Why Just Following Tutorials Won’t Make You a Programmer
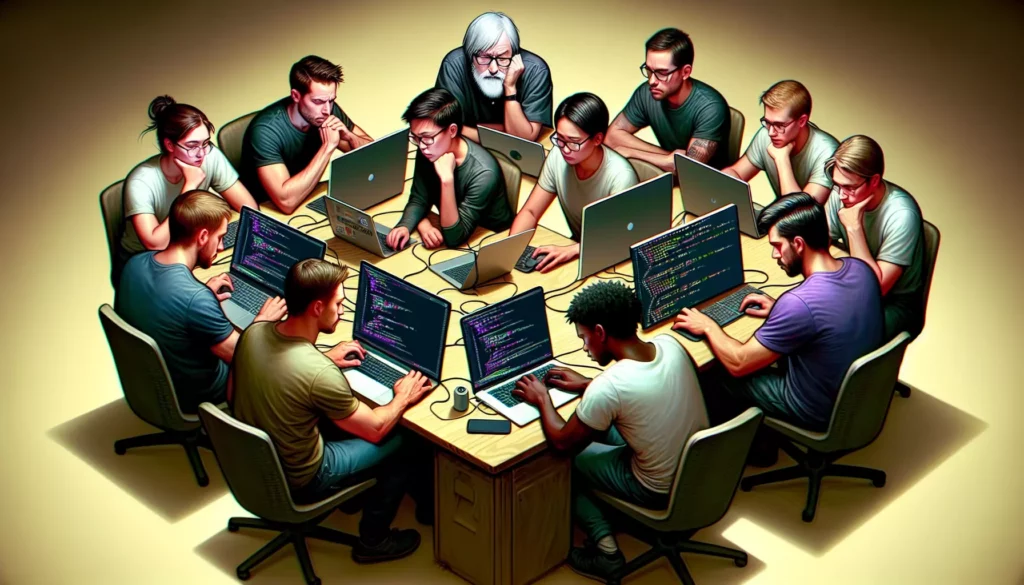
In the vast landscape of coding education, tutorials have become a go-to resource for aspiring programmers. They offer step-by-step guidance, instant gratification, and the illusion of rapid progress. However, as many experienced developers will tell you, simply following tutorials is not enough to transform you into a proficient programmer. This article delves into why relying solely on tutorials can be limiting and what steps you need to take to truly develop your programming skills.
The Tutorial Trap: Understanding the Limitations
Tutorials serve an important purpose in the learning process. They introduce concepts, demonstrate practical applications, and provide a structured approach to learning new technologies or programming languages. However, they come with inherent limitations that can hinder your growth as a programmer if you don’t move beyond them.
1. Passive Learning vs. Active Problem-Solving
One of the primary issues with tutorials is that they often encourage passive learning. You follow along, copy code, and see immediate results. While this can be satisfying, it doesn’t engage the critical thinking and problem-solving skills that are crucial for real-world programming.
In contrast, actual programming involves:
- Analyzing problems
- Breaking down complex issues into smaller, manageable parts
- Designing solutions from scratch
- Debugging and troubleshooting when things don’t work as expected
These skills are rarely developed by simply following along with pre-written code and explanations.
2. Lack of Context and Depth
Tutorials often focus on specific tasks or features without providing the broader context of how these elements fit into larger systems or projects. This can lead to a fragmented understanding of programming concepts and difficulty in applying knowledge to different scenarios.
3. The Illusion of Understanding
Completing a tutorial can give you a false sense of mastery. You might feel confident after successfully following the steps, but this doesn’t necessarily translate to the ability to apply the concepts independently or explain them to others.
4. Limited Exposure to Real-World Challenges
Tutorials typically present idealized scenarios with clean, error-free code. In reality, programming involves dealing with legacy code, integrating disparate systems, and solving problems that don’t have clear-cut solutions.
Beyond Tutorials: Building Real Programming Skills
To truly become a programmer, you need to move beyond the comfort zone of tutorials and engage in activities that challenge you to think, create, and problem-solve independently. Here are some strategies to help you make this transition:
1. Build Projects from Scratch
Nothing beats the learning experience of building your own projects from the ground up. This process forces you to:
- Plan and design your application
- Make decisions about architecture and tools
- Implement features without step-by-step guidance
- Debug your own code
- Learn how different components of an application work together
Start with small projects and gradually increase their complexity as you gain confidence and skills.
2. Participate in Coding Challenges
Platforms like LeetCode, HackerRank, and CodeWars offer coding challenges that test your problem-solving abilities. These exercises:
- Improve your algorithmic thinking
- Expose you to a variety of problem types
- Help you practice coding under time constraints
- Allow you to compare your solutions with others
Regular practice on these platforms can significantly enhance your coding skills and prepare you for technical interviews.
3. Contribute to Open Source Projects
Open source contributions provide invaluable experience in working with real-world codebases. By participating in open source projects, you:
- Learn to read and understand code written by others
- Gain experience in version control and collaboration
- Interact with experienced developers
- Work on solving actual issues and implementing new features
Start by looking for beginner-friendly issues on GitHub and gradually take on more complex tasks as you gain confidence.
4. Dive Deep into Computer Science Fundamentals
While not all programming roles require a deep understanding of computer science, having a solid grasp of fundamental concepts can make you a more effective programmer. Study areas such as:
- Data structures and algorithms
- Operating systems
- Database systems
- Computer networks
Resources like online courses, textbooks, and platforms like AlgoCademy can help you build this foundational knowledge.
5. Practice Explaining Concepts to Others
Teaching or explaining programming concepts to others is an excellent way to solidify your own understanding. You can:
- Write blog posts about topics you’ve learned
- Create video tutorials
- Mentor beginners in coding communities
- Participate in coding forums and help answer questions
This process of articulating your knowledge helps identify gaps in your understanding and reinforces your learning.
6. Read and Analyze Other People’s Code
Reading code is a skill in itself and one that’s crucial for professional developers. Practice by:
- Examining open-source projects on GitHub
- Studying well-written libraries and frameworks
- Participating in code reviews
This exposure to different coding styles and approaches will broaden your perspective and improve your own coding practices.
The Role of Tutorials in Your Learning Journey
While this article emphasizes moving beyond tutorials, it’s important to note that they still have a place in your learning journey. Tutorials can be valuable when:
- You’re getting started with a new language or technology
- You need a quick introduction to a specific concept or tool
- You’re looking for best practices or design patterns
The key is to use tutorials as a starting point, not as your primary or only method of learning. After completing a tutorial, challenge yourself to apply the concepts in a different context or expand upon what you’ve learned.
Developing a Growth Mindset
Becoming a proficient programmer requires more than just technical skills; it demands a growth mindset. This involves:
- Embracing challenges and seeing them as opportunities to learn
- Persisting in the face of setbacks
- Viewing effort as a path to mastery
- Learning from criticism and the success of others
Cultivating this mindset will help you push through the frustrations and challenges that come with learning to program.
The Importance of Consistent Practice
Programming is a skill that requires consistent practice to maintain and improve. Even experienced developers continue to learn and refine their skills. Establish a routine that includes:
- Daily coding practice, even if it’s just for a short period
- Regular project work
- Continuous learning through books, articles, and online resources
- Participation in coding communities and events
Remember, becoming a programmer is a journey, not a destination. It’s about continuous improvement and adaptation to new technologies and practices.
Leveraging AI-Powered Tools for Learning
As the field of programming education evolves, AI-powered tools are becoming increasingly valuable for learners. Platforms like AlgoCademy offer features that can complement your learning beyond tutorials:
- Personalized learning paths based on your skill level and goals
- Interactive coding environments that provide immediate feedback
- AI-assisted problem-solving that guides you through complex concepts
- Adaptive challenges that adjust to your growing skills
While these tools can be powerful aids in your learning journey, remember that they should supplement, not replace, the hands-on experience of building projects and solving real-world problems.
The Path to Becoming a Programmer
Becoming a programmer is about more than just acquiring technical knowledge. It involves developing a problem-solving mindset, learning to think algorithmically, and building the resilience to tackle complex challenges. Here’s a roadmap to guide your journey:
- Start with the basics: Learn the fundamentals of programming concepts and a first language.
- Build small projects: Apply your knowledge to create simple applications.
- Learn data structures and algorithms: These form the backbone of efficient programming.
- Expand your language repertoire: Learn additional programming languages to broaden your perspective.
- Dive into software design and architecture: Understand how to structure larger applications.
- Contribute to open source: Gain experience working on real-world projects.
- Specialize: Focus on areas that interest you, such as web development, mobile apps, or machine learning.
- Never stop learning: Keep up with new technologies and best practices.
Practical Examples: Moving Beyond Tutorials
To illustrate the difference between following tutorials and real programming, let’s look at a practical example. Imagine you’ve completed a tutorial on building a simple to-do list application. Here’s how you might extend this knowledge to develop real programming skills:
Tutorial Project: Basic To-Do List
A typical tutorial might guide you through creating a basic to-do list with features like:
- Adding items to the list
- Marking items as complete
- Deleting items from the list
The tutorial code might look something like this:
<!-- HTML -->
<input id="newItem" type="text">
<button onclick="addItem()">Add Item</button>
<ul id="todoList"></ul>
<!-- JavaScript -->
<script>
function addItem() {
var input = document.getElementById("newItem");
var li = document.createElement("li");
li.appendChild(document.createTextNode(input.value));
document.getElementById("todoList").appendChild(li);
input.value = "";
}
</script>
Moving Beyond the Tutorial: Enhanced To-Do Application
To truly develop your skills, you could extend this project in several ways:
- Add persistent storage: Use localStorage or a backend database to save items.
- Implement user authentication: Allow multiple users to have their own lists.
- Add categories and priority levels: Enhance the organization of tasks.
- Create a RESTful API: Separate the frontend and backend for better architecture.
- Implement drag-and-drop reordering: Improve the user experience.
Here’s a more advanced code snippet that demonstrates some of these enhancements:
// Enhanced JavaScript with localStorage and categories
class TodoApp {
constructor() {
this.todos = JSON.parse(localStorage.getItem('todos')) || [];
this.categories = ['Personal', 'Work', 'Shopping'];
this.renderTodos();
this.setupEventListeners();
}
addTodo(text, category, priority) {
const todo = {
id: Date.now(),
text,
category,
priority,
completed: false
};
this.todos.push(todo);
this.saveTodos();
this.renderTodos();
}
toggleTodo(id) {
const todo = this.todos.find(t => t.id === id);
todo.completed = !todo.completed;
this.saveTodos();
this.renderTodos();
}
deleteTodo(id) {
this.todos = this.todos.filter(t => t.id !== id);
this.saveTodos();
this.renderTodos();
}
saveTodos() {
localStorage.setItem('todos', JSON.stringify(this.todos));
}
renderTodos() {
const todoList = document.getElementById('todoList');
todoList.innerHTML = '';
this.todos.forEach(todo => {
const li = document.createElement('li');
li.innerHTML = `
<span class="${todo.completed ? 'completed' : ''}">${todo.text}</span>
<span class="category">${todo.category}</span>
<span class="priority">Priority: ${todo.priority}</span>
<button onclick="app.toggleTodo(${todo.id})">Toggle</button>
<button onclick="app.deleteTodo(${todo.id})">Delete</button>
`;
todoList.appendChild(li);
});
}
setupEventListeners() {
document.getElementById('addTodo').addEventListener('submit', e => {
e.preventDefault();
const text = document.getElementById('newItem').value;
const category = document.getElementById('category').value;
const priority = document.getElementById('priority').value;
this.addTodo(text, category, priority);
document.getElementById('newItem').value = '';
});
}
}
const app = new TodoApp();
This enhanced version demonstrates several important programming concepts:
- Object-oriented programming with a class-based structure
- Data persistence using localStorage
- Event handling and DOM manipulation
- More complex data structures with multiple properties per todo item
By extending the basic tutorial concept, you’re forced to think about:
- Application structure and organization
- Data management and storage
- User interface design and interaction
- Code reusability and maintainability
This process of extending and enhancing a basic concept is where real learning and skill development occur. It challenges you to apply your knowledge in new ways, research additional concepts, and solve problems that weren’t explicitly covered in the original tutorial.
Conclusion: The Journey to Becoming a True Programmer
While tutorials serve as valuable starting points in your programming journey, they are just the beginning. Becoming a proficient programmer requires a multifaceted approach that includes hands-on project work, problem-solving practice, deep dives into computer science concepts, and continuous learning.
Remember these key points as you progress in your programming journey:
- Use tutorials as a springboard, not a crutch
- Embrace challenges and learn from failures
- Build projects that solve real problems
- Engage with the programming community
- Develop a growth mindset and a passion for continuous learning
- Practice consistently and push your boundaries
By moving beyond tutorials and actively engaging in the process of creating, problem-solving, and learning, you’ll develop the skills and mindset necessary to become not just a coder, but a true programmer capable of tackling complex challenges and creating innovative solutions.
The path to becoming a programmer is challenging but rewarding. It’s a journey of continuous growth and discovery. Embrace the challenges, celebrate your progress, and remember that every experienced programmer was once a beginner. With persistence, practice, and passion, you can transform from a tutorial follower to a confident, skilled programmer ready to make your mark in the world of technology.