Why Learning Git is Non-Negotiable for Programmers
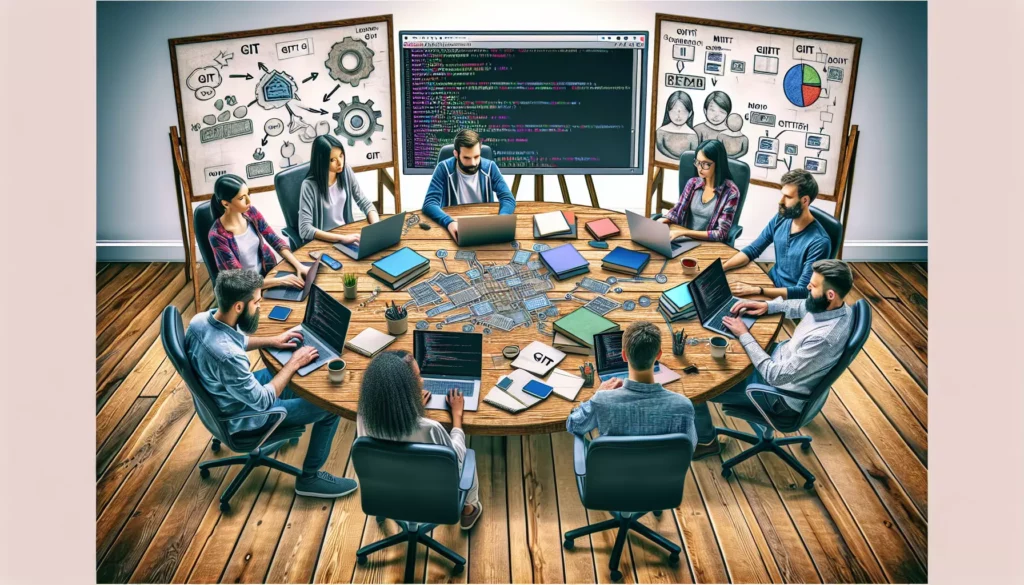
In the ever-evolving world of software development, certain tools and skills become indispensable for programmers. Among these, Git stands out as a cornerstone technology that every developer, from novice to expert, must master. As we delve into the reasons why learning Git is non-negotiable for programmers, we’ll explore its significance, benefits, and how it aligns with the broader goals of coding education and skills development.
What is Git?
Before we dive into the importance of Git, let’s briefly define what it is. Git is a distributed version control system designed to handle everything from small to very large projects with speed and efficiency. It was created by Linus Torvalds in 2005 for development of the Linux kernel, but has since become the most widely adopted version control system for software development.
The Ubiquity of Git in the Industry
One of the primary reasons learning Git is non-negotiable is its widespread adoption in the software industry. Nearly all major tech companies, including those often referred to as FAANG (Facebook, Amazon, Apple, Netflix, Google), use Git for version control. This ubiquity means that proficiency in Git is often a baseline expectation for job applicants in software development roles.
Consider these statistics:
- Over 90% of developers use Git, according to the Stack Overflow Developer Survey 2021.
- GitHub, the largest host of Git repositories, has over 73 million developers and more than 200 million repositories as of 2022.
- GitLab, another popular Git-based platform, is used by more than 100,000 organizations worldwide.
These numbers underscore the fact that Git is not just a tool, but a fundamental part of the modern software development ecosystem. Learning Git is akin to learning a universal language in the programming world – it’s how developers communicate, collaborate, and manage their work across the globe.
Version Control: The Backbone of Collaborative Development
At its core, Git is a version control system, and understanding version control is crucial for any programmer working on projects that evolve over time or involve collaboration with others. Here’s why version control, and by extension Git, is so important:
1. Tracking Changes
Git allows developers to track changes in their codebase over time. This means you can see what changes were made, when they were made, and by whom. This level of insight is invaluable for understanding the evolution of a project and for troubleshooting when issues arise.
2. Collaboration
In today’s interconnected world, software development is rarely a solo endeavor. Git facilitates collaboration by allowing multiple developers to work on the same project simultaneously. It provides mechanisms for merging changes from different sources and resolving conflicts when they occur.
3. Experimentation and Branching
Git’s branching model allows developers to experiment with new features or ideas without affecting the main codebase. This encourages innovation and reduces the fear of breaking existing functionality.
4. Code Reviews
Many Git-based platforms, like GitHub and GitLab, have built-in code review features. These allow team members to review each other’s code, provide feedback, and ensure quality before changes are merged into the main codebase.
5. Backup and Recovery
By using Git, developers always have a backup of their entire project history. If something goes wrong, it’s possible to revert to a previous state of the project with ease.
Git in the Context of Coding Education
When we consider the broader topic of coding education and programming skills development, Git plays a crucial role. Platforms like AlgoCademy, which focus on interactive coding tutorials and resources for learners, often incorporate Git into their curriculum for several reasons:
1. Real-world Skill Application
Learning Git provides students with a real-world skill that they’ll use in their professional careers. It bridges the gap between academic learning and industry practices.
2. Project Management
Git teaches students about project management in software development. It introduces concepts like branching strategies, which are essential for managing complex projects.
3. Collaboration Skills
By using Git, students learn how to collaborate effectively with others on coding projects. This is a crucial skill in the professional world, where teamwork is often the norm.
4. Problem-solving
Git introduces new types of problems to solve, such as merge conflicts. Resolving these issues helps develop critical thinking and problem-solving skills.
5. Portfolio Building
Many developers use Git repositories (often on platforms like GitHub) to showcase their work to potential employers. Learning Git enables students to start building their portfolio from day one.
Git and Algorithmic Thinking
While Git might not directly teach algorithms, it does reinforce certain aspects of algorithmic thinking that are valuable in programming:
1. State Management
Git’s commit history is essentially a series of states. Understanding how to navigate and manage these states is similar to thinking about state in algorithms and data structures.
2. Branching Logic
Git’s branching model is analogous to conditional logic in programming. Deciding when to create branches, how to name them, and when to merge them requires strategic thinking similar to designing control flow in algorithms.
3. Optimization
Advanced Git usage involves optimizing workflows, which is similar to optimizing algorithms. For example, choosing the right branching strategy for a project is akin to selecting the most efficient algorithm for a particular problem.
Git in Technical Interviews
For those preparing for technical interviews, especially at major tech companies, Git knowledge can be a significant advantage:
1. System Design Questions
In system design interviews, candidates might be asked about version control strategies for large-scale projects. Understanding Git’s distributed nature and branching strategies can be helpful in answering these questions.
2. Collaborative Coding Exercises
Some interviews involve collaborative coding exercises. Familiarity with Git can help candidates navigate these scenarios more comfortably.
3. Discussion of Past Projects
When discussing past projects, being able to talk about how version control was used demonstrates a professional approach to software development.
Getting Started with Git
For those convinced of the importance of Git but unsure where to start, here are some steps to begin your Git journey:
1. Install Git
First, you’ll need to install Git on your computer. You can download it from the official Git website: https://git-scm.com/
2. Learn the Basics
Start with the basic Git commands. Here’s a simple example of initializing a repository and making your first commit:
# Initialize a new Git repository
git init
# Add a file to the staging area
git add myfile.txt
# Commit the changes
git commit -m "My first commit"
3. Practice Branching
Create and switch between branches to understand how Git manages different lines of development:
# Create and switch to a new branch
git checkout -b my-new-feature
# Make some changes and commit them
git add .
git commit -m "Added new feature"
# Switch back to the main branch
git checkout main
4. Explore Online Resources
There are numerous online resources for learning Git, including:
- Git’s official documentation: https://git-scm.com/doc
- GitHub’s Git guides: https://github.com/git-guides
- Interactive tutorials like Learn Git Branching: https://learngitbranching.js.org/
5. Join Open Source Projects
Once you’re comfortable with the basics, consider contributing to open source projects on platforms like GitHub. This will give you real-world experience with Git in a collaborative environment.
Advanced Git Concepts
As you become more proficient with Git, you’ll want to explore more advanced concepts:
1. Rebasing
Rebasing is an alternative to merging that can create a cleaner project history. Here’s a basic example:
# While on your feature branch
git rebase main
2. Interactive Rebasing
Interactive rebasing allows you to modify commits in various ways as you move them to a new base. This is useful for cleaning up your commit history before merging:
git rebase -i HEAD~3
3. Cherry-picking
Cherry-picking allows you to pick individual commits from one branch and apply them to another:
git cherry-pick <commit-hash>
4. Submodules
Submodules allow you to keep a Git repository as a subdirectory of another Git repository. This is useful for including external dependencies in your project:
git submodule add <repository-url> <path>
5. Git Hooks
Git hooks are scripts that Git executes before or after events such as: commit, push, and receive. They’re useful for automating or customizing your Git workflow.
Git Best Practices
As you incorporate Git into your development workflow, keep these best practices in mind:
1. Commit Often
Make small, focused commits that encapsulate a single logical change. This makes it easier to understand the project history and to revert changes if necessary.
2. Write Good Commit Messages
Your commit messages should be clear and descriptive. A good format is a short (50 characters or less) summary line, followed by a blank line, followed by a more detailed explanation if necessary.
3. Use Branches
Use branches for new features, bug fixes, or experiments. This keeps the main branch stable and makes it easier to manage different aspects of development.
4. Pull Before You Push
Always pull the latest changes from the remote repository before pushing your own changes. This helps prevent merge conflicts.
5. Use .gitignore
Use a .gitignore file to exclude files that don’t need to be tracked, such as compiled binaries, temporary files, and sensitive information.
Git in the Larger Ecosystem
While Git is powerful on its own, it’s often used in conjunction with other tools and practices in the software development ecosystem:
1. Continuous Integration/Continuous Deployment (CI/CD)
Git integrates well with CI/CD pipelines. Changes pushed to certain branches can automatically trigger build processes, run tests, and deploy to staging or production environments.
2. Issue Tracking
Many Git platforms include issue tracking features. Commits can be linked to issues, providing traceability between code changes and the problems or features they address.
3. Code Review Platforms
Platforms like GitHub and GitLab provide robust code review features that integrate tightly with Git. These allow for detailed discussions about code changes before they’re merged.
4. Documentation
Git repositories often include documentation in formats like Markdown. This allows documentation to evolve alongside the code, ensuring it stays up-to-date.
Conclusion
In the realm of software development, Git has become as fundamental as the programming languages themselves. Its importance in version control, collaboration, and project management makes it a non-negotiable skill for programmers at all levels.
For those on the journey of coding education and skills development, learning Git is a crucial step. It not only provides practical skills that are immediately applicable in the industry but also reinforces important concepts in project management, collaboration, and problem-solving.
As you progress in your programming career, from tackling algorithmic challenges to preparing for technical interviews at major tech companies, your proficiency with Git will serve you well. It will help you manage your projects more effectively, collaborate more efficiently with others, and demonstrate your professional approach to software development.
Remember, mastering Git is not a destination but a journey. As you grow as a developer, you’ll continue to discover new ways to leverage Git to improve your workflow and productivity. Embrace this powerful tool, and let it support you in your quest to become a more skilled and effective programmer.