Why Reading “Cracking the Coding Interview” Isn’t Enough Anymore
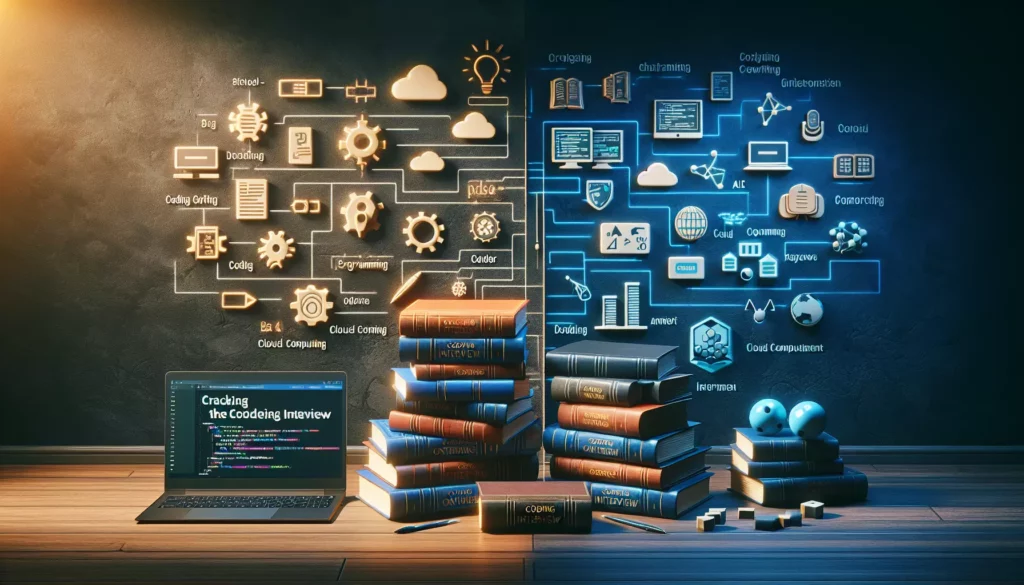
For years, “Cracking the Coding Interview” by Gayle Laakmann McDowell has been the go-to resource for software engineers preparing for technical interviews. This comprehensive guide has helped countless developers land roles at prestigious tech companies. However, as the tech industry evolves, relying solely on this book is no longer sufficient for interview preparation.
In this article, we’ll explore why reading “Cracking the Coding Interview” alone isn’t enough anymore, and what additional strategies and resources you need to succeed in today’s competitive technical interviews.
The Changing Landscape of Technical Interviews
When “Cracking the Coding Interview” was first published in 2008, the tech interview landscape was different. While the book has been updated over the years (currently in its 6th edition), several fundamental shifts in the industry have changed what it takes to succeed:
Increased Competition
The number of computer science graduates and self-taught programmers has exploded. According to the National Center for Education Statistics, the number of CS degrees awarded annually in the US has more than doubled since 2010. This surge means that simply knowing the content in popular books like “Cracking the Coding Interview” no longer gives candidates a significant edge.
Evolving Interview Formats
Many companies have moved beyond the traditional whiteboard coding interview. Today’s technical assessments might include:
- Take-home projects
- Pair programming sessions
- System design interviews
- Behavioral assessments
- Online coding assessments with automated evaluation
- Virtual whiteboarding
While “Cracking the Coding Interview” covers some of these formats, the breadth and depth of modern technical interviews require additional preparation.
Specialized Role Requirements
As the tech industry has matured, roles have become more specialized. Frontend engineers, backend developers, machine learning specialists, and mobile developers all face different types of technical questions. A one-size-fits-all preparation approach is no longer optimal.
What “Cracking the Coding Interview” Does Well
Before discussing its limitations, it’s important to acknowledge the book’s strengths:
Fundamentals Coverage
The book provides excellent coverage of core data structures and algorithms, which remain essential for any technical interview:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Bit Manipulation
- Recursion and Dynamic Programming
- Sorting and Searching
Problem-Solving Framework
The book teaches a structured approach to solving algorithmic problems, which remains valuable:
- Listen carefully to the problem
- Draw an example
- State a brute force approach
- Optimize your approach
- Walk through your algorithm
- Implement with clean code
- Test your code
Behavioral Interview Preparation
It offers solid advice for behavioral interviews, including how to structure responses using the STAR method (Situation, Task, Action, Result).
Where “Cracking the Coding Interview” Falls Short
Despite its strengths, the book has several limitations in today’s interview landscape:
Limited Coverage of Modern Technologies
The book focuses primarily on language-agnostic algorithms and data structures. However, modern interviews often include questions about:
- Cloud services (AWS, Azure, GCP)
- Containerization and orchestration (Docker, Kubernetes)
- Modern frameworks and libraries (React, Vue, Angular, TensorFlow)
- Microservices architecture
- Serverless computing
Insufficient System Design Coverage
While the book includes a chapter on system design, this topic has become increasingly important, especially for mid-level and senior roles. Modern system design interviews are more complex and require deeper knowledge of:
- Distributed systems
- Database scaling strategies
- Caching mechanisms
- Load balancing techniques
- Microservices vs. monoliths
- API design principles
Limited Interactive Learning
Reading about algorithms is not the same as implementing them. The book provides practice problems, but it lacks:
- Interactive coding environments
- Real-time feedback on solutions
- Peer code reviews
- Mock interview simulations
Static Content
Even with new editions, a book cannot keep pace with rapidly evolving interview trends. Companies continuously refine their interview processes, and a static resource cannot reflect these changes in real-time.
The Skills Gap: What Modern Interviews Require
Today’s technical interviews assess a broader range of skills than those covered in “Cracking the Coding Interview.” Let’s explore what else you need to know:
Practical Coding Experience
Many companies now evaluate how candidates approach real-world problems rather than textbook algorithms. This requires:
- Experience with version control (Git)
- Understanding of CI/CD pipelines
- Ability to write testable code
- Knowledge of software design patterns
- Familiarity with code review processes
Consider this example of a modern practical coding question:
/*
Design a rate limiter that restricts the number of API calls a user can make within a time window.
Implement both the class and a simple demo showing how it works with multiple users.
Include proper error handling and tests.
*/
class RateLimiter {
// Your implementation
}
This question tests not just algorithmic thinking but also API design, error handling, and testing strategies.
System Design Expertise
System design interviews have evolved significantly. Modern questions might include:
- Design a distributed file storage system like Dropbox
- Create a real-time chat application that scales to millions of users
- Design a recommendation system like Netflix
- Build a URL shortener with high availability
These questions require understanding components like load balancers, caching layers, database sharding, and message queues.
Language and Framework Proficiency
While “Cracking the Coding Interview” takes a language-agnostic approach, many companies now expect deep knowledge of specific languages and frameworks. For example:
- Frontend roles: Detailed React or Angular knowledge
- Backend roles: Proficiency in Spring Boot, Django, or Express.js
- Data science roles: Expertise in pandas, scikit-learn, or PyTorch
This code snippet illustrates a React-specific interview question that wouldn’t be covered in the book:
/*
Create a React component that fetches data from an API and displays it in a list.
Implement proper loading states, error handling, and pagination.
Use hooks and follow best practices for component structure.
*/
function DataList({ apiEndpoint }) {
// Your implementation
}
Domain-Specific Knowledge
Interviews for specialized roles require domain expertise beyond general algorithms:
- Machine learning engineers: ML algorithms, feature engineering, model evaluation
- Security engineers: Authentication protocols, common vulnerabilities, security best practices
- Mobile developers: Platform-specific guidelines, performance optimization, offline capabilities
Modern Technical Interview Preparation Strategies
To succeed in today’s technical interviews, you need a multi-faceted approach:
Interactive Problem Solving
Supplement book learning with interactive coding platforms:
- LeetCode: Offers thousands of problems with varying difficulty levels and company-specific problem sets
- HackerRank: Provides coding challenges and competitions
- AlgoCademy: Offers guided learning paths with AI-powered assistance and step-by-step guidance
- CodeSignal: Features real-world coding assessments similar to those used by companies
These platforms provide immediate feedback on your solutions and often include discussion forums where you can learn alternative approaches.
Mock Interviews
Practice with real human feedback:
- Pramp: Free peer-to-peer mock interviews
- interviewing.io: Anonymous mock interviews with experienced engineers
- Meetup groups: Many cities have coding interview practice meetups
- Study groups: Form a group with friends or colleagues to practice interviewing each other
The value of mock interviews cannot be overstated. They simulate the pressure of real interviews and provide feedback on both technical solutions and communication style.
System Design Resources
For system design preparation, consider these resources:
- System Design Primer: A comprehensive GitHub repository covering system design concepts
- Designing Data-Intensive Applications by Martin Kleppmann: A deep dive into data systems
- Grokking the System Design Interview: A course focused specifically on system design interviews
- YouTube channels like ByteByteGo and Tech Dummies that break down complex systems
Language and Framework Mastery
Deepen your knowledge of your primary programming language and relevant frameworks:
- Build side projects that showcase your skills
- Contribute to open-source projects
- Read documentation thoroughly
- Follow language-specific best practices
For example, if you’re a JavaScript developer, understanding closures, promises, and async/await is crucial:
// Understanding JavaScript closures
function createCounter() {
let count = 0;
return {
increment: function() {
count++;
return count;
},
decrement: function() {
count--;
return count;
},
getValue: function() {
return count;
}
};
}
const counter = createCounter();
console.log(counter.increment()); // 1
console.log(counter.increment()); // 2
console.log(counter.getValue()); // 2
Behavioral Interview Preparation
Modern technical roles require strong soft skills. Prepare for behavioral questions by:
- Documenting your achievements and challenges in the STAR format
- Practicing your responses to common questions
- Reflecting on your career goals and how they align with the company
- Preparing thoughtful questions for your interviewers
The Role of AI in Modern Interview Preparation
Artificial intelligence is transforming how developers prepare for technical interviews:
AI-Powered Code Reviews
Tools like GitHub Copilot and platforms like AlgoCademy provide AI-powered feedback on your code. These tools can:
- Identify inefficient algorithms
- Suggest optimizations
- Point out edge cases you might have missed
- Recommend cleaner implementations
Personalized Learning Paths
AI can analyze your strengths and weaknesses to create customized study plans, focusing on areas where you need the most improvement.
Interview Simulations
Some platforms offer AI-driven mock interviews that simulate real interview conditions, providing feedback on both technical solutions and communication style.
A Comprehensive Interview Preparation Plan
Based on the limitations of “Cracking the Coding Interview” and the demands of modern technical interviews, here’s a comprehensive 8-week preparation plan:
Weeks 1-2: Fundamentals Review
- Read relevant chapters from “Cracking the Coding Interview” for core algorithms and data structures
- Implement each data structure from scratch in your primary language
- Solve 2-3 easy problems daily on platforms like LeetCode or AlgoCademy
- Review language-specific features and best practices
Weeks 3-4: Problem-Solving Practice
- Increase to 2-3 medium difficulty problems daily
- Focus on core problem types: arrays, strings, trees, graphs, dynamic programming
- Begin weekly mock interviews with peers
- Start a system design study group or course
Weeks 5-6: Advanced Topics
- Tackle 1-2 hard problems daily
- Deep dive into system design concepts
- Practice explaining your thought process while coding
- Build a small project that showcases relevant skills for your target role
Weeks 7-8: Simulation and Refinement
- Conduct full interview simulations (coding + system design + behavioral)
- Review and optimize solutions to problems you found challenging
- Practice company-specific question sets
- Refine your behavioral interview responses
Case Studies: Modern Interview Success Stories
Let’s look at some examples of developers who went beyond “Cracking the Coding Interview” to succeed in technical interviews:
Case Study 1: Frontend Developer Role at a Major Tech Company
Sarah had read “Cracking the Coding Interview” thoroughly but struggled in her first few interviews. She realized that companies were asking detailed questions about React performance optimization, state management, and modern JavaScript features.
Her successful preparation strategy included:
- Building a complex React application with Redux
- Contributing to open-source React libraries
- Practicing frontend-specific interview questions
- Creating a portfolio that demonstrated her UI/UX sensibilities
This comprehensive approach helped her secure a role at a major tech company, where the interview focused heavily on practical React knowledge rather than traditional algorithm questions.
Case Study 2: Backend Engineer at a High-Growth Startup
Michael found that his startup interviews focused more on system design and practical coding than on algorithmic puzzles. His preparation included:
- Designing and deploying a microservices architecture
- Practicing system design by diagramming popular applications
- Learning about database scaling and optimization
- Becoming proficient with Docker and Kubernetes
During his successful interview, he was asked to design a notification system that could handle millions of users—a question that required practical distributed systems knowledge beyond what “Cracking the Coding Interview” covers.
Company-Specific Interview Trends
Different companies have distinct interview styles that require tailored preparation:
Google’s interviews still focus heavily on algorithms and data structures, but they’ve evolved to include:
- More emphasis on code quality and testing
- System design questions even for junior roles
- Stronger focus on problem-solving approach rather than just reaching the correct answer
Amazon
Amazon’s interviews are strongly aligned with their leadership principles:
- Behavioral questions based on leadership principles
- System design questions focused on scalability and reliability
- Practical coding challenges related to real Amazon problems
Startups
Startup interviews often emphasize:
- Practical coding skills over algorithmic puzzles
- Take-home projects that simulate actual work
- Cultural fit and adaptability
- Full-stack knowledge and ability to work across the technology stack
The Future of Technical Interviews
As we look ahead, several trends are shaping the future of technical interviews:
Project-Based Assessments
More companies are moving toward realistic, project-based assessments that better reflect day-to-day work. These might include:
- Contributing to an open-source project
- Building a feature for an existing application
- Debugging and refactoring problematic code
Pair Programming
Collaborative coding sessions are becoming more common, as they assess not just technical skills but also:
- Communication abilities
- Receptiveness to feedback
- Teamwork and collaboration
Specialized Assessments
As roles become more specialized, interviews are becoming more tailored to specific domains:
- Machine learning engineers might be asked to build and evaluate models
- Frontend developers might need to implement responsive designs and animations
- DevOps engineers might need to set up CI/CD pipelines or debug infrastructure issues
Conclusion: Beyond the Book
“Cracking the Coding Interview” remains a valuable resource, but it’s now just one component of a comprehensive interview preparation strategy. Today’s technical interviews require:
- Practical coding experience with modern technologies
- Deep knowledge of system design principles
- Specialized expertise relevant to your target role
- Strong soft skills and communication abilities
- Hands-on practice through interactive platforms and mock interviews
By recognizing the limitations of any single resource and embracing a multi-faceted preparation approach, you’ll be better equipped to succeed in modern technical interviews. The tech landscape continues to evolve, and so must your preparation strategies.
Remember that the ultimate goal of technical interviews is to find candidates who can solve real-world problems and contribute to the company’s success. Focus on developing practical skills, not just memorizing solutions to common interview problems. With the right preparation approach, you can demonstrate your value as a developer who can make an impact from day one.
Frequently Asked Questions
Is “Cracking the Coding Interview” still worth reading?
Yes, it remains a valuable foundation, especially for understanding core algorithms and data structures. However, it should be one component of a broader preparation strategy rather than your only resource.
How many LeetCode problems should I solve before interviews?
Quality matters more than quantity. Focus on understanding patterns across problems rather than solving hundreds of questions. Aim to solve 100-150 carefully selected problems that cover different data structures and algorithms.
How can I prepare for system design interviews as a junior developer?
Start by understanding the components of distributed systems (load balancers, caching, databases, etc.). Practice designing simpler systems like URL shorteners or chat applications. Review system architectures of popular applications and understand why certain design choices were made.
What’s the best way to showcase practical coding experience?
Build a portfolio of projects that demonstrate your skills. Contribute to open-source projects. Maintain an active GitHub profile with clean, well-documented code. Be prepared to discuss the technical challenges you faced and how you overcame them.
How do I balance breadth versus depth in my preparation?
Focus on depth in your primary technology stack and the core computer science fundamentals. For other areas, aim for sufficient breadth to demonstrate adaptability. Tailor this balance based on the specific requirements of your target roles.