Why Learning Basic Syntax is Crucial for Aspiring Programmers
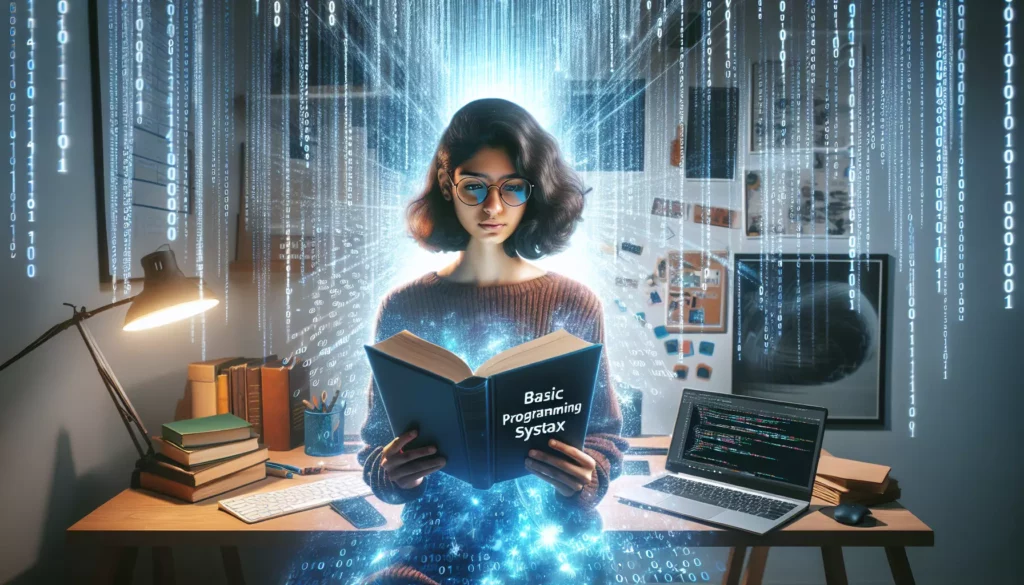
In the ever-evolving world of technology, programming has become an essential skill for many careers. Whether you’re aiming to become a software developer, data scientist, or even a digital marketer, understanding how to code can significantly boost your career prospects. However, the journey to becoming a proficient programmer starts with mastering the basics, and one of the most fundamental aspects of coding is understanding syntax.
At AlgoCademy, we believe that a strong foundation in basic syntax is crucial for aspiring programmers. This article will explore why syntax is so important, how it impacts your coding journey, and provide practical tips for mastering it. Whether you’re just starting out or looking to refine your skills for technical interviews at major tech companies, this guide will help you appreciate the significance of syntax in programming.
What is Syntax in Programming?
Before we dive into why syntax is crucial, let’s define what it actually means in the context of programming. Syntax refers to the set of rules that define how programs written in a specific programming language should be structured. It’s essentially the grammar of a programming language, dictating how words (keywords), punctuation, and symbols should be arranged to create valid code.
Just as natural languages have rules for sentence structure and grammar, programming languages have their own syntactical rules. These rules determine how you should write your code to ensure it can be correctly interpreted and executed by the computer.
The Importance of Syntax in Programming
Now that we understand what syntax is, let’s explore why it’s so crucial for aspiring programmers:
1. Foundation for Learning
Syntax forms the foundation of any programming language. Without a solid understanding of syntax, it’s challenging to write even the simplest programs. It’s like trying to write a sentence without knowing how to structure words or use punctuation correctly. Mastering syntax is the first step towards becoming proficient in a programming language.
2. Error Prevention
Proper syntax helps prevent errors in your code. Many programming errors, especially for beginners, are syntax errors. These occur when the code doesn’t follow the language’s syntactical rules. By understanding and following correct syntax, you can avoid many common errors, saving time and frustration during the debugging process.
3. Code Readability
Good syntax practices lead to more readable code. When you follow syntactical conventions, your code becomes easier for others (and your future self) to read and understand. This is crucial in collaborative environments and for maintaining code over time.
4. Efficient Learning of Multiple Languages
While each programming language has its own syntax, many share similar concepts. Once you’ve mastered the syntax of one language, it becomes easier to learn others. You’ll start recognizing patterns and similarities, making the learning process more efficient.
5. Problem-Solving Focus
When syntax becomes second nature, you can focus more on problem-solving and algorithm development. Instead of getting caught up in how to write the code, you can concentrate on what the code should do.
6. Preparation for Advanced Concepts
Many advanced programming concepts build upon basic syntax. Without a solid foundation, it becomes challenging to grasp more complex ideas. Mastering syntax prepares you for learning advanced topics like object-oriented programming, functional programming, and design patterns.
Common Syntactical Elements Across Programming Languages
While syntax varies between programming languages, there are some common elements you’ll encounter in most languages. Understanding these can help you grasp the importance of syntax and how it’s applied:
1. Variables and Data Types
Almost all programming languages use variables to store data. The syntax for declaring variables and specifying their data types is crucial. For example, in Python, you might declare a variable like this:
x = 5 # An integer variable
name = "John" # A string variable
While in a language like Java, you’d need to specify the data type:
int x = 5;
String name = "John";
2. Control Structures
Control structures like if-else statements, loops, and switch cases are fundamental to programming logic. The syntax for these structures can vary significantly between languages. For instance, an if-else statement in Python looks like this:
if x > 0:
print("Positive")
else:
print("Non-positive")
While in JavaScript, it would be:
if (x > 0) {
console.log("Positive");
} else {
console.log("Non-positive");
}
3. Functions and Methods
Functions are blocks of reusable code. The syntax for defining and calling functions is crucial. In Python, you might define a function like this:
def greet(name):
return f"Hello, {name}!"
print(greet("Alice"))
In Java, the same function would look like:
public static String greet(String name) {
return "Hello, " + name + "!";
}
System.out.println(greet("Alice"));
4. Comments
Comments are crucial for explaining code and improving readability. Most languages have syntax for single-line and multi-line comments. For example, in many languages including Java, JavaScript, and C++:
// This is a single-line comment
/*
This is a
multi-line comment
*/
5. Object-Oriented Programming (OOP) Syntax
For languages that support OOP, understanding the syntax for creating classes, objects, and implementing inheritance is important. Here’s a simple class definition in Python:
class Dog:
def __init__(self, name):
self.name = name
def bark(self):
return f"{self.name} says Woof!"
my_dog = Dog("Buddy")
print(my_dog.bark())
The Impact of Syntax on Code Execution
Understanding how syntax affects code execution is crucial for writing efficient and error-free programs. Here are some ways in which syntax impacts code execution:
1. Compilation vs. Interpretation
Different programming languages use different methods to convert source code into machine-readable instructions. Some languages are compiled, while others are interpreted. The syntax of a language is closely tied to how it’s processed:
- Compiled Languages: Languages like C++ and Java are compiled. The compiler checks the syntax during the compilation process. If there are syntax errors, the compilation fails, and the program won’t run at all.
- Interpreted Languages: Languages like Python and JavaScript are interpreted. While they may have a compilation step, syntax errors might not be caught until the code is actually run, which can lead to runtime errors.
2. Performance Implications
While syntax itself doesn’t directly affect performance, certain syntactical choices can impact how efficiently your code runs. For example:
- In Python, using list comprehensions instead of traditional for loops can often lead to more efficient code execution.
- In JavaScript, using the appropriate loop structure (for, while, for…of, etc.) based on your specific use case can impact performance.
3. Memory Management
Some syntactical elements in programming languages are directly related to memory management. For instance:
- In C++, the syntax for pointers and memory allocation (using
new
anddelete
) directly impacts how memory is used and freed. - In Python, the syntax for creating and using objects affects how memory is allocated and managed by the interpreter.
4. Error Handling
The syntax for error handling varies between languages but is crucial for writing robust code. For example:
try:
# Some code that might raise an exception
result = 10 / 0
except ZeroDivisionError:
print("Cannot divide by zero!")
finally:
print("This will always execute")
Understanding this syntax is essential for gracefully handling errors and exceptions in your programs.
Best Practices for Learning and Mastering Syntax
Now that we’ve established the importance of syntax, let’s look at some best practices for learning and mastering it:
1. Start with One Language
While it’s tempting to try learning multiple languages at once, it’s often more effective to focus on mastering the syntax of one language before moving on to others. This allows you to build a strong foundation and develop good coding habits.
2. Practice Regularly
Consistency is key when learning syntax. Set aside time each day to write code, even if it’s just for 30 minutes. Regular practice helps reinforce syntactical rules and makes them second nature.
3. Use Coding Challenges and Exercises
Platforms like AlgoCademy offer coding challenges and exercises that can help you practice syntax in context. These challenges often require you to apply syntactical knowledge to solve real-world problems, reinforcing your learning.
4. Read and Analyze Code
Reading code written by experienced programmers can help you understand how syntax is applied in practice. Look for open-source projects or code snippets in documentation to see how professional developers structure their code.
5. Leverage IDE Features
Modern Integrated Development Environments (IDEs) often include features like syntax highlighting, auto-completion, and error detection. Use these tools to help you identify and correct syntax errors as you write code.
6. Understand the “Why” Behind Syntax Rules
Don’t just memorize syntax rules; try to understand why they exist. This deeper understanding will help you remember the rules and apply them more effectively.
7. Write Documentation and Comments
Practice writing clear, concise comments and documentation for your code. This not only helps others understand your code but also reinforces your own understanding of the syntax you’re using.
8. Participate in Code Reviews
If possible, participate in code reviews or pair programming sessions. Reviewing others’ code and having your code reviewed can help you identify syntactical improvements and learn best practices.
9. Stay Updated
Programming languages evolve, and syntax can change with new versions. Stay informed about updates to your chosen language and be prepared to adapt your coding style accordingly.
Common Syntax Mistakes to Avoid
Even experienced programmers can make syntax errors. Here are some common mistakes to watch out for:
1. Mismatched Parentheses, Brackets, or Braces
Ensure that all opening parentheses, brackets, and braces have matching closing ones. Many IDEs provide features to help with this, but it’s still a common source of errors.
2. Incorrect Indentation
In languages like Python, where indentation is significant, incorrect indentation can lead to logical errors or syntax errors. Be consistent with your indentation style.
3. Missing Semicolons
In languages that require semicolons to end statements (like JavaScript or Java), forgetting to add them is a common mistake.
4. Case Sensitivity Issues
Many programming languages are case-sensitive. Mixing up the case of variables, function names, or keywords can lead to errors.
5. Using the Wrong Operator
Confusing assignment operators (=) with comparison operators (==) is a common mistake that can lead to logical errors in your code.
6. Incorrect String Concatenation
Different languages have different ways of concatenating strings. Using the wrong method can result in syntax errors or unexpected output.
7. Misusing Single and Double Quotes
Some languages treat single and double quotes differently. Using them incorrectly can lead to syntax errors or unexpected string behavior.
The Role of Syntax in Technical Interviews
For aspiring programmers aiming to work at major tech companies (often referred to as FAANG – Facebook, Amazon, Apple, Netflix, Google), understanding syntax is crucial during technical interviews. Here’s why:
1. First Impressions Matter
Writing syntactically correct code during an interview demonstrates your proficiency and attention to detail. It shows that you have a solid grasp of the basics, which is essential for more complex problem-solving.
2. Focus on Problem-Solving
When syntax comes naturally to you, you can focus more on the problem-solving aspects of the interview questions. This allows you to showcase your algorithmic thinking and coding skills more effectively.
3. Efficient Whiteboard Coding
Many technical interviews involve whiteboard coding. Being able to write syntactically correct code without the aid of an IDE is a valuable skill that comes from a deep understanding of syntax.
4. Adaptability
Interviewers may ask you to solve problems in a language you’re less familiar with. A strong grasp of general programming syntax can help you adapt more quickly to different languages.
5. Code Readability
Clean, well-structured code is highly valued in technical interviews. Proper syntax contributes significantly to code readability, making it easier for interviewers to understand your solution.
Conclusion
Mastering basic syntax is a crucial step for any aspiring programmer. It forms the foundation upon which all other programming skills are built. By understanding and correctly applying syntax, you can write more efficient, readable, and error-free code. This not only makes you a better programmer but also prepares you for more advanced concepts and challenges in your coding journey.
At AlgoCademy, we emphasize the importance of syntax in our interactive coding tutorials and resources. We believe that a strong foundation in syntax, combined with problem-solving skills and algorithmic thinking, is key to success in programming, whether you’re just starting out or preparing for technical interviews at top tech companies.
Remember, learning syntax is an ongoing process. As you continue to code and explore different languages, your understanding of syntax will deepen. Embrace this learning journey, practice regularly, and don’t be afraid to make mistakes – they’re all part of the learning process. With persistence and dedication, you’ll find that mastering syntax becomes second nature, allowing you to focus on the creative and problem-solving aspects of programming that make it such an exciting and rewarding field.