Why Memorizing Syntax Isn’t Teaching You How to Think in Code
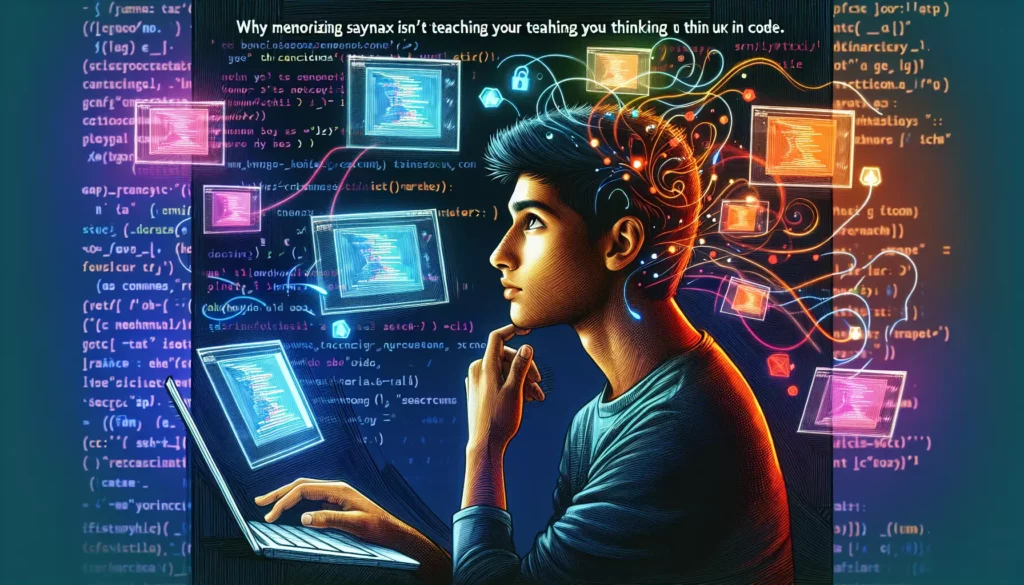
When many people start learning to code, they often focus on memorizing syntax, language rules, and specific commands. They spend hours drilling themselves on semicolons, brackets, and function definitions, believing that mastering these elements is the key to becoming a programmer. But there’s a fundamental problem with this approach: knowing syntax doesn’t mean you know how to code, just as knowing words doesn’t mean you can write a novel.
In this article, we’ll explore why memorizing syntax falls short of teaching you how to think in code, and what you should focus on instead to become a truly proficient programmer.
The Trap of Syntax Memorization
Let’s start with a common scenario: a new programmer spends weeks memorizing Python syntax. They know how to declare variables, write loops, and define functions. They can recite the exact syntax for list comprehensions and dictionary operations. But when faced with an actual problem to solve, they freeze. They have all the tools but don’t know how to use them together to build a solution.
This disconnect happens because:
- Syntax is just the vocabulary of programming, not the thought process
- Real programming requires problem decomposition and algorithmic thinking
- Memorization doesn’t teach you how to approach novel problems
- Programming languages evolve, but thinking patterns endure
As Albert Einstein reportedly said, “Education is not the learning of facts, but the training of the mind to think.” In programming, this couldn’t be more accurate.
What It Really Means to “Think in Code”
Thinking in code is about developing a computational mindset that allows you to:
- Break complex problems into manageable components
- Identify patterns and abstractions
- Think algorithmically and consider efficiency
- Understand data flow and program state
- Reason about code behavior systematically
This mindset transcends any specific programming language. A programmer who truly thinks in code can pick up new languages relatively quickly because they understand the underlying concepts and patterns.
Consider this example: A beginner who has memorized Python syntax might write:
def find_max(numbers):
max_number = numbers[0]
for number in numbers:
if number > max_number:
max_number = number
return max_number
They’ve correctly implemented a function to find the maximum value in a list. But a programmer who thinks in code would also consider:
- What happens if the list is empty?
- Is there a more efficient algorithm?
- Could this be generalized to work with other data types?
- How would this scale with very large inputs?
The Limitations of Syntax-Focused Learning
Learning to code by memorizing syntax is like trying to learn carpentry by memorizing the names and appearances of different tools without ever building anything. Here are some key limitations:
1. It Doesn’t Scale
Programming languages are vast, with extensive libraries and frameworks. Trying to memorize everything is not only impossible but also counterproductive. Professional developers regularly consult documentation, not because they’re inexperienced, but because it’s the efficient way to work.
2. It Creates a False Sense of Progress
Memorizing syntax can feel productive because it’s concrete and measurable. You either know how to write a for-loop or you don’t. But this creates an illusion of learning that can be shattered when facing real programming tasks.
3. It Fails to Build Problem-Solving Skills
The heart of programming is problem-solving. Syntax is merely the medium through which solutions are expressed. Without developing problem-solving skills, memorized syntax becomes a collection of disconnected facts.
4. It Doesn’t Prepare You for the Unexpected
Programming is full of unexpected challenges, edge cases, and bugs. Memorization doesn’t prepare you for troubleshooting or debugging, which require analytical thinking and systematic investigation.
5. It Makes Language Transitions Harder
Programmers often need to work with multiple languages. Those who learn by memorizing syntax tend to struggle when switching languages, while those who understand core concepts adapt more easily.
The Building Blocks of Computational Thinking
Instead of focusing solely on syntax, aspiring programmers should develop computational thinking skills. These are the mental tools that allow you to approach problems like a programmer:
Decomposition
Breaking down complex problems into smaller, more manageable parts is fundamental to programming. For instance, creating a web application can be decomposed into:
- Designing the database schema
- Building the backend API
- Creating the user interface
- Implementing authentication
- Handling form validation
Each of these can be further broken down into smaller tasks. This divide-and-conquer approach makes seemingly insurmountable problems tractable.
Pattern Recognition
Identifying common patterns allows programmers to apply known solutions to new problems. For example, recognizing that a problem involves searching, sorting, or graph traversal immediately suggests relevant algorithms and approaches.
Consider how many programming problems boil down to these common patterns:
- Iterating through collections
- Transforming data from one format to another
- Accumulating results
- Finding items that match certain criteria
Abstraction
Abstraction involves focusing on the essential features while ignoring irrelevant details. In programming, this means creating functions, classes, and modules that hide implementation details behind clean interfaces.
For example, when using a database library, you don’t need to know exactly how it communicates with the database server; you just need to understand its interface:
// You don't need to know how this works internally
const users = database.query("SELECT * FROM users WHERE active = true");
Algorithmic Thinking
Developing step-by-step solutions to problems is the essence of algorithmic thinking. This includes considering efficiency, correctness, and edge cases.
For instance, when tasked with finding duplicate elements in an array, you might consider:
- A nested loop approach (O(n²) time complexity)
- Sorting first, then checking adjacent elements (O(n log n))
- Using a hash set for O(n) time complexity
Each approach has trade-offs in terms of time complexity, space complexity, and implementation difficulty.
How to Develop “Code Thinking”
Now that we understand the importance of thinking in code rather than just memorizing syntax, how do we develop this mindset? Here are practical strategies:
1. Focus on Problem Solving, Not Language Features
Start with problems, not syntax. When learning, pick interesting challenges and then learn the syntax needed to solve them. Sites like LeetCode, HackerRank, and Project Euler offer problems that require computational thinking.
For example, instead of memorizing how to use dictionaries in Python, try solving a problem that naturally requires a dictionary, such as counting word frequencies in a text:
def count_words(text):
words = text.lower().split()
frequency = {}
for word in words:
if word in frequency:
frequency[word] += 1
else:
frequency[word] = 1
return frequency
2. Build Projects That Interest You
Nothing develops coding thinking like building real projects. Choose something you’re passionate about, whether it’s a game, a web app, or a data analysis tool. Projects force you to integrate multiple concepts and solve unexpected problems.
When building projects, you’ll naturally:
- Learn to break down large tasks into manageable pieces
- Develop debugging skills as you encounter and fix errors
- Learn to read documentation and apply new concepts
- Build a holistic understanding of how different parts of a system interact
3. Study Algorithms and Data Structures
Algorithms and data structures are the patterns of programming thought. Understanding them helps you recognize common problem types and solution approaches.
Start with fundamental structures like arrays, linked lists, stacks, queues, trees, and graphs. Then move on to algorithms for searching, sorting, and graph traversal. These concepts appear across all programming domains.
For example, understanding binary search allows you to efficiently find items in sorted collections:
function binarySearch(array, target) {
let left = 0;
let right = array.length - 1;
while (left <= right) {
const mid = Math.floor((left + right) / 2);
if (array[mid] === target) {
return mid;
} else if (array[mid] < target) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return -1; // Target not found
}
4. Read and Analyze Other People’s Code
Reading well-written code exposes you to different problem-solving approaches and coding styles. Study open-source projects, examine solutions to programming challenges, and review code written by experienced developers.
When reading code, ask yourself:
- What problem is this code solving?
- How is it structured and why?
- What alternative approaches could have been used?
- What can I learn from this code and apply to my own projects?
5. Practice Explaining Your Code
Teaching or explaining code to others (or even to yourself) forces you to articulate your thinking process. This reinforces your understanding and reveals gaps in your knowledge.
Try explaining your code using comments, documentation, or by walking through it with a colleague or friend. The “rubber duck debugging” technique, where you explain your code line by line to an inanimate object like a rubber duck, can be surprisingly effective.
// This function takes a string and returns the most frequent character
function findMostFrequentChar(str) {
// Create an object to store character frequencies
const charFrequency = {};
// Count occurrences of each character
for (const char of str) {
charFrequency[char] = (charFrequency[char] || 0) + 1;
}
// Find the character with the highest frequency
let mostFrequentChar = '';
let highestFrequency = 0;
for (const char in charFrequency) {
if (charFrequency[char] > highestFrequency) {
mostFrequentChar = char;
highestFrequency = charFrequency[char];
}
}
return mostFrequentChar;
}
The Role of Syntax in Learning to Code
This isn’t to say that syntax is unimportant. It’s the vocabulary through which we express our solutions. But there’s a more effective way to approach syntax learning:
Learn Syntax in Context
Instead of memorizing syntax in isolation, learn it as you need it to solve specific problems. This contextual learning makes the syntax more meaningful and memorable.
Understand Patterns Across Languages
Focus on understanding common patterns that appear across programming languages, such as:
- Control structures (if statements, loops)
- Functions and methods
- Data structures (arrays, objects/dictionaries)
- Object-oriented concepts (classes, inheritance)
Once you understand these patterns, adapting to the specific syntax of a language becomes much easier.
Use Tools to Your Advantage
Modern development environments provide features like syntax highlighting, auto-completion, and linting that reduce the need for perfect syntax recall. Use these tools to focus more on problem-solving and less on remembering exact syntax.
Recognize That Fluency Comes With Practice
Just as you learn a spoken language through use rather than memorization, programming syntax becomes second nature through regular practice. Don’t worry if you need to look things up frequently at first; this is normal and expected.
Case Studies: Syntax Knowledge vs. Code Thinking
To illustrate the difference between knowing syntax and thinking in code, let’s examine two approaches to solving the same problem.
Problem: Find the First Non-Repeating Character in a String
Approach 1: Syntax-Focused Solution
A programmer who has memorized syntax but lacks deeper understanding might write:
function firstNonRepeatingChar(str) {
for (let i = 0; i < str.length; i++) {
let isRepeated = false;
for (let j = 0; j < str.length; j++) {
if (i !== j && str[i] === str[j]) {
isRepeated = true;
break;
}
}
if (!isRepeated) {
return str[i];
}
}
return null;
}
This solution works but has O(n²) time complexity. The programmer has correctly used syntax (loops, conditionals, variables) but hasn’t thought deeply about efficiency or alternative approaches.
Approach 2: Code-Thinking Solution
A programmer who thinks in code might recognize that this is a frequency counting problem and approach it differently:
function firstNonRepeatingChar(str) {
// Count frequency of each character
const charCount = {};
for (const char of str) {
charCount[char] = (charCount[char] || 0) + 1;
}
// Find the first character with a count of 1
for (const char of str) {
if (charCount[char] === 1) {
return char;
}
}
return null;
}
This solution has O(n) time complexity. The programmer has recognized a more efficient approach by:
- Breaking the problem into two distinct phases
- Using a hash map to store frequency information
- Making a single pass through the string for each phase
Both solutions use correct syntax, but the second demonstrates computational thinking that leads to a more efficient algorithm.
From Syntax Knowledge to Code Fluency
The journey from syntax memorization to truly thinking in code involves several stages:
Stage 1: Syntax Awareness
At this stage, you’re learning the basic vocabulary of programming. You know how to declare variables, write simple functions, and use control structures. But you rely heavily on examples and documentation.
Stage 2: Syntax Fluency
With practice, you become more comfortable with syntax. You can write common constructs without looking them up, and you start to develop a sense of the language’s idioms and conventions.
Stage 3: Problem Decomposition
You begin to see problems in terms of their components. Rather than being overwhelmed by a large task, you can break it down into manageable pieces and tackle each one systematically.
Stage 4: Pattern Application
You recognize common patterns in problems and can apply appropriate solutions. When faced with a sorting problem, you know to consider quicksort, mergesort, or other algorithms based on the specific requirements.
Stage 5: Algorithmic Thinking
You can design algorithms from first principles, considering efficiency, correctness, and edge cases. You think about time and space complexity and make informed trade-offs.
Stage 6: Architectural Thinking
At the highest level, you can design entire systems, considering how components interact, planning for scalability, maintainability, and robustness. You can anticipate problems before they arise and design solutions that accommodate future changes.
The goal isn’t to skip the early stages but to move through them with an awareness that syntax is a means to an end, not the end itself.
Practical Exercises to Develop Code Thinking
Here are some exercises designed specifically to develop computational thinking rather than just syntax knowledge:
1. Mental Algorithm Tracing
Before coding a solution, try to trace through your algorithm mentally or on paper. For example, if you’re implementing a sorting algorithm, walk through it step by step with a small example:
// Original array: [5, 3, 8, 4, 2]
// Step 1: [3, 5, 8, 4, 2] (Compare 5 and 3, swap)
// Step 2: [3, 5, 8, 4, 2] (Compare 5 and 8, no swap)
// Step 3: [3, 5, 4, 8, 2] (Compare 8 and 4, swap)
// And so on...
This practice helps you understand the algorithm’s behavior and identify potential issues before writing any code.
2. Implement the Same Solution in Multiple Languages
After solving a problem in one language, try implementing the same solution in a different language. This forces you to focus on the underlying algorithm rather than the specific syntax.
For example, a binary search in JavaScript:
function binarySearch(arr, target) {
let left = 0;
let right = arr.length - 1;
while (left <= right) {
const mid = Math.floor((left + right) / 2);
if (arr[mid] === target) return mid;
if (arr[mid] < target) left = mid + 1;
else right = mid - 1;
}
return -1;
}
And the same algorithm in Python:
def binary_search(arr, target):
left = 0
right = len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
if arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
3. Solve Problems with Constraints
Challenge yourself by adding constraints to problems. For example:
- Solve a problem without using certain language features (no built-in sorting functions, no loops, etc.)
- Optimize for minimal memory usage or minimal runtime
- Solve a problem using a specific paradigm (functional, object-oriented, etc.)
These constraints force you to think creatively and deeply about algorithms rather than relying on memorized solutions.
4. Code Review Practice
Review code written by others (or your own past code) with a critical eye. Ask questions like:
- What is the time and space complexity?
- Are there edge cases that aren’t handled?
- Could this be more efficient or clearer?
- What assumptions does this code make?
This practice develops your analytical skills and exposes you to different problem-solving approaches.
5. Explain Algorithms Without Code
Practice explaining algorithms in plain language without using code. This helps you focus on the underlying logic rather than syntax details.
For example, explaining quicksort:
“Quicksort works by selecting a ‘pivot’ element and partitioning the array so that all elements less than the pivot come before it, and all elements greater than the pivot come after it. The pivot is now in its final sorted position. We then recursively apply this process to the sub-arrays before and after the pivot until the entire array is sorted.”
The Long-Term Benefits of Thinking in Code
Developing the ability to think in code rather than just memorizing syntax offers numerous long-term benefits:
Language Agnosticism
Programmers who think in code can move between languages with relative ease. They understand that languages are tools, each with strengths and weaknesses, and can choose the right tool for each job.
Problem-Solving Versatility
The ability to decompose problems, recognize patterns, and think algorithmically applies not just to programming but to many other fields and life situations. These skills make you a better problem solver in general.
Continuous Learning
The tech industry evolves rapidly, with new languages, frameworks, and tools emerging constantly. Programmers who focus on fundamental thinking skills rather than specific technologies can adapt to these changes more easily.
Career Longevity
While specific technologies come and go, the ability to think computationally remains valuable. This skill provides career resilience in a changing landscape.
Innovation Potential
True innovation often comes from applying computational thinking to new domains or combining ideas in novel ways. Programmers who think deeply about problems rather than just implementing known solutions are more likely to create innovative approaches.
Conclusion: Beyond Syntax to True Understanding
Memorizing syntax is a necessary but insufficient step in learning to code. True programming proficiency comes from developing computational thinking skills that allow you to decompose problems, recognize patterns, create abstractions, and design algorithms.
As you continue your programming journey, shift your focus from “How do I write this in language X?” to “How do I solve this problem computationally?” This mindset shift will not only make you a better programmer but will also make the learning process more engaging and rewarding.
Remember that professional programmers don’t memorize every detail of a language. They understand core concepts deeply and know how to find specific syntax information when needed. They’re valued for their problem-solving abilities, not their memorization skills.
So the next time you’re tempted to spend hours memorizing syntax, ask yourself: “Am I learning to write code, or am I learning to think in code?” The latter is what truly makes a programmer.
Further Resources for Developing Computational Thinking
If you’re inspired to focus more on thinking in code rather than just memorizing syntax, here are some resources to help you on that journey:
- Books: “Think Like a Programmer” by V. Anton Spraul, “Algorithms to Live By” by Brian Christian and Tom Griffiths
- Online Courses: Harvard’s CS50, MIT’s Introduction to Computational Thinking and Data Science
- Coding Challenge Platforms: LeetCode, HackerRank, Project Euler, Advent of Code
- Open Source Participation: Contributing to open-source projects exposes you to real-world code and collaborative problem-solving
Remember, becoming a programmer is not about accumulating syntax knowledge; it’s about developing a new way of thinking. Embrace the challenge, focus on problem-solving, and the syntax will follow naturally.