Why Learning Data Structures and Algorithms is Essential for Programmers
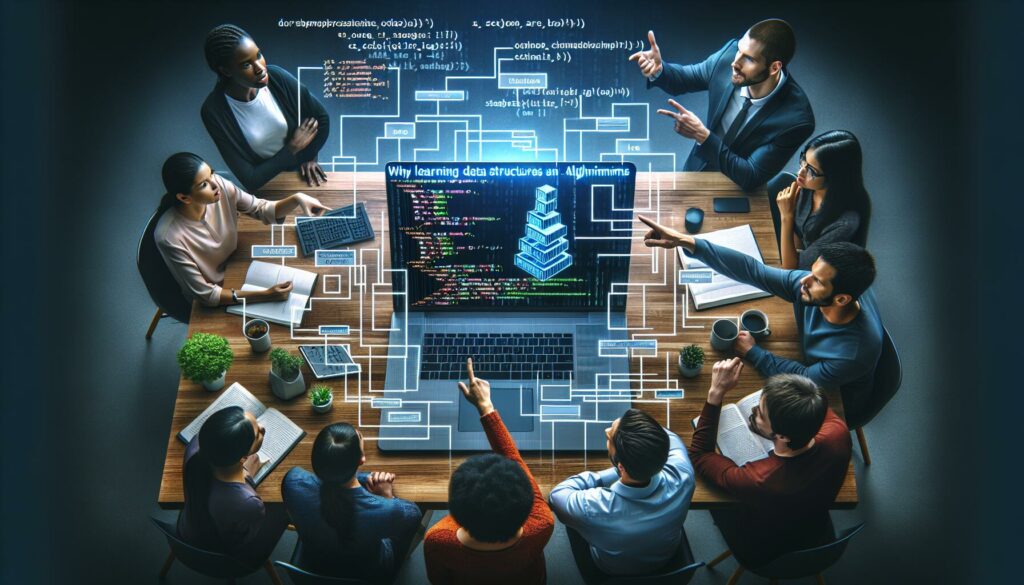
As a programmer, you may have asked yourself, “Why do I need to learn data structures and algorithms? How does that help me if I already know Python and a little bit of JavaScript?” This is a common question among developers, especially those who are comfortable in their current roles but aspire to advance their careers. In this comprehensive guide, we’ll explore the importance of data structures and algorithms (DS&A) and how they can benefit you as a programmer.
The Immediate Benefits of Learning DS&A
Before diving into the deeper reasons, let’s address the most obvious benefit of learning data structures and algorithms:
1. Career Advancement
Learning DS&A can significantly improve your career prospects in several ways:
- Better Job Opportunities: Many top-tier tech companies include DS&A questions in their interview process.
- Higher Salaries: Positions that require DS&A knowledge often come with higher compensation packages.
- Prestigious Companies: FAANG (Facebook, Amazon, Apple, Netflix, Google) and other leading tech firms often seek candidates with strong DS&A skills.
- Professional Growth: Working alongside highly skilled peers can accelerate your learning and career development.
- Promotion Potential: Strong DS&A skills can set you apart and increase your chances of advancement within your organization.
While these reasons alone might be sufficient motivation for many, the benefits of learning DS&A extend far beyond just landing a job or getting a promotion.
The Hidden Value of Data Structures and Algorithms
Beyond the immediate career benefits, understanding DS&A provides several advantages that can make you a more effective and valuable programmer:
2. Solving Complex Problems
Large-scale companies face unique challenges due to their massive user bases and complex systems. When you work for organizations like Meta, Amazon, Netflix, or Google, you’ll encounter problems that require efficient, robust, and well-designed solutions. Your code needs to be:
- Highly efficient to handle millions of users
- Robust enough to withstand various edge cases
- Well-designed for maintainability and scalability
- Readable and understandable by other developers
A strong foundation in DS&A equips you with the tools to tackle these complex problems effectively.
3. Understanding the Purpose Behind DS&A
Each data structure and algorithm was created to solve specific problems. They weren’t invented arbitrarily but developed to address real-world challenges. Understanding their purposes can help you choose the right tool for the job. Here are some practical applications:
- Linked Lists: Used in browser history (back/forward navigation) and music playlists (previous/next functionality)
- Graphs: Employed in social networks (Facebook’s friend connections) and mapping applications (Google Maps)
- Dijkstra’s Algorithm: Used for finding the shortest path in mapping applications
4. Building Custom Solutions
While smaller companies or projects might rely heavily on existing libraries and frameworks, large tech companies often need to create custom solutions. This is because:
- Off-the-shelf solutions may not meet their specific needs
- Custom solutions can be optimized for their particular use cases
- Even small performance improvements can have a massive impact at scale
To build these custom libraries and frameworks, you need a deep understanding of DS&A. This knowledge allows you to create efficient, tailored solutions that can outperform generic alternatives.
Real-World Applications of Data Structures and Algorithms
To further illustrate the importance of DS&A, let’s explore some real-world applications in more detail:
5. Social Media Platforms
Social media giants like Facebook (Meta) rely heavily on graph theory and associated algorithms:
- Friend Recommendations: Graph traversal algorithms help suggest potential connections
- News Feed Generation: Sorting and filtering algorithms determine what content to show users
- Content Distribution: Network flow algorithms optimize how information spreads across the platform
6. E-commerce Platforms
Companies like Amazon use various DS&A concepts to enhance user experience and optimize operations:
- Product Recommendations: Collaborative filtering algorithms suggest items based on user behavior
- Inventory Management: Tree structures and search algorithms efficiently track and locate products
- Shipping Route Optimization: Graph algorithms determine the most efficient delivery paths
7. Streaming Services
Platforms like Netflix employ DS&A to provide a seamless viewing experience:
- Content Delivery: Caching algorithms ensure fast loading times for popular content
- Recommendation Systems: Machine learning algorithms, often built on DS&A principles, suggest shows and movies
- Video Encoding: Compression algorithms reduce file sizes while maintaining quality
8. Search Engines
Google and other search engines rely heavily on advanced DS&A:
- Web Crawling: Graph traversal algorithms explore and index the web
- Page Ranking: Algorithms like PageRank determine the relevance and importance of web pages
- Query Processing: Efficient data structures enable quick retrieval of search results
The Long-Term Benefits of DS&A Proficiency
Beyond solving immediate problems, mastering data structures and algorithms offers several long-term benefits:
9. Problem-Solving Skills
Studying DS&A enhances your ability to:
- Break down complex problems into manageable components
- Analyze the efficiency of different approaches
- Develop optimal solutions by considering various trade-offs
These skills are transferable to many aspects of programming and can make you a more effective problem-solver in general.
10. Code Optimization
Understanding DS&A allows you to write more efficient code by:
- Choosing the most appropriate data structures for specific tasks
- Implementing algorithms that minimize time and space complexity
- Identifying and eliminating performance bottlenecks in existing code
11. System Design Skills
DS&A knowledge is crucial for effective system design, enabling you to:
- Architect scalable and efficient software systems
- Make informed decisions about data storage and retrieval mechanisms
- Design APIs and interfaces that are both powerful and easy to use
12. Understanding Lower-Level Operations
Studying DS&A provides insights into how computers work at a lower level, helping you:
- Understand memory management and allocation
- Appreciate the impact of hardware limitations on software performance
- Make more informed decisions about language and technology choices
Overcoming Common Misconceptions
Despite the clear benefits, some programmers still hesitate to invest time in learning DS&A. Let’s address some common misconceptions:
13. “I Don’t Use DS&A in My Daily Work”
While you may not explicitly implement complex data structures or algorithms daily, the principles behind them inform many aspects of programming:
- Choosing the right data structure (e.g., array vs. object) affects code efficiency
- Understanding time complexity helps you write faster code, even for simple tasks
- Algorithmic thinking improves your overall problem-solving approach
14. “Libraries and Frameworks Handle This for Me”
While it’s true that many libraries implement complex DS&A for you, understanding these concepts is still valuable:
- It helps you choose the right library or function for your specific needs
- You can better understand and debug issues when they arise
- In performance-critical situations, you may need to implement custom solutions
15. “I’m Not Interested in Working for Big Tech Companies”
Even if you don’t aspire to work at FAANG companies, DS&A knowledge is valuable:
- Many mid-sized companies also value these skills
- Startups often face scaling challenges that require DS&A expertise
- The problem-solving skills you gain are universally applicable
How to Approach Learning Data Structures and Algorithms
If you’re convinced of the importance of DS&A but unsure where to start, consider the following approach:
16. Start with the Basics
- Learn fundamental data structures: arrays, linked lists, stacks, queues, trees, and graphs
- Study basic algorithms: sorting, searching, and traversal algorithms
- Understand Big O notation and time complexity analysis
17. Practice Regularly
- Solve problems on platforms like LeetCode, HackerRank, or CodeSignal
- Implement data structures and algorithms from scratch to deepen your understanding
- Participate in coding contests to challenge yourself
18. Apply Your Knowledge
- Look for opportunities to optimize your existing code using DS&A principles
- Contribute to open-source projects that involve complex algorithms
- Build side projects that require you to implement advanced data structures or algorithms
19. Stay Updated
- Follow developments in the field of algorithms and data structures
- Read research papers or articles about new algorithmic approaches
- Attend conferences or workshops focused on algorithms and performance optimization
Conclusion
Learning data structures and algorithms is not just about passing technical interviews or landing a job at a prestigious tech company. It’s about becoming a more skilled, efficient, and versatile programmer. The knowledge and problem-solving skills you gain from studying DS&A will serve you throughout your career, regardless of the specific technologies or domains you work with.
By understanding the fundamental principles behind efficient code and system design, you’ll be better equipped to tackle complex problems, optimize performance, and create robust, scalable solutions. Whether you’re building the next big social media platform, optimizing an e-commerce system, or developing innovative software for a startup, a strong foundation in data structures and algorithms will be invaluable.
So, embrace the challenge of learning DS&A. It may seem daunting at first, but the long-term benefits to your skills, career, and overall effectiveness as a programmer are well worth the effort. Remember, every expert was once a beginner, and with consistent practice and application, you too can master these fundamental concepts and take your programming skills to the next level.