Why Learning Multiple Programming Languages Isn’t Making You a Better Coder
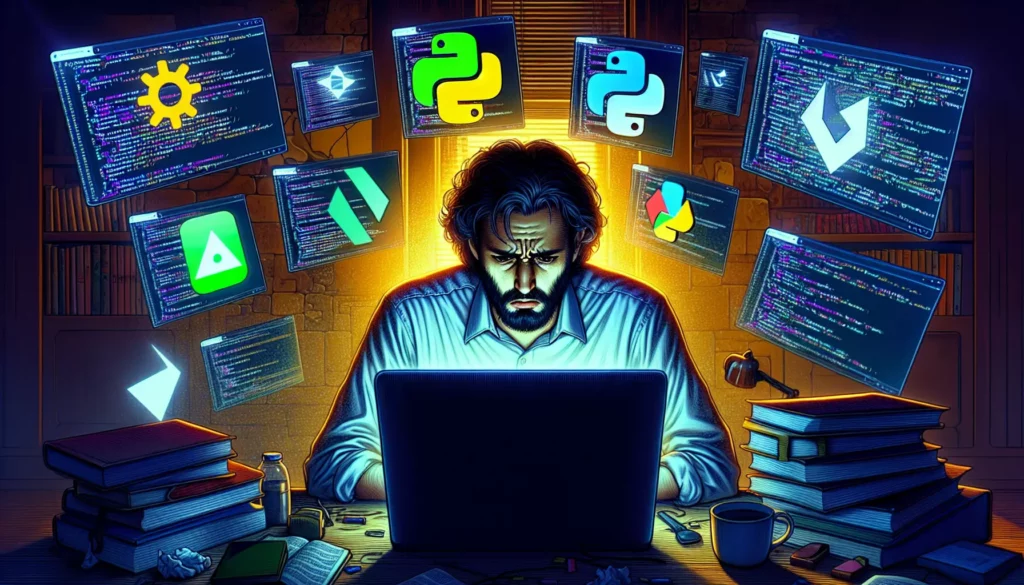
In the constantly evolving world of software development, there’s a persistent belief that learning as many programming languages as possible is the key to becoming an exceptional programmer. Developers often proudly list a dozen languages on their resumes, believing this demonstrates their versatility and expertise. However, this approach might actually be hindering rather than helping your growth as a programmer.
While there’s undeniable value in understanding different programming paradigms and approaches, the obsession with collecting languages like Pokémon cards can lead to a superficial understanding that doesn’t translate to real coding proficiency. This article explores why focusing on depth rather than breadth might be the better path to programming mastery.
The Polyglot Programmer Myth
The tech industry often celebrates the “polyglot programmer” — someone fluent in multiple programming languages. Job listings frequently demand knowledge of numerous languages, frameworks, and tools, creating the impression that the more languages you know, the more valuable you are as a developer.
This mindset has led many programmers to adopt a checklist mentality:
- Learn Python syntax ✓
- Build a simple JavaScript app ✓
- Complete a Ruby tutorial ✓
- Write “Hello World” in Rust ✓
But there’s a fundamental problem with this approach: knowing the syntax and basic constructs of a language is vastly different from understanding how to use it effectively to solve complex problems. It’s the difference between knowing the vocabulary of a foreign language and being able to write poetry in it.
Quantity vs. Quality: The Depth Problem
When developers spread their learning efforts across multiple languages, they often fail to reach proficiency in any of them. Let’s examine why this shallow learning approach is problematic:
1. Syntax Knowledge Isn’t Programming Skill
Programming isn’t primarily about knowing syntax; it’s about problem-solving, architectural thinking, and understanding computational concepts. Jumping from language to language might help you learn different syntaxes, but it doesn’t necessarily improve your ability to solve complex problems.
Consider this example of a simple function in multiple languages:
// JavaScript
function calculateArea(radius) {
return Math.PI * radius * radius;
}
# Python
def calculate_area(radius):
return math.pi * radius * radius
// Java
public double calculateArea(double radius) {
return Math.PI * radius * radius;
}
While the syntax differs, the underlying concept is identical. Learning to write this function in ten different languages doesn’t make you ten times better at programming; it just means you can express the same basic idea in different syntactic forms.
2. Missing the Ecosystem Depth
Each programming language exists within an ecosystem of libraries, frameworks, tools, and community practices. True proficiency comes from understanding not just the language itself, but its entire ecosystem and idiomatic usage patterns.
For instance, becoming proficient in JavaScript involves much more than knowing the language syntax:
- Understanding the event loop and asynchronous programming model
- Mastering the DOM and browser APIs
- Learning common frameworks like React, Angular, or Vue
- Becoming familiar with npm and the module ecosystem
- Understanding build tools like Webpack or Rollup
- Knowing JavaScript’s quirks and best practices
A developer who deeply understands one ecosystem will likely be more effective than someone with surface-level knowledge of five different ecosystems.
3. The Illusion of Transferability
While some programming concepts transfer between languages, many do not. Each language has its own idioms, patterns, and approaches to solving problems. What’s considered a best practice in one language might be an anti-pattern in another.
For example, consider error handling approaches:
// JavaScript: Using promises and catch
fetchData()
.then(data => processData(data))
.catch(error => console.error(error));
// Go: Using explicit error returns
data, err := fetchData()
if err != nil {
log.Fatal(err)
return
}
processData(data)
// Java: Using try-catch blocks
try {
Data data = fetchData();
processData(data);
} catch (Exception e) {
System.err.println(e);
}
Each approach reflects the language’s philosophy and conventions. Knowing all three doesn’t necessarily make you better at error handling; what matters is deeply understanding the principles behind error management in software systems.
The Cognitive Load of Language Switching
The human brain has limited cognitive resources. When you’re constantly switching between different programming languages, you’re adding unnecessary cognitive load that can impede your ability to solve the actual problem at hand.
Context Switching Costs
Research on task switching shows that there’s a significant cognitive cost to switching contexts. When you switch between programming languages, you’re not just changing syntax; you’re shifting between different mental models, tools, and approaches.
This context switching can lead to:
- Reduced productivity as your brain needs time to “warm up” in each language
- Increased error rates as you accidentally use patterns or syntax from one language in another
- Mental fatigue from constantly having to remember different rules and conventions
Professional developers who work deeply in one language often report entering a “flow state” where the language becomes second nature, allowing them to focus entirely on the problem rather than the tools.
The Curse of Shallow Knowledge
When you only have surface-level knowledge of multiple languages, you’re more likely to:
- Miss language-specific optimizations and best practices
- Write code that’s technically correct but not idiomatic
- Struggle with debugging complex issues
- Fail to leverage the full power of the language and its ecosystem
This limitation becomes particularly evident when facing complex problems that require deep language knowledge to solve efficiently.
What Actually Makes a Better Programmer
If collecting programming languages isn’t the path to becoming a better programmer, what is? Let’s explore the skills and approaches that genuinely improve programming ability:
1. Mastering Fundamental Concepts
The core concepts of computer science and software engineering transcend specific languages:
- Data structures and algorithms
- Time and space complexity analysis
- Design patterns and architectural principles
- Testing methodologies
- Debugging strategies
- Concurrency and parallelism
A deep understanding of these fundamentals allows you to approach problems effectively regardless of the language you’re using.
2. Problem-Solving Skills
At its core, programming is problem-solving. The best programmers excel at:
- Breaking down complex problems into manageable components
- Identifying appropriate algorithms and data structures for specific problems
- Recognizing patterns and applying known solutions to new contexts
- Thinking systematically and anticipating edge cases
These skills are language-agnostic and form the foundation of programming expertise.
3. System Design and Architecture
As you progress in your programming career, the ability to design robust, scalable systems becomes increasingly important. This includes:
- Understanding how components interact within a system
- Designing for maintainability, scalability, and reliability
- Making appropriate tradeoffs based on requirements
- Creating clean abstractions and interfaces
These architectural skills have far more impact on code quality than knowledge of multiple languages.
4. Deep Mastery of Tools and Ecosystem
Becoming intimately familiar with your development environment and tools can dramatically improve your effectiveness:
- Mastering your IDE or editor and its shortcuts
- Understanding build systems and deployment pipelines
- Becoming proficient with debugging tools
- Knowing how to profile and optimize performance
A developer who can quickly navigate, debug, and optimize within their environment will outperform one who knows multiple languages but struggles with their tools.
When Learning Multiple Languages Is Valuable
This isn’t to say that learning multiple programming languages has no value. There are specific situations where exposure to different languages can significantly improve your programming skills:
1. Learning Different Programming Paradigms
Programming languages often embody different paradigms, each with its own approach to solving problems:
- Object-Oriented Programming (Java, C#, Ruby)
- Functional Programming (Haskell, Clojure, F#)
- Procedural Programming (C, Pascal)
- Logic Programming (Prolog)
- Concurrent Programming (Go, Erlang)
Exposure to different paradigms can broaden your thinking and provide new tools for approaching problems. For example, understanding functional programming concepts like immutability and higher-order functions can improve how you write code, even in object-oriented languages.
The key is to learn languages that represent genuinely different paradigms, rather than languages that are syntactically different but conceptually similar.
2. Understanding Language Design Tradeoffs
Each programming language makes design tradeoffs that influence how you solve problems. Learning about these tradeoffs can deepen your understanding of programming language design:
- Static vs. dynamic typing
- Memory management approaches (garbage collection vs. manual management)
- Concurrency models (threads, actors, CSP)
- Performance vs. developer productivity
Understanding why these tradeoffs exist helps you choose the right tool for specific problems and appreciate the reasoning behind language features.
3. Expanding Your Problem-Solving Toolkit
Different languages sometimes offer unique approaches to common problems. For instance:
- Pattern matching in languages like Rust and Elixir
- List comprehensions in Python
- Async/await patterns for handling asynchronous code
- Channel-based concurrency in Go
Exposure to these different approaches can enrich your problem-solving toolkit, even when working in your primary language.
The Strategic Approach to Language Learning
If you do decide to learn multiple programming languages, a strategic approach will yield better results than random language collection:
1. Master One Language First
Before branching out, achieve deep proficiency in at least one language. This means:
- Understanding the language’s idioms and best practices
- Being familiar with its standard library and common frameworks
- Knowing its performance characteristics and limitations
- Having built non-trivial projects with it
This deep knowledge provides a solid foundation and point of comparison for other languages.
2. Choose Languages for Specific Purposes
Rather than learning languages at random, select them based on what they can teach you:
- Learn a low-level language like C to understand memory management and system interactions
- Explore a functional language like Haskell to understand pure functions and immutability
- Try a concurrent language like Go to learn about channels and goroutines
Each new language should expand your understanding in a meaningful way.
3. Build Real Projects
Tutorials and exercises only take you so far. To truly understand a language, you need to build real projects that:
- Solve actual problems
- Require you to use the language’s ecosystem
- Involve error handling, testing, and other practical aspects
- Challenge you to write idiomatic code
These projects will reveal the true strengths and weaknesses of each language in a way that toy examples cannot.
4. Study Language Implementation
Understanding how programming languages are implemented can provide deeper insights:
- How compilers and interpreters work
- Runtime systems and memory management
- Performance optimization techniques
This knowledge helps you write more efficient code and better understand language behavior.
Common Pitfalls in the Multi-Language Approach
As you navigate your programming journey, be aware of these common pitfalls:
1. Resume-Driven Development
Learning languages solely to add them to your resume often leads to shallow knowledge. Employers are increasingly valuing depth over breadth, preferring candidates who can demonstrate mastery in relevant technologies rather than a laundry list of languages they’ve “worked with.”
2. Tutorial Hopping
Jumping from tutorial to tutorial across different languages can create the illusion of progress without building real skills. You might complete dozens of “Hello World” projects without ever facing the challenges of building and maintaining complex applications.
3. Neglecting Fundamentals
Focusing on language syntax at the expense of computer science fundamentals leaves you ill-equipped to solve difficult problems. A developer with strong algorithmic thinking and system design skills will outperform a syntax collector when faced with complex challenges.
4. Tool Obsession
Becoming overly focused on tools and languages rather than problem-solving can lead to a “hammer looking for nails” mentality. The best programmers choose their tools based on the problem at hand, not the other way around.
Real-World Perspectives
Let’s look at what successful developers and industry leaders say about language acquisition versus programming mastery:
“Focus on the programming fundamentals, because the languages are just tools.” — John Carmack, co-founder of id Software
“You can be a good programmer in any language, but you can’t be a good programmer in every language.” — Bjarne Stroustrup, creator of C++
“The most important skill for a programmer is the ability to effectively reason about systems.” — Kent Beck, creator of Extreme Programming
Many successful tech companies value depth over breadth. Google, for instance, primarily uses a handful of languages (Java, C++, Python, Go, JavaScript) rather than dozens. Their interview process focuses on problem-solving ability, algorithmic thinking, and system design rather than knowledge of numerous languages.
A Balanced Path Forward
So what’s the optimal approach to becoming a better programmer? Here’s a balanced strategy that combines depth with strategic breadth:
1. Build a Strong Foundation
Invest time in learning computer science fundamentals that transcend specific languages:
- Algorithms and data structures
- Computational complexity
- Computer architecture
- Operating systems concepts
- Database theory
These foundations will serve you throughout your career, regardless of which languages come and go.
2. Develop Deep Expertise
Choose one language that aligns with your interests and career goals, and become genuinely proficient in it:
- Learn its idioms and best practices
- Understand its internal workings
- Master its ecosystem and tools
- Build complex projects with it
- Read and understand sophisticated code written by experts
This deep knowledge will make you valuable and provide a solid reference point for learning other languages.
3. Expand Strategically
Once you have mastery in one area, expand your knowledge in ways that complement your expertise:
- Learn languages that represent different paradigms
- Explore domains adjacent to your specialty
- Study the historical context and evolution of programming languages
This strategic expansion builds meaningful connections between different areas of knowledge rather than isolated facts.
4. Focus on Projects, Not Languages
Let your learning be driven by the projects and problems you want to solve, not by a checklist of languages:
- Choose projects that challenge and interest you
- Select the best tools for each project
- Learn new languages when they’re the right tool for your goals
This project-centered approach ensures that your learning is practical and motivated by genuine interest rather than abstract collection.
Conclusion: Quality Over Quantity
In the pursuit of programming excellence, depth trumps breadth. While there’s value in understanding different programming paradigms and approaches, the obsession with collecting languages often leads to superficial knowledge that doesn’t translate to real programming proficiency.
The most valuable programmers aren’t those who know the most languages, but those who can effectively solve problems, design robust systems, and write clean, maintainable code. These skills come from deep understanding of programming fundamentals, intensive practice, and thoughtful application, not from syntax collection.
As you plan your learning journey, resist the urge to chase every new language or framework. Instead, invest in deep knowledge of fundamental concepts, master one area thoroughly, and expand your skills strategically based on genuine interest and practical needs.
Remember: programming is ultimately about solving problems, not knowing languages. The languages are just tools, and what matters most is your ability to use those tools effectively to create valuable software solutions.
The path to becoming a better coder isn’t about how many languages you know, but how well you can think, design, and solve problems with the languages you do know.