Why Knowing Multiple Languages Could Be a Disadvantage in Coding Education
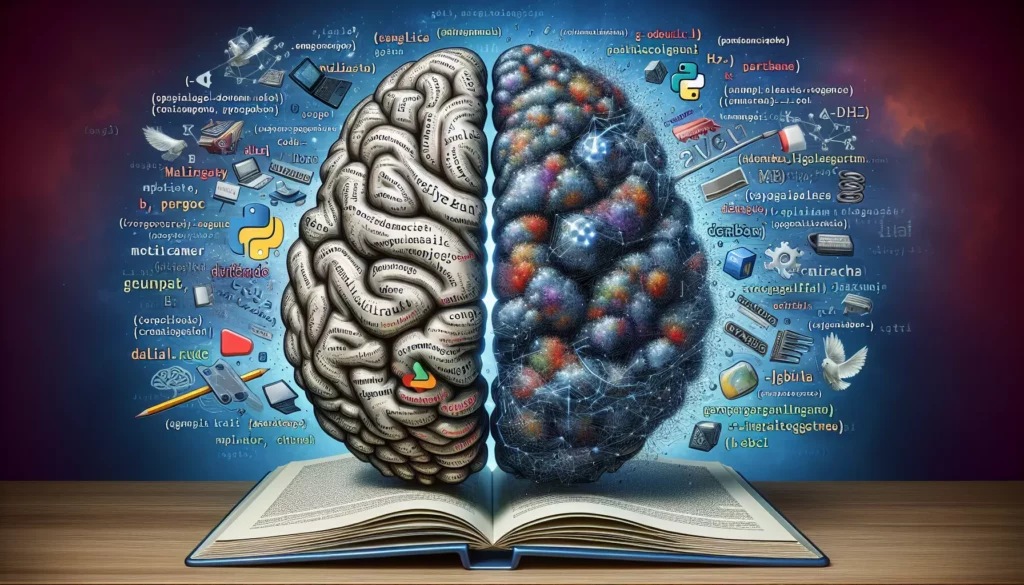
In the ever-evolving world of technology and programming, the ability to speak multiple coding languages is often touted as a valuable skill. However, when it comes to coding education and the journey of becoming a proficient programmer, particularly in the context of preparing for technical interviews at major tech companies, knowing multiple languages could potentially be a disadvantage. This article explores the reasons why focusing on a single language might be more beneficial, especially for those using platforms like AlgoCademy to develop their programming skills.
The Allure of Multilingualism in Programming
Before diving into the potential drawbacks, it’s important to acknowledge why many aspiring programmers are drawn to learning multiple languages:
- Versatility: Different languages are suited for different tasks and platforms.
- Marketability: Job listings often mention multiple languages as requirements or preferences.
- Intellectual curiosity: Learning new languages can be intellectually stimulating.
- Fear of obsolescence: Concerns about a particular language becoming outdated.
While these reasons are valid to some extent, they may not always align with the most effective path to becoming a proficient programmer, especially when preparing for technical interviews at companies like those in the FAANG (Facebook, Amazon, Apple, Netflix, Google) group.
The Case for Language Monogamy in Coding Education
1. Depth Over Breadth
When learning to code, especially in the early stages, depth of knowledge is often more valuable than breadth. Platforms like AlgoCademy emphasize the importance of understanding fundamental concepts and developing strong problem-solving skills. These skills are largely language-agnostic and are better cultivated by diving deep into a single language.
Consider the following example of a simple algorithm implementation in Python:
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
# Usage
sorted_array = [1, 3, 5, 7, 9, 11, 13, 15]
result = binary_search(sorted_array, 7)
print(f"Element found at index: {result}")
Understanding the logic behind this binary search implementation is far more crucial than knowing how to write it in multiple languages. The core concept remains the same across languages, and mastering it in one language allows for easier adaptation to others when necessary.
2. Avoiding Cognitive Overload
Learning to code is challenging, and introducing multiple languages simultaneously can lead to cognitive overload. This is especially true for beginners who are still grappling with fundamental programming concepts. By focusing on a single language, learners can:
- Solidify their understanding of core programming principles
- Build muscle memory for syntax and common patterns
- Reduce confusion caused by language-specific quirks and differences
For instance, consider the difference in handling null or undefined values:
// JavaScript
let value = null;
console.log(value ?? "Default"); // Output: "Default"
# Python
value = None
print(value or "Default") # Output: "Default"
While both languages have ways to handle null/None values, the syntax and exact behavior differ. Focusing on one language at a time allows learners to internalize these patterns without the confusion of juggling multiple syntaxes.
3. Faster Progress in Algorithmic Thinking
AlgoCademy and similar platforms place a strong emphasis on developing algorithmic thinking and problem-solving skills. These skills are best honed through consistent practice and iterative improvement. By sticking to one language, learners can:
- Progress more quickly through increasingly complex problems
- Focus on optimizing their solutions rather than translating between languages
- Develop a deeper intuition for performance and efficiency within that language’s ecosystem
Let’s look at an example of how focusing on one language allows for quicker progress in understanding and optimizing algorithms:
def fibonacci_recursive(n):
if n <= 1:
return n
return fibonacci_recursive(n-1) + fibonacci_recursive(n-2)
def fibonacci_dynamic(n):
if n <= 1:
return n
fib = [0] * (n + 1)
fib[1] = 1
for i in range(2, n + 1):
fib[i] = fib[i-1] + fib[i-2]
return fib[n]
# Comparison
import time
n = 30
start = time.time()
print(f"Recursive result: {fibonacci_recursive(n)}")
print(f"Recursive time: {time.time() - start}")
start = time.time()
print(f"Dynamic result: {fibonacci_dynamic(n)}")
print(f"Dynamic time: {time.time() - start}")
By focusing on Python, a learner can quickly understand the performance difference between recursive and dynamic programming approaches to the Fibonacci sequence. This understanding can then be applied to other problems and languages as needed.
4. More Effective Interview Preparation
For those aspiring to work at major tech companies, technical interviews often focus more on problem-solving abilities and algorithmic thinking than on knowledge of multiple languages. In fact, many companies allow candidates to choose their preferred language for coding interviews. By mastering one language, candidates can:
- Write code more fluently during high-pressure interview situations
- Focus on communicating their thought process clearly
- Avoid syntax errors and language confusion that can waste valuable interview time
Here’s an example of a typical interview question and how focusing on one language (Python in this case) allows for a clearer demonstration of problem-solving skills:
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def reverse_linked_list(head):
prev = None
current = head
while current:
next_temp = current.next
current.next = prev
prev = current
current = next_temp
return prev
# Helper function to create a linked list from a list
def create_linked_list(values):
dummy = ListNode(0)
current = dummy
for val in values:
current.next = ListNode(val)
current = current.next
return dummy.next
# Helper function to convert linked list to list for printing
def linked_list_to_list(head):
result = []
current = head
while current:
result.append(current.val)
current = current.next
return result
# Test the function
original_list = create_linked_list([1, 2, 3, 4, 5])
reversed_list = reverse_linked_list(original_list)
print(f"Original list: {linked_list_to_list(original_list)}")
print(f"Reversed list: {linked_list_to_list(reversed_list)}")
By being proficient in Python, a candidate can focus on explaining the logic of reversing a linked list, discussing time and space complexity, and potentially optimizing the solution, rather than worrying about syntax or language-specific features.
5. Easier Transition to New Languages Later
Counterintuitively, focusing on one language initially can make it easier to learn additional languages later. This is because:
- Core programming concepts transfer between languages
- Strong fundamentals make it easier to identify similarities and differences
- Pattern recognition skills developed in one language apply to others
For example, once you understand object-oriented programming in Python, transitioning to Java becomes much easier:
# Python
class Car:
def __init__(self, make, model):
self.make = make
self.model = model
def display_info(self):
print(f"{self.make} {self.model}")
my_car = Car("Toyota", "Corolla")
my_car.display_info()
The equivalent in Java would be:
// Java
public class Car {
private String make;
private String model;
public Car(String make, String model) {
this.make = make;
this.model = model;
}
public void displayInfo() {
System.out.println(this.make + " " + this.model);
}
public static void main(String[] args) {
Car myCar = new Car("Toyota", "Corolla");
myCar.displayInfo();
}
}
The underlying concepts of classes, constructors, and methods remain the same, with the main differences being syntax and language-specific conventions.
When Multiple Languages Become Beneficial
While focusing on a single language is advantageous during the learning phase and when preparing for interviews, there are situations where knowledge of multiple languages becomes valuable:
1. Advanced Career Stages
As programmers advance in their careers, exposure to multiple languages can broaden their perspective and make them more versatile team members. This is particularly true for senior roles where architectural decisions may involve choosing the most appropriate language for a project.
2. Specialized Domains
Some domains require knowledge of specific languages. For example:
- iOS development primarily uses Swift
- Data science often involves Python and R
- Enterprise software development might require Java or C#
3. Full-Stack Development
Full-stack developers often need to work with multiple languages to handle both front-end and back-end development. For instance:
// JavaScript (Front-end)
document.getElementById("myButton").addEventListener("click", function() {
fetch("/api/data")
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error("Error:", error));
});
# Python (Back-end with Flask)
from flask import Flask, jsonify
app = Flask(__name__)
@app.route("/api/data")
def get_data():
data = {"message": "Hello from the server!"}
return jsonify(data)
if __name__ == "__main__":
app.run(debug=True)
In this scenario, understanding both JavaScript for client-side operations and Python for server-side logic becomes necessary.
Strategies for Effective Single-Language Learning
To maximize the benefits of focusing on a single language, consider the following strategies:
1. Choose a Versatile Language
Select a language that is widely used and has broad applications. Python, for example, is an excellent choice due to its readability, versatility, and strong presence in various domains including web development, data science, and artificial intelligence.
2. Focus on Problem-Solving
Use platforms like AlgoCademy to tackle a wide range of programming problems. This approach helps develop strong algorithmic thinking skills that are transferable across languages.
3. Understand Core Concepts
Pay special attention to fundamental programming concepts such as data structures, algorithms, object-oriented programming, and functional programming. These concepts are largely language-agnostic and form the foundation of computer science.
4. Practice Regularly
Consistent practice is key to mastery. Set aside dedicated time for coding exercises, preferably daily. This helps reinforce learning and builds muscle memory for syntax and problem-solving patterns.
5. Build Projects
Apply your knowledge to real-world projects. This helps consolidate learning and provides practical experience that is valuable for both interviews and actual development work.
6. Engage with the Community
Participate in online forums, contribute to open-source projects, or join local coding meetups. This exposure can provide new perspectives and help you learn best practices within your chosen language’s ecosystem.
Conclusion
While the ability to work with multiple programming languages is undoubtedly valuable in certain contexts, focusing on a single language during the learning phase and when preparing for technical interviews can be significantly advantageous. This approach allows for deeper understanding, faster progress in developing crucial problem-solving skills, and more effective interview preparation.
Platforms like AlgoCademy recognize the importance of strong foundational skills and algorithmic thinking. By providing interactive coding tutorials, resources, and AI-powered assistance, they support learners in developing these critical abilities within the context of a single, well-chosen language.
Remember, the goal of learning to code, especially when aiming for positions at major tech companies, is not to become a polyglot programmer from the outset. Instead, it’s about developing strong problem-solving skills, understanding core computer science concepts, and being able to implement efficient solutions. These skills, once mastered in one language, can then be more easily transferred to other languages as your career progresses and your needs evolve.
So, if you’re at the beginning of your coding journey or preparing for technical interviews, consider the benefits of committing to a single language. Dive deep, practice extensively, and focus on honing your problem-solving abilities. This focused approach may well be your key to success in the competitive world of programming and tech interviews.