Why Online Coding Platforms Aren’t Enough: Balancing Theory with Hands-On Practice
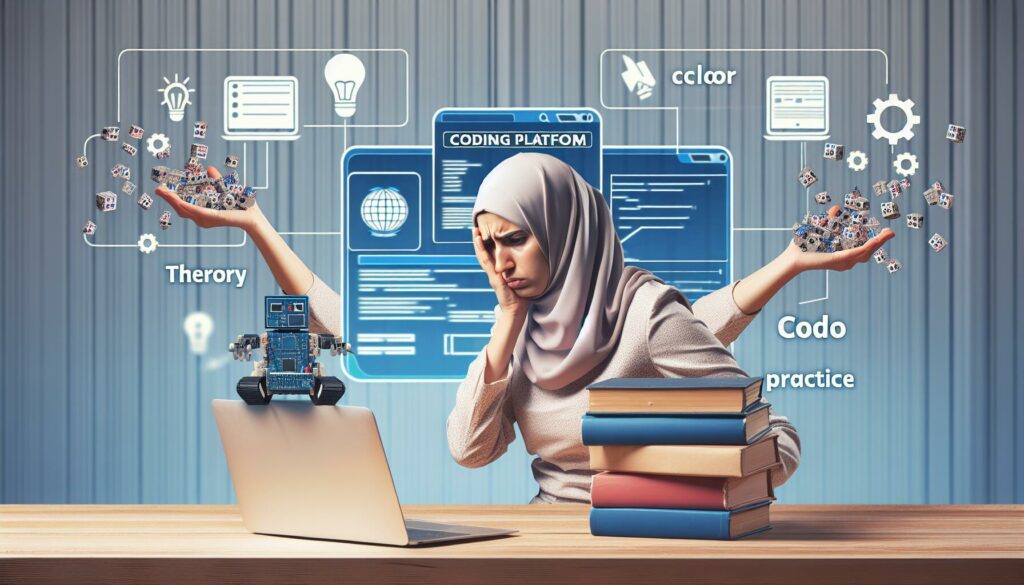
In the digital age, learning to code has become more accessible than ever before. With a plethora of online coding platforms available at our fingertips, aspiring programmers can start their journey with just a few clicks. However, as the demand for skilled developers continues to rise, it’s crucial to understand that relying solely on these platforms may not be enough to truly excel in the field. In this comprehensive guide, we’ll explore why online coding platforms, while valuable, should be complemented with a balanced approach that includes theoretical knowledge and hands-on practice.
The Rise of Online Coding Platforms
Online coding platforms have revolutionized the way people learn programming. These platforms offer a range of benefits that have made them incredibly popular among beginners and even experienced developers looking to expand their skill set:
- Accessibility: Learn from anywhere, at any time
- Interactive lessons: Engage with code in real-time
- Immediate feedback: Get instant results and error messages
- Gamification: Progress through levels and earn badges
- Community support: Connect with other learners and mentors
While these advantages are significant, they don’t tell the whole story of what it takes to become a proficient programmer.
The Limitations of Online Coding Platforms
Despite their benefits, online coding platforms have several limitations that can hinder a learner’s progress:
1. Oversimplification of Concepts
Many online platforms break down complex programming concepts into bite-sized, easily digestible lessons. While this approach can make learning less intimidating, it often fails to convey the depth and complexity of real-world programming challenges.
2. Lack of Comprehensive Theory
Online platforms typically focus on practical coding exercises, which is great for hands-on learning. However, they often neglect the theoretical foundations that are crucial for understanding why certain programming practices are used and how different concepts interconnect.
3. Limited Exposure to Large-Scale Projects
Most online coding exercises involve small, isolated problems. This approach doesn’t prepare learners for the complexities of working on large-scale projects with multiple components and collaborators.
4. Inadequate Preparation for Technical Interviews
While some platforms offer interview preparation modules, they often fall short in simulating the pressure and complexity of real technical interviews, especially those conducted by major tech companies.
5. Lack of Personalized Guidance
Although many platforms offer community forums and some level of mentorship, they can’t replace the personalized guidance and feedback that a dedicated instructor or mentor can provide.
The Importance of Theoretical Knowledge
To truly excel in programming, a solid foundation in computer science theory is essential. Here’s why:
Understanding the ‘Why’ Behind the Code
Theoretical knowledge helps you understand why certain algorithms and data structures are used in specific situations. This understanding is crucial for making informed decisions when designing and implementing solutions.
Problem-Solving Skills
A strong theoretical background enhances your ability to approach problems systematically and devise efficient solutions. It provides you with a toolkit of proven techniques and methodologies.
Adaptability
Technology evolves rapidly. A solid theoretical foundation makes it easier to adapt to new programming languages, frameworks, and paradigms as they emerge.
Optimization and Efficiency
Understanding the theoretical aspects of algorithms and data structures allows you to write more efficient code and optimize existing solutions.
The Value of Hands-On Practice
While theory is crucial, hands-on practice is equally important. Here’s why practical experience is invaluable:
Reinforcement of Concepts
Applying theoretical knowledge to real-world problems helps solidify your understanding of programming concepts.
Debugging Skills
Hands-on practice develops your ability to identify and fix errors in your code, a crucial skill for any programmer.
Project Management
Working on larger projects helps you understand how different components of a program interact and how to manage complex codebases.
Collaboration Skills
Many real-world projects involve working in teams. Hands-on practice, especially in group settings, helps develop collaboration and communication skills essential in professional environments.
Balancing Theory and Practice: The AlgoCademy Approach
At AlgoCademy, we recognize the importance of balancing theoretical knowledge with practical application. Our platform is designed to provide a comprehensive learning experience that addresses the limitations of traditional online coding platforms:
1. In-Depth Theoretical Foundations
We offer comprehensive modules on computer science fundamentals, including data structures, algorithms, and system design. These modules provide the theoretical background necessary for understanding complex programming concepts.
2. Interactive Coding Exercises
Our platform includes a wide range of coding exercises that allow learners to apply theoretical knowledge in practical scenarios. These exercises are designed to challenge learners and reinforce key concepts.
3. Real-World Project Simulations
We provide simulations of large-scale projects that mimic real-world scenarios. These projects help learners understand how different components of a program interact and how to manage complex codebases.
4. AI-Powered Assistance
Our AI-powered assistant provides personalized guidance and feedback, helping learners identify areas for improvement and offering tailored recommendations for further study.
5. Technical Interview Preparation
We offer a comprehensive interview preparation module that simulates the pressure and complexity of technical interviews at major tech companies. This module includes mock interviews, timed coding challenges, and feedback on both technical skills and communication abilities.
Implementing a Balanced Learning Approach
To get the most out of your programming education, consider implementing the following strategies:
1. Start with Fundamentals
Begin by building a strong foundation in computer science basics. This includes understanding data structures, algorithms, and programming paradigms. Resources like textbooks, online courses, and platforms like AlgoCademy can provide structured learning in these areas.
2. Practice Regularly
Set aside time each day for coding practice. This could involve solving coding challenges, working on personal projects, or contributing to open-source initiatives.
3. Work on Diverse Projects
Challenge yourself by working on projects that cover different aspects of programming. This could include web development, mobile apps, data analysis, or machine learning projects.
4. Collaborate with Others
Join coding communities, participate in hackathons, or contribute to open-source projects. These experiences will expose you to different coding styles and approaches while improving your collaboration skills.
5. Seek Mentorship
Connect with experienced programmers who can provide guidance, review your code, and offer insights into industry best practices.
6. Stay Updated
The tech industry evolves rapidly. Stay informed about new technologies, programming languages, and industry trends through blogs, podcasts, and tech conferences.
Code Example: Balancing Theory and Practice
Let’s look at a practical example of how theoretical knowledge and hands-on practice come together. Consider the problem of finding the nth Fibonacci number. We’ll implement this using both a naive recursive approach and a more efficient dynamic programming solution.
Naive Recursive Approach
def fibonacci_recursive(n):
if n <= 1:
return n
return fibonacci_recursive(n-1) + fibonacci_recursive(n-2)
# Test the function
print(fibonacci_recursive(10)) # Output: 55
This recursive solution is simple and intuitive, directly translating the mathematical definition of the Fibonacci sequence. However, it has a time complexity of O(2^n), making it inefficient for large values of n.
Dynamic Programming Approach
def fibonacci_dp(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
# Test the function
print(fibonacci_dp(10)) # Output: 55
This dynamic programming solution has a time complexity of O(n) and is much more efficient for large values of n. It demonstrates how theoretical knowledge of algorithm design techniques can lead to more efficient solutions.
The Role of AlgoCademy in Balanced Learning
AlgoCademy plays a crucial role in helping learners achieve a balance between theoretical knowledge and practical application:
1. Comprehensive Curriculum
Our curriculum covers both theoretical concepts and practical applications, ensuring learners have a well-rounded understanding of programming.
2. Interactive Learning
Our platform provides interactive coding exercises that allow learners to immediately apply theoretical concepts in a practical context.
3. Project-Based Learning
We offer project-based learning experiences that simulate real-world scenarios, helping learners understand how different components of a program interact.
4. Personalized Learning Paths
Our AI-powered system creates personalized learning paths, ensuring that each learner receives a balanced education tailored to their needs and goals.
5. Community and Mentorship
AlgoCademy provides access to a community of learners and experienced mentors, facilitating collaborative learning and providing opportunities for guidance and feedback.
Conclusion: The Path to Programming Mastery
While online coding platforms have made programming education more accessible than ever, they are not a complete solution on their own. True mastery in programming comes from a balanced approach that combines solid theoretical foundations with extensive hands-on practice.
By understanding the limitations of online platforms and actively working to complement them with comprehensive theoretical study and diverse practical experiences, aspiring programmers can develop the skills and knowledge necessary to excel in the field. Platforms like AlgoCademy, which emphasize this balanced approach, can play a crucial role in this journey.
Remember, becoming a proficient programmer is a continuous journey of learning and growth. Embrace both the theoretical and practical aspects of programming, seek out challenges that push your boundaries, and never stop exploring new technologies and concepts. With dedication, persistence, and a balanced approach to learning, you can achieve your goals and thrive in the exciting world of programming.