Why Clarifying Assumptions and Asking Questions Is Key to Interview Success
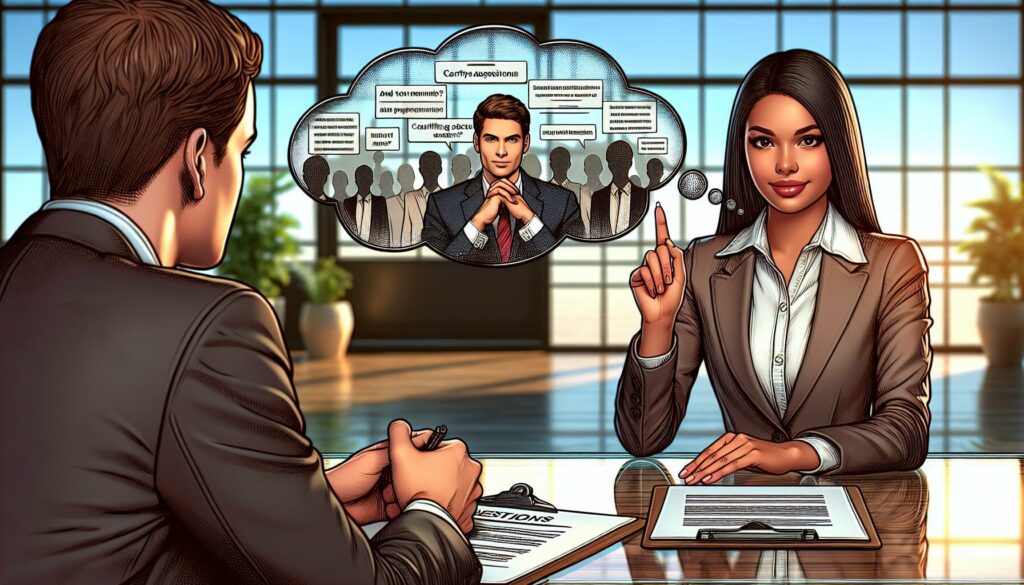
When it comes to technical interviews, particularly those involving coding challenges, many candidates make the mistake of diving straight into writing code without fully understanding the problem at hand. This approach can lead to wasted time, incorrect solutions, and ultimately, a poor impression on the interviewer. In this comprehensive guide, we’ll explore why clarifying assumptions and asking questions is crucial for interview success, and provide you with strategies to master this essential skill.
The Importance of Clarification in Technical Interviews
Before we delve into specific strategies, let’s understand why clarification is so vital in the context of technical interviews:
- Demonstrates critical thinking: By asking thoughtful questions, you show the interviewer that you approach problems analytically and don’t make hasty assumptions.
- Saves time: Clarifying the problem upfront can prevent you from going down the wrong path and having to start over.
- Improves accuracy: A clear understanding of the requirements leads to more accurate and efficient solutions.
- Showcases communication skills: Effective communication is crucial in any development role, and the interview is your chance to demonstrate this skill.
- Builds rapport: Engaging in a dialogue with the interviewer can create a more collaborative atmosphere.
Common Pitfalls of Skipping Clarification
To further emphasize the importance of clarification, let’s look at some common pitfalls that can occur when candidates skip this crucial step:
- Misinterpreting the problem: Without proper clarification, you might solve a different problem than the one presented.
- Overlooking edge cases: Failing to consider all possible scenarios can lead to incomplete or buggy solutions.
- Inefficient solutions: You might choose a suboptimal approach if you don’t fully understand the constraints or requirements.
- Wasting time: Realizing halfway through that you misunderstood the problem can leave you scrambling to finish within the time limit.
- Missing opportunities to showcase knowledge: Clarifying questions can sometimes lead to discussions where you can demonstrate your broader understanding of computer science concepts.
The Clarification Process: A Step-by-Step Guide
Now that we understand the importance of clarification, let’s break down the process into actionable steps:
1. Listen Carefully to the Problem Statement
Pay close attention when the interviewer presents the problem. Take notes if necessary, and resist the urge to start thinking about solutions immediately. Focus on understanding the problem statement fully.
2. Repeat the Problem Back to the Interviewer
After the interviewer finishes explaining the problem, summarize it in your own words. This serves two purposes:
- It confirms your understanding of the problem.
- It gives the interviewer a chance to correct any misunderstandings early on.
3. Ask Clarifying Questions
This is the heart of the clarification process. Ask questions about:
- Input format and constraints
- Output expectations
- Edge cases and special scenarios
- Performance requirements
- Any ambiguous terms or concepts in the problem statement
4. Propose Initial Assumptions
Based on the information gathered, state any assumptions you’re making about the problem. This shows your thought process and gives the interviewer another opportunity to provide guidance.
5. Outline Your Approach
Before coding, briefly describe your planned approach to the interviewer. This allows them to provide early feedback and ensures you’re on the right track.
Examples of Good Clarifying Questions
To help you develop this skill, here are some examples of good clarifying questions for different aspects of a coding problem:
Input Format and Constraints
- “What is the expected input format? Is it an array, a string, or something else?”
- “What is the size range of the input? Should I consider very large inputs?”
- “Are there any constraints on the values in the input? For example, are all numbers positive?”
- “Is the input always valid, or should I handle invalid inputs?”
Output Expectations
- “What exactly should the function return? A boolean, an integer, a new data structure?”
- “How should I handle error cases or invalid inputs in the output?”
- “Is there a specific format required for the output, such as rounding for floating-point numbers?”
Edge Cases and Special Scenarios
- “How should the function behave with empty inputs?”
- “Are there any special cases to consider, like duplicate values or extreme values?”
- “Should I handle case sensitivity for string inputs?”
Performance Requirements
- “Are there any specific time or space complexity requirements for the solution?”
- “Should I optimize for time complexity or space complexity if I can’t achieve both?”
- “Is it okay to use additional data structures, or should I solve it in-place?”
Ambiguous Terms or Concepts
- “Can you clarify what you mean by ‘optimal’ in this context?”
- “When you say ‘sorted’, do you mean in ascending or descending order?”
- “Is this a directed or undirected graph?”
Real-World Example: Clarifying a String Manipulation Problem
Let’s walk through a hypothetical interview scenario to see how effective clarification can make a difference:
Interviewer: “Write a function that takes a string and returns the first non-repeating character.”
Candidate: “Thank you for the problem. Before I start coding, I’d like to clarify a few things to ensure I understand the requirements correctly.”
Interviewer: “Of course, go ahead.”
Candidate: “First, let me summarize my understanding of the problem. I need to write a function that takes a string input and returns the first character in that string that doesn’t repeat anywhere else in the string. Is that correct?”
Interviewer: “Yes, that’s correct.”
Candidate: “Great. Now, I have a few questions:
- What should the function return if there are no non-repeating characters?
- Should the function be case-sensitive? For example, is ‘A’ considered the same as ‘a’?
- Can we assume the input will always be a valid string, or should I handle other input types?
- Are there any constraints on the length of the input string?
- Should I consider only alphabetic characters, or should I include numbers and special characters as well?
Interviewer: “Good questions. Let me clarify:
- If there are no non-repeating characters, return null or an empty string, whichever you prefer.
- Yes, the function should be case-sensitive.
- You can assume the input will always be a valid string.
- The input string can be up to 10^5 characters long.
- Consider all characters, including numbers and special characters.”
Candidate: “Thank you for the clarification. Based on this, I’ll make the following assumptions:
- The input will be a non-null string of length 0 to 10^5.
- I’ll treat all characters as distinct, including different cases of the same letter.
- I’ll return null if no non-repeating character is found.
For my approach, I’m thinking of using a hash map to count the occurrences of each character in a single pass through the string. Then, I’ll make a second pass to find the first character with a count of 1. This should give us a time complexity of O(n) and a space complexity of O(k), where k is the number of unique characters in the string. Does this approach sound reasonable?”
Interviewer: “Yes, that sounds like a good approach. Please proceed with the implementation.”
In this example, the candidate demonstrated several key skills:
- Summarizing the problem to confirm understanding
- Asking specific, relevant questions about edge cases and constraints
- Stating assumptions based on the clarifications
- Outlining the planned approach and its complexity before coding
This thorough clarification process sets the stage for a successful implementation and impresses the interviewer with the candidate’s thoughtful approach to problem-solving.
Balancing Clarification and Time Management
While clarification is crucial, it’s also important to manage your time effectively during an interview. Here are some tips to strike the right balance:
- Prioritize your questions: Ask the most critical questions first, in case time runs short.
- Be concise: Frame your questions clearly and concisely to respect the interviewer’s time.
- Listen actively: Pay attention to the interviewer’s responses, as they might answer multiple questions at once.
- Know when to move on: If you feel you have a good understanding of the problem, don’t spend too much time on minor details.
- Use the clarification process to think: While asking questions, start forming ideas about potential solutions.
Developing Your Clarification Skills
Like any skill, the ability to effectively clarify problems improves with practice. Here are some ways to develop this skill:
- Practice with mock interviews: Have friends or mentors give you practice problems and focus on the clarification stage.
- Analyze problem statements: When solving coding problems on your own, make a habit of writing down questions and assumptions before starting.
- Review real interview experiences: Read about others’ interview experiences and note the types of clarifying questions that were beneficial.
- Participate in coding competitions: Many competitive programming platforms have detailed problem statements that require careful reading and interpretation.
- Teach others: Explaining problems to others can help you identify areas that commonly need clarification.
Common Mistakes to Avoid
As you work on improving your clarification skills, be aware of these common mistakes:
- Asking questions answered in the initial problem statement: This suggests you weren’t paying attention.
- Asking too many hypothetical questions: Focus on information relevant to solving the current problem.
- Being too vague: Ask specific questions rather than general ones like “Is there anything else I should know?”
- Arguing with the interviewer: If the interviewer sets a constraint, accept it and work within those boundaries.
- Neglecting to write down key information: Take notes during the clarification process to refer back to while coding.
The Role of Clarification in Problem-Solving Strategies
Clarification is not just a preliminary step; it’s an integral part of your overall problem-solving strategy. Here’s how it fits into the bigger picture:
- Problem Understanding: Clarification helps you fully grasp the problem’s scope and requirements.
- Solution Design: The information gathered during clarification informs your choice of algorithm and data structures.
- Implementation: Clear requirements guide your coding process, helping you handle edge cases and optimize performance.
- Testing: The scenarios and constraints identified during clarification become the basis for your test cases.
- Optimization: Understanding performance requirements helps you refine your solution effectively.
Clarification in Different Types of Coding Interviews
The importance of clarification remains constant across various interview formats, but the approach might differ slightly:
In-Person Whiteboard Interviews
- Use the whiteboard to write down key points from the clarification process.
- Engage in a dialogue with the interviewer, using visual aids if necessary.
Remote Coding Interviews
- Clearly verbalize your questions and understanding, as non-verbal cues may be limited.
- Use the shared coding environment to type out assumptions and key points.
Take-Home Coding Assignments
- If allowed, email the recruiter or interviewer with any clarifying questions.
- Document your assumptions clearly in your code comments or README file.
Conclusion: Mastering the Art of Clarification
Clarifying assumptions and asking questions is not just a preliminary step in coding interviews; it’s a critical skill that can significantly impact your performance and chances of success. By thoroughly understanding the problem, identifying potential pitfalls, and aligning your approach with the interviewer’s expectations, you set yourself up for a smoother coding process and a more impressive overall performance.
Remember, interviewers are not just evaluating your coding skills but also your problem-solving approach, communication abilities, and how you might function as part of their team. Demonstrating a thoughtful, thorough approach to problem clarification showcases these soft skills that are highly valued in the tech industry.
As you prepare for your coding interviews, make clarification an integral part of your practice routine. Approach each problem with a curious and analytical mindset, always seeking to fully understand before diving into code. With time and practice, this process will become second nature, giving you a significant edge in your technical interviews and your future career as a software developer.
Remember, in the world of coding, asking the right questions is often just as important as writing the right code. Master the art of clarification, and you’ll not only excel in interviews but also in real-world software development scenarios where requirements are often ambiguous and subject to change.