Why “Clean Code” Might Be Overrated in Startups
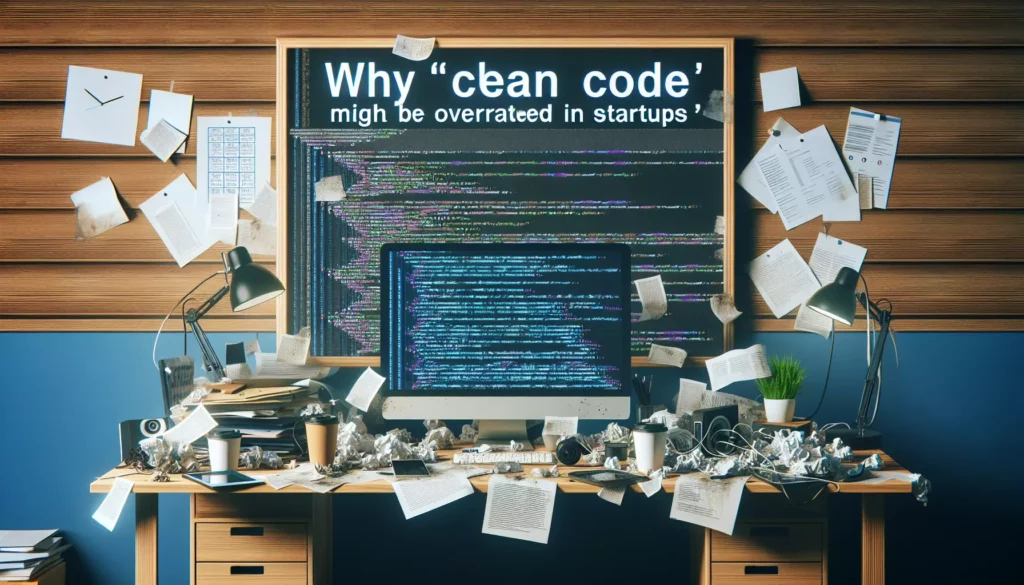
In the world of software development, “Clean Code” has become something of a holy grail. It’s a concept that’s drilled into developers from their early days of learning to code, through bootcamps, and well into their professional careers. But is this obsession with pristine, perfectly structured code always beneficial, especially in the fast-paced, high-pressure environment of a startup? Let’s dive deep into why the pursuit of clean code might sometimes be overrated in the startup world.
What is “Clean Code”?
Before we challenge the concept, let’s define what we mean by “clean code.” The term was popularized by Robert C. Martin in his book “Clean Code: A Handbook of Agile Software Craftsmanship.” According to Martin, clean code is:
- Easy to understand
- Easy to modify
- Easy to test
- Follows a consistent style
- Has meaningful names for variables and functions
- Has minimal dependencies
- Is well-documented
In essence, clean code is supposed to be a joy to read and work with. It’s the kind of code that makes other developers nod in appreciation and think, “This is how it should be done.”
The Case for Clean Code
There’s no denying that clean code has its merits. In an ideal world, all code would be clean, efficient, and easily maintainable. The benefits of clean code include:
- Improved Readability: Clean code is easier for other developers (and your future self) to understand and work with.
- Easier Maintenance: When code is clean and well-structured, it’s easier to update and maintain over time.
- Reduced Bugs: Clean code often leads to fewer bugs and easier debugging when issues do arise.
- Better Collaboration: Teams can work more effectively when the codebase is clean and consistent.
- Scalability: Clean code is often more scalable and can grow with your project.
Given these benefits, it’s easy to see why clean code is so highly valued in the software development community. However, the startup world presents a unique set of challenges that can make the pursuit of clean code problematic.
The Startup Reality
Startups operate in a different reality compared to established companies. They face unique pressures and constraints that can make the relentless pursuit of clean code a potential liability. Here’s why:
1. Time is of the Essence
In the startup world, speed is often more critical than perfection. The ability to quickly iterate, pivot, and respond to market demands can make the difference between success and failure. Spending too much time refactoring code to make it “clean” can slow down development and delay crucial releases.
2. Limited Resources
Startups often operate with limited financial resources and small teams. Every hour spent on code refactoring is an hour not spent on developing new features, fixing critical bugs, or addressing customer needs.
3. Uncertain Future
Many startups pivot multiple times before finding their product-market fit. Code that seems crucial today might be completely irrelevant tomorrow. Investing too heavily in creating pristine code for features that may be discarded can be a waste of valuable resources.
4. Changing Requirements
Startup requirements can change rapidly based on user feedback, market conditions, or investor pressures. Code that was “clean” for yesterday’s requirements might not be suitable for today’s needs.
5. Technical Debt is Not Always Bad
While clean code advocates often view technical debt as a negative, in the startup world, it can be a strategic tool. Sometimes, it’s better to ship a product with less-than-perfect code and gain market traction than to delay launch in pursuit of code perfection.
The Cost of Clean Code in Startups
While clean code has its benefits, pursuing it relentlessly in a startup environment can come with significant costs:
1. Opportunity Cost
Every hour spent refactoring existing code to make it cleaner is an hour not spent on developing new features, acquiring customers, or improving the product based on user feedback.
2. Delayed Time-to-Market
In the race to establish market presence, delays can be costly. If your competitors launch first because they were willing to ship “good enough” code, you might lose critical market share.
3. Premature Optimization
Spending time making code clean and efficient for scale before you’ve even validated your product can be a form of premature optimization. You might be solving problems you don’t have yet, at the expense of solving the problems you do have.
4. Perfectionism Paralysis
The pursuit of clean code can lead to a form of perfectionism that paralyzes progress. Developers might become hesitant to ship code that doesn’t meet their high standards, even when that code is functional and solves the immediate problem.
5. Misaligned Priorities
In a startup, the priority should be on creating value for users and validating business hypotheses. An excessive focus on code cleanliness can distract from these crucial goals.
When Clean Code Matters in Startups
This isn’t to say that clean code has no place in startups. There are certainly times when investing in code quality is crucial:
1. Core Business Logic
The parts of your codebase that represent your core business logic and are likely to stick around for the long term should be written cleanly. This is the code that defines what makes your product unique and valuable.
2. Security-Critical Components
Any code dealing with user data, authentication, or financial transactions should be clean, well-tested, and secure. There’s no room for shortcuts when it comes to user trust and legal compliance.
3. After Achieving Product-Market Fit
Once you’ve validated your product and are preparing to scale, that’s when you should start paying more attention to code cleanliness. At this stage, you’ll be glad you at least kept your core components clean.
4. Collaborative Areas
Parts of the codebase where multiple team members frequently work should be kept reasonably clean to facilitate collaboration and reduce friction.
Balancing Act: The Pragmatic Approach
So, how can startups balance the need for speed and flexibility with the benefits of clean code? Here are some pragmatic approaches:
1. Adopt a “Clean Enough” Philosophy
Instead of aiming for perfect code, aim for code that’s “clean enough.” This means writing code that’s functional, reasonably understandable, and not overly complex. The goal is to find a balance between speed and maintainability.
2. Prioritize Ruthlessly
Be strategic about where you invest your cleaning efforts. Focus on keeping core components and frequently accessed code clean, while being more lenient with experimental features or parts of the codebase that are likely to change.
3. Embrace Iterative Improvement
Instead of trying to write perfect code from the start, embrace an iterative approach. Get the basic functionality working first, then improve and clean up the code in subsequent iterations as the feature proves its value.
4. Use Code Reviews Wisely
Code reviews are a great tool for maintaining code quality, but in a startup context, they should focus more on catching major issues and sharing knowledge rather than nitpicking every detail.
5. Automate What You Can
Use tools like linters, formatters, and static analysis to automatically catch and fix common code style issues. This can help maintain a baseline of code cleanliness without significant manual effort.
6. Document Intentional Technical Debt
When you make a conscious decision to write code that’s not as clean as you’d like, document it. Use comments or tickets to note areas that need refactoring in the future. This helps manage technical debt without slowing down current progress.
7. Educate the Team
Ensure that all team members understand the balance between clean code and startup realities. Foster a culture that values pragmatism and business impact alongside technical excellence.
Code Examples: Clean vs. Pragmatic
Let’s look at some code examples to illustrate the difference between a “clean code at all costs” approach and a more pragmatic one suitable for a startup environment.
Example 1: User Authentication
Clean Code Approach:
class UserAuthenticator:
def __init__(self, user_repository, password_hasher, token_generator):
self.user_repository = user_repository
self.password_hasher = password_hasher
self.token_generator = token_generator
def authenticate(self, username, password):
user = self.user_repository.find_by_username(username)
if not user:
return None
if not self.password_hasher.verify(password, user.password_hash):
return None
return self.token_generator.generate_token(user)
# Usage
authenticator = UserAuthenticator(UserRepository(), PasswordHasher(), TokenGenerator())
token = authenticator.authenticate("username", "password")
Pragmatic Approach for a Startup:
def authenticate(username, password):
user = db.users.find_one({"username": username})
if user and bcrypt.checkpw(password.encode('utf-8'), user['password_hash']):
return jwt.encode({"user_id": str(user['_id'])}, SECRET_KEY, algorithm="HS256")
return None
# Usage
token = authenticate("username", "password")
The clean code approach is more modular and easier to test, but the pragmatic approach is quicker to implement and might be sufficient for an early-stage startup. The pragmatic version sacrifices some flexibility and testability for speed and simplicity.
Example 2: Feature Flag Implementation
Clean Code Approach:
class FeatureFlag:
def __init__(self, name, default_value):
self.name = name
self.default_value = default_value
def is_enabled(self, user):
# Complex logic to check if feature is enabled for user
pass
class FeatureFlagManager:
def __init__(self):
self.flags = {}
def register_flag(self, flag):
self.flags[flag.name] = flag
def is_feature_enabled(self, flag_name, user):
if flag_name not in self.flags:
return False
return self.flags[flag_name].is_enabled(user)
# Usage
manager = FeatureFlagManager()
manager.register_flag(FeatureFlag("new_ui", False))
if manager.is_feature_enabled("new_ui", current_user):
show_new_ui()
else:
show_old_ui()
Pragmatic Approach for a Startup:
FEATURE_FLAGS = {
"new_ui": False,
"beta_feature": True
}
def is_feature_enabled(flag_name, user=None):
return FEATURE_FLAGS.get(flag_name, False)
# Usage
if is_feature_enabled("new_ui"):
show_new_ui()
else:
show_old_ui()
The clean code approach provides a robust system for managing feature flags, but it might be overkill for a startup that just needs to quickly toggle features on and off. The pragmatic approach is simpler and faster to implement, though it lacks the flexibility to enable features for specific users or groups.
Conclusion: Finding the Right Balance
In the world of startups, the relentless pursuit of clean code can sometimes do more harm than good. While clean code has its place and benefits, it’s crucial to balance it against the realities of limited resources, rapid change, and the need for speed in the startup world.
The key is to adopt a pragmatic approach that values business impact and user needs alongside code quality. Write code that’s clean enough to be maintainable and understandable, but don’t let the pursuit of perfect code paralyze your progress or delay your time-to-market.
Remember, in a startup, shipped code that solves real user problems is infinitely more valuable than perfect code that never sees the light of day. As your startup grows and stabilizes, you can gradually increase your focus on code cleanliness, refactoring and improving as you go.
Ultimately, the goal should be to find the sweet spot where your code is clean enough to support your current needs and future growth, but not so clean that it becomes a bottleneck to your startup’s progress. By striking this balance, you can harness the benefits of clean code without falling into the trap of overvaluing it at the expense of your startup’s success.