Why Coding Tutorials Don’t Teach Problem-Solving (And What You Can Do About It)
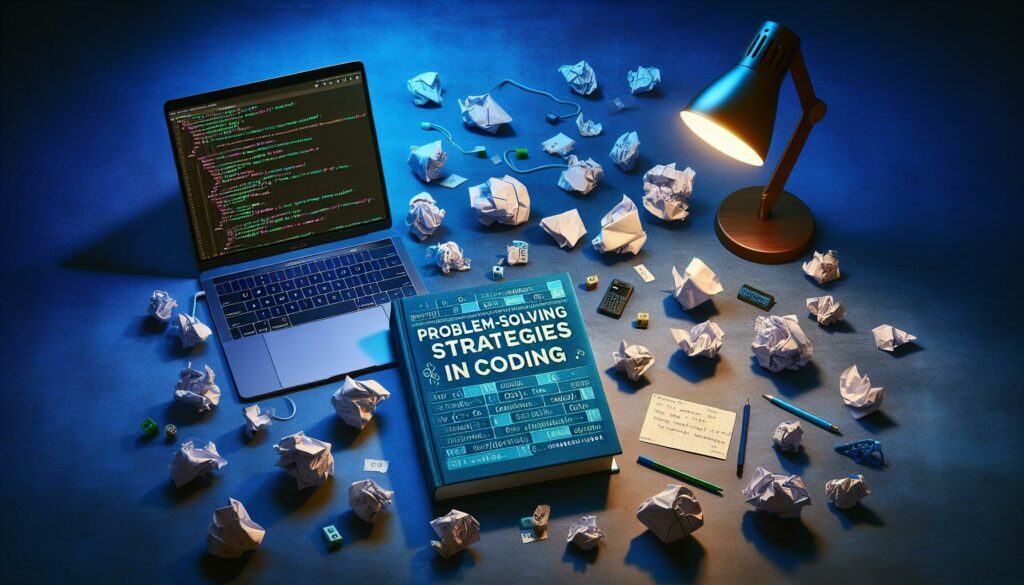
In the ever-evolving world of technology, coding has become an essential skill for many careers. As a result, countless coding tutorials, bootcamps, and online courses have emerged, promising to transform beginners into proficient programmers. However, there’s a glaring issue that many learners encounter: while these resources may teach you how to write code, they often fall short in developing crucial problem-solving skills. In this article, we’ll explore why this happens and, more importantly, what you can do to bridge this gap in your learning journey.
The Limitations of Traditional Coding Tutorials
Before we dive into solutions, let’s examine why many coding tutorials fail to adequately teach problem-solving skills:
1. Focus on Syntax Over Logic
Many tutorials prioritize teaching the syntax and basic structures of a programming language. While this is important, it often comes at the expense of developing logical thinking and problem-solving abilities. Learners may become proficient in writing syntactically correct code without truly understanding how to approach complex problems.
2. Lack of Real-World Context
Tutorials often present isolated examples that don’t reflect the complexity of real-world programming challenges. This can leave learners ill-prepared for the multifaceted problems they’ll encounter in actual development scenarios.
3. Over-Reliance on Step-by-Step Instructions
Many tutorials provide detailed, step-by-step instructions for completing coding tasks. While this approach can be helpful for beginners, it can also create a dependency on explicit directions, hindering the development of independent problem-solving skills.
4. Limited Exposure to Debugging and Troubleshooting
Problem-solving in programming often involves identifying and fixing errors. However, many tutorials focus on writing code from scratch and don’t provide enough practice in debugging existing code or troubleshooting complex issues.
5. Lack of Emphasis on Algorithmic Thinking
Algorithmic thinking—the ability to break down complex problems into smaller, manageable steps—is crucial for effective problem-solving. Unfortunately, many coding tutorials don’t explicitly teach or emphasize this skill.
The Importance of Problem-Solving in Coding
Before we explore solutions, it’s crucial to understand why problem-solving skills are so vital in the world of programming:
1. Adaptability in a Rapidly Changing Field
Technology evolves at a breakneck pace. Strong problem-solving skills allow programmers to adapt to new languages, frameworks, and paradigms more easily.
2. Efficiency in Development
Programmers with solid problem-solving skills can approach challenges more systematically, often leading to more efficient and elegant solutions.
3. Debugging and Troubleshooting
A significant part of a programmer’s job involves identifying and fixing issues in existing code. Strong problem-solving skills are essential for effective debugging.
4. Innovation and Creativity
Problem-solving skills foster creativity in coding, enabling programmers to develop innovative solutions and push the boundaries of what’s possible.
5. Career Advancement
In technical interviews and job performance evaluations, problem-solving abilities often carry more weight than mere coding proficiency.
Bridging the Gap: Developing Problem-Solving Skills
Now that we understand the limitations of many coding tutorials and the importance of problem-solving skills, let’s explore strategies to enhance your abilities in this crucial area:
1. Practice Algorithmic Thinking
Algorithmic thinking is the foundation of effective problem-solving in programming. Here’s how you can develop this skill:
- Break down problems: Before writing any code, practice breaking complex problems into smaller, manageable steps.
- Flowcharting: Use flowcharts to visualize problem-solving processes. This helps in understanding the logical flow of your solution.
- Pseudocode: Write out your solution in plain language before translating it into actual code. This helps focus on the logic rather than syntax.
Here’s an example of how you might approach a problem using pseudocode:
Problem: Calculate the sum of even numbers in an array
Pseudocode:
1. Initialize a variable 'sum' to 0
2. For each number in the array:
a. If the number is even (divisible by 2 with no remainder):
- Add the number to 'sum'
3. Return 'sum'
2. Engage in Coding Challenges and Puzzles
Platforms like LeetCode, HackerRank, and CodeWars offer a wealth of coding challenges that test your problem-solving abilities. These platforms often provide:
- A wide range of difficulty levels
- Problems that mimic real-world scenarios and technical interview questions
- The opportunity to see and learn from others’ solutions
Regularly engaging with these platforms can significantly enhance your problem-solving skills and prepare you for technical interviews.
3. Implement Projects from Scratch
While tutorials are helpful, there’s no substitute for building projects from the ground up. This approach forces you to:
- Plan and structure your code
- Make design decisions
- Troubleshoot issues without step-by-step guidance
Start with simple projects and gradually increase complexity. For example, you might progress from a basic calculator app to a full-fledged task management system.
4. Learn and Apply Design Patterns
Design patterns are reusable solutions to common programming problems. Learning these can enhance your problem-solving toolkit. Some fundamental patterns to start with include:
- Singleton Pattern
- Factory Pattern
- Observer Pattern
- Strategy Pattern
Here’s a simple example of the Singleton pattern in Python:
class Singleton:
_instance = None
def __new__(cls):
if cls._instance is None:
cls._instance = super().__new__(cls)
return cls._instance
# Usage
s1 = Singleton()
s2 = Singleton()
print(s1 is s2) # Output: True
5. Practice Debugging and Code Review
Debugging is a crucial problem-solving skill. To improve:
- Deliberately introduce bugs into working code and practice finding and fixing them
- Use debugging tools in your IDE to step through code execution
- Participate in code reviews to gain exposure to different problem-solving approaches
6. Collaborate on Open Source Projects
Contributing to open-source projects exposes you to real-world codebases and collaborative problem-solving. It allows you to:
- Work on complex, established projects
- Interact with experienced developers
- Practice reading and understanding others’ code
- Gain experience in version control and collaborative development workflows
7. Study Algorithms and Data Structures
A solid understanding of algorithms and data structures is fundamental to problem-solving in programming. Focus on:
- Basic data structures (arrays, linked lists, stacks, queues, trees, graphs)
- Sorting and searching algorithms
- Time and space complexity analysis
Here’s a simple implementation of a binary search algorithm in JavaScript:
function binarySearch(arr, target) {
let left = 0;
let right = arr.length - 1;
while (left <= right) {
let mid = Math.floor((left + right) / 2);
if (arr[mid] === target) return mid;
if (arr[mid] < target) left = mid + 1;
else right = mid - 1;
}
return -1; // Target not found
}
// Usage
let sortedArray = [1, 3, 5, 7, 9, 11, 13, 15];
console.log(binarySearch(sortedArray, 7)); // Output: 3
console.log(binarySearch(sortedArray, 10)); // Output: -1
8. Embrace the Rubber Duck Debugging Technique
This technique involves explaining your code or problem to an inanimate object (like a rubber duck). The process of articulating the problem often leads to insights and solutions. This method:
- Forces you to think through your code step-by-step
- Helps identify assumptions and logical flaws
- Improves your ability to communicate technical concepts
9. Analyze and Refactor Existing Code
Improving existing code is a valuable problem-solving skill. Practice by:
- Analyzing code for inefficiencies or potential improvements
- Refactoring code to be more efficient, readable, or maintainable
- Applying principles like DRY (Don’t Repeat Yourself) and SOLID
10. Utilize Interactive Learning Platforms
Platforms like AlgoCademy offer a more holistic approach to learning coding and problem-solving. These platforms often provide:
- Interactive coding environments
- AI-powered assistance for personalized learning
- A focus on algorithmic thinking and problem-solving strategies
- Preparation for technical interviews at major tech companies
Implementing Problem-Solving in Your Coding Journey
Now that we’ve explored various strategies to enhance your problem-solving skills, let’s discuss how to integrate these practices into your coding journey:
1. Create a Balanced Learning Plan
Develop a learning plan that balances syntax learning with problem-solving practice. For example:
- Spend 50% of your time on tutorials and syntax learning
- Dedicate 30% to coding challenges and algorithmic problems
- Use the remaining 20% for personal projects or open-source contributions
2. Set Specific Problem-Solving Goals
Establish clear, measurable goals for your problem-solving skills. For instance:
- Solve 5 coding challenges per week
- Implement one new design pattern in a project each month
- Contribute to an open-source project quarterly
3. Maintain a Problem-Solving Journal
Keep a journal to track your problem-solving experiences. For each problem you tackle, record:
- The problem statement
- Your initial approach
- Challenges encountered
- The final solution
- Lessons learned or alternative approaches discovered
4. Join a Coding Community
Engage with other learners and experienced programmers. This can be through:
- Online forums like Stack Overflow or Reddit’s programming communities
- Local coding meetups or hackathons
- Coding-focused Discord servers or Slack channels
5. Teach Others
Teaching is an excellent way to reinforce your own understanding and problem-solving skills. Consider:
- Starting a coding blog to explain concepts you’ve learned
- Mentoring junior developers or peers
- Creating tutorial videos on YouTube
6. Regularly Review and Reflect
Set aside time each week or month to review your progress:
- Assess the problems you’ve solved and identify areas for improvement
- Review your problem-solving journal for patterns or recurring challenges
- Adjust your learning plan based on your progress and goals
Conclusion: Embracing the Problem-Solving Mindset
While coding tutorials are valuable for learning syntax and basic concepts, they often fall short in developing crucial problem-solving skills. By implementing the strategies discussed in this article, you can bridge this gap and become a more well-rounded, effective programmer.
Remember, becoming proficient in problem-solving is an ongoing journey. It requires consistent practice, a willingness to tackle challenges, and the ability to learn from both successes and failures. As you progress, you’ll find that strong problem-solving skills not only make you a better programmer but also enhance your ability to tackle challenges in all areas of life.
Platforms like AlgoCademy recognize the importance of this holistic approach to coding education. By combining interactive tutorials with a focus on algorithmic thinking and problem-solving strategies, they provide a more comprehensive learning experience. This approach not only prepares you for coding challenges but also equips you with the skills needed to excel in technical interviews and real-world development scenarios.
Embrace the problem-solving mindset, and you’ll find yourself not just writing code, but creating innovative solutions and pushing the boundaries of what’s possible in the world of technology. Happy coding, and may your problem-solving skills continue to grow and evolve throughout your programming journey!