Why Mastering Frameworks Isn’t Teaching You Fundamental Programming
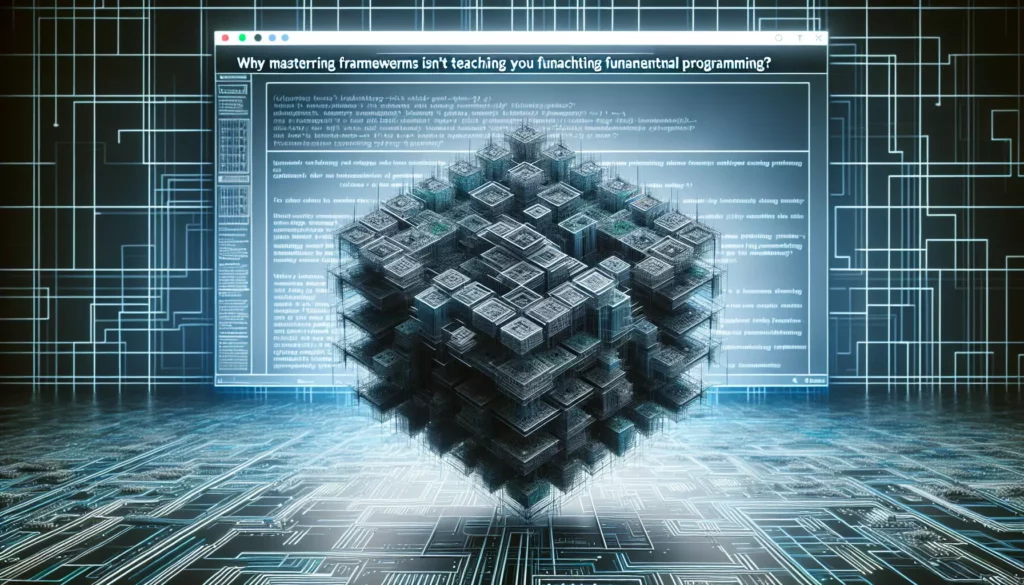
In today’s tech-driven world, the path to becoming a programmer often starts with learning the hottest frameworks. React, Angular, Django, Laravel—these names dominate job listings and coding bootcamp curricula. While frameworks offer a quick route to building functional applications, they create an illusion of programming proficiency that can mask fundamental gaps in core programming knowledge.
As someone who has interviewed hundreds of developers and helped many prepare for technical interviews at top tech companies, I’ve witnessed this phenomenon firsthand: developers who can build impressive applications using frameworks but struggle with basic algorithmic challenges or system design questions.
This article explores why mastering frameworks alone isn’t sufficient for becoming a well-rounded programmer, and why developing fundamental programming skills remains essential for long-term career success.
The Framework Dependency Trap
Modern frameworks are engineering marvels designed to solve common problems and speed up development. They abstract away complexity, offering pre-built solutions for routing, state management, data fetching, and more. This abstraction is both their greatest strength and their greatest weakness when it comes to learning programming.
How Frameworks Create False Confidence
Frameworks provide a comfortable scaffolding that can make beginners feel like they’re progressing rapidly. You follow the documentation, copy some examples, tweak a few parameters, and suddenly you have a working application. This immediate gratification is satisfying and creates a sense of accomplishment.
However, this comfort can be deceptive. Many developers find themselves in what I call the “framework dependency trap”—they become proficient with the tools but not with the underlying principles. When faced with problems outside the framework’s scope or when the framework itself changes (as they inevitably do), these developers often struggle to adapt.
Consider this common scenario: A developer who has spent a year working exclusively with React is asked in an interview to implement a simple algorithm like binary search or to explain how JavaScript’s event loop works. Despite having built several complex applications, they struggle with these fundamental concepts because their learning has been tightly coupled to framework-specific patterns and abstractions.
The Illusion of Transferable Knowledge
Another issue with framework-focused learning is the illusion that knowledge gained in one framework easily transfers to others. While there are certainly overlapping concepts, each framework has its own philosophy, patterns, and quirks.
A developer who understands React’s component-based architecture and unidirectional data flow might still struggle with Angular’s dependency injection system or Vue’s reactivity model. Without a solid understanding of the underlying JavaScript language, these transitions become unnecessarily difficult.
The same applies across language ecosystems. Moving from Rails to Django requires more than learning new syntax—it requires understanding how Ruby and Python differ in their approach to problems, their performance characteristics, and their idiomatic patterns.
What Frameworks Don’t Teach You
Frameworks are designed to help you build applications quickly, not to teach you computer science. Here are critical areas that framework-focused learning often neglects:
Algorithmic Thinking and Problem Solving
Frameworks rarely require you to think deeply about algorithms or data structures. Most provide optimized implementations for common operations, which is great for productivity but detrimental for learning.
When you use Array.sort()
in JavaScript without understanding how sorting algorithms work, you miss an opportunity to learn about time complexity, space complexity, and the tradeoffs between different approaches. These concepts are fundamental to writing efficient code, especially as applications scale.
Consider this example: A developer building a social media feed with React might use .filter()
and .map()
to process a list of posts without realizing that their approach has O(n²) complexity. As the application grows, this seemingly innocent code becomes a performance bottleneck.
Memory Management and System Resources
Frameworks often hide the details of memory management, resource allocation, and garbage collection. This abstraction is convenient but can lead to applications that consume excessive resources or experience mysterious performance issues.
In JavaScript frameworks like React, developers might create closures that unintentionally retain large objects in memory, causing memory leaks. Without understanding JavaScript’s memory model, identifying and fixing these issues becomes challenging.
Similarly, server-side frameworks like Express or Django abstract away details about connection pooling, thread management, and I/O operations. When performance problems arise, developers without this knowledge struggle to diagnose and resolve them effectively.
Understanding the Full Technology Stack
Modern web development involves multiple layers: frontend, backend, databases, APIs, networking, and infrastructure. Frameworks typically focus on one part of this stack, leaving gaps in developers’ understanding of how these pieces fit together.
A React developer might build beautiful UIs without understanding how HTTP requests work under the hood. A Django developer might create robust backend services without knowing how browsers render HTML and CSS. These knowledge gaps become apparent when debugging cross-stack issues or when optimizing application performance.
Full-stack understanding isn’t just about knowing multiple frameworks—it’s about understanding the principles that connect them. How does data flow from a database to a user’s screen? How do authentication systems maintain security across client-server boundaries? These questions require knowledge beyond any single framework.
The Career Limitations of Framework Dependence
Beyond the technical limitations, being overly reliant on frameworks can hamper your career growth in several ways:
Interview Challenges at Top Companies
Major tech companies like Google, Amazon, Facebook (Meta), Apple, and Netflix—often collectively referred to as FAANG—typically don’t focus on framework knowledge in their interviews. Instead, they assess fundamental computer science knowledge, problem-solving abilities, and system design skills.
These companies understand that frameworks come and go, but strong fundamentals enable engineers to learn and adapt to any technology. Their interview processes reflect this priority with questions about data structures, algorithms, system design, and programming language mechanics.
A developer who has invested exclusively in framework knowledge might excel in interviews at agencies or smaller companies that use those specific technologies, but will likely struggle when interviewing at companies that prioritize fundamental skills.
Vulnerability to Technology Changes
The tech industry moves quickly. Today’s popular framework might be tomorrow’s legacy system. Consider the fate of jQuery, which dominated web development for years before being largely replaced by newer frameworks and native browser APIs.
Developers who tied their identity and skills too closely to jQuery found themselves needing to relearn significant portions of their craft. Those who understood JavaScript fundamentals had a much easier transition to React, Vue, or other modern frameworks.
We’re seeing similar transitions today: Angular.js to Angular, React class components to hooks, traditional REST APIs to GraphQL. Each shift requires adaptation, and those with strong fundamentals adapt more easily.
Limited Problem-Solving Versatility
Framework-dependent developers often become specialists in solving problems within the constraints and patterns of their chosen framework. While specialization has value, it can limit versatility.
When faced with novel problems that don’t fit neatly into framework patterns—or problems that span multiple technical domains—these developers may struggle to formulate effective solutions. Their toolbox contains primarily framework-specific tools rather than general-purpose problem-solving approaches.
This limitation becomes especially apparent in roles that require innovation or working at the boundaries of established technology, such as creating new developer tools, optimizing performance in critical systems, or integrating with emerging technologies.
Building a Strong Foundation: The Fundamentals That Matter
Instead of focusing exclusively on frameworks, aspiring developers should invest time in building a strong foundation of programming fundamentals. Here are the key areas that provide lasting value throughout your career:
Core Programming Language Proficiency
Before diving into frameworks, develop deep knowledge of at least one programming language. Understand not just the syntax but the underlying concepts and paradigms:
- Types and type systems (whether static or dynamic)
- Memory models and garbage collection
- Control flow and error handling
- Functions, closures, and scope
- Object-oriented principles or functional programming concepts
- Language-specific idioms and best practices
For web developers, JavaScript is often a natural choice for deep study. Consider this example of understanding JavaScript beyond the basics:
// Many developers use this pattern without fully understanding it
const button = document.querySelector('button');
button.addEventListener('click', function() {
console.log(this); // 'this' refers to the button element
});
// Versus an arrow function
button.addEventListener('click', () => {
console.log(this); // 'this' refers to the surrounding scope, not the button
});
Understanding these nuances helps you debug issues more effectively and write more intentional code, regardless of which framework you’re using.
Data Structures and Algorithms
Data structures and algorithms form the foundation of computer science for good reason—they provide systematic approaches to organizing and manipulating data efficiently.
Key data structures to understand include:
- Arrays and linked lists
- Stacks and queues
- Hash tables (maps/dictionaries)
- Trees and graphs
- Heaps
Essential algorithms and techniques include:
- Sorting and searching
- Recursion
- Dynamic programming
- Graph traversal
- Divide and conquer
Understanding these concepts helps you choose the right tool for each problem and analyze the efficiency of your solutions. For example, knowing when to use a hash table versus an array can make the difference between an application that scales well and one that collapses under load.
Here’s a simple example of how understanding data structures leads to better code:
// Inefficient approach: O(n²) time complexity
function findDuplicates(array) {
const duplicates = [];
for (let i = 0; i < array.length; i++) {
for (let j = i + 1; j < array.length; j++) {
if (array[i] === array[j] && !duplicates.includes(array[i])) {
duplicates.push(array[i]);
}
}
}
return duplicates;
}
// Efficient approach: O(n) time complexity using a hash map
function findDuplicatesEfficient(array) {
const seen = new Set();
const duplicates = new Set();
for (const item of array) {
if (seen.has(item)) {
duplicates.add(item);
} else {
seen.add(item);
}
}
return [...duplicates];
}
Computer Systems and Architecture
Understanding how computers work at a lower level provides valuable context for higher-level programming. Key concepts include:
- How the CPU executes instructions
- Memory hierarchy (registers, cache, RAM, disk)
- Processes, threads, and concurrency
- Operating system fundamentals
- Networking principles (TCP/IP, HTTP, etc.)
This knowledge helps you write more efficient code and debug complex issues. For example, understanding CPU caching can help you organize data structures to minimize cache misses, significantly improving performance in computation-intensive applications.
Similarly, knowing how HTTP works helps you design better APIs and understand the communication between frontend and backend systems, regardless of which frameworks you're using.
Software Design Principles
Good software isn't just about working code—it's about code that's maintainable, extensible, and understandable. Key design principles include:
- SOLID principles (Single Responsibility, Open/Closed, Liskov Substitution, Interface Segregation, Dependency Inversion)
- Design patterns (Factory, Observer, Strategy, etc.)
- Architectural patterns (MVC, MVVM, microservices, etc.)
- Testing methodologies
- Documentation practices
These principles transcend specific frameworks and languages. They represent accumulated wisdom about how to structure code to manage complexity and change over time.
For example, understanding the Single Responsibility Principle helps you create more modular, testable code in any framework:
// Poor design: mixing multiple responsibilities
function saveUser(user) {
// Validate user data
if (!user.name || !user.email) {
throw new Error('Invalid user data');
}
// Format data for storage
const formattedUser = {
name: user.name.trim(),
email: user.email.toLowerCase(),
createdAt: new Date()
};
// Save to database
database.users.insert(formattedUser);
// Send welcome email
const emailContent = `Welcome, ${formattedUser.name}!`;
emailService.send(formattedUser.email, 'Welcome', emailContent);
return formattedUser;
}
// Better design: separated responsibilities
function validateUser(user) {
if (!user.name || !user.email) {
throw new Error('Invalid user data');
}
return true;
}
function formatUserForStorage(user) {
return {
name: user.name.trim(),
email: user.email.toLowerCase(),
createdAt: new Date()
};
}
function saveUserToDatabase(formattedUser) {
return database.users.insert(formattedUser);
}
function sendWelcomeEmail(user) {
const emailContent = `Welcome, ${user.name}!`;
return emailService.send(user.email, 'Welcome', emailContent);
}
// Orchestration function
function registerUser(user) {
validateUser(user);
const formattedUser = formatUserForStorage(user);
const savedUser = saveUserToDatabase(formattedUser);
sendWelcomeEmail(savedUser);
return savedUser;
}
Balancing Frameworks and Fundamentals
The argument against framework dependence isn't an argument against using frameworks entirely. Frameworks exist for good reasons—they solve common problems, enforce consistency, and accelerate development. The key is finding the right balance between leveraging frameworks and developing fundamental skills.
Learning Approaches That Combine Both
Here are strategies for developing both framework proficiency and fundamental knowledge:
1. Learn the "Why" Behind Framework Features
When learning a framework, don't just memorize its API. Understand why it's designed the way it is and what problems it's solving. For example, when learning React, understand why immutable state management is important, not just how to use useState
.
Ask questions like:
- What problem does this feature solve?
- How would I implement this without the framework?
- What are the performance implications of this approach?
2. Implement Core Features from Scratch
One of the best ways to understand frameworks is to rebuild their core features yourself. You don't need to create a production-ready implementation, but the exercise of recreating functionality helps you understand the underlying challenges and solutions.
For example, try building:
- A simple component rendering system (like a mini-React)
- A basic router for single-page applications
- A state management solution (like a simplified Redux)
Here's a simplified example of implementing a basic reactive state system:
function createStore(initialState = {}) {
let state = initialState;
const listeners = new Set();
function getState() {
return {...state}; // Return a copy to prevent direct mutations
}
function setState(newState) {
state = {...state, ...newState};
listeners.forEach(listener => listener(state));
}
function subscribe(listener) {
listeners.add(listener);
return () => listeners.delete(listener);
}
return { getState, setState, subscribe };
}
// Usage example
const store = createStore({ count: 0 });
const unsubscribe = store.subscribe(state => {
console.log('State updated:', state);
});
store.setState({ count: store.getState().count + 1 }); // Logs: State updated: { count: 1 }
unsubscribe(); // Stop receiving updates
store.setState({ count: store.getState().count + 1 }); // No log output
3. Study Multiple Frameworks in the Same Domain
Learning multiple frameworks that solve similar problems helps you identify common patterns and distinguish between fundamental concepts and framework-specific implementations.
For example, if you know React, learn Vue or Svelte as well. Compare how they handle:
- Component composition
- State management
- Side effects
- Performance optimization
This comparative approach helps you build a mental model of the problem space rather than just memorizing framework-specific solutions.
4. Tackle Algorithm Challenges Regularly
Dedicate time to solving algorithm and data structure problems, even if your day-to-day work doesn't require them. Platforms like LeetCode, HackerRank, and AlgoCademy offer structured problem sets that help you develop algorithmic thinking.
Start with simpler problems and gradually increase difficulty. Focus on understanding the approach rather than memorizing solutions. For each problem:
- Analyze the time and space complexity
- Consider multiple approaches
- Identify the data structures that make the solution efficient
5. Build Projects Outside Your Comfort Zone
Challenge yourself with projects that force you to learn new concepts:
- Build a compiler or interpreter for a simple language
- Create a database from scratch
- Implement encryption algorithms
- Develop a simple operating system component
These projects might seem impractical for everyday work, but they provide invaluable insights into how computing systems function and improve your problem-solving abilities.
Finding the Right Balance for Your Career Stage
The ideal balance between framework knowledge and fundamentals varies depending on your career stage and goals:
For Beginners (0-1 Years Experience)
Focus on building a strong foundation while learning one framework deeply:
- Master a programming language before or alongside learning your first framework
- Study basic data structures and algorithms
- Understand HTTP and web fundamentals (for web developers)
- Learn one framework well enough to build complete applications
For Mid-Level Developers (1-3 Years Experience)
Deepen your understanding of both fundamentals and frameworks:
- Study advanced language features and patterns
- Learn a second framework in the same domain to compare approaches
- Develop expertise in relevant algorithms for your field
- Study system design and architecture patterns
For Senior Developers (4+ Years Experience)
Focus on broadening and deepening your knowledge:
- Study multiple programming paradigms
- Learn frameworks in different domains (e.g., frontend, backend, mobile)
- Develop expertise in system design and architecture
- Study performance optimization and scalability
How to Assess and Improve Your Fundamental Skills
If you've been primarily framework-focused, here are steps to assess and strengthen your fundamental programming skills:
Self-Assessment Questions
Ask yourself these questions to identify potential knowledge gaps:
- Can you implement common data structures (linked lists, trees, graphs) from scratch?
- Do you understand the time and space complexity of the algorithms you write?
- Can you explain how your programming language handles memory management?
- Do you understand the underlying protocols and technologies your applications use (HTTP, WebSockets, etc.)?
- Can you solve medium-difficulty algorithm problems without looking up solutions?
- Could you implement core features of your favorite framework if needed?
- Do you understand the tradeoffs between different architectural approaches?
If you answered "no" to several of these questions, you likely have opportunities to strengthen your fundamental skills.
Structured Learning Plan
Here's a structured approach to improving your fundamental programming skills:
1. Focus on One Language Deeply
Choose a language you're already familiar with and study it deeply:
- Read the language specification or comprehensive guides
- Study how standard libraries are implemented
- Learn language-specific performance optimization techniques
- Understand the runtime environment and execution model
2. Work Through a Data Structures and Algorithms Course
Numerous resources teach these fundamental concepts:
- Online courses from platforms like Coursera, edX, or AlgoCademy
- Books like "Introduction to Algorithms" by Cormen et al. or "Algorithms" by Sedgewick and Wayne
- Interactive platforms that combine theory with practice
The key is to implement the data structures and algorithms yourself, not just read about them.
3. Build a Project Without Frameworks
Challenge yourself to build a project using only the standard library of your language or minimal dependencies. This forces you to understand what frameworks are doing for you.
For web developers, try building:
- A simple server without Express or Django
- A frontend application without React or Vue
- A database interaction layer without an ORM
4. Join Algorithm Practice Groups
Regular practice with peers provides accountability and exposes you to different approaches:
- Join or form a study group that meets weekly to solve problems
- Participate in coding competitions
- Contribute to open-source projects that require algorithmic solutions
5. Study Computer Systems
Understanding how computers work improves your intuition about performance and behavior:
- Learn about operating systems and process management
- Study networking fundamentals and protocols
- Understand how databases work internally
- Learn about compiler design and language implementation
Resources for Building Fundamental Skills
Here are some high-quality resources for developing your fundamental programming skills:
Books:
- "Computer Systems: A Programmer's Perspective" by Bryant and O'Hallaron
- "Clean Code" by Robert C. Martin
- "Designing Data-Intensive Applications" by Martin Kleppmann
- "Cracking the Coding Interview" by Gayle Laakmann McDowell
- "The Algorithm Design Manual" by Steven Skiena
Online Courses and Platforms:
- MIT's Introduction to Algorithms (available on OCW and edX)
- Harvard's CS50: Introduction to Computer Science
- Princeton's Algorithms course on Coursera
- AlgoCademy's interactive algorithm tutorials
- Frontend Masters' courses on JavaScript fundamentals and computer science
Practice Platforms:
- LeetCode for algorithm practice
- HackerRank for diverse programming challenges
- Exercism for language-specific practice with mentorship
- Project Euler for mathematically oriented problems
Conclusion: Building a Career on Solid Foundations
Frameworks are powerful tools that enable developers to build complex applications quickly. They have their place in modern software development and learning them is valuable. However, treating frameworks as the primary focus of your learning journey is a mistake that can limit your growth and career potential.
The most successful developers understand that frameworks come and go, but fundamental programming skills endure. They use frameworks as tools to solve specific problems rather than defining their entire skill set around them.
By investing in a strong foundation of programming fundamentals—language proficiency, data structures and algorithms, system design, and software architecture—you build a knowledge base that transcends specific technologies and frameworks. This foundation enables you to:
- Learn new frameworks more quickly and effectively
- Solve problems that fall outside framework boundaries
- Debug complex issues that span multiple systems
- Design scalable, maintainable software architectures
- Adapt to the inevitable changes in technology landscape
- Pass technical interviews at top companies
The path to mastery in programming isn't about accumulating knowledge of as many frameworks as possible. It's about developing a deep understanding of the principles that underlie all software development and using that understanding to make informed choices about which tools to use for each problem.
As you continue your programming journey, strive for balance. Use frameworks to be productive, but invest in fundamental skills to be adaptable and innovative. This balanced approach will serve you well throughout your career, regardless of which technologies dominate the landscape in the years to come.
Remember: Frameworks teach you how to build applications in a specific way. Fundamentals teach you how to think about programming itself. Both are valuable, but the latter is what transforms you from a framework user into a true software engineer.