispensable tool in every programmer’s arsenal.
As you continue your programming journey, make documentation reading a regular part of your learning routine. Embrace it not as a chore, but as a gateway to deeper understanding and more efficient, elegant coding. With practice, you’ll find that the ability to quickly parse and apply information from documentation becomes second nature, propelling you towards programming mastery and success in your technical interviews and beyond.