Why Debugging is Actually a Form of Time Travel
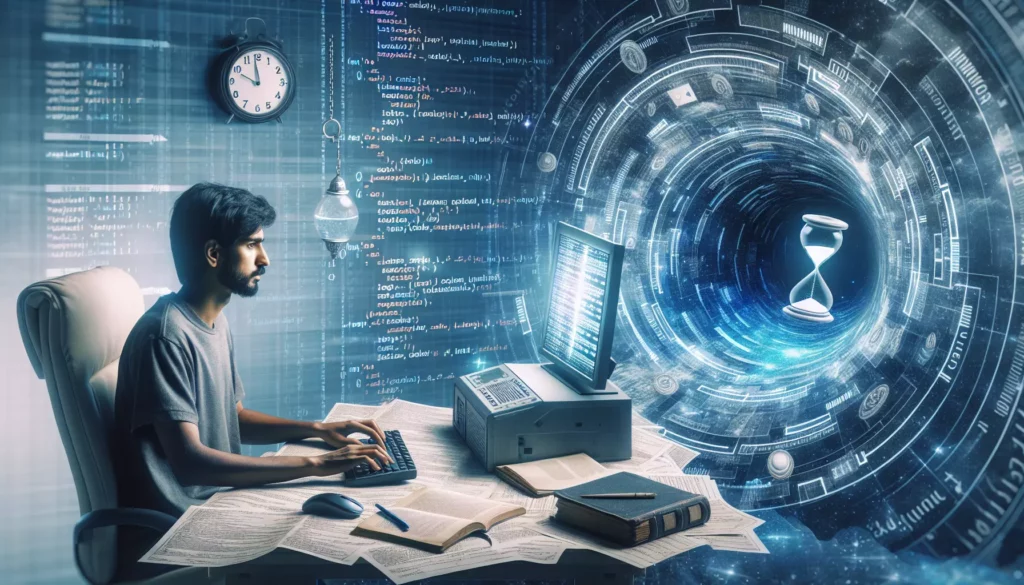
As software developers, we often find ourselves immersed in the intricate world of coding, where lines of instructions come together to create complex systems. Among the various skills we cultivate, debugging stands out as both an art and a science. But have you ever considered that when you’re debugging, you’re actually engaging in a form of time travel? Let’s embark on a journey through the space-time continuum of code to explore this fascinating perspective.
The Time Machine of Code
Imagine your codebase as a timeline. Each line of code represents a moment in your program’s execution, a snapshot of its state at that particular instant. When you debug, you’re essentially navigating through this timeline, moving back and forth between different states of your program. This ability to traverse the execution flow of your code is remarkably similar to the concept of time travel in science fiction.
Breakpoints: Your Time Travel Markers
In the world of debugging, breakpoints are your trusty time machines. By setting a breakpoint, you’re creating a temporal anchor point in your code’s timeline. When you run your program in debug mode, it will pause at these designated spots, allowing you to inspect the state of your variables, the call stack, and other crucial information at that precise moment in time.
def calculate_fibonacci(n):
if n <= 1:
return n
else:
return calculate_fibonacci(n-1) + calculate_fibonacci(n-2)
# Set a breakpoint on the next line
result = calculate_fibonacci(5)
print(result)
In this example, setting a breakpoint on the line where we call calculate_fibonacci(5)
allows us to pause time just before the function is executed. From there, we can step into the function, watching how the values change with each recursive call, effectively traveling through the timeline of the Fibonacci sequence calculation.
Step-by-Step Execution: Walking Through Time
Once you’ve paused at a breakpoint, most debuggers offer the ability to step through your code line by line. This is akin to moving forward in time in slow motion, observing how each instruction affects the state of your program. It’s like having a time machine with precise controls, allowing you to advance moment by moment through your code’s timeline.
The Butterfly Effect in Debugging
In time travel narratives, there’s often a focus on the butterfly effect – the idea that small changes in the past can lead to significant alterations in the future. Debugging exhibits a similar phenomenon. A single misplaced character or an off-by-one error can cascade into large-scale issues in your program’s behavior.
def process_data(data):
for i in range(len(data)):
# Bug: should be data[i], not data[i-1]
if data[i-1] < 0:
data[i-1] = 0
return data
# This will cause an IndexError for the first element
result = process_data([1, -2, 3, -4, 5])
print(result)
In this example, a simple indexing error (data[i-1]
instead of data[i]
) creates a bug that only manifests when processing the first element of the list. By using our debugging time machine, we can travel to the exact moment this error occurs, observe its impact, and make the necessary correction to set the timeline right.
Reversing the Flow of Time
One of the most powerful features of modern debuggers is the ability to step backwards through code execution. This is perhaps the closest we can get to actual time travel in the programming world. By recording the program’s state at each step, debuggers allow us to rewind the execution, undoing the effects of each instruction.
This capability is particularly useful when dealing with complex bugs that only manifest after a long series of operations. Instead of repeatedly running the program from the start, you can simply rewind to the point just before the bug occurs, saving valuable debugging time.
Time Travel Debugging in Action
Let’s consider a more complex scenario where time travel debugging shines:
class BankAccount:
def __init__(self, balance=0):
self.balance = balance
def deposit(self, amount):
self.balance += amount
def withdraw(self, amount):
if self.balance >= amount:
self.balance -= amount
else:
raise ValueError("Insufficient funds")
def process_transactions(account, transactions):
for transaction in transactions:
if transaction[0] == 'D':
account.deposit(int(transaction[1:]))
elif transaction[0] == 'W':
account.withdraw(int(transaction[1:]))
return account.balance
# Bug: The final balance is incorrect
account = BankAccount(1000)
transactions = ['D500', 'W200', 'D300', 'W400', 'W100', 'D50']
final_balance = process_transactions(account, transactions)
print(f"Final balance: {final_balance}") # Expected: 1150, Actual: ???
In this scenario, we have a simple bank account system processing a series of deposits and withdrawals. Let’s say the final balance is incorrect, but it’s not immediately clear why. This is where time travel debugging becomes invaluable.
Using a debugger with reverse execution capabilities, we can:
- Set a breakpoint at the end of the
process_transactions
function. - Run the program until it hits this breakpoint.
- Step backwards through each transaction, observing how the balance changes.
- Identify the exact point where the balance deviates from our expected calculations.
- Investigate the state of the variables at that crucial moment to uncover the root cause of the bug.
This approach allows us to efficiently pinpoint the source of the error without the need for multiple debug sessions or extensive logging.
Parallel Universes: Conditional Breakpoints and Watch Variables
In the realm of time travel, the concept of parallel universes often comes into play. In debugging, we have our own version of parallel universes through conditional breakpoints and watch variables.
Conditional Breakpoints: Traveling to Specific Realities
Conditional breakpoints allow us to pause the execution only when certain conditions are met. This is like traveling to a specific parallel universe where a particular set of circumstances align. For instance, in our bank account example, we might set a conditional breakpoint that triggers only when the balance goes negative:
# Pseudo-code for a conditional breakpoint
if account.balance < 0:
break # This line would be set as a conditional breakpoint
This allows us to instantly travel to the moment when our account balance becomes invalid, helping us quickly identify and fix the issue.
Watch Variables: Observing Alternate Timelines
Watch variables act as windows into parallel timelines of our code’s execution. By setting watch expressions, we can observe how certain variables or expressions change over time without explicitly adding breakpoints at each step.
# Example watch expressions for our bank account scenario
account.balance
sum(int(t[1:]) for t in transactions if t[0] == 'D') - sum(int(t[1:]) for t in transactions if t[0] == 'W')
These watch expressions allow us to compare the actual balance with the expected balance calculated from the transactions, helping us spot discrepancies as they occur.
The Paradoxes of Debugging
Just as time travel in fiction often leads to paradoxes, debugging can sometimes introduce its own set of paradoxical situations. These are moments where the act of observing a bug changes its behavior or even makes it disappear entirely.
The Observer Effect
In quantum physics, the observer effect refers to changes that the act of observation makes on a phenomenon. In debugging, we encounter a similar effect when bugs behave differently or disappear when we’re actively debugging them. This often happens with timing-related issues or race conditions in multi-threaded applications.
import threading
import time
shared_resource = 0
def increment_resource():
global shared_resource
local_copy = shared_resource
time.sleep(0.1) # Simulate some processing time
shared_resource = local_copy + 1
threads = []
for _ in range(10):
thread = threading.Thread(target=increment_resource)
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
print(f"Final value: {shared_resource}") # Expected: 10, Actual: ???
In this example, we have a classic race condition. The bug might be evident when running the program normally, but as soon as we start debugging and stepping through the code, the timing changes, potentially masking the issue. This is where techniques like logging and analyzing the program’s behavior in its natural state become crucial.
The Heisenbug
Named after the Heisenberg Uncertainty Principle, a Heisenbug is a software bug that seems to disappear or alter its behavior when you attempt to study it. This type of bug is particularly challenging because the very tools we use to observe it can affect its manifestation.
Heisenbugs often occur in scenarios involving:
- Memory corruption
- Uninitialized variables
- Timing-dependent code
- Compiler optimizations
Dealing with Heisenbugs requires a different approach to debugging. Instead of relying solely on interactive debugging, we might need to employ techniques such as:
- Extensive logging
- Memory analysis tools
- Stress testing and repeated execution
- Code review and static analysis
The Ripple Effect: Fixing Bugs Across Time
When we fix a bug, we’re not just correcting a mistake in the present; we’re altering the future timeline of our software. This ripple effect can have far-reaching consequences, both positive and potentially negative.
Regression Testing: Ensuring a Stable Timeline
After fixing a bug, it’s crucial to perform regression testing to ensure that our changes haven’t inadvertently introduced new issues or revived old ones. This is akin to checking that our time travel adventures haven’t created any unintended alterations in the timeline.
import unittest
class TestBankAccount(unittest.TestCase):
def setUp(self):
self.account = BankAccount(1000)
def test_deposit(self):
self.account.deposit(500)
self.assertEqual(self.account.balance, 1500)
def test_withdraw(self):
self.account.withdraw(300)
self.assertEqual(self.account.balance, 700)
def test_insufficient_funds(self):
with self.assertRaises(ValueError):
self.account.withdraw(1500)
def test_process_transactions(self):
transactions = ['D500', 'W200', 'D300', 'W400', 'W100', 'D50']
final_balance = process_transactions(self.account, transactions)
self.assertEqual(final_balance, 1150)
if __name__ == '__main__':
unittest.main()
By maintaining a comprehensive suite of unit tests, we can quickly verify that our bug fix hasn’t disrupted the existing functionality. This helps ensure that our journey through the debugging time continuum leaves the codebase in a better state than we found it.
The Time Traveler’s Toolkit: Essential Debugging Skills
To truly master the art of debugging as time travel, one must cultivate a set of essential skills. These skills will enable you to navigate the complex timelines of your code with confidence and precision.
1. Analytical Thinking
Developing strong analytical skills is crucial for effective debugging. This involves:
- Breaking down complex problems into smaller, manageable parts
- Identifying patterns and correlations in program behavior
- Forming and testing hypotheses about the root causes of bugs
2. Patience and Persistence
Debugging can often be a time-consuming process, especially when dealing with elusive bugs. Cultivating patience and persistence is key to:p>
- Methodically working through different scenarios
- Resisting the urge to make hasty assumptions
- Maintaining focus during long debugging sessions
3. Attention to Detail
In the world of coding, a single misplaced character can lead to significant issues. Honing your attention to detail helps in:
- Spotting subtle inconsistencies in code
- Recognizing patterns in error messages and stack traces
- Identifying edge cases that might be causing bugs
4. System-Level Understanding
While it’s important to focus on the specific area where a bug manifests, having a broader understanding of the system can be invaluable. This includes:
- Comprehending how different components of the system interact
- Understanding the flow of data through the application
- Recognizing the potential impact of external factors (e.g., network, database)
5. Familiarity with Debugging Tools
Mastering your debugging tools is like fine-tuning your time machine. This involves:
- Proficiency in using breakpoints, including conditional breakpoints
- Skill in navigating through code using step-in, step-over, and step-out commands
- Ability to effectively use watch variables and expressions
- Understanding how to analyze memory usage and performance metrics
6. Documentation and Communication
Effective debugging often requires clear documentation and communication, especially in team environments. This includes:
- Clearly documenting the steps to reproduce a bug
- Maintaining detailed notes during the debugging process
- Communicating findings and solutions effectively to team members
Conclusion: Embracing the Time Traveler’s Mindset
As we’ve explored throughout this article, debugging is indeed a form of time travel within the realm of code. By adopting this perspective, we can approach debugging not just as a necessary task, but as an exciting journey through the timeline of our software.
Remember, every time you set a breakpoint, step through code, or analyze a stack trace, you’re navigating the intricate web of your program’s execution history. You’re not just fixing bugs; you’re reshaping the past to create a better future for your code.
Embrace this time traveler’s mindset, and you’ll find that debugging becomes more than just a troubleshooting process. It becomes an opportunity to gain deeper insights into your code, to understand the butterfly effects of small changes, and to craft more robust and reliable software.
So the next time you find yourself deep in a debugging session, take a moment to appreciate the incredible power at your fingertips. You’re not just a developer; you’re a time traveler, exploring the vast and intricate timeline of your code, ensuring that each moment in its execution unfolds exactly as it should.
Happy debugging, and may your journeys through the code-time continuum be fruitful and enlightening!