Why Failure Is Essential in the Journey to Learn to Code
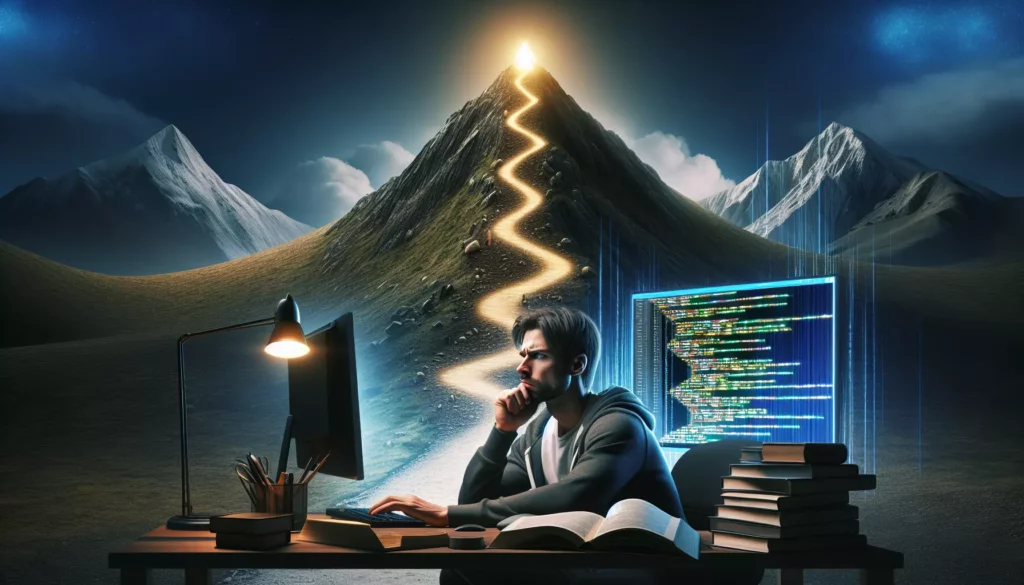
In the world of coding and software development, failure is not just a possibility—it’s an inevitability. As aspiring programmers embark on their journey to master the art of coding, they quickly discover that errors, bugs, and setbacks are par for the course. However, what many newcomers to the field don’t realize is that these moments of failure are not just obstacles to overcome; they are essential stepping stones on the path to becoming a proficient coder.
At AlgoCademy, we believe that embracing failure is a crucial part of the learning process. Our platform is designed to provide a safe and supportive environment for learners to experiment, make mistakes, and grow from their experiences. In this article, we’ll explore why failure is not only unavoidable but also invaluable in your journey to learn to code.
The Nature of Coding: A Process of Trial and Error
Coding, at its core, is a process of problem-solving. When you write code, you’re essentially providing instructions to a computer to perform specific tasks. However, computers are literal machines that execute exactly what they’re told—no more, no less. This means that even the smallest error in your code can lead to unexpected results or complete failure of your program.
For beginners, this can be frustrating. You might spend hours writing what you think is perfect code, only to be met with a barrage of error messages when you try to run it. But here’s the thing: this is normal. Even experienced programmers face errors and bugs on a daily basis. The difference is in how they approach these challenges.
Learning from Syntax Errors
One of the most common types of errors beginners encounter are syntax errors. These occur when you’ve made a mistake in the structure of your code that violates the rules of the programming language you’re using. For example, in Python, forgetting to close a parenthesis or using the wrong indentation can lead to syntax errors.
Here’s an example of code with a syntax error:
def greet(name)
print("Hello, " + name + "!"
greet("Alice")
This code will fail because it’s missing a colon after the function definition and a closing parenthesis in the print statement. Correcting it would look like this:
def greet(name):
print("Hello, " + name + "!")
greet("Alice")
Encountering and fixing these errors teaches you the importance of precision in coding and helps you internalize the syntax rules of your chosen language.
Debugging: A Skill Born from Failure
Beyond syntax errors, logical errors or bugs in your code can be even more challenging to identify and fix. These are instances where your code runs without throwing errors, but it doesn’t produce the expected output. Debugging—the process of identifying and fixing these issues—is a crucial skill that can only be developed through experience with failure.
For instance, consider this Python code that’s meant to find the sum of even numbers in a list:
def sum_even_numbers(numbers):
total = 0
for num in numbers:
if num % 2 == 0:
total += num
return total
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
result = sum_even_numbers(numbers)
print(result) # Expected output: 30
If you run this code and get an unexpected result, you’ll need to debug it. This process involves carefully examining your logic, perhaps adding print statements to track the flow of your program, and testing different inputs. Through this process, you not only fix the current issue but also develop problem-solving skills that will serve you throughout your coding career.
The Growth Mindset: Turning Failures into Opportunities
Adopting a growth mindset is crucial when learning to code. This concept, popularized by psychologist Carol Dweck, suggests that abilities and intelligence can be developed through effort, learning, and persistence. In the context of coding, this means viewing each error or bug not as a personal failure, but as an opportunity to learn and improve.
Embracing Challenges
When you encounter a difficult coding problem or a persistent bug, it’s easy to feel discouraged. However, these challenges are precisely what will help you grow as a programmer. Each obstacle you overcome builds your problem-solving skills and expands your knowledge base.
At AlgoCademy, we structure our learning paths to gradually increase in difficulty. This approach ensures that learners are constantly pushing their boundaries and facing new challenges. By conquering these hurdles, you build confidence in your ability to tackle complex coding problems.
Learning from Mistakes
Every mistake you make while coding is an opportunity to learn something new. Whether it’s a misunderstanding of a programming concept or a logical error in your algorithm, each failure provides valuable feedback that can improve your skills.
For example, let’s say you’re working on a sorting algorithm and your implementation of bubble sort is not working correctly:
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
return arr
numbers = [64, 34, 25, 12, 22, 11, 90]
sorted_numbers = bubble_sort(numbers)
print(sorted_numbers) # Output might not be fully sorted
If this implementation doesn’t produce a fully sorted array, you’ll need to revisit your understanding of the bubble sort algorithm. Through this process, you might realize that you need an additional flag to check if any swaps were made in a pass, allowing the algorithm to terminate early if the array is already sorted:
def improved_bubble_sort(arr):
n = len(arr)
for i in range(n):
swapped = False
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
swapped = True
if not swapped:
break
return arr
numbers = [64, 34, 25, 12, 22, 11, 90]
sorted_numbers = improved_bubble_sort(numbers)
print(sorted_numbers) # Output will be fully sorted
This experience not only corrects your implementation but also deepens your understanding of sorting algorithms and efficiency considerations.
Building Resilience: The Key to Long-Term Success
Learning to code is not just about acquiring technical skills; it’s also about developing the mental fortitude to persist in the face of challenges. Every time you encounter a failure and push through it, you’re building resilience—a quality that’s invaluable not just in coding, but in all aspects of life.
Overcoming Imposter Syndrome
Many programmers, even experienced ones, struggle with imposter syndrome—the feeling that you’re not as competent as others perceive you to be. This feeling can be particularly acute for beginners who are constantly confronted with how much they don’t know.
However, by embracing failure as a natural part of the learning process, you can begin to overcome these feelings of inadequacy. Remember that every programmer, no matter how skilled, started as a beginner and faced the same challenges you’re facing now.
Developing Problem-Solving Skills
Each failure you encounter in coding presents a unique problem to solve. As you work through these issues, you’re not just fixing bugs—you’re developing critical thinking and problem-solving skills that will serve you well throughout your career.
For instance, consider the process of optimizing a recursive function to calculate Fibonacci numbers:
def fibonacci(n):
if n <= 1:
return n
return fibonacci(n-1) + fibonacci(n-2)
print(fibonacci(35)) # This will take a long time to compute
This naive implementation works but is highly inefficient for large values of n. As you encounter performance issues with this code, you might explore concepts like memoization or dynamic programming to optimize it:
def fibonacci_optimized(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fibonacci_optimized(n-1, memo) + fibonacci_optimized(n-2, memo)
return memo[n]
print(fibonacci_optimized(35)) # This will compute much faster
Through this process, you’re not just learning about Fibonacci numbers; you’re developing skills in algorithm analysis and optimization that can be applied to a wide range of programming challenges.
The Role of Community in Learning from Failure
While individual perseverance is crucial, learning to code doesn’t have to be a solitary journey. In fact, one of the most effective ways to learn from failure is to engage with a community of fellow learners and experienced programmers.
Collaborative Problem-Solving
When you’re stuck on a problem, sometimes a fresh perspective can make all the difference. Platforms like AlgoCademy provide forums and community features where learners can share their challenges and work together to find solutions.
For example, if you’re struggling with understanding recursion, you might post a question like this:
I'm having trouble understanding how this recursive function to calculate factorials works:
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n-1)
Can someone explain the step-by-step process of how this function calculates factorial(5)?
By engaging with the community, you not only get help with your specific problem but also learn different approaches and explanations that can enhance your overall understanding of the concept.
Sharing Experiences
Hearing about others’ experiences with failure can be incredibly reassuring and motivating. When you realize that even experienced programmers face challenges and make mistakes, it normalizes the struggle and helps you persevere.
Many coding communities have threads or channels dedicated to sharing “war stories” or lessons learned from difficult bugs. These stories not only provide technical insights but also emotional support and encouragement.
Practical Strategies for Learning from Failure
While understanding the importance of failure is crucial, it’s equally important to have practical strategies for turning these failures into learning opportunities. Here are some approaches you can adopt to make the most of your coding challenges:
Keep a Coding Journal
Maintaining a record of the problems you encounter, the solutions you try, and the eventual resolutions can be incredibly valuable. This practice not only helps you track your progress but also creates a personal knowledge base you can refer back to.
Your journal entries might look something like this:
Date: 2023-06-15
Problem: Reverse a string in Python
Initial Attempt:
def reverse_string(s):
return s[::-1]
Reflection: This solution works, but I don't fully understand how slicing with a step of -1 works. Need to research more on string slicing in Python.
Follow-up: Learned that s[::-1] creates a new string by starting from the end of s and moving backwards. Alternative solution using a loop:
def reverse_string_loop(s):
reversed_str = ''
for char in s:
reversed_str = char + reversed_str
return reversed_str
This helped me understand the process step-by-step.
Set Learning Goals, Not Just Performance Goals
Instead of focusing solely on completing coding challenges or projects, set goals around what you want to learn. This shift in perspective can help you view failures as progress towards your learning objectives rather than setbacks.
For example, instead of “Complete 50 coding challenges this month,” your goal might be “Understand and implement 5 different sorting algorithms.” This approach encourages deeper learning and allows you to celebrate the knowledge gained from each attempt, successful or not.
Practice Deliberate Reflection
After completing a coding task or project, take time to reflect on the process. Ask yourself questions like:
- What were the main challenges I faced?
- How did I overcome these challenges?
- What new concepts or techniques did I learn?
- How can I apply what I’ve learned to future projects?
This reflection process helps consolidate your learning and identify areas for further improvement.
Embrace Code Reviews
Whether you’re working on personal projects or contributing to open source, seeking code reviews can provide invaluable feedback. Even if your code works, experienced developers can offer insights on best practices, efficiency improvements, and alternative approaches.
For instance, you might submit a pull request with a function to check if a number is prime:
def is_prime(n):
if n < 2:
return False
for i in range(2, n):
if n % i == 0:
return False
return True
A code reviewer might suggest optimizations like:
def is_prime_optimized(n):
if n < 2:
return False
if n == 2:
return True
if n % 2 == 0:
return False
for i in range(3, int(n**0.5) + 1, 2):
if n % i == 0:
return False
return True
This feedback not only improves your code but also introduces you to new concepts and optimization techniques.
The Long-Term Benefits of Embracing Failure
As you progress in your coding journey, the ability to learn from failure becomes increasingly valuable. Here are some of the long-term benefits you can expect from embracing this mindset:
Accelerated Learning
By viewing each failure as a learning opportunity, you’ll find that you absorb new concepts and techniques more quickly. This accelerated learning can help you stay current in a field that’s constantly evolving.
Improved Problem-Solving Skills
The more you encounter and overcome coding challenges, the better you’ll become at breaking down complex problems and developing effective solutions. This skill is highly valued in the tech industry and can set you apart in job interviews and professional settings.
Increased Creativity
Failure often forces you to think outside the box and come up with innovative solutions. This fosters creativity in your coding approach, leading to more elegant and efficient solutions over time.
Greater Resilience
As you become more comfortable with failure, you’ll develop greater resilience not just in coding, but in all aspects of your life. This resilience will serve you well as you tackle increasingly complex projects and face new challenges in your career.
Continuous Improvement
Embracing failure as part of the learning process instills a habit of continuous improvement. You’ll always be looking for ways to enhance your skills and refine your code, keeping you at the forefront of your field.
Conclusion: Failure as a Stepping Stone to Success
In the journey to learn to code, failure is not just inevitable—it’s essential. Each error you encounter, each bug you fix, and each concept you struggle to grasp is a stepping stone on the path to becoming a proficient programmer. By embracing these challenges and learning from them, you’re not just acquiring coding skills; you’re developing resilience, problem-solving abilities, and a growth mindset that will serve you well throughout your career.
At AlgoCademy, we believe in creating an environment where failure is seen as an opportunity for growth. Our interactive coding tutorials, AI-powered assistance, and supportive community are all designed to help you navigate the challenges of learning to code and emerge as a skilled, confident programmer.
Remember, every great programmer started as a beginner, facing the same struggles you’re facing now. What sets successful coders apart is not an absence of failures, but a willingness to learn from them and keep pushing forward. So the next time you encounter a bug in your code or struggle with a new concept, embrace it as an opportunity to grow. Your future self—the skilled programmer you’re working to become—will thank you for it.
Happy coding, and may your failures be as enlightening as your successes!