Why Iterating on Your Projects Will Make You a More Efficient Coder
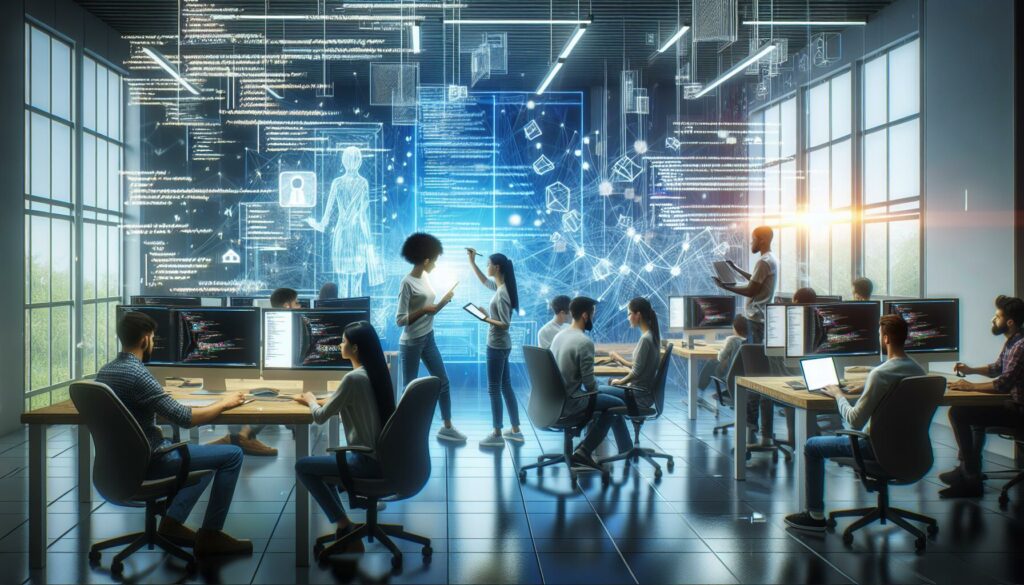
In the world of software development, the ability to iterate on your projects is not just a valuable skill—it’s a necessity. Whether you’re a beginner just starting your coding journey or an experienced developer preparing for technical interviews at top tech companies, understanding and applying the concept of iteration can significantly enhance your coding efficiency and overall project quality. In this comprehensive guide, we’ll explore why iterating on your projects is crucial and how it can transform you into a more proficient and effective coder.
What is Project Iteration?
Before diving into the benefits, let’s clarify what we mean by “iterating on your projects.” Project iteration refers to the process of continuously refining and improving your code or project through multiple cycles of development, testing, and feedback. It’s a cyclical approach that involves:
- Planning and designing
- Implementing features
- Testing and gathering feedback
- Analyzing results
- Making improvements
- Repeating the process
This iterative methodology stands in contrast to the traditional waterfall model, where development follows a linear, sequential path. Iteration allows for more flexibility, adaptability, and continuous improvement throughout the development lifecycle.
The Benefits of Iterating on Your Projects
1. Improved Code Quality
One of the most significant advantages of iterating on your projects is the substantial improvement in code quality. Each iteration provides an opportunity to:
- Refactor and optimize existing code
- Identify and fix bugs
- Implement better algorithms and data structures
- Enhance code readability and maintainability
As you revisit your code multiple times, you’ll naturally spot areas for improvement that you might have missed initially. This continuous refinement leads to cleaner, more efficient, and more robust code.
2. Enhanced Problem-Solving Skills
Iteration forces you to approach problems from different angles and consider alternative solutions. As you work through multiple cycles, you’ll:
- Develop a more comprehensive understanding of the problem domain
- Learn to break down complex issues into manageable components
- Improve your ability to analyze and debug code
- Cultivate creative thinking in finding innovative solutions
These enhanced problem-solving skills are invaluable, especially when preparing for technical interviews at major tech companies where algorithmic thinking is heavily emphasized.
3. Faster Learning and Skill Development
Iterating on projects accelerates your learning process and skill development. Each iteration cycle provides:
- Hands-on experience with different coding techniques
- Exposure to various programming concepts and patterns
- Opportunities to learn from mistakes and implement best practices
- A deeper understanding of the technologies and tools you’re using
This rapid skill acquisition is particularly beneficial for those using platforms like AlgoCademy, where the focus is on progressing from beginner-level coding to more advanced concepts.
4. Increased Adaptability
In the ever-evolving world of technology, adaptability is a crucial skill. Iterating on projects helps you:
- Become more comfortable with change and uncertainty
- Learn to pivot quickly when faced with new requirements or constraints
- Develop a growth mindset that embraces continuous improvement
- Stay up-to-date with emerging technologies and industry trends
This adaptability is essential for success in technical interviews and real-world development scenarios, where requirements often change and new challenges arise frequently.
5. Better Time Management and Prioritization
Iteration teaches you to manage your time more effectively and prioritize tasks. Through the process, you’ll learn to:
- Break down large projects into smaller, manageable tasks
- Estimate time and effort more accurately
- Focus on the most critical features first (MVP approach)
- Balance between perfecting existing code and adding new functionality
These time management skills are crucial for efficient coding and are often tested in technical interviews through time-constrained coding challenges.
Implementing Iteration in Your Coding Projects
Now that we’ve explored the benefits, let’s discuss how you can effectively implement iteration in your coding projects:
1. Start with a Minimum Viable Product (MVP)
Begin by implementing the core functionality of your project. This MVP approach allows you to:
- Get a working version quickly
- Gather early feedback
- Identify potential issues or improvements early in the development process
For example, if you’re building a to-do list application, your MVP might include basic functionality for adding, viewing, and deleting tasks.
2. Set Clear Goals for Each Iteration
Before starting each iteration cycle, define specific goals and objectives. This might include:
- Adding new features
- Improving performance
- Enhancing user interface
- Fixing known bugs
Having clear goals helps you stay focused and measure progress effectively.
3. Implement Automated Testing
Automated tests are crucial for efficient iteration. They allow you to:
- Quickly identify regressions or unintended side effects
- Refactor with confidence
- Maintain code quality throughout multiple iterations
Here’s a simple example of a unit test in Python using the pytest framework:
def add_numbers(a, b):
return a + b
def test_add_numbers():
assert add_numbers(2, 3) == 5
assert add_numbers(-1, 1) == 0
assert add_numbers(0, 0) == 0
4. Use Version Control
Version control systems like Git are essential for effective iteration. They allow you to:
- Track changes over time
- Experiment with new features without affecting the main codebase
- Collaborate with others more easily
- Revert to previous versions if needed
Here’s a basic Git workflow for iterating on a project:
git checkout -b new-feature
# Make changes and commit them
git add .
git commit -m "Implement new feature"
git push origin new-feature
# Create a pull request for review
# Merge changes into main branch after review
5. Gather and Incorporate Feedback
Feedback is a crucial component of effective iteration. Seek input from:
- Peers or mentors
- Users or stakeholders
- Automated code analysis tools
Use this feedback to guide your improvements in subsequent iterations.
6. Reflect and Learn from Each Iteration
After each iteration cycle, take time to reflect on what worked well and what could be improved. This might involve:
- Conducting a personal or team retrospective
- Documenting lessons learned
- Updating your development processes based on insights gained
Real-World Example: Iterating on a Sorting Algorithm
Let’s look at a practical example of how iteration can improve your coding skills and efficiency. Suppose you’re working on implementing a sorting algorithm as part of your preparation for technical interviews. Here’s how you might iterate on a bubble sort implementation:
Iteration 1: Basic Implementation
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n - i - 1):
if arr[j] > arr[j + 1]:
arr[j], arr[j + 1] = arr[j + 1], arr[j]
return arr
# Test the function
print(bubble_sort([64, 34, 25, 12, 22, 11, 90]))
This initial implementation works but is inefficient for large arrays.
Iteration 2: Adding Early Termination
def bubble_sort(arr):
n = len(arr)
for i in range(n):
swapped = False
for j in range(0, n - i - 1):
if arr[j] > arr[j + 1]:
arr[j], arr[j + 1] = arr[j + 1], arr[j]
swapped = True
if not swapped:
break
return arr
# Test the function
print(bubble_sort([64, 34, 25, 12, 22, 11, 90]))
This iteration adds an optimization to terminate early if no swaps are made in a pass.
Iteration 3: Implementing as a Generator
def bubble_sort_generator(arr):
n = len(arr)
for i in range(n):
swapped = False
for j in range(0, n - i - 1):
if arr[j] > arr[j + 1]:
arr[j], arr[j + 1] = arr[j + 1], arr[j]
swapped = True
yield arr # Yield the current state of the array
if not swapped:
break
# Test the function
arr = [64, 34, 25, 12, 22, 11, 90]
for step in bubble_sort_generator(arr):
print(step)
This iteration transforms the function into a generator, allowing us to visualize the sorting process step by step.
Iteration 4: Adding Type Hints and Docstring
from typing import List, Generator
def bubble_sort_generator(arr: List[int]) -> Generator[List[int], None, None]:
"""
Perform bubble sort on the input array and yield each intermediate state.
Args:
arr (List[int]): The input array to be sorted.
Yields:
List[int]: The current state of the array after each pass.
"""
n = len(arr)
for i in range(n):
swapped = False
for j in range(0, n - i - 1):
if arr[j] > arr[j + 1]:
arr[j], arr[j + 1] = arr[j + 1], arr[j]
swapped = True
yield arr
if not swapped:
break
# Test the function
arr = [64, 34, 25, 12, 22, 11, 90]
for step in bubble_sort_generator(arr):
print(step)
This final iteration adds type hints and a docstring, improving code readability and maintainability.
Through these iterations, we’ve not only improved the functionality and efficiency of the sorting algorithm but also enhanced its usability and documentation. This process demonstrates how iteration can lead to more robust, efficient, and well-documented code.
Conclusion
Iterating on your projects is a powerful technique that can significantly enhance your coding skills and efficiency. By embracing an iterative approach, you’ll improve code quality, develop stronger problem-solving skills, accelerate your learning, increase adaptability, and enhance your time management abilities. These benefits are particularly valuable for those using platforms like AlgoCademy to progress from beginner-level coding to preparing for technical interviews at major tech companies.
Remember, iteration is not about achieving perfection in a single pass. It’s about continuous improvement, learning from each cycle, and gradually refining your skills and your code. As you apply these principles to your coding projects, you’ll find yourself becoming a more efficient, adaptable, and skilled programmer, well-prepared for the challenges of real-world development and technical interviews.
So, the next time you start a new coding project or revisit an old one, embrace the power of iteration. Set clear goals, implement in small increments, gather feedback, and continuously refine your work. With each iteration, you’ll not only improve your project but also take significant strides in your journey as a programmer.