Why Do I Keep Getting Stuck on Simple Coding Problems? Overcoming Common Hurdles in Programming
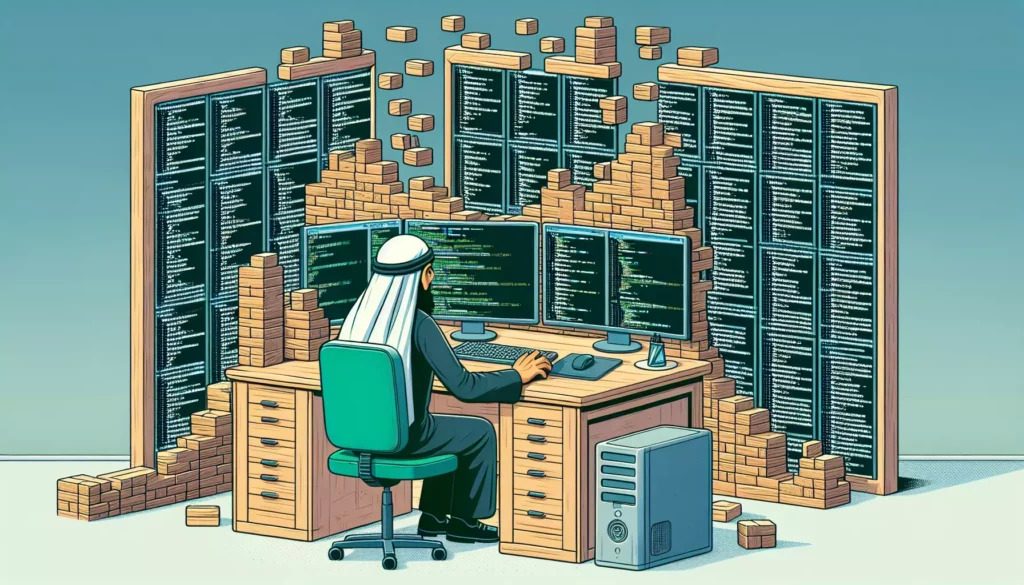
As you embark on your coding journey, it’s not uncommon to find yourself scratching your head over what seems like a simple problem. You’re not alone in this experience. Many aspiring programmers, and even seasoned developers, occasionally hit roadblocks when tackling coding challenges. In this comprehensive guide, we’ll explore the reasons behind these struggles and provide practical strategies to help you overcome them.
Understanding the Root Causes
Before we dive into solutions, it’s crucial to understand why you might be getting stuck on simple coding problems. Here are some common reasons:
1. Lack of Fundamental Knowledge
Often, what appears to be a simple problem actually requires a solid understanding of programming fundamentals. If you’re missing key concepts, even basic tasks can become challenging.
2. Overthinking the Problem
Sometimes, we tend to overcomplicate things. You might be looking for a complex solution when a simpler approach would suffice.
3. Limited Problem-Solving Skills
Programming is as much about problem-solving as it is about coding. If you’re not used to breaking down problems into smaller, manageable parts, you might struggle to find a starting point.
4. Insufficient Practice
Like any skill, coding improves with practice. If you’re not regularly working on coding problems, you might find it difficult to apply your knowledge effectively.
5. Imposter Syndrome
Sometimes, the belief that you’re not good enough or that you should be able to solve these problems easily can create mental blocks that hinder your progress.
Strategies to Overcome Coding Challenges
Now that we’ve identified some common causes, let’s explore strategies to help you overcome these hurdles and improve your coding skills.
1. Strengthen Your Foundation
A solid understanding of programming basics is crucial. Make sure you have a good grasp of the following:
- Data types and variables
- Control structures (if statements, loops)
- Functions and methods
- Basic data structures (arrays, lists, dictionaries)
- Object-oriented programming concepts
If you find yourself struggling with these concepts, it’s worth revisiting them through tutorials, courses, or textbooks.
2. Practice, Practice, Practice
Consistent practice is key to improving your coding skills. Here are some ways to incorporate regular practice into your routine:
- Solve coding challenges on platforms like LeetCode, HackerRank, or CodeWars
- Participate in coding competitions or hackathons
- Work on personal projects that interest you
- Contribute to open-source projects
Remember, the goal is not just to solve problems, but to understand the underlying concepts and improve your problem-solving skills.
3. Break Down the Problem
When faced with a coding problem, resist the urge to start coding immediately. Instead, follow these steps:
- Read the problem statement carefully and make sure you understand it
- Break the problem down into smaller, manageable sub-problems
- Solve each sub-problem one at a time
- Combine the solutions to solve the overall problem
This approach, often referred to as “divide and conquer,” can make even complex problems more approachable.
4. Use Pseudocode
Before diving into actual coding, try writing pseudocode. Pseudocode is a plain language description of the steps in an algorithm. It can help you organize your thoughts and plan your solution without getting bogged down in syntax details.
Here’s an example of pseudocode for a simple problem of finding the maximum number in an array:
function findMaxNumber(array):
set max to first element of array
for each element in array:
if element > max:
set max to element
return max
Once you have your pseudocode, translating it into actual code becomes much easier.
5. Learn to Debug Effectively
Debugging is an essential skill for any programmer. When you’re stuck, effective debugging can help you identify where things are going wrong. Here are some debugging tips:
- Use print statements or logging to track the flow of your program
- Utilize breakpoints and step through your code line by line
- Check your assumptions about what your code is doing
- Read error messages carefully – they often point directly to the problem
Most modern IDEs have powerful debugging tools. Learn to use them effectively to save time and frustration.
6. Embrace the Power of Rubber Duck Debugging
“Rubber Duck Debugging” is a method of debugging code by explaining it, line-by-line, to an inanimate object (traditionally, a rubber duck). This process often helps you spot errors or inconsistencies in your logic.
You don’t actually need a rubber duck – explaining your code out loud to yourself or writing it down can be just as effective. The act of articulating your thought process can often lead to “aha!” moments where you spot the issue.
7. Learn from Others
Programming is a collaborative field, and there’s always something to learn from others. Here are some ways to leverage the knowledge of the programming community:
- Join coding forums or communities (like Stack Overflow or Reddit’s programming subreddits)
- Participate in pair programming sessions
- Attend coding meetups or workshops
- Read other people’s code on platforms like GitHub
Remember, it’s okay to ask for help when you’re stuck. Just make sure you’ve made a genuine effort to solve the problem on your own first.
8. Improve Your Algorithmic Thinking
Many coding problems require a good understanding of algorithms and data structures. Improving your algorithmic thinking can make a big difference in your problem-solving abilities. Here are some ways to do this:
- Study common algorithms and data structures
- Analyze the time and space complexity of your solutions
- Practice implementing algorithms from scratch
- Solve problems using different approaches and compare their efficiency
Resources like “Introduction to Algorithms” by Cormen, Leiserson, Rivest, and Stein, or online courses on algorithms can be incredibly helpful.
9. Use Online Resources Wisely
The internet is a treasure trove of coding resources, but it’s important to use them wisely. Here are some tips:
- Use documentation as your first resource – it’s often the most accurate and up-to-date
- When looking for solutions online, try to understand the code, not just copy-paste it
- Use multiple sources to verify information
- Be cautious of outdated information, especially for rapidly evolving technologies
Remember, the goal is to learn and improve, not just to find quick fixes.
10. Take Breaks and Manage Stress
Sometimes, the best thing you can do when stuck on a problem is to step away from it for a while. Taking breaks can help you:
- Clear your mind and return to the problem with fresh eyes
- Reduce stress and frustration
- Allow your subconscious mind to work on the problem
Don’t underestimate the power of a good night’s sleep or a short walk in helping you solve coding problems.
Common Simple Coding Problems and How to Approach Them
Let’s look at a few examples of simple coding problems that often trip up beginners, and how to approach them:
1. Reversing a String
Problem: Write a function to reverse a string.
Approach:
- Understand that strings are often immutable, so you might need to convert to a mutable data structure
- Consider using a two-pointer approach, swapping characters from the start and end
- Alternatively, use built-in functions if available in your language
Here’s a simple Python solution:
def reverse_string(s):
return s[::-1]
# Or a more manual approach:
def reverse_string_manual(s):
chars = list(s)
left, right = 0, len(chars) - 1
while left < right:
chars[left], chars[right] = chars[right], chars[left]
left += 1
right -= 1
return ''.join(chars)
2. Finding the Maximum Number in an Array
Problem: Write a function to find the maximum number in an array.
Approach:
- Initialize a variable to store the maximum value
- Iterate through the array, updating the maximum if a larger number is found
- Return the maximum value
Here’s a Python solution:
def find_max(arr):
if not arr:
return None
max_num = arr[0]
for num in arr:
if num > max_num:
max_num = num
return max_num
3. Checking if a Number is Prime
Problem: Write a function to check if a given number is prime.
Approach:
- Remember that 1 is not a prime number
- Check for divisibility up to the square root of the number
- Use early return if any divisor is found
Here’s a Python solution:
import math
def is_prime(n):
if n < 2:
return False
for i in range(2, int(math.sqrt(n)) + 1):
if n % i == 0:
return False
return True
The Role of Persistence and Growth Mindset
As you work on improving your coding skills, it’s important to maintain a growth mindset. Remember that:
- Everyone struggles with coding problems at times, even experienced developers
- Each problem you face is an opportunity to learn and grow
- Persistence is key – don’t give up when faced with challenges
- It’s okay to make mistakes; they’re a natural part of the learning process
Cultivating a growth mindset can help you stay motivated and resilient in the face of coding challenges.
Leveraging AI-Powered Tools for Learning
In today’s tech landscape, AI-powered tools can be valuable allies in your coding journey. Platforms like AlgoCademy offer AI-assisted learning experiences that can help you:
- Get personalized feedback on your code
- Receive step-by-step guidance for solving problems
- Practice with dynamically generated coding challenges
- Track your progress and identify areas for improvement
While these tools can be incredibly helpful, remember that they should supplement, not replace, your own problem-solving efforts. Use them wisely to enhance your learning experience.
Conclusion: Embracing the Journey
Getting stuck on simple coding problems is a common experience for programmers at all levels. It’s not a reflection of your abilities or potential, but rather a natural part of the learning process. By strengthening your foundation, practicing regularly, improving your problem-solving skills, and leveraging available resources, you can overcome these challenges and continue to grow as a programmer.
Remember, every programmer you admire was once a beginner who struggled with simple problems. What sets successful programmers apart is their persistence, willingness to learn, and ability to embrace challenges as opportunities for growth.
So the next time you find yourself stuck on a coding problem, take a deep breath, break down the problem, and approach it step by step. With time and practice, you’ll find that those “simple” problems become increasingly manageable, and you’ll be ready to tackle even more complex challenges.
Happy coding, and remember – every line of code you write is a step forward in your programming journey!