Why Is Dynamic Programming So Difficult to Master?
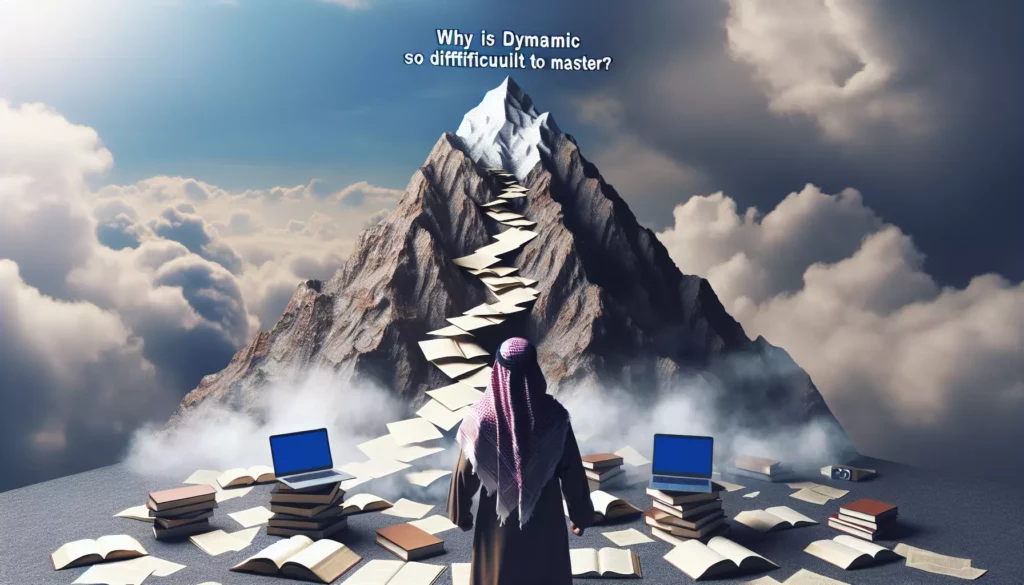
Dynamic Programming (DP) is a powerful algorithmic technique that has gained notoriety among programmers and computer science students for its complexity and difficulty to master. As an essential topic in coding interviews and a crucial skill for efficient problem-solving, understanding why DP is challenging can help learners develop better strategies to conquer this formidable subject. In this comprehensive guide, we’ll explore the reasons behind the difficulty of mastering dynamic programming and provide insights on how to overcome these challenges.
1. The Conceptual Leap
One of the primary reasons dynamic programming is difficult to master is the significant conceptual leap it requires from traditional problem-solving approaches. Unlike more straightforward algorithmic techniques, DP demands a shift in thinking that can be jarring for many learners.
1.1 Breaking the Problem-Solving Mold
Most programming problems can be solved using straightforward approaches like brute force, divide-and-conquer, or greedy algorithms. These methods often align with our intuitive problem-solving skills. However, DP requires us to approach problems from a different angle, which can be counterintuitive at first.
1.2 Thinking in Terms of Subproblems
Dynamic programming relies heavily on breaking down a problem into smaller subproblems and using the solutions to these subproblems to build the overall solution. This concept, known as “optimal substructure,” can be challenging to grasp and apply consistently across different problem types.
1.3 Recognizing Overlapping Subproblems
Another key aspect of DP is identifying overlapping subproblems. This requires a keen eye for patterns and the ability to recognize when computations are being repeated unnecessarily. Developing this skill takes time and practice, which contributes to the difficulty of mastering DP.
2. The Variety of Problem Types
Dynamic programming can be applied to a wide range of problem types, each with its own nuances and challenges. This variety can make it difficult for learners to develop a consistent approach to solving DP problems.
2.1 One-Dimensional vs. Multi-Dimensional DP
DP problems can involve one-dimensional arrays, two-dimensional matrices, or even higher-dimensional data structures. Each dimension adds a layer of complexity to the problem-solving process, requiring different approaches and mental models.
2.2 Top-Down vs. Bottom-Up Approaches
Dynamic programming solutions can be implemented using either a top-down (memoization) or bottom-up (tabulation) approach. Understanding when to use each method and how to translate between them adds another layer of complexity to mastering DP.
2.3 State Representation
One of the most challenging aspects of DP is determining the appropriate state representation for a given problem. This involves identifying the key variables that define the state of the problem at each step, which can be non-trivial for complex problems.
3. The Abstraction Challenge
Dynamic programming often requires a high level of abstraction, which can be difficult for many learners to grasp and apply effectively.
3.1 Formulating Recurrence Relations
A crucial step in solving DP problems is formulating the recurrence relation, which describes how the solution to a larger problem can be constructed from solutions to smaller subproblems. This process often involves abstract thinking and can be challenging to master.
3.2 Visualizing the Solution Space
Understanding and visualizing the solution space for DP problems can be difficult, especially for multi-dimensional problems. This visualization is often crucial for developing an effective solution strategy.
3.3 Translating Abstract Concepts to Code
Once a DP solution is conceptualized, translating that abstract solution into concrete code can be another significant hurdle. This translation process requires a deep understanding of both the problem and the programming language being used.
4. The Optimization Mindset
Dynamic programming is fundamentally about optimization, which requires a specific mindset that can be challenging to develop.
4.1 Thinking in Terms of Optimal Solutions
DP problems often ask for the optimal solution (e.g., minimum cost, maximum profit). Developing the intuition to recognize when a problem requires finding an optimal solution and how to approach it using DP can be difficult.
4.2 Balancing Time and Space Complexity
While DP solutions often provide significant time complexity improvements over naive approaches, they frequently come at the cost of increased space complexity. Balancing these trade-offs and understanding when to prioritize one over the other is a crucial skill in mastering DP.
4.3 Recognizing Optimization Opportunities
Even within DP solutions, there are often opportunities for further optimization. Recognizing these opportunities and implementing them effectively requires a deep understanding of both the problem and the DP technique.
5. The Learning Curve
The learning curve for dynamic programming is notoriously steep, which contributes significantly to its perceived difficulty.
5.1 Limited Exposure in Introductory Courses
Many introductory computer science courses only briefly touch on dynamic programming, if at all. This limited exposure means that many learners encounter DP for the first time in more advanced courses or during interview preparation, where the problems are already quite complex.
5.2 The Need for Extensive Practice
Mastering dynamic programming requires solving many problems across various types and difficulty levels. This extensive practice is necessary to develop the intuition and pattern recognition skills crucial for DP success.
5.3 The Psychological Factor
The reputation of DP as a difficult topic can create a psychological barrier for learners, leading to increased anxiety and decreased confidence when approaching DP problems. Overcoming this mental hurdle is an important part of the learning process.
6. Strategies for Mastering Dynamic Programming
While dynamic programming is undoubtedly challenging, there are several strategies that can help learners overcome these difficulties and master this powerful technique.
6.1 Start with the Basics
Begin by thoroughly understanding the fundamental concepts of DP, such as optimal substructure and overlapping subproblems. Practice identifying these elements in simple problems before moving on to more complex ones.
6.2 Develop a Systematic Approach
Create a step-by-step approach for tackling DP problems. This might include:
- Identifying the problem as a DP candidate
- Defining the state representation
- Formulating the recurrence relation
- Determining the base cases
- Deciding between top-down and bottom-up implementation
- Implementing the solution
- Optimizing the solution if necessary
6.3 Practice, Practice, Practice
Solve a wide variety of DP problems, starting from simple ones and gradually increasing in complexity. Platforms like AlgoCademy offer a curated selection of DP problems with step-by-step guidance, which can be invaluable for building your skills.
6.4 Visualize the Problem
Use diagrams, tables, or other visual aids to represent the problem and its subproblems. This can help in understanding the relationships between subproblems and in formulating the recurrence relation.
6.5 Analyze Existing Solutions
Study well-written solutions to DP problems, paying attention to how they approach state representation, recurrence relations, and implementation details. This can help you develop better problem-solving intuition.
6.6 Implement Both Top-Down and Bottom-Up Approaches
For each problem you solve, try implementing both the top-down (memoization) and bottom-up (tabulation) approaches. This will help you understand the pros and cons of each method and when to use them.
6.7 Focus on Pattern Recognition
As you solve more DP problems, try to identify common patterns and problem types. This will help you quickly recognize similar problems in the future and apply appropriate solving strategies.
6.8 Utilize Learning Resources
Take advantage of online courses, tutorials, and platforms like AlgoCademy that offer structured learning paths for mastering dynamic programming. These resources often provide valuable insights and practice problems tailored to different skill levels.
7. Common Dynamic Programming Patterns
To help you navigate the vast landscape of DP problems, it’s useful to familiarize yourself with some common patterns. Here are a few examples:
7.1 0/1 Knapsack
This pattern involves selecting items with specific weights and values to maximize the total value while staying within a weight constraint. Many DP problems are variations of the knapsack problem.
7.2 Longest Common Subsequence (LCS)
The LCS problem involves finding the longest subsequence common to two sequences. This pattern is often used in string manipulation and comparison problems.
7.3 Matrix Chain Multiplication
This pattern focuses on finding the most efficient way to multiply a chain of matrices. It’s a classic example of how DP can be used to optimize operations.
7.4 Shortest Path Problems
Many graph-based shortest path problems, such as the Floyd-Warshall algorithm, use dynamic programming to efficiently compute optimal paths.
8. Implementing a Simple DP Solution
To illustrate the process of implementing a DP solution, let’s look at a simple example: the Fibonacci sequence. While this isn’t the most efficient way to calculate Fibonacci numbers, it serves as a good introduction to DP concepts.
8.1 Top-Down Approach (Memoization)
Here’s a Python implementation of the Fibonacci sequence using the top-down DP approach:
def fibonacci(n, memo={}):
if n <= 1:
return n
if n not in memo:
memo[n] = fibonacci(n-1, memo) + fibonacci(n-2, memo)
return memo[n]
# Example usage
print(fibonacci(10)) # Output: 55
8.2 Bottom-Up Approach (Tabulation)
Now, let’s implement the same solution using the bottom-up DP approach:
def fibonacci(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
# Example usage
print(fibonacci(10)) # Output: 55
Both implementations solve the Fibonacci sequence problem using dynamic programming, but they illustrate the difference between the top-down and bottom-up approaches.
9. Conclusion
Dynamic programming is undoubtedly one of the more challenging topics in computer science and algorithmic problem-solving. Its difficulty stems from the conceptual leap required, the variety of problem types, the high level of abstraction involved, and the steep learning curve. However, with a systematic approach, consistent practice, and the right resources, mastering dynamic programming is an achievable goal.
Remember that the journey to mastering DP is a marathon, not a sprint. Each problem you solve and each concept you grasp brings you one step closer to proficiency. Platforms like AlgoCademy can provide structured learning paths, curated problem sets, and AI-powered assistance to guide you through this journey, helping you build the skills and confidence needed to tackle even the most complex dynamic programming challenges.
As you continue to develop your DP skills, you’ll find that the ability to solve complex problems efficiently becomes second nature. This not only prepares you for technical interviews at top tech companies but also equips you with a powerful tool for tackling real-world optimization problems in your future career as a software engineer or computer scientist.
So embrace the challenge, persist through the difficulties, and remember that every DP problem you solve is a step towards mastery. With time, practice, and the right approach, you’ll find that dynamic programming transforms from a daunting obstacle into a powerful ally in your problem-solving toolkit.