Why Learning Event-Driven Architecture is Crucial for System Design
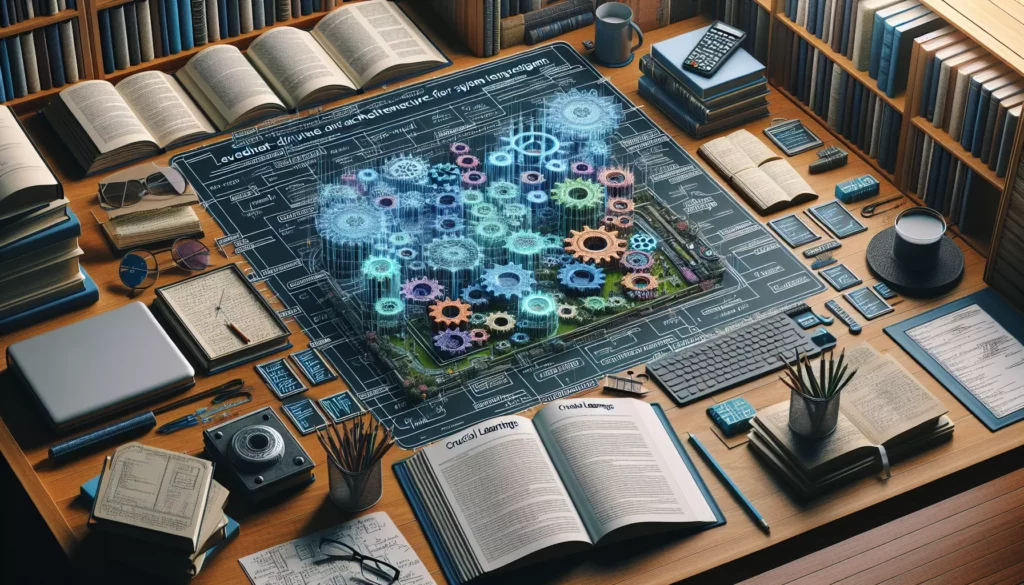
In the ever-evolving landscape of software development, staying ahead of the curve is essential for both aspiring and seasoned programmers. As we dive deeper into the world of distributed systems and microservices, one architectural pattern has emerged as a game-changer: Event-Driven Architecture (EDA). This blog post will explore why learning Event-Driven Architecture is crucial for system design and how it can significantly impact your career as a software engineer, especially when preparing for technical interviews at major tech companies.
What is Event-Driven Architecture?
Before we delve into the importance of Event-Driven Architecture, let’s first understand what it is. Event-Driven Architecture is a software design pattern in which the flow of the program is determined by events such as user actions, sensor outputs, or messages from other programs or services. In this paradigm, the system produces, detects, consumes, and reacts to events.
An event can be defined as a significant change in state. For example, when a customer places an order, that’s an event. When an IoT device registers a temperature change, that’s an event. When a user clicks a button on a website, that’s an event too.
The core components of an Event-Driven Architecture typically include:
- Event producers: Components that generate events
- Event channels: Mechanisms for transmitting events from producers to consumers
- Event consumers: Components that receive and process events
- Event processing engines: Systems that analyze and act on events
Why is Event-Driven Architecture Important?
Now that we have a basic understanding of what Event-Driven Architecture is, let’s explore why it’s becoming increasingly important in modern system design:
1. Scalability
One of the primary advantages of Event-Driven Architecture is its inherent scalability. In a traditional monolithic architecture, scaling often means duplicating the entire application. However, with EDA, you can scale individual components independently based on their specific needs. This granular scalability allows for more efficient resource utilization and cost-effective operations.
For example, imagine an e-commerce platform where the order processing service experiences high load during holiday seasons. In an Event-Driven Architecture, you can scale up just the order processing service without affecting other parts of the system.
2. Loose Coupling
Event-Driven Architecture promotes loose coupling between components. Services in an EDA system communicate through events, which means they don’t need to have direct knowledge of each other. This decoupling makes the system more flexible and easier to maintain and evolve over time.
Consider a scenario where you need to add a new feature to your application, such as a recommendation engine. In a tightly coupled system, this might require changes to multiple services. However, in an EDA system, you could simply add a new service that listens to relevant events without modifying existing components.
3. Real-time Processing
In today’s fast-paced digital world, real-time data processing is often a requirement rather than a luxury. Event-Driven Architecture excels at handling real-time scenarios. As events occur, they can be immediately processed and acted upon, enabling systems to respond quickly to changing conditions.
This capability is particularly valuable in domains such as financial trading, where milliseconds can make a difference, or in IoT applications where immediate responses to sensor data are crucial.
4. Improved Fault Tolerance
Event-Driven Architecture can enhance a system’s fault tolerance. Because components are loosely coupled, the failure of one component doesn’t necessarily bring down the entire system. Events can be persisted and replayed, allowing the system to recover from failures more gracefully.
For instance, if an order processing service goes down temporarily, incoming order events can be queued and processed once the service is back online, ensuring no orders are lost.
5. Better Adaptability to Change
In the fast-moving tech industry, the ability to adapt quickly to changing requirements is crucial. Event-Driven Architecture provides a flexible foundation that can accommodate changes more easily than traditional architectures. New functionality can often be added by creating new event consumers without modifying existing code.
Event-Driven Architecture in Practice
To better understand how Event-Driven Architecture works in practice, let’s consider a simple example of an online bookstore system:
// Event Producer: Order Service
function placeOrder(order) {
// Process the order
const orderEvent = {
type: "ORDER_PLACED",
data: order
};
publishEvent(orderEvent);
}
// Event Consumer: Inventory Service
function handleOrderPlaced(event) {
if (event.type === "ORDER_PLACED") {
const order = event.data;
// Update inventory
updateInventory(order.items);
}
}
// Event Consumer: Shipping Service
function handleOrderPlaced(event) {
if (event.type === "ORDER_PLACED") {
const order = event.data;
// Initiate shipping process
initiateShipping(order);
}
}
// Event Consumer: Notification Service
function handleOrderPlaced(event) {
if (event.type === "ORDER_PLACED") {
const order = event.data;
// Send confirmation email
sendOrderConfirmation(order.customerEmail);
}
}
In this example, when an order is placed, the Order Service publishes an “ORDER_PLACED” event. Multiple services (Inventory, Shipping, Notification) can independently consume this event and perform their respective actions without direct communication with each other or the Order Service.
Challenges of Event-Driven Architecture
While Event-Driven Architecture offers numerous benefits, it’s important to be aware of its challenges:
1. Complexity
Event-Driven systems can be more complex to design, implement, and debug compared to traditional monolithic architectures. The asynchronous nature of event processing can make it harder to trace the flow of operations and understand the system’s behavior.
2. Eventual Consistency
In distributed Event-Driven systems, achieving strong consistency can be challenging. Often, these systems rely on eventual consistency, which means that the system will become consistent over time, but there may be periods where different parts of the system have different views of the data.
3. Event Schema Evolution
As the system evolves, the structure of events may need to change. Managing these changes while ensuring backward compatibility can be complex.
4. Ordering and Exactly-Once Processing
Ensuring that events are processed in the correct order and exactly once can be challenging, especially in distributed environments with potential network issues or service failures.
Event-Driven Architecture and FAANG Companies
Understanding Event-Driven Architecture is particularly valuable when preparing for technical interviews at major tech companies, often referred to as FAANG (Facebook, Amazon, Apple, Netflix, Google) or MANGA (Microsoft, Amazon, Netflix, Google, Apple). These companies often deal with large-scale, distributed systems where Event-Driven Architecture plays a crucial role.
Amazon
Amazon extensively uses Event-Driven Architecture in its e-commerce platform and AWS services. For example, Amazon’s Simple Notification Service (SNS) and Simple Queue Service (SQS) are building blocks for creating event-driven systems.
Netflix
Netflix’s microservices architecture heavily relies on event-driven patterns. They use Apache Kafka for their event streaming platform, enabling real-time data processing for recommendations, content delivery, and more.
Google Cloud Pub/Sub is a fully-managed real-time messaging service that allows you to send and receive messages between independent applications, showcasing Google’s commitment to event-driven systems.
Facebook uses a custom-built event streaming platform called Scribe for logging and real-time data processing across its massive infrastructure.
Preparing for Technical Interviews
When preparing for technical interviews, especially at FAANG companies, consider the following aspects of Event-Driven Architecture:
1. System Design Questions
Be prepared to discuss how you would design large-scale systems using Event-Driven Architecture. Practice explaining the benefits and trade-offs of this approach.
2. Scalability
Understand how Event-Driven Architecture contributes to system scalability and be ready to discuss scenarios where it would be particularly beneficial.
3. Fault Tolerance
Be prepared to explain how Event-Driven Architecture can improve system reliability and how you would handle failures in an event-driven system.
4. Consistency Models
Understand different consistency models (strong consistency, eventual consistency) and their implications in event-driven systems.
5. Event Sourcing and CQRS
Familiarize yourself with related patterns like Event Sourcing and Command Query Responsibility Segregation (CQRS), which are often used in conjunction with Event-Driven Architecture.
Practical Learning Approaches
To gain practical experience with Event-Driven Architecture, consider the following approaches:
1. Build a Project
Develop a small-scale event-driven application. This could be a simple chat application, a task management system, or a basic e-commerce platform. Use technologies like Apache Kafka, RabbitMQ, or cloud services like AWS SNS/SQS to implement the event-driven components.
2. Contribute to Open Source
Look for open-source projects that use Event-Driven Architecture and contribute to them. This will give you exposure to real-world implementations and best practices.
3. Online Courses and Tutorials
Platforms like Coursera, edX, and Udemy offer courses on Event-Driven Architecture and related technologies. These can provide structured learning paths and hands-on exercises.
4. Read Case Studies
Study how large companies have implemented Event-Driven Architecture. Many tech companies publish engineering blog posts and case studies detailing their architectures.
Coding Challenge: Implementing a Simple Event Bus
To get hands-on experience with the concepts of Event-Driven Architecture, let’s implement a simple event bus in JavaScript. This event bus will allow components to publish events and subscribe to them.
class EventBus {
constructor() {
this.subscribers = {};
}
subscribe(event, callback) {
if (!this.subscribers[event]) {
this.subscribers[event] = [];
}
this.subscribers[event].push(callback);
}
publish(event, data) {
if (!this.subscribers[event]) {
return;
}
this.subscribers[event].forEach(callback => callback(data));
}
}
// Usage example
const eventBus = new EventBus();
// Order Service
function placeOrder(order) {
console.log("Order placed:", order);
eventBus.publish("ORDER_PLACED", order);
}
// Inventory Service
eventBus.subscribe("ORDER_PLACED", (order) => {
console.log("Updating inventory for order:", order.id);
});
// Shipping Service
eventBus.subscribe("ORDER_PLACED", (order) => {
console.log("Initiating shipping for order:", order.id);
});
// Place an order
placeOrder({ id: 1, items: ["book", "pen"] });
This simple implementation demonstrates the core concepts of event publishing and subscription. In a real-world scenario, you’d need to consider additional factors like error handling, event persistence, and scalability.
Conclusion
Event-Driven Architecture is more than just a trendy concept in software development; it’s a fundamental shift in how we design and build scalable, resilient, and flexible systems. As the demand for real-time, highly available applications continues to grow, the importance of Event-Driven Architecture in system design will only increase.
For aspiring software engineers and those preparing for technical interviews at top tech companies, understanding Event-Driven Architecture is no longer optional—it’s essential. It not only demonstrates your ability to think at scale but also shows that you’re in tune with modern software development practices.
Remember, learning Event-Driven Architecture is not just about understanding the theory. It’s about applying these concepts in practical scenarios, solving real-world problems, and continuously refining your approach based on new challenges and experiences.
As you continue your journey in software development, make Event-Driven Architecture a key part of your toolkit. Practice designing event-driven systems, experiment with different event-streaming technologies, and always be on the lookout for scenarios where this architectural pattern can add value.
By mastering Event-Driven Architecture, you’ll not only be better prepared for technical interviews but also equipped to build the next generation of scalable, responsive, and robust software systems. Happy coding!