Why Coding Problems Are Like Escape Rooms: How to ‘Escape’ with the Solution
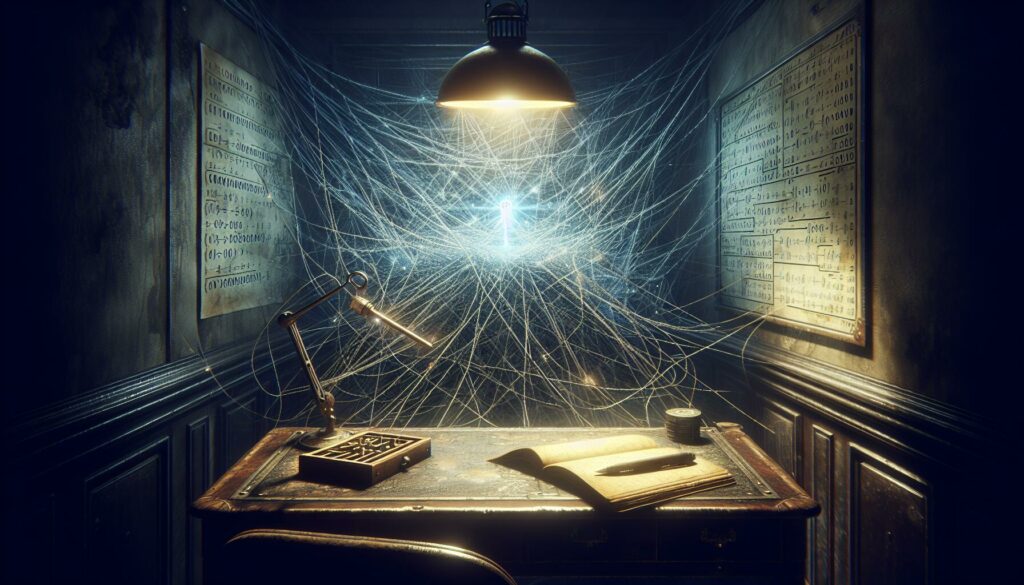
Have you ever found yourself stuck in a coding problem, feeling like you’re trapped in a virtual maze with no clear way out? If so, you’re not alone. Many programmers, from beginners to seasoned professionals, often encounter challenging problems that seem impossible to solve at first glance. But what if I told you that tackling coding problems is a lot like solving an escape room puzzle? In this article, we’ll explore the fascinating parallels between coding challenges and escape rooms, and how understanding this connection can help you become a more effective problem-solver and coder.
The Escape Room Analogy
Before we dive into the similarities, let’s briefly explain what an escape room is for those who might not be familiar. An escape room is a physical adventure game where players are locked in a room and must use elements within that room to solve a series of puzzles, find clues, and escape within a set time limit. Sound familiar? That’s because coding problems often present a similar challenge: you’re given a set of constraints (the room), tools (your programming language and its features), and a goal (escaping, or in coding terms, solving the problem).
Similarities Between Coding Problems and Escape Rooms
1. Limited Resources
In an escape room, you’re limited to what’s available within the room. Similarly, in coding problems, you’re often restricted to certain data structures, algorithms, or language features. This constraint forces you to think creatively and use what you have efficiently.
2. Time Pressure
Escape rooms typically have a time limit, adding an element of urgency to the puzzle-solving process. While coding problems may not always have a strict time limit, there’s often an implicit pressure to solve problems efficiently, especially in technical interviews or when working on time-sensitive projects.
3. Logical Thinking
Both coding and escape rooms require strong logical thinking skills. You need to analyze the problem, identify patterns, and make connections between different elements to progress towards the solution.
4. Trial and Error
In both scenarios, you’ll likely try multiple approaches before finding the right solution. This process of trial and error is crucial for learning and problem-solving in both coding and escape rooms.
5. Collaboration
While not always the case, both coding problems and escape rooms can benefit from collaboration. In team coding projects or pair programming sessions, multiple minds can work together to solve complex problems, much like how escape rooms are often designed for group participation.
6. Incremental Progress
In an escape room, you often solve a series of smaller puzzles that lead to the final solution. Similarly, in coding, you might break down a larger problem into smaller, more manageable sub-problems.
7. The “Aha!” Moment
Both coding and escape rooms offer that satisfying “Aha!” moment when you finally crack a difficult puzzle or solve a challenging problem. This sense of accomplishment is a powerful motivator in both activities.
How to ‘Escape’ with the Solution
Now that we’ve established the similarities, let’s explore strategies for “escaping” coding problems, inspired by escape room tactics:
1. Survey Your Surroundings
In an escape room, the first step is usually to take a good look around and inventory what’s available. In coding:
- Read the problem statement carefully
- Identify the inputs and expected outputs
- Note any constraints or special conditions
- Consider what data structures and algorithms might be relevant
2. Start with the Obvious
Escape room designers often include some easier puzzles to build momentum. In coding:
- Begin with a brute force solution if possible
- Solve any simpler sub-problems first
- Don’t overlook straightforward approaches; sometimes the simple solution is the correct one
3. Look for Patterns and Connections
In escape rooms, clues often relate to each other. In coding:
- Analyze the problem for recurring patterns
- Consider how different parts of the problem might be connected
- Look for similarities to problems you’ve solved before
4. Use Your Tools Wisely
Escape rooms provide tools to help solve puzzles. In coding, your tools include:
- Your chosen programming language and its standard library
- Data structures (arrays, linked lists, trees, graphs, etc.)
- Algorithms (sorting, searching, dynamic programming, etc.)
- Debugging techniques and tools
5. Don’t Be Afraid to Backtrack
In an escape room, you might need to revisit earlier puzzles. In coding:
- If your current approach isn’t working, be willing to start over
- Reevaluate your assumptions if you’re stuck
- Consider alternative data structures or algorithms
6. Collaborate and Communicate
Even when coding alone, you can apply collaborative principles:
- Explain your thought process out loud (rubber duck debugging)
- Seek input from peers or online communities when truly stuck
- In team settings, clearly communicate your ideas and listen to others
7. Manage Your Time
Time management is crucial in both escape rooms and coding challenges:
- Set a time limit for your initial attempt
- If stuck, take short breaks to refresh your mind
- Know when to move on to a different approach
8. Learn from Your Mistakes
After completing an escape room or solving a coding problem:
- Reflect on what worked and what didn’t
- Analyze more efficient solutions if available
- Apply lessons learned to future problems
Real-World Coding Example: The Maze Solver
Let’s put these principles into practice with a coding problem that mirrors the escape room experience: solving a maze. This problem showcases many of the parallels we’ve discussed and provides a concrete example of how to approach a coding challenge like an escape room puzzle.
The Problem Statement
You are given a 2D grid representing a maze. The maze consists of open paths (represented by 0) and walls (represented by 1). Your task is to find a path from the start point (0, 0) to the end point (n-1, m-1), where n and m are the number of rows and columns in the maze, respectively. You can move in four directions: up, down, left, and right.
The Escape Room Approach
Let’s tackle this problem using our escape room-inspired strategies:
1. Survey Your Surroundings
- We have a 2D grid representing the maze
- Start point is (0, 0), end point is (n-1, m-1)
- We can move in four directions
- The goal is to find a path from start to end
2. Start with the Obvious
A straightforward approach would be to use a depth-first search (DFS) algorithm to explore all possible paths.
3. Look for Patterns and Connections
This problem is similar to graph traversal problems, where each cell is a node, and adjacent cells are connected.
4. Use Your Tools Wisely
We can use a recursive DFS implementation, utilizing the call stack to keep track of our path.
The Solution
Here’s a Python implementation that solves the maze problem:
def solve_maze(maze):
def dfs(x, y, path):
if x < 0 or x >= len(maze) or y < 0 or y >= len(maze[0]) or maze[x][y] == 1:
return False
path.append((x, y))
if x == len(maze) - 1 and y == len(maze[0]) - 1:
return True
maze[x][y] = 1 # Mark as visited
# Explore in all four directions
for dx, dy in [(0, 1), (1, 0), (0, -1), (-1, 0)]:
if dfs(x + dx, y + dy, path):
return True
path.pop() # Backtrack
return False
path = []
if dfs(0, 0, path):
return path
else:
return None
# Example usage
maze = [
[0, 0, 0, 0],
[1, 1, 0, 1],
[0, 0, 0, 0],
[0, 1, 1, 0]
]
solution = solve_maze(maze)
if solution:
print("Path found:", solution)
else:
print("No path found")
Explaining the Solution
This solution demonstrates several escape room-like problem-solving techniques:
- Surveying the surroundings: We define the problem constraints (maze boundaries, valid moves) in the DFS function.
- Starting with the obvious: We use a simple DFS approach to explore all possible paths.
- Using tools wisely: We leverage recursion and backtracking, common tools in algorithmic problem-solving.
- Looking for patterns: We treat the maze as a graph, applying graph traversal techniques.
- Backtracking: If a path doesn’t lead to the exit, we backtrack and try another route.
The Benefits of the Escape Room Mindset
Approaching coding problems with an escape room mindset can yield several benefits:
1. Enhanced Problem-Solving Skills
By viewing coding challenges as puzzles to be solved, you develop a more creative and analytical approach to problem-solving. This mindset encourages you to look at problems from multiple angles and consider various solutions.
2. Improved Persistence
Escape rooms teach us that the solution isn’t always immediately apparent. This perspective can help you persist through difficult coding problems, knowing that the solution is there if you keep exploring.
3. Better Time Management
The time pressure in escape rooms translates well to coding challenges, especially in interview situations. You learn to balance thoroughness with efficiency.
4. Enhanced Collaboration Skills
Even when coding alone, the escape room mindset encourages you to think about how you might explain your approach to others or consider alternative viewpoints.
5. Increased Enjoyment
Viewing coding challenges as exciting puzzles to be solved can make the process more enjoyable and rewarding, leading to increased motivation and learning.
Conclusion
The parallels between solving coding problems and escaping from puzzle rooms are striking and insightful. By adopting an escape room mindset, you can transform challenging coding problems from daunting obstacles into exciting puzzles waiting to be solved. Remember to survey your surroundings, start with the obvious, look for patterns, use your tools wisely, and don’t be afraid to backtrack when needed.
As you continue your coding journey, whether you’re a beginner tackling your first algorithms or an experienced developer preparing for technical interviews, keep this escape room analogy in mind. It can help you approach problems with creativity, persistence, and a sense of adventure. After all, every great coder is also a great problem solver, and every coding challenge is just another room waiting for you to escape.
So the next time you face a difficult coding problem, take a deep breath, imagine you’re stepping into an escape room, and start exploring. The solution is out there, and with the right mindset and approach, you’ll find your way to the exit. Happy coding, and may all your escapes be successful!