Why Failure in Coding Interviews Is Often the Best Thing That Can Happen to You
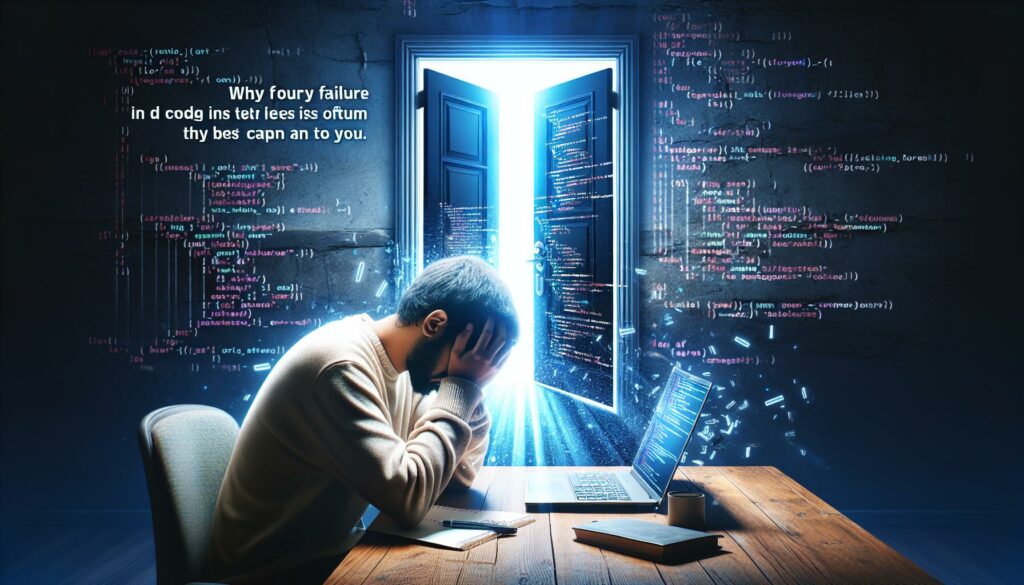
In the competitive world of tech, coding interviews are often seen as make-or-break moments. Aspiring developers and seasoned programmers alike can feel the weight of these high-stakes encounters. But what if we told you that failing a coding interview could be one of the best things to happen in your career? In this comprehensive guide, we’ll explore why failure in coding interviews can be a catalyst for growth, learning, and ultimate success in the tech industry.
The Reality of Coding Interviews
Before we dive into the benefits of failure, let’s set the stage by understanding the nature of coding interviews, especially those conducted by major tech companies often referred to as FAANG (Facebook, Amazon, Apple, Netflix, Google).
The High-Pressure Environment
Coding interviews are notorious for their intense, pressure-cooker atmosphere. Candidates are often asked to solve complex algorithmic problems on the spot, explain their thought process, and write clean, efficient code—all while being observed and evaluated. This environment can be nerve-wracking even for the most experienced developers.
The Breadth of Knowledge Required
These interviews typically cover a wide range of topics, including:
- Data structures (arrays, linked lists, trees, graphs, etc.)
- Algorithms (sorting, searching, dynamic programming, etc.)
- System design
- Object-oriented programming concepts
- Problem-solving skills
- Code optimization and efficiency
The sheer breadth of knowledge required can be overwhelming, and it’s not uncommon for candidates to stumble on certain areas.
The Subjective Nature of Evaluation
While there are objective elements to coding interviews, such as whether a solution works correctly, there’s also a significant subjective component. Interviewers assess factors like communication skills, problem-solving approach, and cultural fit, which can vary based on the interviewer’s personal preferences and experiences.
The Hidden Benefits of Interview Failure
Now that we’ve established the challenging nature of coding interviews, let’s explore why failing one can be a blessing in disguise.
1. Identifying Knowledge Gaps
Failure in a coding interview often serves as a powerful diagnostic tool. It highlights areas where your knowledge or skills may be lacking, providing a clear roadmap for improvement. For instance, if you struggle with a graph traversal problem, it becomes evident that you need to focus more on graph algorithms.
Action Item:
After a failed interview, make a list of topics you found challenging. Use this as a study guide to strengthen your weak areas.
2. Improving Problem-Solving Skills
Each interview question you face, whether you solve it successfully or not, contributes to your problem-solving repertoire. The process of tackling these challenges enhances your ability to break down complex problems, consider edge cases, and develop efficient solutions.
Example:
Let’s say you encountered a dynamic programming problem you couldn’t solve. Even if you didn’t arrive at the correct solution, the experience of grappling with the problem improves your approach to similar challenges in the future.
3. Enhancing Communication Skills
Coding interviews aren’t just about writing code; they’re also about effectively communicating your thought process. Failing an interview often highlights areas where your communication could improve, such as explaining your approach clearly or asking clarifying questions.
Tip:
Practice explaining your problem-solving approach out loud, even when studying alone. This will help you articulate your thoughts more clearly during interviews.
4. Building Resilience
Failure is an inevitable part of any professional journey, and coding interviews are no exception. Each unsuccessful attempt builds resilience, teaching you to bounce back stronger. This resilience is crucial not just for future interviews, but for navigating the challenges of a career in tech.
Quote:
“Success is not final, failure is not fatal: it is the courage to continue that counts.” – Winston Churchill
5. Gaining Insider Knowledge
Every interview, successful or not, provides valuable insights into the interview process, the company culture, and the types of problems you might encounter. This insider knowledge is invaluable for future preparation.
Action Item:
After each interview, regardless of the outcome, write down the types of questions asked, the interview structure, and any insights you gained about the company or role.
6. Refining Your Career Goals
Sometimes, failing an interview can lead to important realizations about your career path. It might help you understand what type of role or company culture truly aligns with your goals and values.
Reflection Question:
After a failed interview, ask yourself: “Does this setback change my career aspirations, or does it reinforce my determination to succeed in this field?”
Turning Failure into Success: Practical Steps
Now that we’ve explored the benefits of failure, let’s discuss how to leverage these experiences for future success.
1. Conduct a Thorough Post-Mortem
After each interview, regardless of the outcome, take time to reflect on the experience. Ask yourself:
- What questions were particularly challenging?
- Where did I feel most confident?
- What feedback did the interviewer provide?
- How can I improve my performance next time?
2. Create a Structured Study Plan
Based on your post-mortem analysis, develop a targeted study plan. This might include:
- Revisiting fundamental data structures and algorithms
- Practicing coding problems on platforms like AlgoCademy
- Studying system design principles
- Improving your knowledge of specific programming languages or frameworks
3. Leverage Online Resources
Take advantage of the wealth of online resources available for interview preparation. Platforms like AlgoCademy offer interactive coding tutorials, AI-powered assistance, and step-by-step guidance to help you progress from beginner-level coding to advanced interview preparation.
Code Example: Using AlgoCademy’s AI Assistant
Here’s an example of how you might use AlgoCademy’s AI assistant to help solve a coding problem:
// Problem: Implement a function to reverse a linked list
// Your initial attempt
function reverseLinkedList(head) {
// Your code here
}
// AI Assistant suggestion
"To reverse a linked list, you can use three pointers: prev, current, and next.
Here's a step-by-step approach:
1. Initialize prev as null, current as head
2. Traverse the list:
a. Store next node
b. Reverse the link
c. Move prev and current one step forward
3. Return prev as the new head
Let's implement this:"
function reverseLinkedList(head) {
let prev = null;
let current = head;
while (current !== null) {
let next = current.next;
current.next = prev;
prev = current;
current = next;
}
return prev;
}
4. Practice Mock Interviews
Simulate the interview environment as closely as possible. This can help reduce anxiety and improve your performance under pressure. Consider:
- Partnering with a friend or colleague for mock interviews
- Using online platforms that offer mock interview services
- Recording yourself solving problems to review your communication style
5. Focus on Soft Skills
While technical skills are crucial, don’t neglect the importance of soft skills in coding interviews. Practice:
- Clearly explaining your thought process
- Active listening and asking clarifying questions
- Maintaining composure under pressure
- Demonstrating enthusiasm and a growth mindset
6. Stay Updated with Industry Trends
The tech industry evolves rapidly. Stay current with:
- New programming languages and frameworks
- Emerging technologies (e.g., AI, blockchain)
- Best practices in software development
- Industry news and developments
Embracing the Growth Mindset
Perhaps the most crucial aspect of turning interview failure into success is adopting a growth mindset. This concept, popularized by psychologist Carol Dweck, emphasizes the belief that abilities and intelligence can be developed through dedication and hard work.
Key Principles of a Growth Mindset in Coding Interviews:
- Embrace challenges: View difficult interview questions as opportunities to learn and grow, not as threats to your abilities.
- Persist in the face of setbacks: See failure as a temporary setback, not a permanent condition.
- Learn from criticism: Treat feedback from interviewers as valuable input for improvement, not as personal attacks.
- Find lessons and inspiration in others’ success: Instead of feeling threatened by others’ achievements, use them as motivation and learning opportunities.
Applying the Growth Mindset to Coding Interviews
Here’s how you can apply the growth mindset principles to your coding interview experiences:
- Reframe failure: Instead of saying “I failed this interview,” say “I learned valuable lessons from this interview experience.”
- Set learning goals: Before each interview, set goals focused on what you want to learn, not just on getting the job.
- Celebrate effort: Acknowledge the hard work you put into preparation, regardless of the outcome.
- Seek out challenges: Deliberately tackle interview questions that are outside your comfort zone to expand your skills.
Real-World Success Stories
To illustrate the power of learning from interview failures, let’s look at some real-world examples of tech professionals who turned setbacks into success:
1. The Persistent Engineer
Sarah, a software engineer, applied to a FAANG company and failed the interview three times over two years. Each time, she meticulously analyzed her performance, identified weak areas, and focused on improvement. On her fourth attempt, she not only passed but received offers from multiple top-tier tech companies.
2. The Career Changer
Mike, a former teacher, decided to switch to a career in tech. His first coding interviews were disastrous, as he lacked the necessary technical knowledge. Instead of giving up, he used these experiences to create a structured learning plan. After six months of intense study and practice, he successfully landed a role as a junior developer at a startup.
3. The Startup Founder
Lisa failed several coding interviews at established tech companies. This experience led her to realize that her true passion lay in creating her own product. She used the knowledge gained from her interview preparations to launch a successful tech startup, which now employs over 50 people.
Conclusion: Embracing Failure as a Stepping Stone to Success
In the world of coding interviews, failure is not just common; it’s often a crucial step on the path to success. By reframing these experiences as opportunities for growth, leveraging resources like AlgoCademy, and adopting a growth mindset, you can transform interview setbacks into powerful catalysts for professional development.
Remember, every failed interview brings you one step closer to success. It provides invaluable insights, helps you identify areas for improvement, and builds the resilience necessary for a thriving career in tech. So the next time you face a challenging coding interview, embrace it as an opportunity to learn, grow, and ultimately succeed in your tech career journey.
As you continue your preparation, keep in mind that platforms like AlgoCademy are designed to support your growth at every stage. From interactive tutorials to AI-powered assistance, these tools can help you transform your coding interview experiences from sources of anxiety into stepping stones toward your dream tech career.
Embrace the journey, learn from every experience, and remember: in the world of coding interviews, failure is often just the beginning of your success story.