Why Maintaining Legacy Code Teaches More Than Building New Features
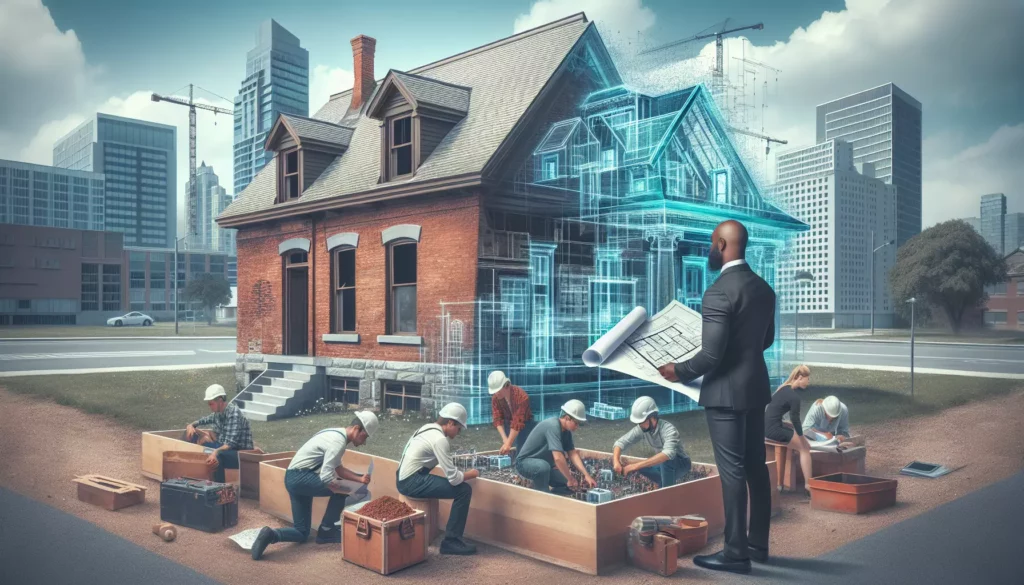
When developers envision their dream jobs, they often picture themselves crafting elegant new features using the latest technologies. The reality, however, often includes substantial time spent maintaining legacy code—systems written years ago, possibly with outdated practices, limited documentation, and accumulated technical debt. While maintaining legacy code might not seem as glamorous as greenfield development, it offers invaluable learning opportunities that can significantly accelerate your growth as a developer.
In this comprehensive guide, we’ll explore why working with legacy code can be more educational than always building new features, and how embracing this often-avoided aspect of development can transform you into a more well-rounded and capable software engineer.
The Hidden Value of Legacy Code Maintenance
Legacy code has a reputation problem. Many developers view it as a necessary evil—something to be tolerated rather than embraced. But what if we reframe this perspective? What if maintaining legacy code is actually one of the most educational experiences available to developers?
What Exactly Is Legacy Code?
Before diving deeper, let’s clarify what we mean by legacy code. While definitions vary, legacy code typically refers to:
- Code written by developers who are no longer with the organization
- Systems using outdated technologies or methodologies
- Applications with limited or outdated documentation
- Software that’s critical to business operations but difficult to modify
- Code with limited or no test coverage
Michael Feathers, in his influential book “Working Effectively with Legacy Code,” offers perhaps the most pragmatic definition: legacy code is simply code without tests. This definition highlights a key challenge: code that can’t be easily verified when changed.
Comprehensive Understanding of Complex Systems
One of the most significant benefits of maintaining legacy code is the deep understanding it provides of complex, real-world systems.
Learning to Navigate the Unknown
When you build a new feature from scratch, you control the architecture, design patterns, and implementation details. With legacy code, you must first understand what exists before making changes. This process of discovery and comprehension develops critical skills:
- Code archaeology: Piecing together how systems work without complete documentation
- System thinking: Understanding how components interact within a larger ecosystem
- Impact analysis: Predicting how changes might affect dependent systems
Consider a developer tasked with fixing a bug in a payment processing system. To make the fix, they must understand not just the immediate code, but how it interacts with authentication, user accounts, inventory management, and external payment providers. This forced exploration provides a comprehensive education in system architecture that’s difficult to gain when building isolated new features.
Seeing the Evolution of Software
Legacy code tells a story—of business requirements changing over time, of architectural decisions made under different constraints, and of the evolution of best practices. By studying legacy code, you witness the real-world lifecycle of software:
// Original implementation circa 2005
function processOrder(orderId) {
// Simple synchronous implementation
const order = database.getOrder(orderId);
const result = paymentGateway.processPayment(order.amount);
if (result.success) {
order.status = 'PAID';
database.updateOrder(order);
}
return result;
}
// Updated in 2010 to handle errors better
function processOrder(orderId) {
try {
const order = database.getOrder(orderId);
if (!order) {
logger.error('Order not found: ' + orderId);
return { success: false, error: 'ORDER_NOT_FOUND' };
}
const result = paymentGateway.processPayment(order.amount);
if (result.success) {
order.status = 'PAID';
database.updateOrder(order);
}
return result;
} catch (e) {
logger.error('Payment processing error: ' + e.message);
return { success: false, error: 'SYSTEM_ERROR' };
}
}
// Modified in 2015 to support async operations
async function processOrder(orderId) {
try {
const order = await database.getOrderAsync(orderId);
if (!order) {
logger.error(`Order not found: ${orderId}`);
return { success: false, error: 'ORDER_NOT_FOUND' };
}
const result = await paymentGateway.processPaymentAsync(order.amount);
if (result.success) {
order.status = 'PAID';
order.processedAt = new Date();
await database.updateOrderAsync(order);
}
return result;
} catch (e) {
logger.error(`Payment processing error: ${e.message}`);
return { success: false, error: 'SYSTEM_ERROR' };
}
}
This evolution teaches valuable lessons about how code adapts to changing requirements, technological advancements, and improved practices over time.
Technical Debt and Code Quality Insights
Legacy code often contains examples of technical debt—shortcuts taken to meet deadlines that now make the code harder to maintain. Working with such code provides practical lessons in code quality.
Recognizing Anti-Patterns
When you encounter difficulties maintaining legacy code, you’re often experiencing the consequences of anti-patterns firsthand. These concrete examples teach you what to avoid in your own code:
- God objects that try to do too much
- Copy-paste code duplication
- Excessive global state
- Deeply nested conditionals
- Magic numbers and strings
- Tight coupling between components
For example, encountering a 3000-line class that handles user authentication, permission checking, session management, and audit logging provides a visceral lesson in the importance of single responsibility and separation of concerns.
Appreciating Good Design
The flip side is also valuable—when you find parts of legacy code that remain maintainable despite years of changes, you gain insights into what constitutes genuinely good design:
// A well-designed component from 2008 that's still easy to maintain
class PaymentProcessor {
constructor(paymentGatewayService, configProvider, logger) {
this.paymentGateway = paymentGatewayService;
this.config = configProvider;
this.logger = logger;
}
async processPayment(amount, paymentMethod, currency = 'USD') {
this.logger.info(`Processing ${currency} ${amount} payment`);
// Validate inputs before proceeding
if (amount <= 0) {
throw new InvalidAmountError('Payment amount must be positive');
}
if (!this.isSupportedCurrency(currency)) {
throw new UnsupportedCurrencyError(`Currency not supported: ${currency}`);
}
// Process based on payment method
switch(paymentMethod.type) {
case 'CREDIT_CARD':
return this.processCreditCardPayment(amount, paymentMethod, currency);
case 'BANK_TRANSFER':
return this.processBankTransfer(amount, paymentMethod, currency);
default:
throw new UnsupportedPaymentMethodError();
}
}
// Additional methods...
}
This code demonstrates dependency injection, clear separation of concerns, input validation, and other design principles that have stood the test of time.
The Cost of Technical Debt
Perhaps most importantly, maintaining legacy code gives you a tangible sense of the real cost of technical debt. When you spend days untangling complex dependencies to make what should be a simple change, you internalize the importance of clean code in a way that reading books or articles cannot provide.
Problem-Solving Under Constraints
When building new features, you often have the luxury of choosing the best tools and approaches for the job. Legacy code maintenance forces you to solve problems under significant constraints.
Working Within Limitations
Legacy systems often come with limitations that cannot be easily changed:
- Outdated technology stacks
- Established architectural patterns
- Limited test coverage
- Production dependencies that can’t be disrupted
- Performance requirements for existing functionality
These constraints force creativity and pragmatism. For instance, if you need to add a feature to a monolithic application without disrupting its thousands of daily users, you might employ the Strangler Fig pattern to gradually introduce new components while maintaining compatibility with the existing system.
Balancing Idealism with Pragmatism
Working with legacy code teaches the crucial skill of balancing technical idealism with business pragmatism. You learn to:
- Identify when a complete rewrite is justified versus incremental improvement
- Make targeted refactoring that provides the most value
- Apply the “boy scout rule” (leave the code better than you found it) without getting lost in perfectionism
- Communicate technical trade-offs to non-technical stakeholders
This balance is essential for career advancement, as senior engineering roles require both technical excellence and business acumen.
Debugging and Diagnostic Skills
Legacy code maintenance is a masterclass in debugging and diagnostic skills, often more challenging than debugging new code.
Advanced Debugging Techniques
When troubleshooting issues in legacy systems, you often need to employ advanced debugging techniques:
- Log analysis across multiple systems
- Production debugging with minimal instrumentation
- Reproducing complex, intermittent issues
- Working with unfamiliar codebases
- Identifying root causes in deeply nested call stacks
For example, diagnosing a race condition in a legacy multi-threaded application might require careful analysis of thread dumps, log correlation, and creating minimal reproducible test cases.
Developing a Systematic Approach
Legacy code debugging teaches the importance of a systematic approach:
- Reproduce the issue consistently
- Narrow down the scope of potential causes
- Form hypotheses about what might be happening
- Test each hypothesis with minimal changes
- Verify the fix addresses the root cause, not just symptoms
These skills transfer directly to debugging any software system, making you a more effective developer regardless of whether you’re working with legacy or new code.
Business Domain Knowledge
Legacy code often encapsulates years of business domain knowledge and edge cases that may not be documented elsewhere.
Uncovering Business Rules
As you work with legacy code, you’ll discover business rules embedded in the implementation:
// This seemingly simple function contains critical business rules
function calculateOrderDiscount(order, customer) {
let discount = 0;
// Enterprise customers get 15% discount
if (customer.type === 'ENTERPRISE') {
discount = 0.15;
}
// Loyal customers (>3 years) get 10% discount
else if (customer.signupDate < getDateYearsAgo(3)) {
discount = 0.10;
}
// First-time customers get 5% discount
else if (customer.orderCount === 0) {
discount = 0.05;
}
// Holiday season additional 5% (October through December)
const currentMonth = new Date().getMonth();
if (currentMonth >= 9 && currentMonth <= 11) {
discount += 0.05;
}
// Orders over $1000 get additional 3% volume discount
if (order.subtotal > 1000) {
discount += 0.03;
}
// Maximum discount cap of 25%
return Math.min(discount, 0.25);
}
This function reveals important business rules about customer classifications, seasonal promotions, volume discounts, and maximum discount policies. Understanding these rules gives you insight into the business domain that goes beyond what might be in requirements documents.
Understanding the “Why” Behind the Code
Legacy code often contains special cases and exception handling that reflect real-world business scenarios:
function processShipment(order) {
// Special handling for Hawaii and Alaska
if (order.shippingAddress.state === 'HI' || order.shippingAddress.state === 'AK') {
return processRemoteStateShipment(order);
}
// Special handling for PO boxes
if (isPOBox(order.shippingAddress)) {
return processPOBoxShipment(order);
}
// Special handling for hazardous materials
if (orderContainsHazardousMaterials(order)) {
return processHazmatShipment(order);
}
// Standard shipping for everything else
return processStandardShipment(order);
}
These special cases reveal important business constraints and requirements. Understanding why these exceptions exist gives you valuable context for future development work.
Testing and Verification Skills
Working with legacy code often means adding tests to previously untested code, which develops sophisticated testing skills.
Retrofitting Tests
Adding tests to legacy code requires different approaches than test-driven development for new features:
- Characterization testing to document existing behavior
- Creating seams in tightly coupled code to enable testing
- Identifying critical paths that need test coverage
- Balancing test coverage with development time
For example, you might use dependency injection to make a previously untestable class testable:
// Original untestable code
class PaymentProcessor {
processPayment(amount) {
const gateway = new PaymentGateway();
return gateway.submitPayment(amount);
}
}
// Refactored for testability
class PaymentProcessor {
constructor(gateway = new PaymentGateway()) {
this.gateway = gateway;
}
processPayment(amount) {
return this.gateway.submitPayment(amount);
}
}
// Now testable with a mock
function testPaymentProcessor() {
const mockGateway = {
submitPayment: jest.fn().mockReturnValue({ success: true })
};
const processor = new PaymentProcessor(mockGateway);
const result = processor.processPayment(100);
expect(result.success).toBe(true);
expect(mockGateway.submitPayment).toHaveBeenCalledWith(100);
}
Risk-Based Testing
With limited time and resources, maintaining legacy code teaches you to prioritize testing efforts based on risk:
- Critical business functions
- Areas with frequent bugs
- Code with high complexity
- Components with many dependencies
This pragmatic approach to testing is valuable throughout your career, as no project has unlimited time for testing.
Communication and Documentation Skills
Legacy code maintenance often involves working with stakeholders who have historical knowledge of the system, developing crucial soft skills.
Effective Knowledge Transfer
Working with legacy code requires effective knowledge transfer:
- Interviewing longtime team members about system history
- Documenting discoveries for future developers
- Creating diagrams to visualize complex systems
- Writing clear commit messages that explain the “why” not just the “what”
These skills are transferable to any software development role and become increasingly important as you advance in your career.
Improving Documentation
Legacy code maintenance provides opportunities to improve documentation:
/**
* Processes a customer refund request
*
* @param {string} orderId - The original order identifier
* @param {number} amount - Refund amount in cents
* @param {string} reason - Reason code for the refund
* @param {Object} options - Additional refund options
* @param {boolean} options.immediate - If true, process immediately instead of batch
* @param {string} options.approvedBy - Employee ID of manager who approved refund
*
* @returns {Promise<Object>} Refund result object
*
* @throws {OrderNotFoundError} If the order doesn't exist
* @throws {RefundExceedsOrderError} If refund amount exceeds original order
* @throws {RefundAlreadyProcessedError} If order was already refunded
*
* @example
* // Process a standard refund
* const result = await processRefund('ORD-12345', 2500, 'CUSTOMER_REQUEST', {
* approvedBy: 'EMP-789'
* });
*
* // Process an immediate refund for damaged item
* const result = await processRefund('ORD-12345', 5000, 'DAMAGED_ITEM', {
* immediate: true,
* approvedBy: 'EMP-789'
* });
*/
async function processRefund(orderId, amount, reason, options = {}) {
// Implementation...
}
By adding comprehensive documentation to legacy functions, you create value for the entire development team and practice clear technical communication.
Refactoring and Modernization
Legacy code maintenance often includes opportunities for refactoring and modernization, which teaches valuable transformation skills.
Incremental Improvement
Working with legacy code teaches incremental improvement strategies:
- Identifying high-value, low-risk refactoring opportunities
- Breaking down large refactoring efforts into manageable steps
- Using the Strangler Fig pattern to gradually replace components
- Maintaining backward compatibility during transitions
For example, you might modernize a callback-based API to use Promises while maintaining backward compatibility:
// Original callback-based function
function fetchUserData(userId, callback) {
// Implementation with callback
}
// Modernized version with Promise support that maintains backward compatibility
function fetchUserData(userId, callback) {
// Create a Promise-based implementation
const promise = new Promise((resolve, reject) => {
// Implementation that calls resolve/reject
});
// Support for callback pattern if provided
if (typeof callback === 'function') {
promise
.then(result => callback(null, result))
.catch(err => callback(err));
return;
}
// Otherwise return the Promise
return promise;
}
Measuring Improvement
Legacy code refactoring teaches the importance of measuring improvements:
- Establishing baseline metrics before changes
- Quantifying the impact of refactoring
- Communicating value to stakeholders
For instance, you might track metrics like reduced error rates, improved performance, or decreased time required for future changes to demonstrate the value of refactoring efforts.
Career Benefits of Legacy Code Experience
Beyond the technical skills, there are significant career benefits to gaining experience with legacy code maintenance.
Becoming an Essential Team Member
Developers who can effectively maintain legacy systems often become indispensable team members:
- They understand critical systems that power the business
- They can navigate complex codebases efficiently
- They build institutional knowledge that’s valuable to the organization
- They can bridge the gap between old and new systems
This expertise often leads to job security, leadership opportunities, and increased influence within the organization.
Preparing for Senior Roles
Experience with legacy code maintenance prepares you for senior engineering roles:
- Technical leadership positions require understanding complex systems
- Architectural roles benefit from seeing the long-term evolution of systems
- Team leads need to balance technical debt with new feature development
- Engineering managers must communicate technical constraints to product teams
Many CTOs and principal engineers credit their experience with legacy systems as crucial to their career advancement.
Practical Strategies for Learning from Legacy Code
To maximize the educational value of legacy code maintenance, consider these practical strategies:
Approach Legacy Code with Curiosity
Rather than dreading legacy code assignments, approach them with curiosity:
- Ask “why was it built this way?” instead of judging the code
- Look for patterns and design decisions that have stood the test of time
- Seek to understand the business context when the code was written
- Appreciate the constraints the original developers were working under
This mindset transforms legacy code maintenance from a chore into a learning opportunity.
Document Your Discoveries
Create value for yourself and others by documenting what you learn:
- Maintain a personal knowledge base of patterns and anti-patterns
- Create architecture diagrams as you understand system components
- Add comments explaining complex business logic
- Write “archeological” reports for particularly complex subsystems
This documentation process reinforces your learning and creates artifacts that demonstrate your expertise.
Practice Incremental Improvement
Use each maintenance task as an opportunity for incremental improvement:
- Add tests around code you need to change
- Improve variable names and function signatures
- Extract reusable functions from duplicated code
- Add or improve documentation
These small improvements compound over time and provide practical experience in code quality improvement.
Conclusion: Embracing the Legacy Code Journey
While building new features might seem more exciting on the surface, maintaining legacy code offers a depth of learning that’s difficult to achieve otherwise. From understanding complex systems to developing advanced debugging skills, from uncovering business domain knowledge to practicing incremental improvement, legacy code maintenance is a powerful accelerator for your development skills.
The next time you’re assigned to maintain a legacy system, consider it not as a burden but as an opportunity to develop skills that will serve you throughout your career. The developers who can effectively bridge the gap between legacy systems and modern development are uniquely valuable in our industry.
By embracing the educational value of legacy code maintenance, you’ll not only become a more well-rounded developer but also position yourself for career advancement opportunities that require the depth of understanding that comes from working with complex, established systems.
Remember: today’s cutting-edge feature is tomorrow’s legacy code. Learning to work effectively with legacy systems prepares you for the full lifecycle of software development and makes you a more versatile and valuable software engineer.