Why is Recursion So Hard to Understand? A Comprehensive Guide
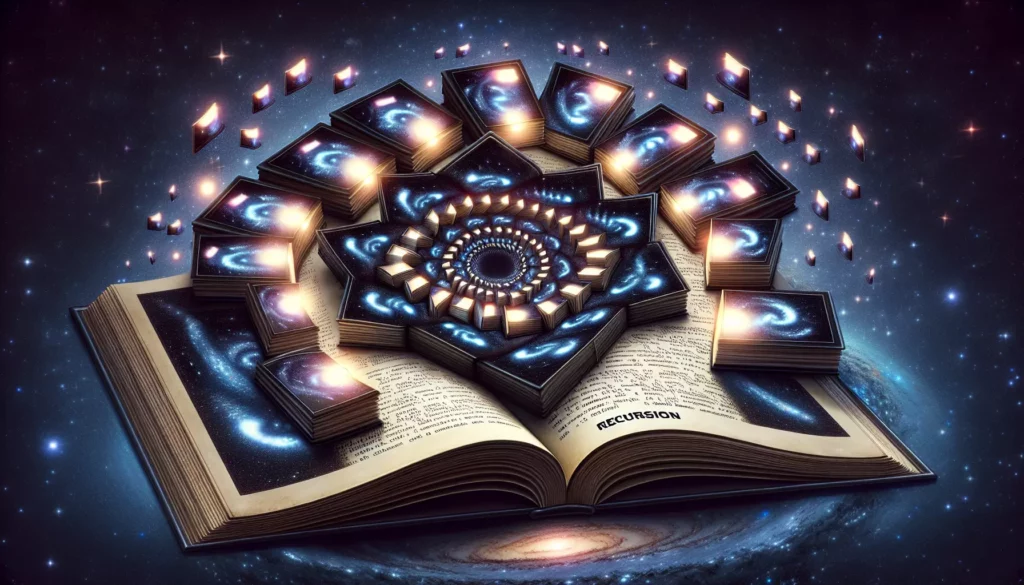
Recursion is a fundamental concept in computer science and programming that often leaves many learners scratching their heads. It’s a powerful technique used in various algorithms and problem-solving strategies, yet it remains one of the most challenging concepts for many to grasp fully. In this comprehensive guide, we’ll explore why recursion is difficult to understand, break down its core concepts, and provide strategies to help you master this essential programming skill.
Table of Contents
- What is Recursion?
- Why Recursion is Challenging
- Common Misconceptions About Recursion
- Benefits of Recursion
- Understanding Recursion: Step by Step
- Practical Examples of Recursion
- Tips for Mastering Recursion
- Recursion vs. Iteration
- Advanced Concepts in Recursion
- Conclusion
What is Recursion?
Before diving into why recursion is challenging, let’s establish a clear definition. Recursion is a programming technique where a function calls itself to solve a problem by breaking it down into smaller, more manageable subproblems. The function continues to call itself until it reaches a base case, which is a condition that stops the recursive calls and begins to unwind the stack of function calls.
A simple example of a recursive function is the calculation of factorial:
def factorial(n):
if n == 0 or n == 1:
return 1
else:
return n * factorial(n - 1)
In this example, the function calls itself with a smaller input (n – 1) until it reaches the base case (n == 0 or n == 1).
Why Recursion is Challenging
There are several reasons why recursion can be difficult to understand and master:
1. It’s Counter-Intuitive
Our minds are naturally inclined to think iteratively, step by step. Recursion requires a mental shift to think in terms of self-referential processes, which can feel unnatural at first.
2. Visualizing the Process is Difficult
Unlike loops, where you can easily trace the flow of execution, recursive calls create multiple layers of function calls that can be hard to visualize mentally.
3. Stack Overflow Concerns
Recursive functions can lead to stack overflow errors if not implemented correctly, adding an extra layer of complexity to consider when designing recursive solutions.
4. Base Case and Recursive Case Confusion
Identifying and implementing the correct base case and recursive case can be tricky, especially for complex problems.
5. Performance Considerations
Recursive solutions can sometimes be less efficient than their iterative counterparts, leading to confusion about when to use recursion appropriately.
Common Misconceptions About Recursion
Several misconceptions contribute to the difficulty in understanding recursion:
1. “Recursion Always Leads to Infinite Loops”
While it’s true that poorly implemented recursion can result in infinite loops, properly designed recursive functions with well-defined base cases will terminate.
2. “Recursion is Always Less Efficient Than Iteration”
In some cases, recursive solutions can be more elegant and even more efficient than iterative ones, especially for problems that have a naturally recursive structure (e.g., tree traversals).
3. “Recursion is Only Used in Academic Settings”
Recursion is widely used in real-world applications, particularly in areas like parsing, graph algorithms, and divide-and-conquer strategies.
4. “You Need to Understand the Entire Process to Use Recursion”
While understanding the entire process is beneficial, you can often implement recursive solutions by focusing on the current step and trusting that the recursive calls will handle the rest.
Benefits of Recursion
Despite its challenges, recursion offers several advantages:
1. Elegant Solutions
Recursive solutions can often express complex algorithms in a more concise and elegant manner compared to iterative approaches.
2. Natural Fit for Certain Problems
Some problems, like tree traversals or fractal generation, have a naturally recursive structure that makes recursive solutions more intuitive.
3. Divide and Conquer
Recursion is a key component in divide-and-conquer algorithms, which are efficient for solving large, complex problems.
4. Code Reusability
Recursive functions can often be more easily adapted to similar problems, promoting code reuse.
Understanding Recursion: Step by Step
To better understand recursion, let’s break it down into key components:
1. Base Case
The base case is the condition that stops the recursion. It’s crucial to define one or more base cases to prevent infinite recursion.
2. Recursive Case
This is where the function calls itself with a modified input, working towards the base case.
3. State
Each recursive call maintains its own state, including local variables and the point of execution.
4. Stack
The call stack keeps track of each function call, allowing the program to return to the correct point after each recursive call completes.
Let’s illustrate these concepts with a simple example of calculating the sum of numbers from 1 to n:
def sum_to_n(n):
if n == 1: # Base case
return 1
else: # Recursive case
return n + sum_to_n(n - 1)
Here’s how this function works:
- If n is 1 (base case), it returns 1.
- Otherwise, it returns n plus the result of calling sum_to_n with n-1.
- This process continues until n reaches 1.
- The results are then combined as the call stack unwinds.
Practical Examples of Recursion
To further illustrate the concept, let’s look at some practical examples of recursion:
1. Fibonacci Sequence
def fibonacci(n):
if n <= 1:
return n
else:
return fibonacci(n - 1) + fibonacci(n - 2)
2. Binary Tree Traversal
class TreeNode:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
def inorder_traversal(root):
if root:
inorder_traversal(root.left)
print(root.value)
inorder_traversal(root.right)
3. Quicksort Algorithm
def quicksort(arr):
if len(arr) <= 1:
return arr
else:
pivot = arr[len(arr) // 2]
left = [x for x in arr if x < pivot]
middle = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
return quicksort(left) + middle + quicksort(right)
Tips for Mastering Recursion
Here are some strategies to help you become more comfortable with recursion:
1. Start with Simple Problems
Begin with basic recursive problems like factorial or Fibonacci sequence calculations before moving on to more complex algorithms.
2. Visualize the Process
Use diagrams or a debugger to step through recursive calls and understand how the call stack works.
3. Focus on the Current Step
When designing a recursive solution, focus on solving the current step and trust that the recursive calls will handle the rest.
4. Identify Base Cases First
Always start by identifying and implementing the base case(s) before working on the recursive case.
5. Practice, Practice, Practice
Solve a variety of recursive problems to build your intuition and understanding.
6. Analyze Time and Space Complexity
Understand the performance implications of your recursive solutions to make informed decisions about when to use recursion.
Recursion vs. Iteration
While recursion and iteration can often solve the same problems, they have distinct characteristics:
Recursion:
- Often leads to more elegant and concise code
- Can be more intuitive for naturally recursive problems
- May have higher memory overhead due to the call stack
- Can lead to stack overflow for deeply nested calls
Iteration:
- Generally more efficient in terms of memory usage
- Often easier to understand for simple problems
- Less prone to stack overflow errors
- Can be more verbose for complex algorithms
Consider this comparison of recursive and iterative approaches to calculating factorial:
def recursive_factorial(n):
if n == 0 or n == 1:
return 1
else:
return n * recursive_factorial(n - 1)
def iterative_factorial(n):
result = 1
for i in range(1, n + 1):
result *= i
return result
Both solutions work, but the recursive version is more concise and arguably more elegant, while the iterative version may be more efficient for large inputs.
Advanced Concepts in Recursion
As you become more comfortable with basic recursion, you can explore more advanced concepts:
1. Tail Recursion
Tail recursion is a special form of recursion where the recursive call is the last operation in the function. Some compilers can optimize tail-recursive functions to use constant stack space.
def tail_recursive_factorial(n, accumulator=1):
if n == 0 or n == 1:
return accumulator
else:
return tail_recursive_factorial(n - 1, n * accumulator)
2. Memoization
Memoization is a technique used to optimize recursive functions by caching the results of expensive function calls and returning the cached result when the same inputs occur again.
def memoized_fibonacci(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
result = memoized_fibonacci(n - 1, memo) + memoized_fibonacci(n - 2, memo)
memo[n] = result
return result
3. Mutual Recursion
Mutual recursion occurs when two or more functions call each other recursively. This can be useful for solving certain types of problems.
def is_even(n):
if n == 0:
return True
else:
return is_odd(n - 1)
def is_odd(n):
if n == 0:
return False
else:
return is_even(n - 1)
4. Recursive Backtracking
Recursive backtracking is a technique used to solve problems by trying out different possibilities and backtracking when a solution is not found. It’s commonly used in puzzle-solving algorithms.
def solve_maze(maze, x, y, end_x, end_y, path=[]):
if x == end_x and y == end_y:
return path + [(x, y)]
if x < 0 or y < 0 or x >= len(maze) or y >= len(maze[0]) or maze[x][y] == 1:
return None
maze[x][y] = 1 # Mark as visited
for dx, dy in [(0, 1), (1, 0), (0, -1), (-1, 0)]:
solution = solve_maze(maze, x + dx, y + dy, end_x, end_y, path + [(x, y)])
if solution:
return solution
maze[x][y] = 0 # Unmark
return None
Conclusion
Recursion is undoubtedly a challenging concept to master, but it’s an essential tool in a programmer’s toolkit. Its difficulty stems from its counter-intuitive nature, the complexity of visualizing recursive processes, and the need to carefully manage base cases and recursive cases. However, with practice and the right approach, recursion can become a powerful and elegant technique for solving complex problems.
Remember that understanding recursion is a gradual process. Start with simple problems, visualize the process, and focus on mastering one concept at a time. As you gain experience, you’ll develop an intuition for when and how to apply recursive solutions effectively.
By embracing the challenge of recursion, you’re not just learning a programming technique; you’re developing a new way of thinking about problem-solving that will serve you well throughout your programming career. Keep practicing, stay curious, and don’t be afraid to dive deep into the recursive rabbit hole – you might be surprised at the elegant solutions you discover along the way.