Why Knowing 20 Programming Languages Isn’t Helping You Ship Better Software
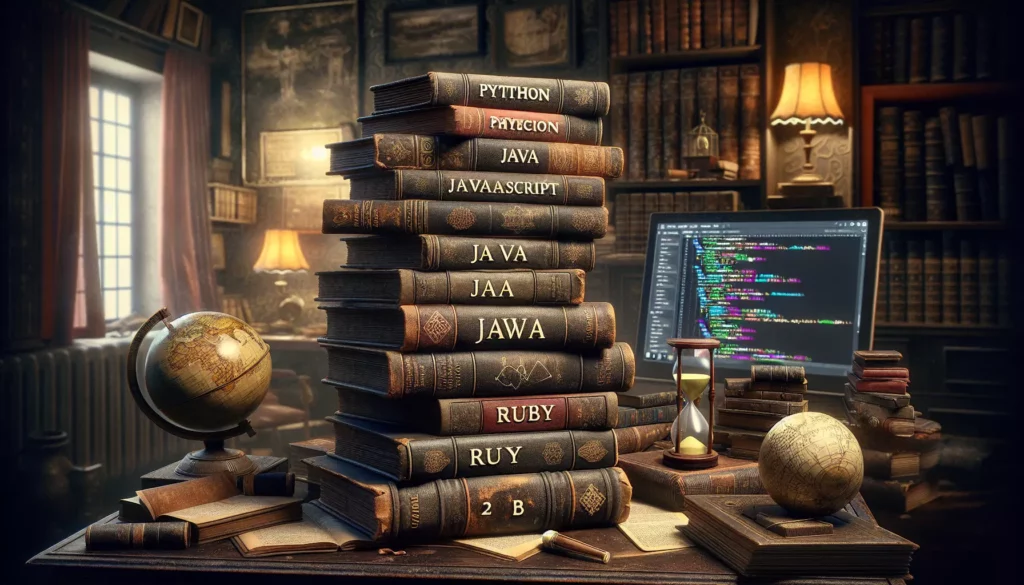
In the world of software development, there’s a peculiar badge of honor that many developers wear proudly: the number of programming languages they know. LinkedIn profiles showcase long lists of languages, frameworks, and tools. Resumes boast proficiency in everything from COBOL to Rust. But here’s a controversial truth: knowing 20 programming languages probably isn’t making you a better developer or helping you ship better software.
This might sound counterintuitive. After all, doesn’t more knowledge equal better outcomes? Not necessarily, and today we’re going to explore why language accumulation can become a distraction from what truly matters in software development.
The Polyglot Programmer Paradox
Let’s start with a scenario many of us have encountered: the interview candidate who claims proficiency in a dozen languages but struggles to implement a basic algorithm in any of them. Or perhaps the senior developer who constantly suggests rewriting parts of the codebase in the latest trendy language, without considering the maintenance burden this creates.
This is what I call the “polyglot programmer paradox” — the phenomenon where expanding your language repertoire begins to yield diminishing or even negative returns on your ability to deliver valuable software.
Don’t get me wrong — having experience with multiple programming paradigms and languages can be valuable. It broadens your perspective and helps you approach problems from different angles. The issue arises when collecting languages becomes an end in itself rather than a means to solve real problems.
Depth vs. Breadth: The Real Trade-off
Every hour you spend learning the syntax of a new language is an hour not spent on:
- Deepening your understanding of software architecture
- Mastering testing strategies
- Learning about system design
- Improving your debugging skills
- Understanding user needs
- Collaborating more effectively with your team
These skills are language-agnostic and ultimately more important for shipping quality software than knowing how to write a “Hello World” program in 20 different languages.
Consider two developers:
- Developer A knows JavaScript, Python, Ruby, Go, Rust, C++, Haskell, Elixir, Kotlin, Swift, PHP, C#, Java, Scala, TypeScript, Clojure, Perl, R, Julia, and Dart.
- Developer B has deep expertise in JavaScript and Python, with extensive knowledge of testing methodologies, performance optimization, security best practices, and system design patterns.
Who would you trust to build a critical application for your business? In most cases, Developer B will deliver more reliable, maintainable, and effective software despite knowing fewer languages.
The Cognitive Load of Language Switching
One underappreciated aspect of knowing many languages is the cognitive burden it places on you. Each language has its own:
- Syntax and grammar
- Idioms and best practices
- Ecosystem of libraries and tools
- Debugging workflows
- Performance characteristics
When you’re actively working with multiple languages, you’re constantly context-switching between these different mental models. This switching comes with a cognitive cost that can reduce your effectiveness.
Research in psychology has consistently shown that multitasking and context-switching reduce productivity. The same applies to programming languages. When you’re juggling mental models for multiple languages, you’re more likely to:
- Mix up syntax between languages
- Forget language-specific idioms
- Waste time looking up basic operations
- Implement non-idiomatic solutions
This is especially true for languages you use only occasionally. That Scala knowledge isn’t very useful if you only write Scala code once a year and spend half your time relearning the basics.
The Most Valuable Programming Skills Aren’t Language-Specific
The skills that truly differentiate exceptional developers from average ones transcend any specific language:
1. Problem Decomposition
The ability to break down complex problems into manageable, solvable components is fundamental to software development. This skill works the same way whether you’re coding in Python or Rust.
2. Algorithmic Thinking
Understanding time and space complexity, choosing appropriate data structures, and optimizing algorithms are universal skills that apply across all languages.
Consider this example of finding duplicates in an array. The approach is fundamentally the same regardless of language:
// In JavaScript
function findDuplicates(array) {
const seen = new Set();
const duplicates = [];
for (const item of array) {
if (seen.has(item)) {
duplicates.push(item);
} else {
seen.add(item);
}
}
return duplicates;
}
# In Python
def find_duplicates(array):
seen = set()
duplicates = []
for item in array:
if item in seen:
duplicates.append(item)
else:
seen.add(item)
return duplicates
The core algorithm remains identical despite the syntactic differences. What matters is understanding the approach, not the specific syntax.
3. System Design
The ability to design scalable, maintainable systems is language-agnostic. Concepts like load balancing, caching strategies, database design, and microservice architecture work similarly regardless of implementation language.
4. Debugging and Troubleshooting
Skilled debugging is about logical thinking, hypothesis testing, and systematic elimination of possibilities — skills that transfer across languages, even if the specific tools differ.
5. Testing Strategies
Understanding what, when, and how to test your code effectively is a universal skill. The principles of unit testing, integration testing, and end-to-end testing remain consistent across languages.
When Language Diversity Actually Matters
To be fair, there are legitimate reasons to learn multiple programming languages:
Understanding Different Programming Paradigms
Learning languages from different paradigms can genuinely expand your thinking:
- Object-oriented programming (Java, C#, Ruby)
- Functional programming (Haskell, Clojure, Elm)
- Procedural programming (C, Go)
- Logic programming (Prolog)
Exposure to different paradigms can help you recognize when a particular approach might be better suited to the problem at hand. For instance, functional programming techniques can be particularly effective for data transformation pipelines, while object-oriented approaches might better model complex domain relationships.
However, you don’t need to master 5 functional languages to understand functional programming. Learning one language deeply from each major paradigm will give you most of the benefit.
Specialized Problem Domains
Some languages are specifically designed for certain domains:
- R for statistical computing
- CUDA for GPU programming
- SQL for database queries
- Verilog for hardware description
If your work spans multiple specialized domains, then yes, you might genuinely need multiple languages in your toolkit.
Legacy System Maintenance
If you’re working in an organization with systems built over decades, you might need to maintain code written in older languages. In these cases, having knowledge of multiple languages is a practical necessity rather than a choice.
The T-Shaped Developer: A Better Approach
Rather than spreading yourself thin across many languages, consider the concept of becoming a “T-shaped developer”:
- The vertical bar of the T represents deep expertise in one or two languages and their ecosystems
- The horizontal bar represents broader knowledge across the software development landscape
This approach gives you both the depth needed to be truly productive and the breadth to adapt to different situations.
Developing Your Primary Language Expertise
For your primary language(s), aim for mastery beyond just syntax:
- Ecosystem knowledge: Understand the standard libraries, popular frameworks, and tools
- Performance characteristics: Know how to profile and optimize code in this language
- Idiomatic code: Understand what “good” code looks like in this language
- Language internals: Have some understanding of how the language works under the hood
For example, if JavaScript is your primary language, you should aim to understand:
- The event loop and asynchronous programming model
- Closure scoping rules and prototypal inheritance
- The module system and bundling tools
- Common performance pitfalls and optimization techniques
- Testing frameworks and methodologies
This depth allows you to write not just functional code, but elegant, efficient, and maintainable code.
Broadening Your Horizontal Knowledge
For the horizontal part of your T, focus on concepts rather than specific languages:
- Software architecture patterns
- Database design principles
- Network protocols and API design
- Security best practices
- Development methodologies
- User experience considerations
This broader knowledge helps you make better system-level decisions regardless of your implementation language.
The Real Cost of Language Proliferation in Teams
At the team and organizational level, language proliferation comes with additional costs that are often overlooked:
Increased Maintenance Burden
Every language in your stack multiplies your maintenance burden:
- Different dependency management systems to maintain
- Multiple build pipelines to configure and debug
- More security vulnerabilities to track across different ecosystems
- Increased complexity in deployment processes
Knowledge Silos and Bus Factor
When different parts of your system are written in different languages, you risk creating knowledge silos where only one or two people can effectively maintain certain components. This increases your “bus factor” — the number of team members who would need to be hit by a bus (or leave the company) before a project is in serious trouble.
Hiring and Onboarding Challenges
Each additional language in your stack narrows your hiring pool and increases onboarding time for new team members. A developer who needs to learn multiple languages and their ecosystems will take longer to become productive than one who can focus on a single language.
Inconsistent Practices
With multiple languages come multiple sets of best practices, coding standards, and quality controls. This can lead to inconsistent code quality and practices across your codebase.
How to Actually Improve Your Software Delivery
If collecting programming languages isn’t the path to better software, what is? Here are more effective ways to improve your ability to ship quality software:
1. Master Your Tools
Instead of learning new languages, invest in mastering the tools you already use:
- IDE/Editor: Learn advanced features, keyboard shortcuts, and debugging capabilities
- Version Control: Understand advanced git workflows, not just basic commits and pushes
- Testing Frameworks: Become proficient with mocking, test coverage, and test-driven development
- Profiling Tools: Learn how to identify and fix performance bottlenecks
These skills directly translate to productivity gains in your daily work.
2. Study Software Architecture
Understanding how to structure software for maintainability, scalability, and reliability will improve every system you build, regardless of language:
- Learn common architectural patterns like microservices, event-driven architecture, and hexagonal architecture
- Study how successful systems handle concerns like caching, load balancing, and fault tolerance
- Understand trade-offs between different architectural choices
3. Improve Your Requirements Analysis Skills
Many software projects fail not because of coding issues but because they solve the wrong problem. Getting better at understanding user needs and translating them into technical requirements will help you build more valuable software.
4. Develop Your Testing Strategy
Quality software requires effective testing. Develop a comprehensive understanding of:
- Different types of tests (unit, integration, end-to-end, performance, security)
- When to apply each type of test
- How to write testable code
- How to measure test effectiveness
5. Learn About DevOps and Continuous Delivery
The ability to reliably and frequently deliver software to production is a critical skill:
- Understand CI/CD pipelines and automation
- Learn about infrastructure as code
- Study monitoring and observability practices
- Develop strategies for zero-downtime deployments
6. Focus on the User Experience
Great software solves real problems for real users. Develop empathy for your users and learn how to:
- Gather and incorporate user feedback
- Design intuitive interfaces
- Optimize performance for user-facing applications
- Build accessible software
When You Should Learn a New Language
Despite the arguments against language accumulation, there are legitimate reasons to add a new language to your toolkit:
When It Enables a New Paradigm
If you’ve only worked with imperative, object-oriented languages like Java or C#, learning a functional language like Clojure or Haskell can genuinely expand your thinking and introduce you to new problem-solving approaches.
When It’s Required for a Specific Domain
If you’re moving into data science, learning R or Julia makes sense. If you’re getting into embedded systems, C or Rust would be appropriate choices. The key is that the language serves a specific purpose rather than being collected for its own sake.
When Your Current Language Is a Limitation
Sometimes your current language genuinely becomes a limiting factor for what you’re trying to achieve. For example, if you need bare-metal performance for a specific component, moving from Python to Rust might be justified.
When It’s Strategic for Your Career
If you’re looking to move into a field or company where a specific language dominates, learning that language is a strategic career move. Just be honest with yourself about whether this is the real motivation.
How to Evaluate Whether You Need a New Language
Before investing time in learning a new programming language, ask yourself these questions:
- What specific problem will this language help me solve that I can’t solve with my current tools?
- Is this language genuinely better for this problem, or just different?
- Will I use this language frequently enough to maintain proficiency?
- What’s the opportunity cost of learning this language versus deepening my skills elsewhere?
- Does this language introduce concepts I haven’t encountered before?
If you can’t provide clear answers to these questions, you might be falling into the language collection trap.
A Better Approach to Learning
Instead of collecting languages, consider this more focused approach to expanding your programming knowledge:
1. Master One Language Deeply
Pick a versatile, widely-used language as your primary tool and learn it deeply. This could be JavaScript, Python, Java, C#, or similar languages with broad applicability and strong ecosystems.
Aim to understand:
- Language idioms and best practices
- Performance characteristics and optimization techniques
- The standard library and core frameworks
- Testing approaches specific to this language
- Debugging and profiling tools
2. Learn One Representative from Each Major Paradigm
Rather than learning multiple languages that are conceptually similar, learn one language from each major programming paradigm:
- An object-oriented language (Java, C#, Ruby)
- A functional language (Haskell, Clojure, F#)
- A systems/procedural language (C, Rust, Go)
- A dynamic scripting language (Python, JavaScript)
This gives you exposure to different ways of thinking without the overhead of maintaining proficiency in too many languages.
3. Focus on Problem Domains, Not Languages
Instead of saying “I want to learn Rust,” say “I want to learn about systems programming” or “I want to understand memory management without garbage collection.” This keeps your learning focused on concepts rather than syntax.
4. Build Real Projects
Theoretical knowledge only goes so far. To truly understand a language or concept, you need to build something real with it. Focus on completing projects rather than accumulating surface-level knowledge.
Case Study: The Polyglot Project That Failed
Let me share a story from my own experience that illustrates the dangers of language proliferation. A few years ago, I worked with a startup that had embraced the “use the best tool for each job” philosophy with enthusiasm.
Their stack included:
- Ruby on Rails for the main web application
- Node.js for real-time features
- Python for data processing
- Go for performance-critical microservices
- React with TypeScript for the frontend
- Kotlin for their Android app
- Swift for their iOS app
On paper, each choice made sense. Ruby on Rails for rapid development, Node.js for its event-driven architecture, Python for its data science libraries, and so on.
The reality was chaos. With a small team of 8 developers, no one had deep expertise in more than a couple of these languages. Bug fixes required context-switching between multiple languages and frameworks. The CI/CD pipeline was a complex mess trying to handle different build processes. Onboarding new developers took months instead of weeks.
Most critically, when they needed to scale the system to handle increased load, no one had enough depth of knowledge in any particular part to optimize effectively. They ended up rewriting critical components multiple times, trying different languages in hopes of finding a silver bullet, rather than deeply understanding and optimizing what they had.
Eventually, they simplified to a mostly Ruby/JavaScript stack, focusing on mastering those technologies rather than constantly adding new ones. Productivity improved, bugs decreased, and they were finally able to focus on delivering features instead of fighting their own infrastructure.
Conclusion: Quality Over Quantity
The ability to ship high-quality software consistently depends far more on your understanding of software design principles, testing strategies, and problem-solving skills than on the number of languages you know.
Rather than collecting languages like Pokémon cards, focus on:
- Mastering a small set of tools deeply
- Understanding fundamental concepts that transcend specific languages
- Developing your skills in requirements analysis, architecture, and testing
- Building real systems that solve real problems
This focused approach will make you a more effective developer and help you ship better software, even if your language count remains in single digits.
Remember: the best developers aren’t those who know the most languages; they’re those who can consistently deliver valuable, maintainable software that solves real problems. Focus on developing those skills, and you’ll be well on your way to becoming a more effective software developer.