Why Debugging Takes Longer Than Writing the Actual Code
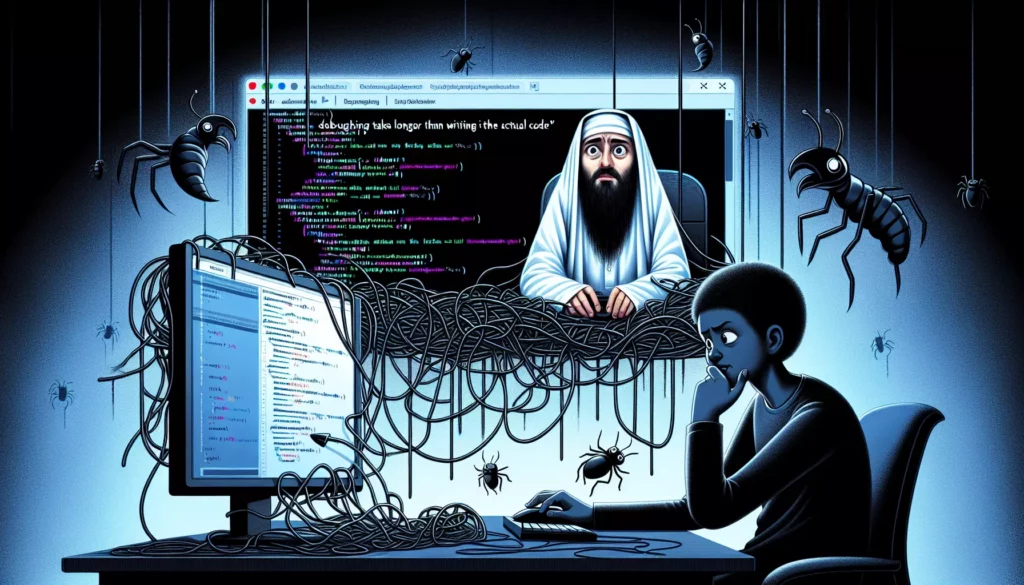
Every programmer has experienced that moment: you’ve spent hours, perhaps days, coding a feature that should have taken a fraction of that time. The culprit? Debugging. It’s a universal truth in software development that debugging often consumes more time than writing the initial code.
As an instructor at AlgoCademy, I’ve watched countless students breeze through writing algorithms only to get stuck for hours tracking down a single misplaced character or logical flaw. This phenomenon isn’t just frustrating; it’s an essential part of the programming experience that deserves deeper exploration.
The Debugging Reality: By the Numbers
Research consistently shows that debugging occupies a disproportionate amount of development time:
- According to studies, developers spend 30-50% of their time debugging code
- For complex systems, debugging can consume up to 75% of the development lifecycle
- The cost of fixing bugs increases exponentially the later they’re discovered in the development process
These statistics aren’t just academic; they reflect the daily reality of programmers from novices to experts. But why exactly does hunting down bugs take so much longer than writing code in the first place?
The Asymmetry Between Writing and Debugging
Writing code and debugging code engage fundamentally different cognitive processes and face different challenges:
1. Direction of Reasoning
When writing code, you engage in forward reasoning: you start with a problem and work toward a solution. This is a natural thought process that humans are generally good at.
Debugging, however, requires backward reasoning: you start with symptoms (errors or unexpected behavior) and work backward to find the cause. This reverse detective work is inherently more difficult for human cognition.
2. State Complexity
When writing code, you’re typically focused on a specific component or feature. Debugging often requires understanding the entire program state, including:
- Variable values across different scopes
- Call stack history
- Interaction between multiple components
- Side effects that may be distant from the code you’re examining
Consider this seemingly simple JavaScript function:
function processUserData(users) {
const processedUsers = [];
for (let i = 0; i < users.length; i++) {
const user = users[i];
if (user.status === "active") {
const processedUser = {
id: user.id,
name: user.name,
score: calculateScore(user)
};
processedUsers.push(processedUser);
}
}
return processedUsers;
}
function calculateScore(user) {
let score = user.baseScore || 0;
if (user.completedTasks) {
for (let i = 0; i < user.completedTasks.length; i++) {
score += user.completedTasks[i].points;
}
}
return score;
}
If this code produces incorrect scores, debugging might require tracing through multiple objects, checking the structure of each user, verifying the completedTasks array, and ensuring the points are being properly accumulated.
3. Visibility Issues
Writing code is an active, constructive process where you can see everything you’re creating. Debugging often involves invisible elements:
- Trying to visualize execution paths not explicitly visible in the code
- Working with memory states that aren’t directly observable
- Understanding timing issues in asynchronous operations
The Psychological Factors
Beyond the technical challenges, several psychological factors make debugging particularly time-consuming:
1. The Curse of Knowledge
When you write code, you have certain assumptions and mental models about how it should work. These same assumptions can blind you to bugs because you literally can’t see the code the way a fresh observer would.
This is why the simple act of explaining your code to someone else (or even to a rubber duck) often leads to discovering bugs. The process forces you to articulate your assumptions and examine them from a different perspective.
2. Emotional Attachment
Programmers often develop an emotional attachment to their code. This makes it harder to:
- Accept that there might be fundamental flaws in your approach
- Consider radical alternatives when stuck in debugging loops
- Recognize when to abandon a particular implementation and start fresh
3. Debugging Fatigue
The longer you spend debugging, the less effective you become. Cognitive resources deplete, leading to:
- Tunnel vision where you keep checking the same code paths
- Reduced ability to make connections between distant parts of the code
- Increased frustration that further impairs problem-solving abilities
At AlgoCademy, we’ve observed that students who take breaks during difficult debugging sessions often return with fresh insights that lead to quick resolution of problems that seemed insurmountable before.
Common Debugging Time Sinks
Certain types of bugs consistently consume disproportionate amounts of debugging time:
1. Heisenberg Bugs
These bugs seem to disappear or change behavior when you try to observe or debug them. They’re often related to:
- Race conditions in concurrent code
- Timing-dependent issues
- Memory corruption that’s affected by debugging tools
Consider this classic example of a Heisenberg bug in C:
// This might work in debug mode but fail in release mode
char *str = malloc(10);
strcpy(str, "Hello");
free(str);
// The bug: using str after freeing it
printf("%s\n", str);
In debug builds, the memory might remain accessible after being freed, masking the bug. In release builds, the same code might crash or produce garbage output.
2. Integration Bugs
These occur at the boundaries between components, especially when they’re developed by different people or teams. They’re time-consuming because:
- They require understanding multiple components in depth
- They often involve mismatched assumptions between components
- Responsibility for fixing them may be unclear
3. Environmental Bugs
These bugs only appear in specific environments:
- “Works on my machine” scenarios
- Production-only issues that can’t be reproduced in development
- Platform-specific bugs (different browsers, operating systems, etc.)
Environmental bugs are especially time-consuming because they often require setting up complex environments just to reproduce them.
4. Silent Logic Errors
Unlike syntax errors that prevent code from running, logic errors produce no error messages. The code runs, but produces incorrect results. These are particularly insidious because:
- There’s no clear starting point for debugging
- The effects might be subtle and noticed only in specific circumstances
- The cause might be far removed from where the symptoms appear
Take this Python example:
def calculate_average(numbers):
total = 0
for num in numbers:
total += num
return total / len(numbers)
# Silent error: What if numbers is an empty list?
# This will throw a division by zero error
This function works perfectly until it encounters an edge case, at which point it fails silently (from a logical perspective) but catastrophically from an execution perspective.
The Complexity Multiplier
As systems grow in complexity, debugging time doesn’t increase linearly—it increases exponentially. This happens because:
1. Interaction Explosion
In a system with n components, there are potentially n² interactions between components. Each interaction is a potential source of bugs.
When debugging complex systems, you’re often dealing with emergent behavior that arises from these interactions rather than from any single component.
2. State Space Expansion
Modern applications maintain complex state that can exist in countless configurations. Bugs might only appear in specific state combinations that are difficult to reproduce.
Consider a typical React application with multiple interconnected components. A bug might only appear when:
- The user has performed actions in a specific sequence
- Certain components have updated while others haven’t
- The application is in a particular routing state
- External data has loaded in a specific pattern
The possible combinations are virtually infinite, making some bugs extremely elusive.
3. Technical Debt Accumulation
As codebases age, they accumulate technical debt that makes debugging progressively harder:
- Outdated documentation that misleads developers
- Workarounds built on top of workarounds
- Legacy code that no current team member fully understands
Each layer of technical debt acts as a multiplier on debugging time.
Case Study: The One-Line Fix That Took Two Days
At AlgoCademy, we recently encountered a particularly illustrative debugging scenario. A student was working on a binary search tree implementation and encountered a bug where the tree would occasionally lose nodes after a series of insertions and deletions.
The fix, when eventually found, was adding a single line of code to update a parent reference. However, finding that fix took nearly two days of intensive debugging. Why?
- Intermittent nature: The bug only appeared with certain sequences of operations
- Delayed symptoms: The error in the tree structure didn’t cause immediate problems, only becoming apparent much later
- Visualization difficulty: Tree structures are hard to visualize mentally, especially when they become unbalanced
- Assumption errors: The student had incorrectly assumed a certain invariant was being maintained
The final fix:
void deleteNode(Node* node) {
// Case 1: Node has no children
if (!node->left && !node->right) {
if (node->parent) {
if (node->parent->left == node) {
node->parent->left = NULL;
} else {
node->parent->right = NULL;
}
}
free(node);
return;
}
// Case 2: Node has one child
if (!node->left || !node->right) {
Node* child = node->left ? node->left : node->right;
if (node->parent) {
if (node->parent->left == node) {
node->parent->left = child;
} else {
node->parent->right = child;
}
}
child->parent = node->parent; // This line was missing
free(node);
return;
}
// Case 3: Node has two children
// [implementation omitted for brevity]
}
This example perfectly illustrates how a simple oversight can lead to disproportionate debugging time. The missing line was conceptually simple, but finding it required understanding the entire tree structure and operation sequence.
Strategies to Reduce Debugging Time
While debugging will always be time-consuming, several strategies can help reduce its impact:
1. Prevention Is Better Than Cure
The most effective way to reduce debugging time is to prevent bugs in the first place:
- Test-Driven Development (TDD): Writing tests before code helps clarify requirements and catch bugs early
- Code Reviews: Having others examine your code brings fresh perspectives that can identify potential issues
- Static Analysis Tools: Tools like ESLint, TypeScript, or PyLint can catch many bugs before code even runs
2. Systematic Debugging Approaches
Rather than random trial and error, systematic approaches save time:
- Binary Search Debugging: Systematically narrowing down the location of a bug by testing at midpoints
- Scientific Method: Forming hypotheses about the bug’s cause and designing experiments to test them
- Rubber Duck Debugging: Explaining the code line by line to force a methodical review
3. Improving Visibility
Many debugging challenges stem from poor visibility into code execution:
- Comprehensive Logging: Strategic log statements can provide insights into program flow
- Debugging Tools: Proficient use of debuggers with breakpoints, watch expressions, and call stack analysis
- Visualization Tools: Using tools to visualize data structures, state changes, and program flow
At AlgoCademy, we teach students to use visualization tools like recursion trees and state diagrams to make complex algorithms more tractable:
// Instead of this:
function fibonacci(n) {
if (n <= 1) return n;
return fibonacci(n-1) + fibonacci(n-2);
}
// Consider a version with logging to understand the execution path:
function fibonacci(n, depth = 0) {
const indent = ' '.repeat(depth * 2);
console.log(`${indent}Calculating fibonacci(${n})`);
if (n <= 1) {
console.log(`${indent}Base case: returning ${n}`);
return n;
}
const result1 = fibonacci(n-1, depth + 1);
const result2 = fibonacci(n-2, depth + 1);
const sum = result1 + result2;
console.log(`${indent}fibonacci(${n}) = ${result1} + ${result2} = ${sum}`);
return sum;
}
This instrumented version makes the recursive calls visible, helping identify inefficiencies or logical errors.
4. Building Debugging Skills
Debugging is a skill that improves with deliberate practice:
- Study Common Bug Patterns: Familiarize yourself with frequent error types in your language or domain
- Post-Mortem Analysis: After fixing a bug, analyze how it occurred and how you could prevent similar issues
- Pair Debugging: Working with another developer can bring complementary perspectives and skills
The Role of Modern Tools and Practices
The software development landscape continues to evolve with tools and practices that address debugging challenges:
1. Advanced Debugging Tools
Modern tools go beyond traditional debugging:
- Time-Travel Debugging: Tools like rr for C/C++ or Replay for JavaScript allow stepping backward through execution
- Omniscient Debugging: Recording the entire program state at each step for comprehensive analysis
- AI-Assisted Debugging: Emerging tools that use machine learning to suggest likely bug locations or fixes
2. Architectural Approaches
Certain architectural patterns make systems inherently easier to debug:
- Immutable Data Structures: Eliminating side effects reduces the complexity of state tracking
- Pure Functions: Functions without side effects are easier to test and debug in isolation
- Event Sourcing: Recording all state changes as events allows for detailed reconstruction of bugs
Consider this React example using immutable state updates:
// Hard to debug: mutating state directly
function addTodo(todos, newTodo) {
todos.push(newTodo); // Mutation!
return todos;
}
// Easier to debug: immutable updates
function addTodo(todos, newTodo) {
return [...todos, newTodo]; // Creates new array
}
The immutable version makes state changes explicit and traceable, simplifying debugging significantly.
3. Observability Practices
Modern systems increasingly incorporate comprehensive observability:
- Distributed Tracing: Following requests across service boundaries
- Metrics and Alerting: Identifying anomalies before they become critical issues
- Structured Logging: Making logs machine-parseable for better analysis
Learning from Debugging: The Silver Lining
While debugging is time-consuming, it’s also one of the most valuable learning opportunities in programming:
1. System Understanding
Debugging forces you to develop a deeper understanding of:
- How components interact within your system
- The actual (rather than intended) behavior of libraries and frameworks
- Runtime environments and their quirks
2. Problem-Solving Skills
The detective work of debugging builds broadly applicable skills:
- Systematic hypothesis testing
- Breaking complex problems into manageable pieces
- Working under constraints and with incomplete information
3. Resilience and Persistence
Perhaps most importantly, debugging builds psychological resilience:
- The ability to persist through frustration
- Comfort with uncertainty and ambiguity
- The satisfaction of solving difficult puzzles
At AlgoCademy, we emphasize that debugging frustrations are not just obstacles but opportunities for growth. The student who spent two days finding a one-line fix developed debugging skills that will serve them throughout their career.
Conclusion: Embracing the Debugging Reality
The fact that debugging takes longer than writing code isn’t a failure of programming or programmers; it’s an inherent aspect of the complex systems we build. Understanding why this happens helps us:
- Set realistic expectations for development timelines
- Allocate appropriate resources to testing and quality assurance
- Invest in tools and practices that make debugging more efficient
- Approach debugging as a valuable skill rather than a necessary evil
As software continues to eat the world, the ability to efficiently debug complex systems becomes increasingly valuable. The best developers aren’t necessarily those who write code the fastest, but those who can effectively navigate the inevitable debugging process.
The next time you find yourself hours into debugging what seemed like a simple feature, remember: this isn’t an exception to the programming experience; it is the programming experience. And each debugging session makes you a more effective problem solver for the challenges yet to come.
Practical Debugging Exercise
To put these concepts into practice, try this debugging challenge that we use at AlgoCademy:
function findMedian(arr1, arr2) {
// Merge two sorted arrays
const merged = [];
let i = 0, j = 0;
while (i < arr1.length && j < arr2.length) {
if (arr1[i] < arr2[j]) {
merged.push(arr1[i]);
i++;
} else {
merged.push(arr2[j]);
j++;
}
}
// Add remaining elements
while (i < arr1.length) {
merged.push(arr1[i]);
i++;
}
while (j < arr2.length) {
merged.push(arr2[j]);
j++;
}
// Find median
const mid = Math.floor(merged.length / 2);
if (merged.length % 2 === 0) {
return (merged[mid - 1] + merged[mid]) / 2;
} else {
return merged[mid];
}
}
This function is supposed to find the median of two sorted arrays. It contains a subtle bug that only appears in certain input combinations. Can you find and fix it?
Remember to apply the systematic debugging approaches discussed in this article rather than random trial and error. Good luck!