Why Coders Should Study Philosophy: The Art of Logic and Reasoning
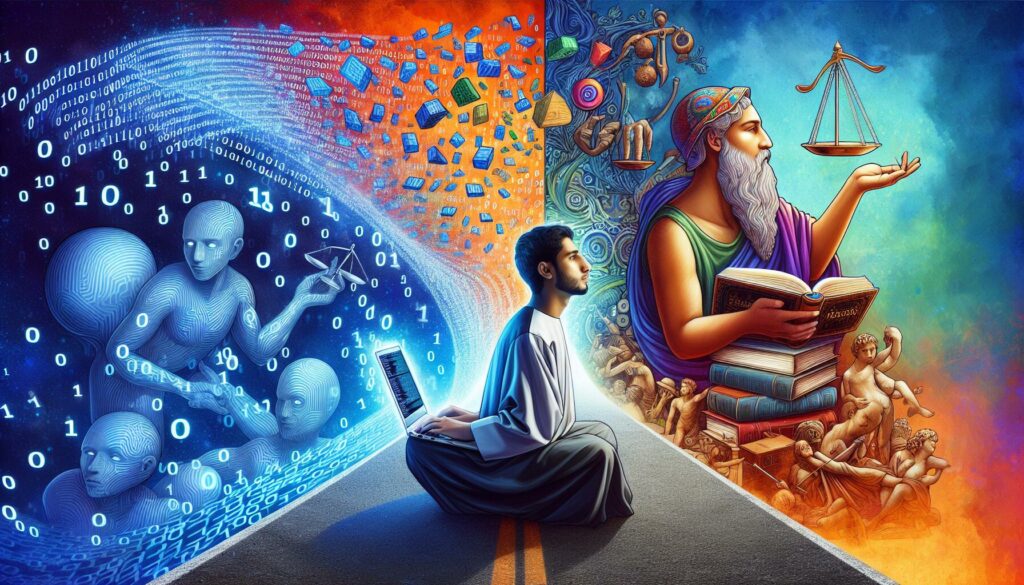
In the fast-paced world of technology and coding, it’s easy to get caught up in the latest programming languages, frameworks, and tools. However, there’s an often-overlooked discipline that can significantly enhance a coder’s skills and problem-solving abilities: philosophy. At first glance, coding and philosophy might seem worlds apart, but upon closer inspection, they share fundamental principles that can greatly benefit aspiring and seasoned programmers alike. In this article, we’ll explore why coders should consider studying philosophy and how it can improve their coding skills, particularly in the context of preparing for technical interviews at major tech companies.
The Connection Between Coding and Philosophy
At its core, coding is about solving problems through logical reasoning and structured thinking. Similarly, philosophy is concerned with understanding complex ideas, analyzing arguments, and developing critical thinking skills. Both disciplines require a methodical approach to breaking down problems and finding solutions.
Here are some key areas where philosophy intersects with coding:
- Logic and reasoning
- Problem-solving
- Ethical considerations
- Abstract thinking
- Communication and argumentation
Let’s delve deeper into each of these areas to understand how they can benefit coders in their professional journey.
Logic and Reasoning: The Foundation of Both Disciplines
Logic is the backbone of both philosophy and computer science. In philosophy, formal logic is used to analyze arguments and determine their validity. In coding, logical thinking is essential for creating efficient algorithms and debugging code.
Studying philosophical logic can help coders:
- Improve their ability to construct valid arguments
- Identify logical fallacies in their own and others’ reasoning
- Enhance their understanding of boolean logic, which is fundamental to programming
- Develop a more structured approach to problem-solving
For example, consider the following logical argument in philosophy:
Premise 1: All humans are mortal.
Premise 2: Socrates is a human.
Conclusion: Therefore, Socrates is mortal.
This structure is similar to the logical flow in programming:
if (isHuman(x)) {
isMortal(x) = true;
}
if (isHuman(Socrates)) {
// Socrates is mortal
}
By studying philosophical logic, coders can strengthen their ability to construct and analyze logical structures, which is crucial for writing clean, efficient code and solving complex programming problems.
Problem-Solving: A Shared Goal
Both philosophy and coding are fundamentally about solving problems. Philosophers tackle abstract, conceptual problems, while coders solve concrete, practical ones. However, the approaches used in both fields can be remarkably similar.
Philosophical problem-solving techniques that can benefit coders include:
- Breaking down complex problems into smaller, manageable parts
- Considering multiple perspectives and potential solutions
- Questioning assumptions and challenging established ideas
- Using thought experiments to explore hypothetical scenarios
These techniques align closely with best practices in coding, such as:
- Modular programming and breaking down complex functions
- Considering edge cases and alternative implementations
- Refactoring code to improve efficiency and readability
- Using unit tests to validate code behavior
For instance, the philosophical concept of Occam’s Razor, which states that the simplest explanation is often the correct one, can be applied to coding in the form of the KISS principle (Keep It Simple, Stupid). This principle encourages programmers to write clear, concise code that’s easy to understand and maintain.
Ethical Considerations in Coding
As technology becomes increasingly integrated into our daily lives, the ethical implications of coding decisions have become more important than ever. Studying philosophy, particularly ethics, can help coders navigate the complex moral landscape of modern technology.
Some ethical issues that coders may encounter include:
- Data privacy and security
- Algorithmic bias and fairness
- The social impact of automation and AI
- Intellectual property and open-source software
Philosophy provides frameworks for thinking about these issues, such as:
- Utilitarianism: Maximizing overall benefit for the greatest number of people
- Deontological ethics: Following moral rules and duties
- Virtue ethics: Cultivating moral character and virtues
By studying these ethical frameworks, coders can make more informed decisions about the potential consequences of their work and contribute to the development of responsible technology.
Abstract Thinking: Bridging Concepts and Code
Philosophy excels at dealing with abstract concepts, which is a crucial skill for coders working with complex systems and algorithms. Studying philosophy can enhance a programmer’s ability to think abstractly and work with high-level concepts.
Benefits of abstract thinking for coders include:
- Improved ability to design and implement object-oriented programming structures
- Better understanding of functional programming concepts
- Enhanced capacity to work with abstract data types and algorithms
- Increased skill in creating and understanding system architectures
For example, the philosophical concept of Platonic forms, which suggests that abstract ideas exist independently of physical objects, can be related to the concept of classes and objects in object-oriented programming:
class Dog:
def __init__(self, name, breed):
self.name = name
self.breed = breed
def bark(self):
print(f"{self.name} says: Woof!")
In this example, the Dog
class represents the abstract idea or “form” of a dog, while individual dog objects are instances of this abstract concept.
Communication and Argumentation: Expressing Ideas Clearly
Philosophy places a strong emphasis on clear communication and well-structured arguments. These skills are invaluable for coders, especially when it comes to:
- Writing clear and concise documentation
- Explaining complex technical concepts to non-technical stakeholders
- Defending design decisions and proposing new solutions
- Collaborating effectively with team members
Studying philosophical argumentation can help coders:
- Organize their thoughts more effectively
- Anticipate and address potential counterarguments
- Present ideas in a logical, persuasive manner
- Improve their ability to understand and critique others’ arguments
These skills are particularly useful when preparing for technical interviews at major tech companies, where clear communication and problem-solving abilities are highly valued.
Applying Philosophical Thinking to Coding Challenges
Let’s examine how philosophical thinking can be applied to a common coding challenge: implementing a sorting algorithm. We’ll use the quicksort algorithm as an example.
The quicksort algorithm follows a divide-and-conquer approach, which aligns with the philosophical method of breaking down complex problems into smaller, more manageable parts. Here’s a Python implementation of quicksort:
def quicksort(arr):
if len(arr) <= 1:
return arr
else:
pivot = arr[len(arr) // 2]
left = [x for x in arr if x < pivot]
middle = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
return quicksort(left) + middle + quicksort(right)
Now, let’s apply some philosophical thinking to analyze and improve this implementation:
- Logical reasoning: We can use logical reasoning to verify the correctness of the algorithm. For instance, we can prove that the algorithm will always terminate by showing that the subproblems (left and right arrays) are always smaller than the original problem.
- Abstract thinking: The concept of a “pivot” in quicksort is an abstract idea that helps us divide the problem. This abstraction allows us to think about the algorithm at a higher level, focusing on the strategy rather than the implementation details.
- Ethical considerations: While quicksort is generally efficient, it can have poor performance in certain cases. We might consider the ethical implications of using this algorithm in critical systems where consistent performance is crucial.
- Problem-solving: We can apply philosophical problem-solving techniques to optimize the algorithm. For example, we might question the assumption that the middle element is always the best pivot and explore alternative pivot selection strategies.
- Communication: When explaining this algorithm to others, we can use clear, logical arguments to describe how and why it works, drawing on our philosophical training in argumentation.
Philosophy and Technical Interviews
For coders preparing for technical interviews at major tech companies, philosophical skills can be a significant asset. Here’s how:
- Problem decomposition: Many interview questions involve complex problems that need to be broken down into smaller, more manageable parts. The analytical skills developed through studying philosophy can help candidates approach these problems methodically.
- Logical reasoning: Technical interviews often include questions that test a candidate’s ability to reason logically about code and algorithms. A strong foundation in philosophical logic can enhance performance in these areas.
- Abstract thinking: System design questions, which are common in interviews at large tech companies, require the ability to think abstractly about complex systems. Philosophical training in dealing with abstract concepts can be invaluable here.
- Clear communication: Interviewers are not just looking for correct solutions; they want to understand a candidate’s thought process. The communication skills honed through studying philosophy can help candidates articulate their ideas clearly and persuasively.
- Ethical awareness: As tech companies face increasing scrutiny over the ethical implications of their products, demonstrating an understanding of ethical considerations in technology can set a candidate apart.
Integrating Philosophy into Coding Education
Given the benefits of philosophical thinking for coders, it’s worth considering how to integrate elements of philosophy into coding education. Here are some suggestions:
- Introduce logical reasoning exercises: Incorporate exercises that focus on formal logic and argumentation alongside traditional coding problems.
- Explore ethical case studies: Discuss real-world ethical dilemmas in technology to help students develop a nuanced understanding of the impact of their work.
- Encourage abstract thinking: Introduce philosophical concepts that relate to programming paradigms and encourage students to think about the abstract principles underlying different approaches to coding.
- Emphasize clear communication: Include assignments that require students to explain their code and design decisions clearly and persuasively.
- Foster critical thinking: Encourage students to question assumptions and critically evaluate different solutions to coding problems.
For platforms like AlgoCademy that focus on coding education and interview preparation, incorporating philosophical elements could involve:
- Adding logic puzzles and reasoning exercises to complement algorithmic challenges
- Including discussions of ethical considerations in system design questions
- Providing resources on clear communication and argumentation for technical discussions
- Offering modules on abstract thinking and problem decomposition
Conclusion: The Synergy of Code and Philosophy
While coding and philosophy might seem like disparate fields at first glance, they share fundamental principles that can greatly benefit aspiring and experienced programmers alike. By studying philosophy, coders can enhance their logical reasoning, problem-solving abilities, ethical awareness, abstract thinking, and communication skills.
These skills are not only valuable for writing better code and creating more thoughtful technology solutions but are also highly prized by major tech companies in their hiring processes. As the tech industry continues to grapple with complex ethical and societal issues, the ability to think critically and reason clearly about these challenges will become increasingly important.
For platforms like AlgoCademy and other coding education initiatives, integrating philosophical elements into their curricula can provide a more well-rounded education that prepares students not just for coding challenges, but for the broader challenges they’ll face in their careers as technologists.
In the end, the study of philosophy can help coders not only become better programmers but also more thoughtful and responsible creators of technology. By embracing both the logical rigor of coding and the analytical depth of philosophy, we can foster a new generation of technologists equipped to tackle the complex challenges of our increasingly digital world.