Why Memorizing Solutions Isn’t Building Problem Solving Skills
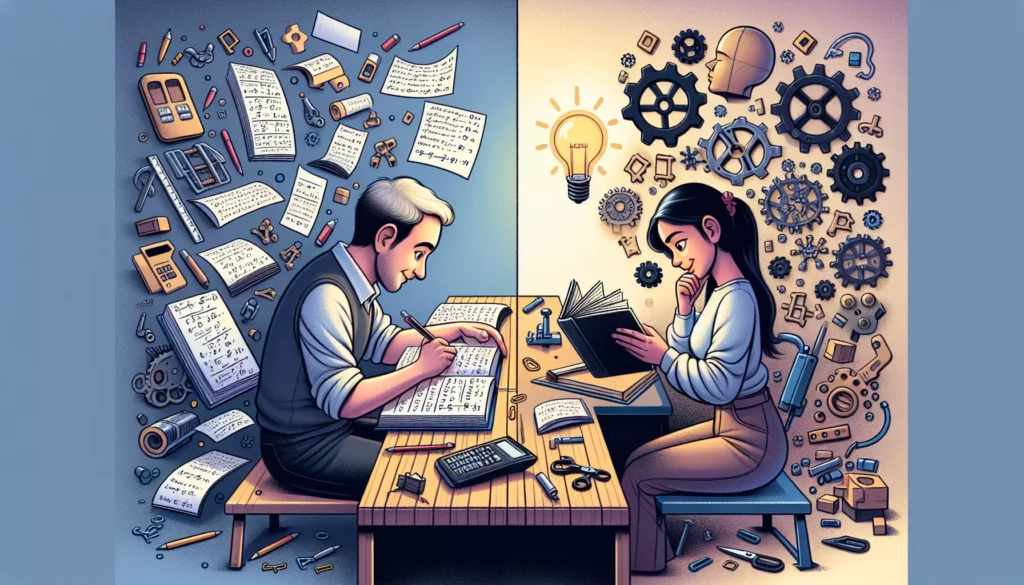
In the world of programming and technical interviews, there’s a common misconception that memorizing solutions to coding problems is the path to success. Many aspiring developers spend countless hours memorizing algorithms and solutions to popular interview questions, hoping to regurgitate them when faced with similar problems. However, this approach fundamentally misses the point of what technical interviews and real world programming actually test: your ability to think critically and solve problems.
At AlgoCademy, we’ve observed thousands of learners on their journey from coding beginners to successful technical interview candidates. One pattern stands out clearly: those who focus on understanding concepts rather than memorizing solutions invariably perform better in the long run. Let’s explore why memorization falls short and what approaches actually build lasting problem solving skills.
The False Promise of Memorization
When preparing for technical interviews, especially for major tech companies, it’s tempting to view the process as an exercise in memorization. After all, many companies draw from a relatively consistent pool of problem types. Why not just memorize the solutions to common problems like reversing a linked list or implementing a breadth first search?
The Limitations of Pure Memorization
Memorization might help you solve specific problems you’ve seen before, but it creates several critical limitations:
- Inflexibility: Real world problems rarely match textbook examples perfectly. Even a slight variation can completely stump someone who has only memorized solutions.
- Shallow Understanding: Memorizing without understanding means you can’t explain your reasoning or adapt your approach when necessary.
- Short Term Thinking: Knowledge gained through memorization tends to be transient, often disappearing shortly after the interview or test.
- Stress Vulnerability: Under the pressure of an interview, memorized solutions can be forgotten or misremembered, leaving you stranded.
Consider this common scenario: you’ve memorized the solution to a classic dynamic programming problem like the “Coin Change” problem. During an interview, you’re presented with a variation that asks for the combination of coins rather than just the count. If you’ve only memorized the solution without understanding the underlying principles, you’ll likely struggle to adapt.
What Technical Interviews Actually Test
Contrary to popular belief, technical interviews at top companies aren’t designed to test your memory. They’re structured to evaluate several key competencies:
Problem Solving Process
Interviewers are primarily interested in how you approach problems. They want to see:
- How you break down complex problems into manageable components
- Your ability to consider edge cases and constraints
- How you evaluate and optimize solutions
- Your communication throughout the problem solving process
A candidate who methodically works through a problem, even if they don’t reach the optimal solution, often impresses interviewers more than someone who immediately jumps to a memorized solution without demonstrating their thought process.
Adaptability and Learning
Technical interviews often include deliberate curveballs or follow up questions designed to test how you adapt when facing unfamiliar territory. Interviewers might ask:
- “What if we add this constraint?”
- “How would your solution change if the input was structured differently?”
- “Can you optimize this further for a specific case?”
These questions reveal whether you truly understand the underlying principles or have merely memorized a specific implementation.
Fundamental Knowledge
While specific algorithm implementations might vary, fundamental computer science concepts remain consistent. Interviewers assess your grasp of:
- Data structure tradeoffs and appropriate selection
- Algorithm complexity analysis (time and space)
- System design principles
- Code organization and readability
These fundamentals can’t be effectively memorized; they must be understood through practice and application.
The Real Cost of the Memorization Approach
Beyond simply being ineffective for interviews, the memorization approach can have lasting negative consequences on your development as a programmer:
Imposter Syndrome
Many developers who rely on memorization develop severe imposter syndrome when working in real environments. When faced with novel problems that don’t match their memorized solutions, they question their abilities and feel like frauds. This psychological burden can significantly impact career satisfaction and progression.
Stunted Growth
Problem solving is a muscle that grows with exercise. By relying on memorization, you’re depriving yourself of the very practice that builds this crucial skill. Over time, this creates a widening gap between your perceived and actual capabilities.
Reduced Creativity
Some of the most valuable contributions programmers make come from creative approaches to problems. Memorization trains your brain to follow established paths rather than exploring innovative solutions, potentially limiting your professional impact.
Career Ceiling
While memorization might help you squeak through an initial interview, it creates a ceiling on your career advancement. Senior roles require strong problem solving skills that can’t be faked through memorization. Eventually, this limitation becomes apparent and restricts your growth.
Building True Problem Solving Skills
If memorization isn’t the answer, what approach actually builds lasting problem solving abilities? At AlgoCademy, we’ve identified several key practices that consistently help learners develop genuine problem solving proficiency:
Focus on Patterns, Not Problems
Instead of memorizing specific problem solutions, learn to recognize common patterns and approaches. For example, understanding when and why to apply techniques like:
- Two pointer techniques
- Sliding window approaches
- Breadth first vs. depth first search
- Dynamic programming principles
- Divide and conquer strategies
When you understand these patterns, you can apply them flexibly to new problems rather than trying to match problems to memorized solutions.
Implement From First Principles
After solving a problem, challenge yourself to implement the solution again from scratch without referring to your previous code. This forces you to engage with the underlying logic rather than memorizing syntax.
For example, if you’ve just implemented a breadth first search algorithm for a graph problem, try implementing it again for a different problem without looking at your previous code. This reinforces your understanding of the core principles.
Verbalize Your Thought Process
Practice explaining your approach out loud, as you would in an interview. This “rubber duck debugging” technique helps solidify your understanding and identifies gaps in your reasoning. It also prepares you for the communication aspect of technical interviews.
A helpful exercise is to record yourself solving a problem while explaining your thought process. When you review the recording, you’ll often notice logical leaps or assumptions that weren’t fully reasoned through.
Analyze Multiple Solutions
For each problem you solve, explore alternative approaches. Ask yourself:
- What are the tradeoffs between different solutions?
- How does the optimal approach change if the constraints change?
- Which solution is most readable or maintainable?
This comparative analysis builds a deeper understanding than simply memorizing a single “correct” solution.
Deliberate Practice with Feedback
Random problem solving is less effective than structured practice that targets your specific weaknesses. Seek out problems that challenge your understanding of particular concepts, and actively seek feedback on your solutions from peers, mentors, or automated tools.
For instance, if you struggle with dynamic programming, focus specifically on problems that require this approach, starting with simpler examples and progressively tackling more complex ones.
Case Study: Two Approaches to Learning
Let’s examine how two different learners might approach the classic “Merge K Sorted Lists” problem, which is commonly asked in technical interviews:
The Memorization Approach
Alex decides to memorize the solution. They study the code for a heap based approach:
def mergeKLists(self, lists):
heap = []
for i, lst in enumerate(lists):
if lst:
heapq.heappush(heap, (lst.val, i, lst))
dummy = ListNode(0)
curr = dummy
while heap:
val, i, node = heapq.heappop(heap)
curr.next = node
curr = curr.next
if node.next:
heapq.heappush(heap, (node.next.val, i, node.next))
return dummy.next
Alex memorizes this solution and successfully reproduces it in a practice session. However, when faced with a variation asking to merge K sorted arrays instead of linked lists, they struggle to adapt their memorized solution.
The Understanding Approach
Jamie takes a different approach. Instead of memorizing the code, they focus on understanding the problem:
- They recognize this as a problem of merging multiple sorted structures
- They consider multiple approaches:
- Merging lists two at a time (analyzing the time complexity)
- Using a min heap to efficiently find the next smallest element
- Divide and conquer by merging pairs of lists recursively
- They implement the heap solution after understanding why it’s efficient
- They analyze the time and space complexity of their solution
When faced with the array variation, Jamie easily adapts their approach because they understand the core principle: using a heap to efficiently find the next element to add to the result.
The Difference in Outcomes
Six months later, Alex has forgotten the exact implementation and needs to re memorize it before interviews. Jamie, however, can still solve the problem and its variations because they internalized the underlying pattern rather than the specific code.
Effective Learning Strategies for Long Term Success
Based on our observations of successful learners, here are concrete strategies to build lasting problem solving skills:
The Feynman Technique
Named after physicist Richard Feynman, this technique involves explaining concepts in simple terms as if teaching someone else. This process reveals gaps in your understanding and forces you to clarify your thinking.
For programming concepts, try explaining an algorithm like quicksort to someone with no technical background. If you can make it understandable without resorting to jargon, you likely have a solid grasp of the concept.
Spaced Repetition
Instead of cramming, review concepts at increasing intervals over time. This approach, backed by cognitive science research, leads to stronger long term retention than intensive short term study.
For example, after learning about graph algorithms, review them after 1 day, then 3 days, then a week, then two weeks. This spacing helps cement the concepts in long term memory.
Interleaved Practice
Rather than focusing intensively on one topic at a time, mix different problem types and concepts in your practice sessions. This approach creates more challenging learning conditions but leads to better transfer of skills to new situations.
Instead of doing 10 binary tree problems in a row, mix in array problems, dynamic programming challenges, and string manipulation exercises. This variety forces your brain to select the appropriate technique rather than simply applying the same approach repeatedly.
Elaborative Interrogation
When learning a new concept or solution, ask yourself “why” questions:
- Why is this approach more efficient?
- Why would this data structure be preferred over alternatives?
- Why does this edge case require special handling?
This questioning deepens your understanding beyond simply knowing the steps of an algorithm.
Retrieval Practice
Instead of passively reviewing solutions, actively test yourself by attempting to solve problems from memory. This process strengthens neural pathways and improves recall under pressure.
After solving a problem, wait a few days, then try to solve it again without referring to your previous solution. This active recall is more effective than simply re reading your code.
Common Objections and Counterarguments
Despite the evidence against memorization as a primary learning strategy, many learners still defend this approach. Let’s address some common objections:
“But Many Interview Problems Are Standard Algorithms”
While it’s true that some interview questions involve implementing standard algorithms like breadth first search or binary search, interviewers are typically more interested in your understanding of when and how to apply these algorithms than in perfect recall of their implementation.
Understanding the principles behind these algorithms allows you to implement them correctly even if you don’t remember every detail. More importantly, it enables you to recognize when they’re applicable to novel problems.
“I Don’t Have Time to Deeply Understand Everything”
Time constraints are real, especially when preparing for interviews on a deadline. However, trying to memorize solutions without understanding is usually a false economy that costs more time in the long run.
A more efficient approach is to focus on deeply understanding a smaller set of fundamental patterns and techniques that can be applied broadly, rather than superficially memorizing many specific solutions.
“Memorization Worked for My Exams in School”
Academic exams often reward memorization more than technical interviews do. In school, problems are typically presented in the context of the relevant chapter or lecture, providing implicit hints about which technique to use.
Technical interviews deliberately avoid these contextual clues, requiring you to identify the appropriate approach from scratch. This fundamental difference makes memorization less effective in interview contexts than it might have been in academic settings.
“Some People Just Aren’t Good at Problem Solving”
While natural aptitude varies, problem solving is fundamentally a skill that can be developed through practice, not an innate talent. Many successful developers report that their problem solving abilities improved dramatically with deliberate practice and appropriate learning techniques.
The belief that problem solving ability is fixed can become a self fulfilling prophecy that limits growth. Adopting a growth mindset and focusing on incremental improvement yields better results than assuming a fixed ceiling on your capabilities.
Implementing Better Learning Practices
Converting these principles into actionable practices can transform your learning journey. Here are concrete ways to implement more effective learning strategies:
Create a Structured Learning Plan
Rather than randomly solving problems, develop a structured curriculum that progressively builds your skills:
- Start with foundational data structures (arrays, linked lists, trees, graphs)
- Progress to basic algorithms (searching, sorting, traversal)
- Advance to more complex techniques (dynamic programming, backtracking)
- Practice applying these concepts to novel problems
This systematic approach ensures you’re building on solid foundations rather than attempting to memorize advanced solutions without understanding the basics.
Implement a Problem Solving Framework
Develop a consistent approach to problem solving that you apply to each new challenge:
- Understand the problem: Clarify requirements, constraints, and expected outputs
- Explore examples: Work through sample inputs and edge cases
- Break it down: Identify subproblems and potential approaches
- Solve step by step: Implement a solution, starting with a naive approach if necessary
- Review and optimize: Analyze time/space complexity and look for improvements
This framework forces you to engage deeply with each problem rather than immediately searching for a memorized solution.
Form a Study Group
Learning with peers provides multiple benefits:
- Explaining concepts to others reinforces your understanding
- Hearing different approaches expands your problem solving toolkit
- Regular meeting schedules create accountability
- Mock interviews provide realistic practice
A study group doesn’t need to be large; even one consistent study partner can significantly enhance your learning.
Maintain a Learning Journal
Document your problem solving journey by keeping notes on:
- Problems you’ve solved and your approaches
- Concepts you found challenging and how you overcame them
- Patterns you’ve identified across different problems
- Mistakes you’ve made and lessons learned
This reflective practice helps consolidate learning and reveals patterns in your progress over time.
Use Spaced Repetition Software
Tools like Anki can help implement effective spaced repetition for programming concepts. Rather than creating cards with complete solutions, focus on:
- Identifying which technique applies to which problem type
- Understanding the tradeoffs between different approaches
- Recognizing the time and space complexity of various algorithms
This approach reinforces conceptual understanding rather than rote memorization.
The Role of Practice Platforms and Resources
The right resources can significantly enhance your learning journey. Here’s how to leverage various platforms effectively:
Interactive Coding Platforms
Platforms like AlgoCademy provide structured learning paths that build skills progressively. When using these platforms:
- Complete all steps of each problem, including the explanation and analysis phases
- Revisit problems after a delay to test your understanding
- Use built in hints as a last resort rather than immediately looking at solutions
The guided approach of these platforms helps develop a systematic problem solving process.
Competitive Programming Sites
Sites like LeetCode, HackerRank, and Codeforces offer thousands of problems to practice. To use them effectively:
- Start with easier problems to build confidence
- Focus on understanding the solutions to problems you couldn’t solve
- Participate in contests to practice under time pressure
- Study multiple solutions to the same problem to broaden your perspective
These platforms are most valuable when used for practice after developing a solid understanding of core concepts.
Conceptual Resources
Books, courses, and videos that explain fundamental concepts provide the theoretical foundation for problem solving. When using these resources:
- Take active notes rather than passively consuming content
- Implement concepts as you learn them
- Create your own examples to test your understanding
- Relate new concepts to problems you’ve previously encountered
These resources help develop the mental models necessary for effective problem solving.
The Long Term View: Beyond the Interview
While interview preparation is often the immediate goal, the skills you develop through proper learning approaches pay dividends throughout your career:
Adapting to New Technologies
The technology landscape evolves rapidly, with new frameworks, languages, and paradigms emerging regularly. Developers who understand fundamental principles adapt more quickly than those who have memorized specific implementations.
When you understand the “why” behind programming concepts, you can transfer that knowledge to new contexts rather than starting from scratch with each technological shift.
Solving Novel Problems
In real world development, you’ll frequently encounter problems that don’t match any textbook example. Strong problem solving skills enable you to tackle these challenges confidently, breaking them down into manageable components and applying appropriate techniques.
This ability to navigate uncharted territory often distinguishes the most valuable developers on a team.
Growing into Leadership Roles
As you advance in your career, your value increasingly comes from helping others solve problems rather than solving every problem yourself. The deep understanding developed through proper learning approaches enables you to:
- Effectively mentor junior developers
- Make architectural decisions based on sound principles
- Evaluate technical tradeoffs with nuanced understanding
These capabilities are essential for technical leadership roles and cannot be developed through memorization.
Conclusion: Building a Sustainable Approach
The journey from coding beginner to confident problem solver isn’t about accumulating a library of memorized solutions. It’s about developing a robust mental toolkit that enables you to approach new challenges systematically and creatively.
By focusing on understanding patterns rather than memorizing implementations, you build skills that remain valuable throughout your career, not just during interviews. This approach may require more effort initially, but it creates a foundation for continuous growth rather than a temporary facade of competence.
The next time you’re tempted to memorize a solution, challenge yourself to understand it deeply instead. Ask why each component exists, how it relates to other problems you’ve solved, and how you might adapt it to different constraints. This deeper engagement is the true path to programming mastery.
Remember that building problem solving skills is a marathon, not a sprint. Each problem you solve thoughtfully, each concept you truly understand, and each mistake you learn from contributes to your growth as a developer. Embrace this iterative process, and you’ll develop capabilities that extend far beyond what memorization could ever provide.