Why LLMs Freeze When Asked to Explain Their Thought Process
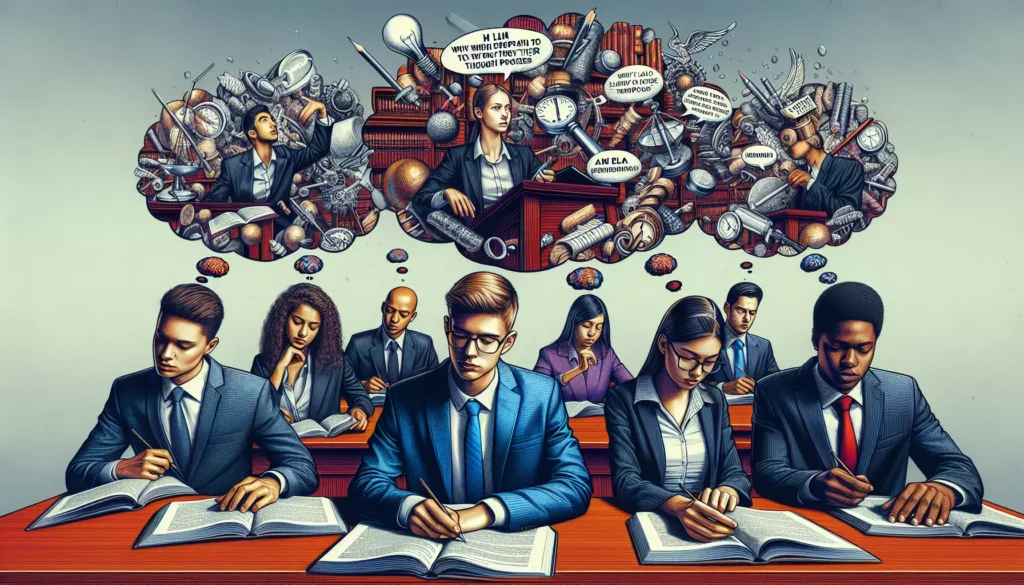
In the world of artificial intelligence and machine learning, large language models (LLMs) have revolutionized how we interact with technology. These sophisticated systems can write code, answer complex questions, and even mimic human conversation with remarkable accuracy. Yet, when asked to explain their own thought processes, these otherwise eloquent models often freeze, falter, or provide unsatisfactory explanations. This phenomenon is particularly relevant in coding education platforms like AlgoCademy, where understanding the reasoning behind solutions is just as important as the solutions themselves.
This article explores why LLMs struggle to articulate their internal processes, the implications for programming education, and how we might address these limitations to create more effective learning experiences.
The Black Box Nature of Large Language Models
To understand why LLMs struggle to explain themselves, we first need to understand how they work. Unlike traditional rule based systems where developers explicitly code every decision path, modern LLMs like GPT-4 operate as “black boxes” whose internal workings are not fully transparent even to their creators.
How LLMs Actually Work
At their core, LLMs are pattern recognition machines trained on vast amounts of text data. They learn to predict the next token (word or part of a word) in a sequence based on the patterns they’ve observed during training. This process involves:
- Training on billions or even trillions of tokens from diverse sources
- Using neural networks with billions of parameters to capture complex patterns
- Employing attention mechanisms to weigh the importance of different parts of the input
- Making predictions based on statistical correlations rather than logical reasoning
When an LLM generates code or explains a programming concept, it’s not “thinking” in the human sense. It’s predicting what tokens would most likely follow in a given context based on its training data.
The Emergence of “Reasoning” in LLMs
What makes modern LLMs so remarkable is that despite not being explicitly designed to reason, they appear to do so. This emergent behavior occurs because:
- The training data includes examples of human reasoning
- The models are large enough to capture subtle patterns in how humans express logical thought
- They’ve been fine-tuned to mimic the structure of human reasoning
However, this appearance of reasoning is fundamentally different from how humans think. The model isn’t consciously working through logical steps; it’s generating text that statistically resembles reasoning based on patterns in its training data.
Why Explaining Their Process Is Difficult for LLMs
Given this background, several factors contribute to LLMs’ difficulty in explaining their own processes:
No Access to Their Own Mechanisms
LLMs don’t have introspective access to their own computational processes. When a model generates code like:
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
It doesn’t “know” that it selected this algorithm because binary search is efficient for sorted arrays with O(log n) time complexity. It generated this code because similar patterns appeared in its training data in contexts related to efficient searching.
When asked to explain its thought process, the model can’t reference its actual internal computations. Instead, it must generate a new response that mimics what a human might say when explaining binary search, which is an entirely separate prediction task.
Post-hoc Rationalization vs. True Explanation
What LLMs provide when asked to explain their reasoning is post-hoc rationalization, not a true explanation of their process. This is analogous to certain aspects of human cognition where we often create logical-sounding explanations for intuitive decisions after the fact.
For example, if an LLM suggests using a hash map to solve a coding problem, and you ask why, it might say:
“I chose a hash map because it provides O(1) average time complexity for lookups, which is optimal for this frequency counting problem.”
This explanation sounds reasonable, but it doesn’t reflect how the model actually arrived at its solution. The model didn’t explicitly evaluate different data structures and their time complexities; it predicted text that statistically follows the pattern of “code solution followed by technical justification.”
The Confidence Illusion
LLMs are trained to sound confident and authoritative, even when their internal “confidence” (probability distribution over possible next tokens) might be quite uncertain. This creates a mismatch between how certain the model appears and how certain it actually is about its responses.
When pushed to explain uncertain outputs, models may freeze or provide inconsistent explanations because they’re being forced to generate high-confidence explanations for outputs that came from uncertain internal states.
The Technical Challenges of Self-Explanation
Beyond these conceptual issues, there are specific technical challenges that make self-explanation difficult for LLMs:
Attention Mechanism Limitations
The attention mechanisms that power modern LLMs are excellent at capturing relationships between tokens but don’t provide a clear audit trail of how a particular output was generated. Unlike a decision tree that can show exactly which branches led to a conclusion, the distributed nature of neural processing means there’s no simple path to trace.
For programming education platforms like AlgoCademy, this creates a challenge: how can an AI tutor explain why a particular coding approach is correct if it can’t trace its own reasoning?
Training Objective Mismatch
LLMs are trained to predict the next token, not to provide accurate explanations of their internal processes. This creates a fundamental mismatch between what we ask them to do (explain themselves) and what they’re optimized to do (predict plausible continuations of text).
When an LLM explains a sorting algorithm, it’s not retrieving its understanding of sorting; it’s generating text that statistically follows the pattern of “sorting algorithm explanation” in its training data.
Lack of Causal Understanding
LLMs lack true causal understanding of the concepts they discuss. When explaining code, they don’t have an internal model of program execution or memory management. They’re pattern-matching against their training data rather than simulating the execution of the code.
Consider this simple code snippet:
x = 5
y = x
x = 10
print(y) # What will this print?
An LLM might correctly state that this will print “5”, but not because it simulated the program’s execution. Rather, it recognizes patterns in how variable assignment and printing work based on examples in its training data.
Implications for Coding Education
These limitations have significant implications for platforms like AlgoCademy that use AI to teach programming:
The Explanation Gap in Learning
Effective learning requires not just seeing the right answer but understanding why it’s right. When LLMs struggle to explain their reasoning, students miss out on the crucial “why” behind coding solutions.
This explanation gap is particularly problematic for complex algorithmic concepts. A student might see a correct implementation of a dynamic programming solution but not grasp the underlying principles if the AI can’t properly articulate them.
Reinforcing Misconceptions
When LLMs provide post-hoc rationalizations rather than true explanations, they risk reinforcing misconceptions. If a model suggests an inefficient algorithm but provides a confident-sounding justification, students might internalize incorrect principles.
For example, if an LLM recommends bubble sort for a large dataset and justifies it with plausible-sounding but incorrect reasoning, students might not recognize this as a suboptimal choice.
The Challenge of Debugging Assistance
Debugging is a critical skill in programming, often requiring deep understanding of how code executes. LLMs’ inability to truly trace through code execution limits their effectiveness as debugging assistants.
When a student asks why their code is producing unexpected output, an LLM might struggle to provide the kind of step-by-step execution analysis that would be most helpful, instead offering generic debugging advice or guessing at likely issues based on pattern recognition.
Strategies for Improving LLM Explanations
Despite these challenges, there are several approaches that can help improve the quality of explanations from LLMs in coding education:
Chain-of-Thought Prompting
One effective technique is chain-of-thought prompting, which encourages LLMs to break down their reasoning into explicit steps. By structuring prompts to elicit step-by-step thinking, we can help models produce more coherent and traceable explanations.
For example, instead of asking “Why is quicksort faster than bubble sort?”, we might prompt:
“Let’s analyze quicksort and bubble sort step by step. First, describe how each algorithm works. Then, analyze their time complexity in best, average, and worst cases. Finally, explain which situations might make quicksort preferable to bubble sort.”
This structured approach helps the model generate more comprehensive and logical explanations, even if they’re still post-hoc rationalizations.
Hybrid Systems: Combining LLMs with Symbolic Reasoning
Another promising approach is to combine LLMs with symbolic reasoning systems that can provide explicit, traceable logic. For programming education, this might involve:
- Using LLMs to generate natural language explanations
- Employing code execution engines to verify correctness and trace through examples
- Utilizing formal verification tools to prove properties of algorithms
For example, when explaining a sorting algorithm, the system could use an LLM to generate the conceptual explanation while a separate component visualizes the algorithm’s execution on example data, showing exactly how elements move through each iteration.
Specialized Fine-tuning for Explanations
Models can be specifically fine-tuned to improve their explanation capabilities. This might involve:
- Training on datasets of high-quality human explanations of programming concepts
- Reinforcement learning from human feedback that rewards clear, accurate explanations
- Developing specific prompting techniques that elicit better explanations for coding concepts
AlgoCademy and similar platforms could benefit from models fine-tuned specifically for explaining programming concepts rather than using general-purpose LLMs.
The Role of Transparency in AI Coding Education
Beyond improving explanations, there’s a broader need for transparency about the limitations of AI in coding education:
Setting Appropriate Expectations
Students using AI-powered platforms should understand that the AI is not “thinking” like a human programmer. Setting appropriate expectations helps prevent confusion when models struggle to explain their reasoning or provide inconsistent explanations.
Platforms like AlgoCademy can include brief explanations of how their AI assistants work, making it clear that:
- The AI generates responses based on patterns in its training data
- It may not always be able to explain its suggestions in the way a human tutor would
- Its explanations should be critically evaluated, not accepted uncritically
Encouraging Critical Thinking
Rather than positioning AI as an authoritative source of programming knowledge, platforms can encourage students to critically evaluate AI-generated explanations. This might involve:
- Prompting students to verify explanations through their own research
- Providing alternative explanations from different sources
- Encouraging students to test concepts through practical coding exercises
This approach turns the limitations of AI explanations into an opportunity to develop critical thinking skills, which are essential for professional programmers.
Human-in-the-Loop Approaches
For complex concepts or when students are struggling, platforms can incorporate human expertise alongside AI assistance. This hybrid approach might include:
- AI-generated initial explanations with human review and enhancement
- Escalation paths to human tutors for concepts the AI struggles to explain
- Community forums where students can discuss AI-generated explanations with peers and experts
This acknowledges the current limitations of AI while still leveraging its strengths in providing immediate, personalized assistance.
Case Study: Teaching Recursion with LLMs
To illustrate these challenges and potential solutions, let’s consider how an LLM might approach teaching recursion, a concept many programming students find challenging.
The LLM’s Initial Explanation
When asked to explain recursion, an LLM might generate something like:
“Recursion is a programming technique where a function calls itself to solve a problem. It’s useful for solving problems that can be broken down into simpler versions of the same problem.
Here’s a simple example of calculating factorial using recursion:
def factorial(n): if n == 0 or n == 1: return 1 else: return n * factorial(n-1)
This function works by breaking down the factorial calculation into smaller subproblems until it reaches the base case.”
This explanation is technically correct but may not address the conceptual difficulties many students face with recursion, such as understanding the call stack or visualizing the execution flow.
Improving the Explanation
Using the strategies discussed earlier, we could improve this explanation:
- Chain-of-thought prompting: “Explain recursion by first defining it, then walking through the execution of a factorial function call for n=3, showing each recursive call and return value step by step.”
- Hybrid approach: Combine the textual explanation with a visual representation of the call stack as the factorial function executes.
- Critical thinking prompt: After the explanation, ask students: “What would happen if we removed the base case? Try to trace through the execution and explain what would occur.”
The enhanced explanation might include a trace table showing how factorial(3) calls factorial(2), which calls factorial(1), along with the corresponding values at each step:
Function Call | n Value | Return Value | Call Stack Depth |
---|---|---|---|
factorial(3) | 3 | 3 * factorial(2) = 3 * 2 = 6 | 1 |
factorial(2) | 2 | 2 * factorial(1) = 2 * 1 = 2 | 2 |
factorial(1) | 1 | 1 (base case) | 3 |
This combination of approaches addresses the limitations of the LLM’s explanation capabilities by providing structured guidance, visual aids, and opportunities for deeper understanding.
The Future of Explainable AI in Coding Education
As AI continues to evolve, we can expect improvements in the ability of LLMs to explain their processes. Several emerging approaches show promise:
Interpretability Research
The field of AI interpretability is working to develop techniques for understanding what’s happening inside neural networks. Advances in this area could eventually allow LLMs to provide more accurate accounts of their internal processes rather than post-hoc rationalizations.
For coding education, this might eventually enable AI tutors that can genuinely explain why they suggested a particular algorithm or data structure based on their internal reasoning rather than pattern matching.
Multimodal Learning Approaches
Combining text with visualizations, code execution, and interactive elements can create more effective learning experiences that compensate for the explanatory limitations of LLMs.
For example, when teaching graph algorithms, an AI might generate both a textual explanation and an interactive visualization showing how the algorithm traverses a graph step by step, giving students multiple ways to understand the concept.
Personalized Explanation Styles
Future systems might adapt their explanation approaches based on individual learning styles and needs. A student who struggles with abstract concepts might receive more concrete, example-based explanations, while one who prefers theoretical understanding might get more formal, mathematical explanations.
This personalization could help address the “one-size-fits-all” limitation of current LLM explanations, where the model doesn’t adapt its explanatory approach to the student’s level of understanding.
Conclusion: Embracing the Limitations While Pushing Forward
The tendency of LLMs to freeze when asked to explain their thought processes stems from fundamental aspects of how these models work. They don’t “think” in the human sense but rather predict text based on patterns in their training data. When asked to explain themselves, they can only generate plausible-sounding explanations, not reveal their actual internal processes.
For coding education platforms like AlgoCademy, these limitations present real challenges. Effective programming education requires not just correct solutions but clear explanations of why those solutions work. However, by combining LLMs with other tools, structuring prompts carefully, and maintaining appropriate expectations, we can still create valuable learning experiences.
The future of AI in coding education likely lies not in expecting LLMs to perfectly mimic human teachers, but in creating hybrid systems that leverage the strengths of AI while compensating for its limitations. By embracing transparency about what AI can and cannot do, we can build educational tools that help students develop not just programming skills but also the critical thinking abilities needed to evaluate and learn from AI-generated content.
As we continue to integrate AI into programming education, the goal should be to use these powerful tools to augment human learning rather than replace human understanding. The limitations of LLMs in explaining their processes can serve as a reminder that true mastery of programming comes not just from knowing what code to write, but understanding deeply why it works the way it does.