Why Learning Python First Could Hold You Back
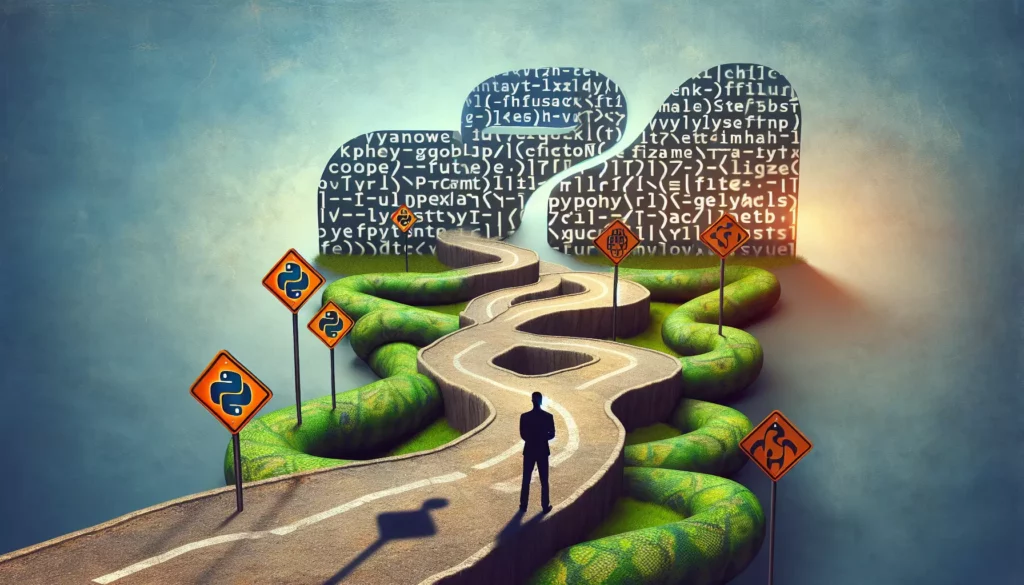
In the world of programming, Python has emerged as a popular choice for beginners. Its simplicity, readability, and vast ecosystem have made it an attractive starting point for those venturing into the realm of coding. However, while Python offers numerous advantages, starting your programming journey with this language might not always be the best approach. In this comprehensive guide, we’ll explore why learning Python first could potentially hold you back in your coding career and discuss alternative paths that might better serve your long-term goals.
The Python Phenomenon
Before we dive into the potential drawbacks of starting with Python, it’s essential to understand why this language has become so popular among beginners:
- Readability: Python’s syntax is clean and easy to understand, making it less intimidating for newcomers.
- Versatility: From web development to data science, Python has applications in various fields.
- Large Community: A vast community of developers means abundant resources and support for learners.
- Rapid Development: Python allows for quick prototyping and development of ideas.
These factors have contributed to Python’s rise as a go-to language for coding bootcamps, online courses, and self-taught programmers. However, this popularity doesn’t necessarily translate to it being the ideal starting point for everyone.
The Hidden Pitfalls of Starting with Python
While Python’s strengths are undeniable, beginning your programming journey with this language can potentially lead to several challenges:
1. Oversimplification of Programming Concepts
Python’s simplicity, while beneficial for quick learning, can sometimes oversimplify important programming concepts. This oversimplification might create gaps in your understanding of fundamental principles that are crucial in other languages.
For example, consider variable declaration and typing:
# Python
x = 5 # Variable declaration and assignment in one line
y = "Hello" # No need to specify data type
Compare this to a language like Java:
// Java
int x = 5; // Explicit type declaration
String y = "Hello"; // Type must be specified
Python’s approach, while convenient, might not prepare you for the explicit type declarations required in many other languages. This can lead to confusion when transitioning to languages with stricter typing systems.
2. Lack of Low-Level Understanding
Python abstracts away many low-level details of how computers work. While this abstraction can speed up development, it may leave you with a limited understanding of memory management, pointers, and other crucial concepts in computer science.
For instance, in Python, you don’t need to worry about memory allocation and deallocation:
# Python
numbers = [1, 2, 3, 4, 5] # List creation and memory allocation handled automatically
del numbers # Memory deallocation handled by Python's garbage collector
However, in a language like C, you need to manage memory explicitly:
// C
int* numbers = (int*)malloc(5 * sizeof(int)); // Explicit memory allocation
// ... use the array ...
free(numbers); // Explicit memory deallocation
Understanding these low-level concepts is crucial for writing efficient code and debugging complex issues, especially in performance-critical applications.
3. Potential for Developing Bad Habits
Python’s flexibility and forgiving nature can lead to the development of coding habits that might not translate well to other languages or larger, more complex projects. For example:
- Indentation-based syntax: While this enforces clean code in Python, it doesn’t prepare you for languages that use braces or keywords for block delimitation.
- Dynamic typing: Python’s dynamic typing can lead to a cavalier attitude towards variable types, which can cause issues in statically-typed languages.
- Lack of semicolons: Not using semicolons in Python might make it harder to adapt to languages where they are required.
4. Limited Exposure to Object-Oriented Programming (OOP)
While Python supports object-oriented programming, its implementation is quite different from languages like Java or C++. This difference can lead to a skewed understanding of OOP principles.
Consider this simple class definition in Python:
# Python
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def greet(self):
print(f"Hello, my name is {self.name}")
person = Person("Alice", 30)
person.greet()
Now, compare it to Java:
// Java
public class Person {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public void greet() {
System.out.println("Hello, my name is " + this.name);
}
}
Person person = new Person("Alice", 30);
person.greet();
The Java version introduces concepts like access modifiers, explicit constructor definition, and the ‘new’ keyword for object creation. These concepts are crucial in many OOP languages but are not as prominent in Python.
5. Difficulty in Transitioning to Compiled Languages
Python is an interpreted language, which means it doesn’t need to be compiled before running. This can make the transition to compiled languages like C++ or Java more challenging. Understanding the compilation process, dealing with compiler errors, and managing build systems are skills that Python doesn’t necessarily prepare you for.
Alternative Starting Points for Programming
Given these potential drawbacks, what alternatives should aspiring programmers consider? Here are a few options that might provide a more robust foundation:
1. C or C++
Starting with C or C++ can provide a deep understanding of how computers work at a lower level. These languages require you to manage memory, understand pointers, and deal with strict type systems. While they have a steeper learning curve, the knowledge gained is invaluable and transferable to many other languages.
// C++
#include <iostream>
int main() {
int* numbers = new int[5]; // Dynamic memory allocation
for (int i = 0; i < 5; i++) {
numbers[i] = i + 1;
}
for (int i = 0; i < 5; i++) {
std::cout << numbers[i] << " ";
}
delete[] numbers; // Memory deallocation
return 0;
}
2. Java
Java offers a good balance between low-level control and high-level abstractions. It enforces object-oriented programming principles and provides a strong foundation in concepts like static typing, interfaces, and design patterns.
// Java
public class Example {
public static void main(String[] args) {
int[] numbers = new int[5];
for (int i = 0; i < numbers.length; i++) {
numbers[i] = i + 1;
}
for (int number : numbers) {
System.out.print(number + " ");
}
}
}
3. JavaScript
As the language of the web, JavaScript provides immediate visual feedback and introduces concepts like asynchronous programming. It also offers a good mix of functional and object-oriented programming paradigms.
// JavaScript
let numbers = Array(5).fill().map((_, i) => i + 1);
numbers.forEach(number => console.log(number));
Balancing Act: Incorporating Python in Your Learning Journey
While we’ve discussed the potential drawbacks of starting with Python, it’s important to note that Python still has a valuable place in a programmer’s toolkit. The key is to approach it strategically:
1. Learn the Fundamentals First
Consider starting with a language that exposes you to more fundamental concepts. Once you have a solid grasp of these basics, transitioning to Python will be easier, and you’ll appreciate its abstractions more.
2. Use Python as a Complementary Language
Python excels in areas like data analysis, machine learning, and scripting. After learning a more foundational language, you can leverage Python’s strengths in these domains.
3. Focus on Computer Science Concepts
Regardless of the language you start with, focus on learning core computer science concepts like data structures, algorithms, and software design principles. These concepts transcend specific languages and will serve you well throughout your career.
4. Practice in Multiple Languages
Once you’re comfortable with one language, start practicing in others. This will help you understand the strengths and weaknesses of different languages and make you a more versatile programmer.
The Role of Platforms Like AlgoCademy
In your journey to become a proficient programmer, platforms like AlgoCademy can play a crucial role. AlgoCademy focuses on developing algorithmic thinking and problem-solving skills, which are fundamental to programming regardless of the language you use.
Here’s how AlgoCademy can complement your learning path:
- Language-Agnostic Problem Solving: AlgoCademy emphasizes algorithmic thinking, which is applicable across all programming languages.
- Preparation for Technical Interviews: The platform’s focus on FAANG-style interview questions helps you develop the skills needed for high-level technical interviews.
- AI-Powered Assistance: Get personalized guidance as you work through coding challenges, helping you understand and overcome obstacles.
- Practical Coding Skills: Apply theoretical concepts to real-world coding problems, bridging the gap between academic knowledge and practical skills.
Conclusion: Choosing the Right Path for You
While Python’s popularity as a first programming language is understandable, it’s crucial to consider the potential limitations of this approach. Starting with a language that exposes you to more fundamental concepts can provide a stronger foundation for your programming career.
However, the “best” first language often depends on your goals, learning style, and the specific area of programming you’re interested in. Here are some final thoughts to consider:
- If you’re interested in system programming or want a deep understanding of computer architecture, consider starting with C or C++.
- If you’re aiming for a career in enterprise software development, Java might be a good starting point.
- If web development is your goal, you might want to begin with JavaScript.
- If you’re specifically interested in data science or machine learning, Python could indeed be a good first choice.
Remember, no single language will teach you everything you need to know. The key is to focus on understanding core programming concepts, practicing problem-solving, and continuously expanding your skills. Platforms like AlgoCademy can be invaluable in this journey, helping you develop the algorithmic thinking and problem-solving skills that are crucial across all areas of programming.
Ultimately, the most important factor is not which language you start with, but your commitment to learning, practicing, and growing as a programmer. With dedication and the right resources, you can overcome any initial limitations and become a skilled, versatile developer capable of tackling complex challenges in any language.