Why Problem Decomposition is the Most Underrated Skill in Coding Interviews
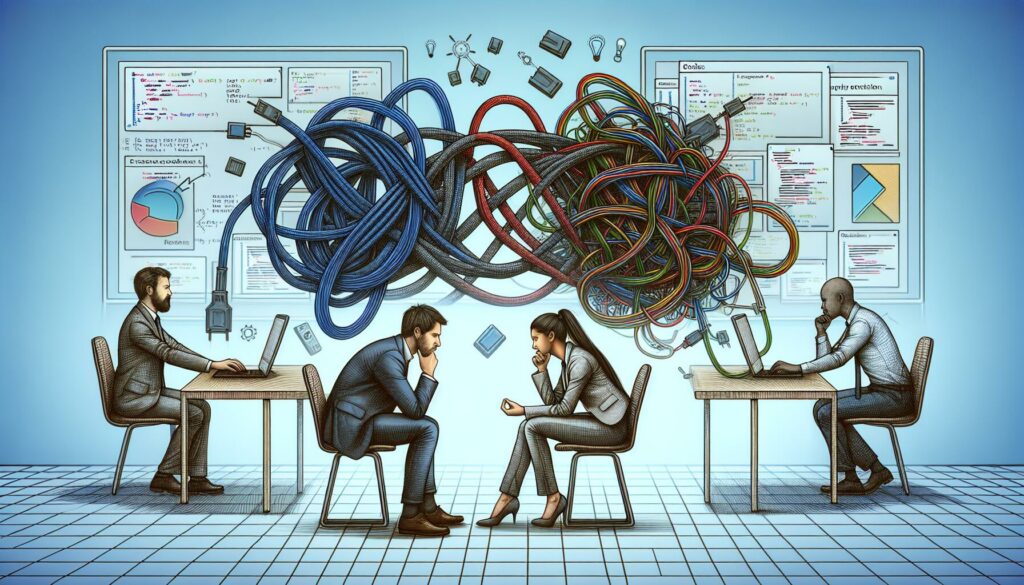
In the high-stakes world of coding interviews, especially those for coveted positions at major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), candidates often focus on mastering complex algorithms and data structures. While these are undoubtedly crucial, there’s a fundamental skill that’s often overlooked but can make or break your interview performance: problem decomposition. This article will explore why problem decomposition is the most underrated skill in coding interviews and how mastering it can significantly boost your chances of success.
What is Problem Decomposition?
Problem decomposition is the process of breaking down a complex problem into smaller, more manageable sub-problems. It’s a fundamental principle in computer science and a key aspect of algorithmic thinking. In the context of coding interviews, problem decomposition involves:
- Analyzing the given problem statement
- Identifying the main components or steps required to solve the problem
- Breaking these components down into smaller, more manageable tasks
- Organizing these tasks in a logical sequence
- Addressing each sub-problem individually before combining the solutions
This approach allows you to tackle complex problems systematically, making the overall solution more achievable and less overwhelming.
Why is Problem Decomposition Underrated?
Despite its importance, problem decomposition often takes a backseat to other skills in coding interview preparation. There are several reasons for this:
- Focus on Algorithmic Knowledge: Many candidates prioritize memorizing complex algorithms and data structures, believing that recalling the perfect algorithm is the key to interview success.
- Emphasis on Speed: The time-pressured nature of coding interviews can lead candidates to rush into coding without proper problem analysis.
- Overlooked in Traditional Education: While computer science curricula cover problem-solving, the specific skill of problem decomposition is often not explicitly taught or emphasized.
- Difficulty in Quantifying: Unlike algorithmic knowledge, which can be tested through specific problem types, problem decomposition skills are harder to measure and practice in isolation.
The Power of Problem Decomposition in Coding Interviews
Now, let’s explore why problem decomposition is so crucial in coding interviews:
1. Clarity of Thought
When you decompose a problem, you’re essentially creating a roadmap for your solution. This clarity of thought helps you:
- Understand the problem requirements better
- Identify potential edge cases early
- Communicate your approach clearly to the interviewer
For example, consider a problem where you need to implement a function to find the kth largest element in an unsorted array. A good problem decomposition might look like this:
- Clarify if we need to handle duplicate elements
- Decide on an approach: sorting vs. selection algorithm
- If sorting:
- Choose a sorting algorithm
- Sort the array
- Return the element at index (length – k)
- If using selection algorithm:
- Implement quickselect or a similar algorithm
- Partition the array until the kth largest element is found
- Handle edge cases (empty array, k larger than array size)
This decomposition immediately showcases your thought process and problem-solving approach to the interviewer.
2. Efficient Problem Solving
Breaking down a complex problem into smaller parts allows you to:
- Tackle each component individually, reducing cognitive load
- Identify reusable components or patterns
- Spot potential optimizations early in the process
Let’s take an example of implementing a basic calculator that can handle addition, subtraction, multiplication, and division of integers. A problem decomposition approach might look like this:
- Parse the input string into numbers and operators
- Handle operator precedence (multiplication/division before addition/subtraction)
- Implement individual functions for each operation
- Create a main function to coordinate the operations
- Handle edge cases (division by zero, invalid input)
By breaking it down this way, you can focus on implementing each part separately, making the overall problem much more manageable.
3. Improved Time Management
In a time-constrained interview, proper problem decomposition helps you:
- Estimate the time required for each component
- Prioritize critical parts of the solution
- Identify areas where you might need to make trade-offs
For instance, if you’re asked to implement a simplified version of a file system, you might break it down like this:
- Define the basic structure (files, directories)
- Implement file creation and deletion
- Implement directory creation and deletion
- Implement file/directory listing
- Handle path navigation
- (Optional) Implement file content reading/writing
This decomposition allows you to focus on the core functionality first and add additional features if time permits.
4. Better Error Handling and Edge Case Coverage
When you decompose a problem, you’re more likely to:
- Identify potential edge cases and error conditions
- Plan for error handling from the beginning
- Create more robust and reliable solutions
Consider a problem where you need to implement a function to validate IPv4 addresses. A good decomposition might include:
- Split the input string into octets
- Check the number of octets (should be exactly 4)
- For each octet:
- Check if it’s a valid integer
- Check if it’s in the range 0-255
- Check for leading zeros
- Handle edge cases (empty string, extra dots, non-numeric characters)
This approach ensures you cover all aspects of validation, including potential error conditions.
5. Easier Debugging and Testing
A well-decomposed solution is easier to debug and test because:
- Each component can be tested independently
- Errors are more likely to be isolated to specific components
- You can implement and test incrementally
For example, if you’re implementing a basic spell checker, you might decompose it like this:
- Implement a function to load a dictionary
- Implement a function to check if a word is in the dictionary
- Implement a function to generate possible corrections for a misspelled word
- Implement the main spell-checking function that uses the above components
This decomposition allows you to test each component separately, making it easier to ensure the correctness of your overall solution.
How to Improve Your Problem Decomposition Skills
Now that we understand the importance of problem decomposition, let’s look at some ways to improve this crucial skill:
1. Practice, Practice, Practice
Like any skill, problem decomposition improves with practice. Here are some ways to incorporate it into your coding practice:
- Before jumping into coding, spend time writing down your problem decomposition for each practice problem you attempt.
- Review and refine your decompositions after solving problems to identify areas for improvement.
- Practice decomposing problems even when you’re not coding, such as when reading about algorithms or system designs.
2. Study Well-Designed Systems and Algorithms
Analyzing how complex systems and algorithms are structured can provide insights into effective problem decomposition:
- Study the architecture of popular software systems and how they’re broken down into components.
- Analyze the structure of well-known algorithms and how they tackle complex problems step by step.
- Read code written by experienced developers and note how they organize and structure their solutions.
3. Use Visualization Techniques
Visual aids can be powerful tools for problem decomposition:
- Use flowcharts to map out the logical flow of your solution.
- Create mind maps to brainstorm and organize different aspects of the problem.
- Sketch diagrams to represent data structures or system components.
4. Collaborate and Discuss
Engaging with others can provide new perspectives on problem decomposition:
- Participate in coding workshops or hackathons where you can observe how others approach problem-solving.
- Engage in pair programming sessions to see how your peers break down problems.
- Discuss your problem decomposition approaches with mentors or more experienced developers for feedback.
5. Reflect on Real-World Systems
Look for examples of problem decomposition in everyday life:
- Analyze how complex tasks (like organizing an event) are broken down into smaller, manageable steps.
- Consider how large organizations are structured into departments and teams to handle complex operations.
- Observe how complex products (like smartphones) are composed of various subsystems and components.
Applying Problem Decomposition in Coding Interviews
Now that we’ve explored the importance of problem decomposition and how to improve this skill, let’s look at how to effectively apply it during coding interviews:
1. Start with Clarifying Questions
Before diving into problem decomposition, make sure you fully understand the problem:
- Ask about input formats and constraints
- Clarify any ambiguous terms or requirements
- Confirm the expected output and any performance expectations
2. Think Aloud
Verbalize your problem decomposition process to the interviewer:
- Explain how you’re breaking down the problem
- Discuss potential approaches for each sub-problem
- Share your reasoning for choosing specific methods or data structures
3. Use Pseudocode or Comments
Before writing actual code, outline your solution using pseudocode or comments:
// Function to find the kth largest element in an unsorted array
function findKthLargest(nums, k):
// 1. Handle edge cases
if nums is empty or k > len(nums):
return null
// 2. Choose approach: QuickSelect algorithm
// 3. Implement QuickSelect
// 3.1 Define partition function
function partition(left, right, pivotIndex):
// ... implementation details ...
// 3.2 Implement QuickSelect logic
function quickSelect(left, right, k):
// ... implementation details ...
// 4. Call QuickSelect with initial parameters
return quickSelect(0, len(nums) - 1, len(nums) - k)
This approach demonstrates your problem-solving process and makes it easier to implement the actual code.
4. Implement Incrementally
Based on your decomposition, implement your solution step by step:
- Start with core functionality and basic cases
- Add error handling and edge cases as you go
- Refine and optimize your solution if time permits
5. Test Your Solution
Use your problem decomposition to guide your testing:
- Test each component or sub-problem individually
- Use a variety of test cases, including edge cases you identified during decomposition
- Walk through your solution with a sample input to verify correctness
Conclusion
Problem decomposition is indeed the most underrated skill in coding interviews. While algorithmic knowledge and coding proficiency are important, the ability to break down complex problems into manageable parts is what truly sets apart exceptional problem solvers.
By mastering problem decomposition, you not only improve your performance in coding interviews but also enhance your overall programming skills. This foundational skill will serve you well throughout your career, enabling you to tackle increasingly complex challenges with confidence and clarity.
As you prepare for your next coding interview, remember to give problem decomposition the attention it deserves. Practice breaking down problems, communicate your thought process clearly, and approach each challenge systematically. With this powerful skill in your toolkit, you’ll be well-equipped to handle whatever coding challenges come your way, whether in interviews or in your day-to-day work as a software developer.
Happy coding, and may your problem decomposition skills lead you to success in your next coding interview!