Why Learning Multiple Programming Languages Isn’t Making You a Better Coder
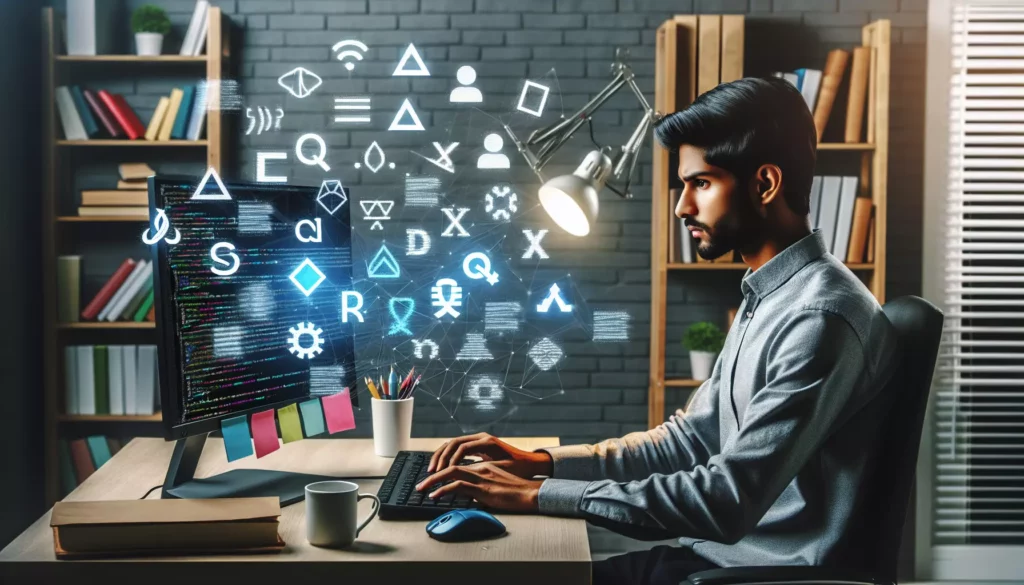
In the ever evolving world of programming, there’s a common belief that mastering multiple programming languages is the key to becoming an exceptional coder. Bootcamps, online courses, and career advisors often push the narrative that the more languages you know, the more marketable and skilled you become. But what if this conventional wisdom is leading you down an inefficient path?
As someone deeply involved in coding education at AlgoCademy, I’ve observed thousands of learners on their journey from beginners to professional developers. A pattern has emerged: those who chase language after language often struggle more with fundamental problem solving than those who deeply master core programming concepts through focused study.
In this article, we’ll explore why the “collect programming languages like Pokémon” approach might be hindering rather than helping your development as a programmer, and what you should focus on instead to truly level up your coding abilities.
The Language Collection Fallacy
Open any junior developer’s resume, and you’ll likely see a laundry list of programming languages: “Proficient in Python, JavaScript, Java, C++, Ruby, PHP…” The list goes on. This phenomenon stems from a fundamental misunderstanding about what makes a great programmer.
The truth is that professional coding is not primarily about the languages you know but about your ability to solve problems effectively. Yet the allure of adding another language to your toolkit is strong, especially when job postings seem to demand familiarity with an ever expanding set of technologies.
Consider this scenario: You’ve spent three months learning Python basics. Instead of deepening that knowledge, you pivot to JavaScript because a job posting mentioned it. Three months later, you switch to learning Java because another interesting opportunity requires it. A year passes, and you have surface level knowledge of multiple languages but struggle to build anything substantial in any of them.
This approach creates what I call “shallow programmers” who know just enough syntax across multiple languages to be dangerous but lack the depth to solve complex problems efficiently.
The Hidden Costs of Language Hopping
1. Cognitive Overhead
Each programming language comes with its own paradigms, idioms, and ecosystems. When you’re constantly switching between languages, you’re forcing your brain to context switch between different ways of thinking about and structuring code.
Research in cognitive psychology suggests that this type of context switching can reduce productivity by up to 40%. For programmers, this means more time spent consulting documentation about syntax and less time spent on the actual problem solving that creates value.
2. Shallow Understanding
Learning the syntax of a language is just the beginning. True mastery comes from understanding the language’s strengths, weaknesses, common pitfalls, and best practices. It comes from writing thousands of lines of code, making mistakes, and refining your approach.
When you hop between languages, you rarely get past the “hello world” phase to develop this deeper understanding. You might know how to write a for loop in five different languages, but do you know when to use a for loop versus a more elegant approach in each of those languages?
3. Reinventing the Wheel
Each time you start with a new language, you spend valuable learning time on basics that don’t significantly improve your problem solving abilities. Consider the time spent learning how to declare variables or write conditional statements in a new syntax when you could be learning about algorithm optimization or system design.
4. Illusory Competence
Perhaps most dangerously, knowing multiple languages at a surface level can create a false sense of competence. You might feel like you’re making progress because you can now write basic programs in several languages, but this doesn’t necessarily translate to being able to build complex, maintainable systems.
What Top Tech Companies Actually Look For
At AlgoCademy, we prepare programmers for technical interviews at major tech companies like Google, Facebook, Amazon, Apple, and Netflix (often collectively referred to as FAANG). These companies are known for their rigorous interview processes, which might lead you to believe they want candidates who know every language under the sun.
The reality is quite different. These companies are primarily looking for strong problem solvers who demonstrate:
- Algorithmic thinking: Can you break down complex problems into solvable components?
- Data structure knowledge: Do you understand which data structures to use for different scenarios?
- System design skills: Can you architect scalable, maintainable solutions?
- Clean coding practices: Do you write code that others can understand and maintain?
- Testing methodology: Can you ensure your code works as expected?
Notice that specific programming languages are not on this list. In fact, many FAANG companies allow candidates to interview in the language of their choice, recognizing that a strong programmer can adapt to new languages relatively quickly if they have solid fundamentals.
As a former interviewer at one of these companies shared with me: “I’d much rather hire someone who’s excellent in one language and has strong computer science fundamentals than someone who knows six languages but struggles with basic algorithm implementation.”
The Transferable Skills That Actually Matter
If collecting languages isn’t the path to programming excellence, what should you focus on instead? Here are the skills that truly make you a better coder, regardless of the language you’re using:
1. Problem Decomposition
The ability to take a complex problem and break it down into manageable, solvable parts is perhaps the most valuable skill a programmer can develop. This is language agnostic and forms the foundation of all good software development.
// Example of problem decomposition for calculating user activity metrics
// 1. Gather raw user activity data
// 2. Filter out irrelevant actions
// 3. Group actions by user
// 4. Calculate metrics per user
// 5. Aggregate for overall statistics
// 6. Format for presentation
2. Algorithmic Thinking
Understanding algorithm design patterns, time and space complexity analysis, and when to apply specific algorithms to problems will serve you well in any language. This is why competitive programming and algorithm challenges are valuable regardless of your primary language.
// Understanding that this is O(n²) in any language matters more than the syntax
function hasDuplicates(array) {
for (let i = 0; i < array.length; i++) {
for (let j = i + 1; j < array.length; j++) {
if (array[i] === array[j]) return true;
}
}
return false;
}
// And knowing how to optimize to O(n) is even more valuable
function hasDuplicatesOptimized(array) {
const seen = new Set();
for (const item of array) {
if (seen.has(item)) return true;
seen.add(item);
}
return false;
}
3. Data Structure Knowledge
Deeply understanding when and why to use arrays, linked lists, trees, graphs, hash tables, and other data structures will make you more effective regardless of which language you’re coding in. Each structure has strengths and weaknesses that transcend language specifics.
// The concept of using a hash map for O(1) lookups is universal
// Python
def find_pair_with_sum(numbers, target_sum):
complements = {}
for i, num in enumerate(numbers):
if target_sum - num in complements:
return (complements[target_sum - num], i)
complements[num] = i
return None
# Java would use a different syntax but the same concept
# public static int[] findPairWithSum(int[] numbers, int targetSum) {
# Map<Integer, Integer> complements = new HashMap<>();
# for (int i = 0; i < numbers.length; i++) {
# if (complements.containsKey(targetSum - numbers[i])) {
# return new int[] {complements.get(targetSum - numbers[i]), i};
# }
# complements.put(numbers[i], i);
# }
# return null;
# }
4. Code Organization and Design Patterns
Learning how to structure code for readability, maintainability, and scalability is far more valuable than learning new syntax. Design patterns like MVC, Singleton, Factory, and Observer can be applied across languages and will help you build better software regardless of the implementation details.
5. Debugging and Problem Solving
The ability to methodically track down and fix issues in code is invaluable. This includes understanding how to use debugging tools, how to isolate problems, how to test hypotheses, and how to verify solutions. These skills transfer across languages even though the specific tools might differ.
When Learning Multiple Languages Does Make Sense
I’m not suggesting that you should only ever learn one programming language. There are legitimate reasons to expand your language portfolio, but they should be strategic rather than collect them all.
Expanding Your Programming Paradigms
Languages often represent different programming paradigms. Learning a language from a different paradigm can genuinely expand your thinking about problems:
- Object Oriented Programming: Java, C++, Python
- Functional Programming: Haskell, Clojure, F#
- Procedural Programming: C, Pascal
- Logic Programming: Prolog
- Concurrent Programming: Go, Erlang
For example, if you’re proficient in an object oriented language like Java, learning a functional language like Haskell can introduce you to new ways of thinking about state and side effects. This paradigm shift can influence how you write code even when you return to Java.
Solving Different Types of Problems
Some languages are better suited for specific domains:
- Systems Programming: Rust, C
- Web Development: JavaScript, PHP
- Data Science: Python, R
- Mobile Development: Swift (iOS), Kotlin (Android)
- Game Development: C#, C++
If you’re looking to solve problems in a new domain, learning the language most commonly used in that domain makes sense. However, this should be driven by the problems you want to solve, not just collection for its own sake.
Career Advancement
Sometimes, learning a new language is necessary for a specific job opportunity or career path. If you’re eyeing a role that requires a language you don’t know, by all means, learn it. But do so with purpose and depth rather than just adding it to your resume.
A Better Approach: Depth Over Breadth
Instead of spreading yourself thin across multiple languages, consider taking a “T-shaped” approach to your programming education:
The Vertical Bar: Deep Expertise
Choose one language as your primary focus and dive deep. Learn not just the syntax but:
- The language’s idioms and best practices
- Common pitfalls and how to avoid them
- Performance characteristics and optimization techniques
- The ecosystem of libraries, frameworks, and tools
- Testing methodologies specific to the language
- Advanced features that experienced developers use
For most beginners, Python is an excellent choice for this deep dive due to its readability, versatility, and strong community. JavaScript is another solid option, especially if web development interests you.
The Horizontal Bar: Transferable Skills
Across the top of your T, focus on the language agnostic skills we discussed earlier:
- Algorithm design and analysis
- Data structure implementation and usage
- System design principles
- Testing strategies
- Debugging methodologies
- Code organization and architecture
These skills will make you a better programmer regardless of which language you’re using and will make it easier to pick up new languages when necessary.
Practical Steps to Become a Better Coder
If you’re convinced that depth is more valuable than breadth, here are some practical steps to improve your coding skills:
1. Master One Language First
Commit to spending at least six months to a year focusing primarily on one language. Build projects of increasing complexity, refactor your code, and learn from experienced developers in that language community.
For example, if you choose Python:
- Start with basic syntax and control structures
- Move on to Python specific features like list comprehensions and generators
- Learn the standard library thoroughly
- Understand Python’s object model and how it differs from other languages
- Practice writing Pythonic code that follows community conventions
- Explore advanced topics like decorators, context managers, and metaclasses
2. Focus on Problem Solving
Regularly practice solving algorithmic problems and coding challenges in your chosen language. Sites like LeetCode, HackerRank, and Codeforces offer thousands of problems that will strengthen your problem solving muscles.
// The approach to solving this problem is what matters, not the language
function twoSum(nums, target) {
const numMap = new Map();
for (let i = 0; i < nums.length; i++) {
const complement = target - nums[i];
if (numMap.has(complement)) {
return [numMap.get(complement), i];
}
numMap.set(nums[i], i);
}
return null;
}
3. Build Complete Projects
Theory is important, but nothing beats building real projects. Choose projects that interest you and push your boundaries. Complete them from start to finish, including:
- Planning and requirements gathering
- System design and architecture
- Implementation
- Testing and debugging
- Deployment and maintenance
This end to end experience will teach you far more than hopping between tutorials in different languages.
4. Read and Understand Others’ Code
Reading high quality code written by experienced developers is one of the best ways to improve. Contribute to open source projects, review codebases on GitHub, or participate in code reviews at work.
Pay attention to how experienced developers structure their code, handle edge cases, and document their work. These patterns can be applied regardless of language.
5. Teach What You Learn
Teaching forces you to solidify your understanding. Start a blog, create tutorials, mentor junior developers, or simply explain concepts to peers. The process of articulating what you know will reveal gaps in your understanding and help you learn more deeply.
6. Only Add Languages When Necessary
When you do decide to learn a new language, do so with purpose. Ask yourself:
- What specific problems will this language help me solve?
- What new concepts or paradigms will it teach me?
- How does it complement my existing knowledge?
And when you learn that new language, leverage your existing knowledge. Focus on the differences and similarities with languages you already know rather than starting from scratch.
How AlgoCademy Approaches Programming Education
At AlgoCademy, our approach to teaching programming reflects this philosophy of depth over breadth. Our curriculum focuses on building strong fundamentals that transfer across languages rather than teaching multiple languages superficially.
Focus on Problem Solving
Our interactive coding tutorials emphasize algorithmic thinking and problem decomposition. We teach you how to approach problems methodically, regardless of the specific language you’re using.
// Example of how we might teach array manipulation
function rotateArray(arr, k) {
// Step 1: Normalize k to avoid unnecessary rotations
k = k % arr.length;
// Step 2: Reverse the entire array
reverse(arr, 0, arr.length - 1);
// Step 3: Reverse the first k elements
reverse(arr, 0, k - 1);
// Step 4: Reverse the remaining elements
reverse(arr, k, arr.length - 1);
return arr;
}
function reverse(arr, start, end) {
while (start < end) {
[arr[start], arr[end]] = [arr[end], arr[start]];
start++;
end--;
}
}
This approach teaches you both the specific implementation and the general problem solving strategy that can be applied in any language.
Language as a Tool, Not the Goal
We view programming languages as tools to express solutions, not as goals in themselves. While we provide tutorials in popular languages like Python and JavaScript, we emphasize that the concepts are what matter most.
Progression from Basics to Advanced
Our curriculum is structured to take learners from beginner level coding to preparation for technical interviews at major tech companies. This progressive approach ensures that you build a solid foundation before tackling more complex topics.
AI Powered Assistance
Our AI powered assistance helps identify gaps in your understanding and provides targeted guidance. This personalized approach ensures that you develop a deep, holistic understanding rather than just memorizing syntax.
Case Studies: Depth vs. Breadth
To illustrate the benefits of focusing on depth over breadth, let’s look at two contrasting case studies from our students:
Case Study 1: Michael the Language Collector
Michael came to AlgoCademy after spending two years learning programming on his own. He had completed introductory courses in Python, JavaScript, Java, and C++, and listed all four on his resume. However, when faced with our assessment challenges, he struggled with fundamental concepts like recursion, dynamic programming, and efficient algorithm design.
During mock interviews, Michael would often get confused about syntax and resort to pseudocode because he couldn’t remember the specific implementation details in any single language. His solutions tended to be brute force approaches that didn’t leverage language specific optimizations.
After six months of focusing exclusively on Python and computer science fundamentals through our program, Michael showed dramatic improvement. By channeling his efforts into mastering one language and core concepts, he was able to solve complex problems efficiently and communicate his solutions clearly.
Case Study 2: Sarah the Deep Diver
Sarah took a different approach. When she joined AlgoCademy, she had been coding in JavaScript for just over a year but had delved deeply into the language. She understood closures, prototypal inheritance, asynchronous programming, and the event loop in detail.
While Sarah initially only knew JavaScript, she excelled at problem solving because she had developed strong analytical skills through her deep study. When our curriculum required her to learn some Python for data structures and algorithms, she picked it up quickly by mapping concepts to her existing JavaScript knowledge.
In mock interviews, Sarah impressed interviewers with her thorough understanding of time and space complexity and her ability to optimize algorithms. Although she sometimes needed to look up specific syntax for more advanced features, her strong fundamentals allowed her to communicate her approach clearly.
Sarah received three job offers within two months of completing our program, including one from a FAANG company, despite having fewer languages on her resume than many other candidates.
Conclusion: Quality Over Quantity
In the world of programming, depth beats breadth almost every time. While it might be tempting to add language after language to your resume, the reality is that becoming an expert in one language and developing strong computer science fundamentals will make you a far more effective programmer than having surface level knowledge of many languages.
Remember:
- Focus on mastering one language deeply before branching out
- Invest time in language agnostic skills like algorithms, data structures, and system design
- Learn new languages only when they serve a specific purpose or introduce new paradigms
- Build complete projects that demonstrate your problem solving abilities
- Value understanding over memorization
By taking this approach, you’ll not only become a better coder more quickly, but you’ll also find it easier to learn new languages when necessary. The deep understanding of programming concepts you develop will transfer across languages, allowing you to pick up new syntax and idioms with relative ease.
At AlgoCademy, we’ve seen this approach succeed time and again. Our most successful students aren’t those who come in knowing the most languages but those who commit to mastering the fundamental skills that make a great programmer in any language.
So the next time you’re tempted to start learning a new language just to add it to your resume, ask yourself: Would my time be better spent deepening my understanding of concepts I can apply everywhere? The answer, more often than not, is yes.