Why Copying “Clean Code” Patterns Isn’t Improving Your Software Design
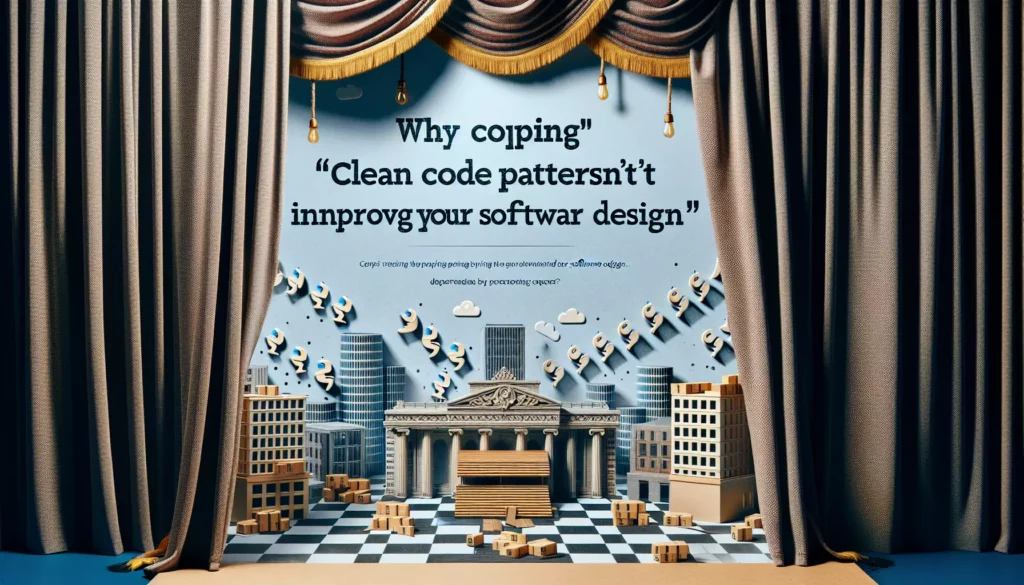
Every programmer has been there: you read a popular book on clean code, watch a conference talk about software design patterns, or study the architecture of a well-regarded open source project. Inspired, you eagerly apply these patterns to your own codebase, expecting dramatic improvements. Yet weeks later, your code feels just as unwieldy as before, perhaps even more complex.
Why don’t these seemingly universal “clean code” practices consistently lead to better software?
In this article, we’ll explore why blindly following clean code principles often fails to improve software design, and what you should be doing instead to create truly maintainable, robust software systems.
The Allure of Clean Code Patterns
The software development world loves patterns. From the Gang of Four design patterns to Uncle Bob’s SOLID principles to modern microservice architectures, we’re constantly searching for reusable templates that promise to make our software better.
These patterns appeal to us for good reasons:
- They provide mental shortcuts for complex design decisions
- They create a shared vocabulary among developers
- They feel like objective measures of code quality
- They promise consistency across codebases
When you’re faced with a messy codebase or a complex feature request, it’s comforting to reach for an established pattern. It feels like standing on the shoulders of giants—leveraging the collective wisdom of the software development community.
The Problem: Context Matters More Than Patterns
The fundamental issue with copying clean code patterns is that they’re abstractions removed from their original context. When we apply them in new situations without understanding why they worked in their original context, we risk creating more problems than we solve.
Consider these common clean code recommendations:
- “Functions should do one thing”
- “Don’t repeat yourself (DRY)”
- “Classes should be small”
- “Use dependency injection”
- “Prefer composition over inheritance”
These sound reasonable in isolation, but their blind application leads to unexpected consequences.
Example: The DRY Principle Gone Wrong
Let’s examine a common scenario. You notice two similar blocks of code in different parts of your application:
// In the user registration service
function validateEmail(email) {
const emailRegex = /^[a-zA-Z0-9._-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,6}$/;
return emailRegex.test(email);
}
// In the newsletter subscription service
function checkEmailFormat(email) {
const emailRegex = /^[a-zA-Z0-9._-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,6}$/;
return emailRegex.test(email);
}
Dutifully following the DRY principle, you extract this to a shared utility:
// In shared/validation.js
export function validateEmail(email) {
const emailRegex = /^[a-zA-Z0-9._-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,6}$/;
return emailRegex.test(email);
}
Six months later, the marketing team wants to accept more email formats for newsletters, while user registration needs stricter validation. Now your “clean” abstraction becomes a problem—these two contexts had different needs all along, and your premature abstraction created coupling where none was necessary.
The SOLID Principles: Often Misapplied
The SOLID principles (Single Responsibility, Open/Closed, Liskov Substitution, Interface Segregation, and Dependency Inversion) represent some of the most widely taught “clean code” concepts. Yet their application often leads to overengineered code.
Consider the Single Responsibility Principle (SRP). It states that a class should have only one reason to change. This sounds sensible, but it frequently leads to excessive fragmentation—tiny classes that do almost nothing, resulting in a system where understanding the big picture becomes nearly impossible.
In practice, real-world concerns don’t neatly separate into “single responsibilities.” A user registration system might handle validation, persistence, notification, and security concerns. Artificially splitting these into separate classes often creates more complexity through indirection than it solves through separation.
The Cost of Premature Abstraction
When we copy clean code patterns without understanding their context, we often create what I call “premature abstractions”—code structures that add complexity without solving actual problems.
These premature abstractions exact a heavy toll:
1. Increased Cognitive Load
Every abstraction requires mental effort to understand. When abstractions don’t align with the problem domain, they create additional cognitive load without corresponding benefits.
Consider this example of an overly abstracted “clean” design:
class UserFactory {
createUser(userData) {
return new User(userData);
}
}
class UserValidator {
validate(userData) {
// Validation logic
}
}
class UserRepository {
save(user) {
// Persistence logic
}
}
class UserService {
constructor(userFactory, userValidator, userRepository) {
this.userFactory = userFactory;
this.userValidator = userValidator;
this.userRepository = userRepository;
}
registerUser(userData) {
if (this.userValidator.validate(userData)) {
const user = this.userFactory.createUser(userData);
this.userRepository.save(user);
return user;
}
throw new ValidationError();
}
}
Compare that to a more straightforward approach:
class UserRegistration {
registerUser(userData) {
if (!this.isValid(userData)) {
throw new ValidationError();
}
const user = new User(userData);
this.saveToDatabase(user);
return user;
}
isValid(userData) {
// Validation logic
}
saveToDatabase(user) {
// Persistence logic
}
}
The first example follows common “clean code” patterns, but requires tracking multiple classes and their relationships. The second is more direct and often easier to understand and modify for most real-world scenarios.
2. Indirection and Navigation Overhead
Excessive abstraction creates what’s often called the “arrow anti-pattern”—where following program logic requires jumping between multiple files and classes. This makes debugging and code comprehension significantly harder.
A developer trying to understand how user registration works in our overabstracted example would need to navigate through multiple files, tracking the flow across class boundaries.
3. Flexibility in the Wrong Places
Clean code patterns often add flexibility where it’s not needed. For instance, dependency injection is frequently overused to make everything “testable,” resulting in complex configuration that rarely changes in practice.
Consider this typical example:
// Excessive flexibility
class OrderProcessor {
constructor(
productRepository,
inventoryService,
pricingCalculator,
taxService,
discountStrategy,
paymentGateway,
orderRepository,
notificationService
) {
this.productRepository = productRepository;
this.inventoryService = inventoryService;
this.pricingCalculator = pricingCalculator;
this.taxService = taxService;
this.discountStrategy = discountStrategy;
this.paymentGateway = paymentGateway;
this.orderRepository = orderRepository;
this.notificationService = notificationService;
}
// Process order method
}
In real systems, most of these dependencies will never be swapped out, making this flexibility an unnecessary burden.
The Real Problem: Misunderstanding the Purpose of Design Patterns
The core issue isn’t that design patterns and clean code principles are wrong—it’s that we misunderstand their purpose.
Design patterns aren’t recipes to be followed blindly. They’re tools for communicating common solutions to recurring problems. Their value isn’t in the implementation details but in the shared vocabulary they create.
When the Gang of Four introduced design patterns, they explicitly warned against using them prematurely. Each pattern addresses specific forces and constraints. Without those constraints, the pattern becomes an unnecessary complication.
A Better Approach: Context-Aware Design
Instead of copying clean code patterns, we need a more nuanced approach to software design—one that considers the specific context of our system.
1. Understand the Problem Domain First
Before applying any design pattern or principle, deeply understand the problem you’re solving. This means:
- Identifying the core concepts in your domain
- Understanding how these concepts relate to each other
- Recognizing which aspects are likely to change and which will remain stable
Domain-Driven Design (DDD) offers a rich vocabulary for this process, focusing on creating a “ubiquitous language” that bridges technical implementation and business concepts.
2. Start Simple, Then Evolve
Rather than beginning with complex abstractions, start with the simplest solution that could possibly work. Then let the design evolve as you encounter real, not imagined, problems.
This approach, sometimes called “incremental design” or “emergent design,” aligns with the agile principle of simplicity: “Maximize the amount of work not done.”
For example, instead of creating a complex inheritance hierarchy for different user types upfront, start with a simple User class and extract abstractions only when concrete requirements demonstrate their necessity.
3. Refactor Based on Actual Pain Points
Wait for code smells to emerge in your actual codebase before applying remedies. Common pain points that justify refactoring include:
- Genuine duplication that causes maintenance headaches
- Changes that consistently affect multiple parts of the system
- Code that’s difficult to test due to tight coupling
- Sections that developers consistently misunderstand or modify incorrectly
When these issues arise, then reach for appropriate design principles and patterns as solutions to concrete problems.
4. Consider the Full System Context
Software design doesn’t happen in a vacuum. Consider factors beyond the code itself:
- Team size and experience level
- Project lifespan and maintenance expectations
- Performance and scalability requirements
- Deployment and operational constraints
A complex microservice architecture might be appropriate for a large team building a long-lived system with strict scalability requirements. For a smaller team or shorter-lived project, such complexity could be detrimental.
Case Study: Evolving Design in a Real Project
Let’s examine how context-aware design might evolve in a real-world scenario.
Imagine you’re building a coding education platform (like AlgoCademy) that helps users learn programming through interactive exercises. Initially, you start with a simple structure for coding challenges:
class CodingChallenge {
constructor(title, description, startingCode, testCases) {
this.title = title;
this.description = description;
this.startingCode = startingCode;
this.testCases = testCases;
}
evaluate(userCode) {
// Run the user's code against test cases
const results = this.testCases.map(testCase => {
try {
// Execute user code with test inputs
const result = executeCode(userCode, testCase.input);
return {
passed: isEqual(result, testCase.expectedOutput),
input: testCase.input,
expected: testCase.expectedOutput,
actual: result
};
} catch (error) {
return {
passed: false,
input: testCase.input,
expected: testCase.expectedOutput,
error: error.message
};
}
});
return {
success: results.every(r => r.passed),
results: results
};
}
}
This simple design works well initially. But as the platform grows, you encounter new requirements:
- Supporting different programming languages
- Adding different types of challenges (multiple choice, fill-in-the-blank, etc.)
- Supporting more sophisticated test cases with custom validators
- Adding hints and solution explanations
A “clean code” enthusiast might immediately jump to creating a complex hierarchy with interfaces like IChallenge
, abstract classes, factories, and strategy patterns. But let’s take an evolutionary approach instead.
Step 1: Handle Multiple Languages
First, we identify that language-specific code execution is a separate concern:
class CodeExecutor {
constructor(language) {
this.language = language;
}
execute(code, input) {
switch(this.language) {
case 'javascript':
return this.executeJavaScript(code, input);
case 'python':
return this.executePython(code, input);
default:
throw new Error(`Unsupported language: ${this.language}`);
}
}
executeJavaScript(code, input) {
// JavaScript execution logic
}
executePython(code, input) {
// Python execution logic
}
}
class CodingChallenge {
constructor(title, description, startingCode, testCases, language) {
this.title = title;
this.description = description;
this.startingCode = startingCode;
this.testCases = testCases;
this.executor = new CodeExecutor(language);
}
evaluate(userCode) {
const results = this.testCases.map(testCase => {
try {
const result = this.executor.execute(userCode, testCase.input);
// Rest of evaluation logic
} catch (error) {
// Error handling
}
});
// Return results
}
}
We’ve extracted the language-specific execution logic without overhauling the entire design.
Step 2: Support Different Challenge Types
As we need to support multiple challenge types, we notice they share some aspects but differ in others. Now inheritance makes sense:
class Challenge {
constructor(title, description) {
this.title = title;
this.description = description;
}
evaluate(userSubmission) {
throw new Error("Subclasses must implement evaluate");
}
}
class CodingChallenge extends Challenge {
constructor(title, description, startingCode, testCases, language) {
super(title, description);
this.startingCode = startingCode;
this.testCases = testCases;
this.executor = new CodeExecutor(language);
}
evaluate(userCode) {
// Coding-specific evaluation
}
}
class MultipleChoiceChallenge extends Challenge {
constructor(title, description, options, correctOptionIndex) {
super(title, description);
this.options = options;
this.correctOptionIndex = correctOptionIndex;
}
evaluate(selectedOptionIndex) {
return {
success: selectedOptionIndex === this.correctOptionIndex,
correctOption: this.options[this.correctOptionIndex]
};
}
}
We introduced inheritance only when we had a clear need for polymorphism—different challenge types with a common interface.
Step 3: Add Custom Validators
As test cases become more complex, we need custom validation beyond simple equality checks:
class TestCase {
constructor(input, expectedOutput, validator = null) {
this.input = input;
this.expectedOutput = expectedOutput;
this.validator = validator || this.defaultValidator;
}
defaultValidator(actual, expected) {
return isEqual(actual, expected);
}
validate(actual) {
return this.validator(actual, this.expectedOutput);
}
}
class CodingChallenge extends Challenge {
// Constructor remains the same
evaluate(userCode) {
const results = this.testCases.map(testCase => {
try {
const result = this.executor.execute(userCode, testCase.input);
return {
passed: testCase.validate(result),
input: testCase.input,
expected: testCase.expectedOutput,
actual: result
};
} catch (error) {
// Error handling
}
});
// Return results
}
}
We encapsulated validation logic within the TestCase class, allowing for custom validators while maintaining a clean interface.
The Result: Practical, Evolving Design
Notice how our design evolved in response to specific needs rather than conforming to predetermined patterns. We added complexity only where necessary, keeping the system understandable at each step.
This approach leads to a design that:
- Addresses actual rather than imagined requirements
- Maintains simplicity where possible
- Introduces abstractions only when their benefit is clear
- Remains flexible in the dimensions that actually change
Beyond Clean Code: Principles for Better Software Design
Rather than focusing solely on clean code patterns, consider these broader principles for effective software design:
1. Design for Understanding, Not Perfection
The primary goal of good design isn’t theoretical purity but enabling humans to understand and modify the system effectively. Prioritize clarity over cleverness.
As Martin Fowler noted: “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.”
2. Embrace the Domain
Your software should reflect the domain it serves. The structure of your code should mirror the structure of the problem it solves.
This means naming classes, methods, and variables using domain terminology and organizing code around domain concepts rather than technical concerns when possible.
3. Optimize for Change
Software design isn’t about creating the perfect structure; it’s about creating a structure that can evolve gracefully as requirements change.
This means:
- Making the most likely changes easy
- Isolating volatile parts of the system
- Maintaining appropriate boundaries between components
4. Value Simplicity
Complexity is the enemy of maintainable software. Each abstraction, pattern, or layer adds cognitive overhead that must be justified by concrete benefits.
As Rich Hickey famously said, we should distinguish between “simple” (not compound, having one focus) and “easy” (familiar, at hand). Prioritize simplicity over ease when designing software.
5. Test Strategically
Tests should guide your design, not constrain it. Don’t add complexity to your production code solely to make it testable. Instead, design both your code and your tests to reflect the actual use cases and constraints of your system.
High-value tests focus on behavior and outcomes rather than implementation details, allowing your design to evolve without breaking tests that encode implementation assumptions.
Learning from Design Principles Without Blindly Copying Them
So how should we approach established design principles and patterns? They remain valuable, but we need to engage with them more thoughtfully:
Understand the Why, Not Just the How
When learning about a design pattern or principle, focus on understanding the problem it was created to solve. Ask:
- What specific issue does this pattern address?
- What forces or constraints does it balance?
- What are the tradeoffs of applying this pattern?
For example, the Factory pattern isn’t just “a class that creates objects.” It’s a solution to the problem of decoupling object creation from object use, particularly valuable when the exact class to instantiate isn’t known at compile time or when creation logic is complex.
Study Anti-patterns Too
Understanding what not to do is as valuable as knowing what to do. Study common anti-patterns and failed designs to recognize problematic structures in your own code.
For instance, understanding the “God Object” anti-pattern (a class that knows or does too much) helps you recognize when your own classes are taking on too many responsibilities.
Learn Multiple Design Philosophies
Different programming paradigms offer different approaches to software design. Exposure to multiple philosophies provides a richer toolkit for addressing diverse problems.
For example:
- Object-oriented design emphasizes encapsulation and polymorphism
- Functional programming focuses on immutability and composition
- Data-oriented design prioritizes efficient data access patterns
- Domain-driven design centers on modeling business concepts
Each approach has strengths for certain types of problems. The best designers know when to apply each philosophy.
Conclusion: From Pattern Copying to Contextual Design
Clean code patterns and principles aren’t inherently problematic—they become problematic when applied without consideration for context. The best software designers don’t blindly follow patterns; they understand patterns deeply and apply them judiciously where appropriate.
As you develop your design skills:
- Start by understanding your specific problem domain
- Begin with simple designs that directly address current needs
- Let abstractions emerge from actual, not anticipated, requirements
- Study patterns and principles to understand the problems they solve
- Apply patterns only when you face the problems they were designed to address
Remember that software design is ultimately about managing complexity in service of human understanding. The best designs aren’t the ones that check the most “clean code” boxes, but those that most effectively empower developers to understand, maintain, and extend the system over time.
By moving beyond pattern-copying to context-aware design, you’ll create software that’s not just theoretically clean, but practically maintainable, robust, and adaptable to changing requirements.
Further Reading
If you’re interested in deepening your understanding of thoughtful software design, consider these resources:
- “A Philosophy of Software Design” by John Ousterhout
- “Domain-Driven Design” by Eric Evans
- “Simple Made Easy” talk by Rich Hickey
- “Design Patterns: Elements of Reusable Object-Oriented Software” by Gamma, Helm, Johnson, and Vlissides
- “Refactoring” by Martin Fowler
Each of these works goes beyond prescriptive patterns to explore the deeper principles of effective software design.