Why Following Best Practices Isn’t Making You a Better Architect
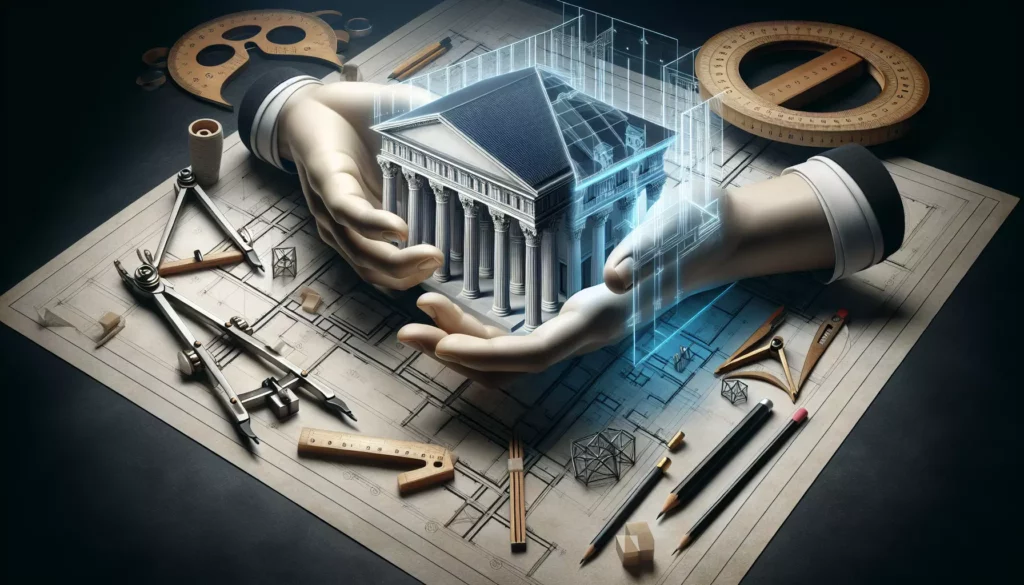
In the world of software architecture, “best practices” have become our guiding stars. They’re the collection of principles, patterns, and approaches that the industry collectively agrees lead to better outcomes. They’re what we learn in books, what senior architects mentor us on, and what we discuss in conferences and meetups.
But here’s a provocative question: What if following best practices isn’t actually making you a better architect?
This isn’t about throwing away everything you’ve learned. Rather, it’s about examining the limitations of a “best practices” mindset and understanding why relying solely on established patterns might be holding you back from true architectural excellence.
The Allure of Best Practices
Let’s start by acknowledging why best practices are so appealing:
- They reduce risk: Following established patterns means you’re less likely to make common mistakes.
- They provide efficiency: You don’t have to reinvent the wheel for every decision.
- They create consistency: Teams can align around shared principles and vocabulary.
- They offer comfort: There’s safety in knowing that others have successfully used these approaches.
These are genuine benefits. When you’re building a system, leveraging patterns like microservices, event sourcing, or CQRS can save you from making architectural mistakes that others have already made and corrected.
But the problem starts when “following best practices” becomes the primary goal rather than a means to an end.
The Hidden Problems with Best Practices
1. Context is Everything (and Best Practices Often Ignore It)
The term “best practice” itself is misleading. It suggests there’s a universal “best” way to do something, regardless of context. In reality, there are only “contextually appropriate practices.”
Consider microservices architecture. It’s often presented as a best practice for building scalable systems. But for a small team building a straightforward application with moderate traffic expectations, microservices might introduce unnecessary complexity, deployment challenges, and operational overhead.
As architect Ruth Malan famously said: “Architecture is about the important stuff. Whatever that is.” The important stuff varies dramatically based on your specific context.
2. Best Practices Lag Behind Innovation
Best practices are, by definition, backwards-looking. They codify what has worked well in the past. While this is valuable, it also means they’re always playing catch-up with the cutting edge.
Consider that at one point, SOAP was considered a best practice for building web services. Teams that rigidly adhered to this “best practice” might have been slower to adopt REST and later GraphQL, potentially missing opportunities for simpler, more flexible APIs.
True architectural innovation often comes from questioning current best practices and exploring alternatives that might better address emerging challenges or leverage new capabilities.
3. They Create a False Sense of Security
Following best practices can lull architects into a false sense that they’re making optimal decisions simply because they’re following established patterns.
I once consulted for a company that had diligently implemented a microservices architecture because it was “best practice.” They had all the right patterns: API gateway, service discovery, circuit breakers, the works. But they had split their domain into services based on technical layers rather than business capabilities, creating a distributed monolith with all the complexity of microservices but none of the benefits.
They had followed the “best practices” mechanically without understanding the underlying principles and context that make those practices valuable.
4. They Can Stifle Critical Thinking
Perhaps most concerning is how best practices can become substitutes for critical thinking. When “because it’s best practice” becomes a sufficient justification for architectural decisions, we stop asking the crucial questions:
- Why is this considered a best practice?
- What problem was it originally designed to solve?
- Does that problem align with our current challenges?
- What are the tradeoffs involved?
- Are there alternative approaches that might work better in our specific context?
These questions are the essence of architectural thinking. When we skip them, we’re not really architecting; we’re just implementing predefined patterns.
The Difference Between Good and Great Architects
Good architects know the best practices. Great architects understand when and why to apply them, when to adapt them, and occasionally, when to discard them entirely in favor of a novel approach.
Let’s explore what sets truly exceptional architects apart from those who simply follow the rulebook.
Understanding the “Why” Behind the “What”
Great architects don’t just know that “CQRS is a best practice for complex domains with different read and write requirements.” They understand the underlying principles:
- Why separating read and write models can simplify complex domains
- How this separation enables independent scaling
- Where the complexity costs manifest
- What kinds of domains benefit most from this approach
This deeper understanding allows them to apply the pattern appropriately, adapt it when needed, or recognize when a simpler approach would suffice.
Consider this code example that demonstrates a simplistic implementation of CQRS:
// A naive CQRS implementation
class OrderWriteModel {
createOrder(orderData) {
// Validate business rules
// Apply domain logic
// Persist to write database
eventBus.publish('OrderCreated', orderData);
}
}
class OrderReadModel {
constructor() {
eventBus.subscribe('OrderCreated', this.handleOrderCreated);
}
handleOrderCreated(orderData) {
// Transform and store in read-optimized format
}
getOrderSummary(orderId) {
// Return from read database
}
}
A good architect might implement this pattern because it’s “best practice.” A great architect would understand that this pattern introduces eventual consistency challenges, operational complexity, and development overhead that may or may not be justified by the specific needs of the system.
Embracing Trade-offs
There are no perfect architectural decisions, only trade-offs. Great architects explicitly acknowledge and navigate these trade-offs rather than hiding behind best practices.
For example, when considering whether to use a monolithic or microservices architecture, a great architect doesn’t simply default to microservices because it’s trendy. Instead, they might create a decision matrix like this:
Consideration | Monolith | Microservices |
---|---|---|
Development Speed (Short-term) | Faster | Slower |
Team Structure | Works well with smaller, centralized teams | Better for larger, distributed teams |
Deployment Complexity | Simpler | More complex |
Scalability | Limited, all-or-nothing | Fine-grained, targeted |
Technology Diversity | Limited | Flexible |
They then weigh these factors based on the specific context of their project, team, and organization to make an informed decision rather than defaulting to what’s considered “best practice.”
Continuous Learning and Adaptation
Great architects view best practices as starting points for learning, not ending points. They stay curious about:
- New architectural patterns and approaches
- Emerging technologies and their architectural implications
- Failures and successes in similar systems
- Academic research in distributed systems, databases, etc.
They’re not afraid to experiment with new approaches when appropriate, knowing that today’s experiment might become tomorrow’s best practice.
Moving Beyond Best Practices: A Better Approach
If blindly following best practices isn’t the path to architectural excellence, what is? Here’s a more nuanced approach:
Start with First Principles
Rather than beginning with patterns, start with principles and the specific problems you’re trying to solve. Ask questions like:
- What are the core business capabilities this system needs to enable?
- What quality attributes (scalability, reliability, security, etc.) are most important for this system?
- What constraints (technical, organizational, regulatory) must we work within?
For example, instead of immediately deciding to use microservices, you might identify that independent deployability is crucial for your team structure. This principle could lead you to microservices, but it might also lead to a well-modularized monolith with clear boundaries if that better suits your context.
Understand the Problem Space Deeply
Great architecture starts with a deep understanding of the domain and the problem you’re solving. This means:
- Spending time with domain experts
- Creating shared models (like Domain-Driven Design suggests)
- Identifying the core domain and supporting subdomains
This understanding provides the context necessary to evaluate which architectural approaches are truly appropriate.
Learn Patterns as a Vocabulary, Not as Rules
Architectural patterns are incredibly valuable, but they’re most useful as a vocabulary for describing solutions rather than as prescriptive rules.
When you understand patterns like CQRS, Event Sourcing, or Hexagonal Architecture as concepts with specific benefits and tradeoffs, you can mix, match, and adapt them to create solutions tailored to your specific context.
For instance, you might apply CQRS principles only to the most complex parts of your domain while keeping simpler parts more straightforward.
Make Deliberate, Informed Decisions
Document your architectural decisions along with the context, constraints, and reasoning that led to them. Architecture Decision Records (ADRs) are a great format for this.
Here’s a simplified example:
# Architecture Decision Record: Adopting Event Sourcing for Order Processing
## Context
Our order processing system needs to maintain a complete audit trail of all changes to orders for compliance reasons. Additionally, we need to reconstruct the state of orders at any point in time for dispute resolution.
## Decision
We will implement Event Sourcing for the Order aggregate in our system. All changes to orders will be stored as a sequence of events rather than just the current state.
## Consequences
- Positive: Complete audit trail built into the architecture
- Positive: Ability to reconstruct historical states
- Negative: Increased complexity in the programming model
- Negative: Need for eventual consistency in read models
## Alternatives Considered
1. Traditional CRUD with separate audit logging
2. Temporal tables in the database
This approach forces you to think through decisions rather than just applying best practices by default.
Embrace Evolutionary Architecture
Accept that your architecture will evolve as you learn more about the problem domain, as requirements change, and as technology advances. Build in mechanisms for this evolution:
- Clear interfaces between components
- Feature toggles for new architectural approaches
- Metrics to evaluate architectural decisions
- Regular architectural reviews
This mindset prevents you from becoming too attached to particular patterns or approaches and keeps you focused on the outcomes rather than the means.
Real-World Examples: Beyond Best Practices
Let’s examine some real-world examples of architectural thinking that goes beyond best practices.
Amazon’s Service-Oriented Architecture
When Amazon began its transition to a service-oriented architecture in the early 2000s, microservices weren’t yet a “best practice.” Instead, Amazon’s architects focused on the specific problems they were facing:
- Teams were moving too slowly due to tight coupling
- The monolithic codebase was becoming unwieldy
- Different parts of the system had different scaling needs
Their “two-pizza team” principle and service-oriented approach weren’t about following best practices but about solving these specific organizational and technical challenges. The principles they developed (like “you build it, you run it”) later influenced what would become microservices “best practices,” but Amazon was thinking from first principles rather than following a playbook.
Netflix’s Chaos Engineering
When Netflix moved to the cloud, there was no “best practice” for ensuring reliability in this new environment. Instead of just implementing traditional high-availability patterns, they invented Chaos Engineering.
By deliberately introducing failures into their production system (starting with their famous “Chaos Monkey” tool), they created a fundamentally new approach to system reliability that challenged conventional wisdom about how to build resilient systems.
This wasn’t about following best practices; it was about deeply understanding their specific reliability challenges in a cloud environment and developing novel approaches to address them.
Shopify’s Modular Monolith
While many companies rushed to adopt microservices as a “best practice,” Shopify took a different approach. They recognized that for their context, a well-designed monolith with clear module boundaries offered many of the benefits of microservices with less operational complexity.
Their “Modular Monolith” approach wasn’t about ignoring best practices but about understanding the principles behind microservices (like modularity, clear boundaries, and team autonomy) and finding a different way to achieve those benefits that better suited their specific context.
Here’s a simplified example of how they might structure code in their modular monolith:
// In the Products module
module Shopify::Products
class Product
# Product logic here
# Clear, well-defined interfaces to other modules
def add_to_cart(cart_id, quantity)
Shopify::Carts.add_product(cart_id, self.id, quantity)
end
end
end
// In the Carts module
module Shopify::Carts
class CartService
def self.add_product(cart_id, product_id, quantity)
# Cart logic here
end
end
end
This approach maintains clear boundaries between modules while avoiding the distributed systems challenges of microservices.
How to Develop Your Architectural Thinking
If you want to move beyond simply following best practices to developing true architectural thinking, here are some practical steps:
Study the Classics, But Ask “Why?”
Read the canonical texts on software architecture, patterns, and principles, but always ask:
- Why does this pattern exist?
- What problem was it solving?
- What were the alternatives?
- What are the tradeoffs?
Books like “Clean Architecture” by Robert C. Martin, “Building Evolutionary Architectures” by Neal Ford et al., and “Designing Data-Intensive Applications” by Martin Kleppmann are excellent for developing this deeper understanding.
Learn Across Domains
Great architectural thinking often comes from cross-pollination between domains. Study architecture patterns from:
- Different types of systems (web, enterprise, embedded, etc.)
- Different industries (finance, healthcare, gaming, etc.)
- Different technological eras (mainframe, client-server, cloud, etc.)
This broader perspective helps you see patterns and principles that transcend specific technologies or trends.
Practice Decision-Making with Constraints
Give yourself architectural challenges with specific constraints:
- “How would you design this system if you couldn’t use a relational database?”
- “What if you had to support 10x the current load with the same infrastructure?”
- “How would your architecture change if regulatory requirements mandated all data stay in-country?”
These exercises force you to think beyond standard patterns and develop more flexible architectural thinking.
Analyze Existing Systems
Study the architecture of systems you admire or use regularly:
- What architectural patterns do they employ?
- Why might they have made those choices?
- What quality attributes do they prioritize?
- How have they evolved over time?
Many tech companies publish details about their architecture in blogs, conference talks, and academic papers. These real-world case studies are invaluable for understanding how architectural decisions play out in practice.
Build and Reflect
Nothing beats practical experience. Build systems, observe what works and what doesn’t, and reflect on the outcomes. Some approaches:
- Create side projects with different architectural approaches
- Volunteer for architectural responsibilities in your current role
- Participate in architectural reviews
- Mentor others on architectural concepts
After each project or significant phase, conduct a retrospective specifically focused on architectural decisions and their outcomes.
Balancing Innovation and Proven Approaches
While this article argues against blindly following best practices, it’s important to strike a balance. You don’t want to reinvent everything or ignore the collective wisdom of the industry.
When to Embrace Best Practices
Best practices are most valuable when:
- You’re working in a well-understood domain: If you’re building a standard web application with common requirements, established patterns likely address your needs well.
- You have time constraints: When you need to move quickly, starting with proven approaches can provide a solid foundation.
- You’re addressing common technical challenges: For problems like authentication, caching, or job processing, established solutions often represent years of refinement.
- Your team is less experienced: Best practices can provide guardrails that help less experienced teams avoid common pitfalls.
When to Innovate Beyond Best Practices
Innovation becomes more important when:
- You’re facing unique challenges: If your specific requirements don’t align well with common patterns, you’ll need to adapt or create new approaches.
- You’re working at extreme scale: At very high scale, the assumptions underlying many best practices may break down, requiring novel solutions.
- You’re in a rapidly evolving domain: In cutting-edge areas, best practices may not yet exist or may be changing rapidly.
- Standard approaches create unacceptable trade-offs: If following best practices would compromise critical quality attributes for your system, you need alternatives.
A Pragmatic Approach
A balanced approach might look like this:
- Start with understanding your specific context, constraints, and quality requirements
- Consider relevant best practices as potential solutions
- Evaluate how well each practice aligns with your specific needs
- Adopt practices that fit well, adapt those that need adjustment, and innovate where nothing suitable exists
- Continuously evaluate and evolve your approach based on feedback and changing requirements
This approach leverages the value of best practices while maintaining the critical thinking and context-awareness that characterize great architectural work.
Conclusion: From Following to Understanding
The journey from good to great architect isn’t about memorizing more patterns or collecting more best practices. It’s about developing a deeper understanding of architectural principles, trade-offs, and context-sensitivity.
Great architects:
- Understand the why behind architectural patterns and practices
- Recognize that context determines what’s “best” in any given situation
- Make deliberate, informed decisions rather than defaulting to convention
- Balance proven approaches with innovation where needed
- Focus on outcomes rather than adherence to specific patterns
So by all means, study the best practices. Learn the patterns. Understand the principles. But remember that they’re tools in your toolkit, not a substitute for architectural thinking.
The next time someone justifies an architectural decision with “because it’s best practice,” gently push for deeper understanding. Ask about the specific context, the trade-offs considered, and the alternatives evaluated. This is how we collectively move from a “best practice” mindset to true architectural thinking.
In the end, the best architects aren’t those who follow the rules most faithfully, but those who understand when the rules apply, when they need adaptation, and occasionally, when they need to be broken entirely in service of creating systems that truly meet their specific needs.
Your goal shouldn’t be to follow best practices perfectly, but to understand deeply enough that you can make the right architectural decisions for your unique context. That’s what separates the good architects from the truly great ones.