Why Building Your Own Framework or Tool is the Best Way to Learn to Code
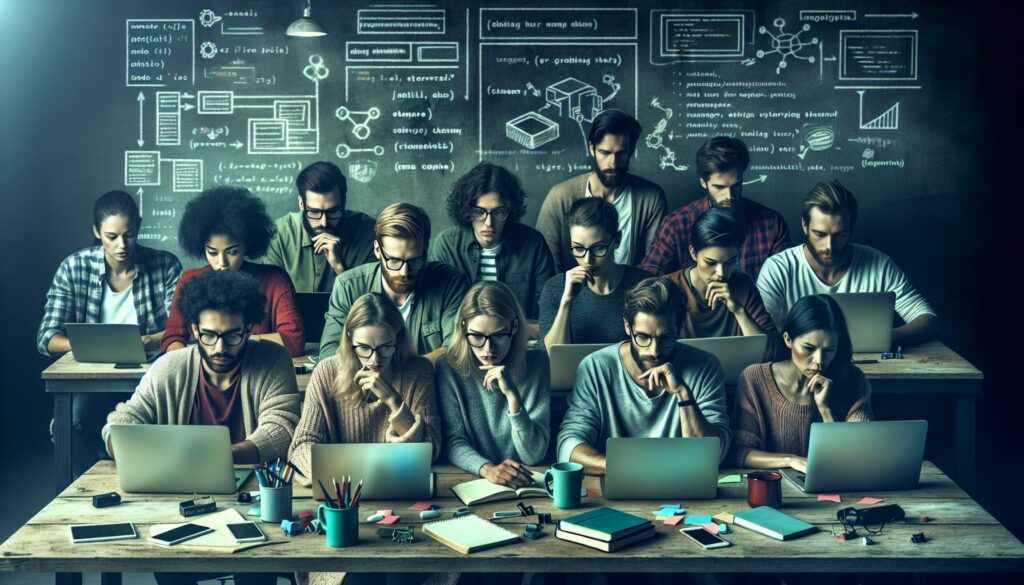
In the vast landscape of coding education, there’s a golden rule that often gets overlooked: the best way to truly master programming is by building your own framework or tool. This approach not only deepens your understanding of coding concepts but also hones your problem-solving skills in ways that traditional learning methods can’t match. In this comprehensive guide, we’ll explore why creating your own framework or tool is an invaluable experience for aspiring developers and how it aligns perfectly with the principles of platforms like AlgoCademy.
The Power of Learning by Doing
Before we dive into the specifics of building frameworks and tools, it’s crucial to understand the fundamental principle behind this approach: learning by doing. This concept is at the heart of effective coding education and is a cornerstone of platforms like AlgoCademy.
Active vs. Passive Learning
When you’re actively engaged in creating something, your brain processes information differently compared to passive learning methods like watching video tutorials or reading documentation. Active learning involves:
- Problem-solving in real-time
- Applying theoretical knowledge to practical situations
- Debugging and troubleshooting issues as they arise
- Making decisions about design and architecture
These activities stimulate deeper cognitive processes, leading to better retention and understanding of coding concepts.
The AlgoCademy Approach
Platforms like AlgoCademy recognize the power of active learning and incorporate it into their teaching methodologies. By providing interactive coding tutorials and AI-powered assistance, they create an environment where learners can experiment, make mistakes, and learn from hands-on experience.
Why Build Your Own Framework or Tool?
Now that we’ve established the importance of active learning, let’s explore why building your own framework or tool is particularly beneficial for aspiring coders.
1. Deep Understanding of Core Concepts
When you build a framework from scratch, you’re forced to understand the underlying principles that make it work. This process exposes you to:
- Design patterns and architectural decisions
- Performance optimization techniques
- Memory management and resource allocation
- API design and usability considerations
For example, if you’re building a web framework, you’ll need to understand HTTP protocols, routing mechanisms, and server-side rendering. This hands-on experience provides insights that are difficult to gain from simply using existing frameworks.
2. Enhanced Problem-Solving Skills
Creating your own tool or framework presents unique challenges that require creative problem-solving. You’ll encounter situations where:
- You need to optimize algorithms for better performance
- You must design intuitive interfaces for other developers
- You have to handle edge cases and unexpected inputs
- You need to balance flexibility with ease of use
These scenarios push you to think critically and develop robust solutions, skills that are invaluable in technical interviews and real-world programming tasks.
3. Improved Debugging and Troubleshooting Abilities
When you’re building something from the ground up, you’ll inevitably encounter bugs and issues. This process of identifying and fixing problems is crucial for developing strong debugging skills. You’ll learn to:
- Use debugging tools effectively
- Read and understand error messages
- Trace code execution to pinpoint issues
- Develop systematic approaches to problem-solving
These skills are essential for any programmer and are often tested in technical interviews at major tech companies.
4. Better Understanding of Existing Frameworks
After building your own framework or tool, you’ll have a much deeper appreciation for existing solutions. You’ll understand:
- Why certain design decisions were made
- The trade-offs between different approaches
- How to leverage advanced features more effectively
- When to use a framework and when to build custom solutions
This knowledge allows you to make more informed decisions when choosing and using frameworks in your future projects.
5. Portfolio Enhancement
A custom-built framework or tool is an impressive addition to your portfolio. It demonstrates:
- Your ability to tackle complex projects
- Your understanding of software architecture
- Your creativity and problem-solving skills
- Your commitment to learning and self-improvement
These qualities are highly valued by employers, especially at top tech companies like those in the FAANG group.
Practical Steps to Build Your Own Framework or Tool
Now that we’ve covered the benefits, let’s look at how you can get started with building your own framework or tool.
1. Choose a Problem to Solve
Start by identifying a problem or inefficiency in your coding workflow. This could be:
- A repetitive task that could be automated
- A gap in functionality in existing tools
- A simplified version of a complex framework for learning purposes
For example, you might decide to build a lightweight CSS framework for rapid prototyping or a command-line tool for managing git repositories.
2. Plan Your Architecture
Before diving into coding, sketch out the overall structure of your framework or tool. Consider:
- The core features you want to implement
- How different components will interact
- The programming paradigms you’ll use (OOP, functional, etc.)
- How users will interact with your tool
This planning phase is crucial for creating a solid foundation for your project.
3. Start with a Minimum Viable Product (MVP)
Begin by implementing the core functionality of your framework or tool. Focus on:
- Creating a basic working version
- Establishing a clear API or interface
- Ensuring your code is modular and extensible
This approach allows you to get something functional quickly and iterate from there.
4. Implement Core Features
With your MVP in place, start adding the main features of your framework or tool. This might involve:
- Writing utility functions
- Implementing data structures
- Creating user interface components
- Developing parsing or processing algorithms
As you work on these features, you’ll encounter challenges that will push your coding skills to new levels.
5. Test Thoroughly
Testing is a critical part of framework and tool development. Implement:
- Unit tests for individual components
- Integration tests for how components work together
- Performance tests to ensure efficiency
- User acceptance tests to verify usability
This process will not only improve the quality of your code but also teach you valuable skills in test-driven development.
6. Document Your Work
Clear documentation is essential for any framework or tool. Practice:
- Writing clear and concise API documentation
- Creating tutorials and examples
- Explaining your design decisions and rationale
- Providing installation and setup instructions
This step is often overlooked by beginners but is crucial for the usability and maintainability of your project.
7. Refactor and Optimize
As your project grows, take time to refactor your code and optimize performance. This involves:
- Identifying and eliminating code smells
- Improving algorithmic efficiency
- Enhancing code readability and maintainability
- Implementing advanced features or optimizations
This process will deepen your understanding of clean code principles and performance optimization techniques.
Real-World Examples and Success Stories
To illustrate the power of building your own frameworks and tools, let’s look at some real-world examples and success stories.
Vue.js: From Personal Project to Popular Framework
Vue.js, now one of the most popular JavaScript frameworks, started as a personal project by Evan You. He created it to solve specific problems he encountered while working at Google. By building Vue.js, Evan:
- Deepened his understanding of reactive programming
- Learned how to design intuitive APIs
- Developed skills in performance optimization
- Built a thriving open-source community
This example shows how a personal project can evolve into a widely-used tool, providing immense learning opportunities along the way.
Lodash: Simplifying JavaScript Development
Lodash, a utility library for JavaScript, was created by John-David Dalton to provide a more consistent and efficient alternative to existing solutions. Through this project, John-David:
- Mastered advanced JavaScript concepts
- Developed a deep understanding of cross-browser compatibility
- Learned how to write highly performant code
- Gained experience in maintaining a large-scale open-source project
Lodash’s success demonstrates how creating a tool to solve common problems can lead to significant learning and career opportunities.
Create React App: Simplifying React Development
Create React App, developed by Facebook engineers, is a tool that simplifies the process of setting up a new React project. By working on this tool, the developers:
- Gained insights into build processes and tooling
- Learned how to create developer-friendly CLIs
- Developed skills in managing complex dependencies
- Improved their understanding of React’s ecosystem
This example shows how creating tools to improve developer workflows can lead to significant learning experiences and contribute to the broader community.
Overcoming Challenges and Common Pitfalls
While building your own framework or tool is an excellent learning experience, it’s not without challenges. Here are some common pitfalls and how to overcome them:
1. Scope Creep
It’s easy to get carried away and try to add too many features. To avoid this:
- Define clear goals and stick to them
- Use an agile approach, implementing features incrementally
- Regularly reassess your project’s scope and priorities
2. Reinventing the Wheel
While the goal is to build something yourself, you don’t need to recreate everything from scratch. It’s okay to:
- Use existing libraries for non-core functionality
- Draw inspiration from existing solutions
- Focus on the unique aspects of your project
3. Perfectionism
Striving for perfection can lead to never-ending projects. Instead:
- Aim for a working solution first, then iterate
- Set deadlines for yourself
- Seek feedback early and often
4. Lack of Documentation
As a learning exercise, it’s crucial to document your work. Make sure to:
- Write documentation as you go
- Explain your thought process and decisions
- Create examples and tutorials for using your framework or tool
Leveraging Your Experience for Career Growth
Building your own framework or tool isn’t just about learning; it can also significantly boost your career prospects. Here’s how to leverage this experience:
1. Showcase Your Project
- Include your framework or tool in your portfolio
- Write blog posts or create videos explaining your project
- Present your work at local meetups or conferences
2. Contribute to Open Source
Use the skills you’ve gained to contribute to open-source projects. This can:
- Further enhance your skills
- Increase your visibility in the developer community
- Provide networking opportunities
3. Prepare for Technical Interviews
The experience of building a framework or tool prepares you well for technical interviews, especially at major tech companies. You’ll be able to:
- Discuss complex technical decisions with confidence
- Demonstrate your problem-solving skills with real-world examples
- Show your ability to design and implement large-scale systems
4. Identify Your Specialization
Through this process, you might discover areas of programming that particularly interest you. Use this insight to:
- Guide your future learning
- Choose job roles that align with your interests
- Develop expertise in specific areas of software development
Conclusion: The Journey of Continuous Learning
Building your own framework or tool is more than just a coding exercise; it’s a journey of continuous learning and self-improvement. This approach embodies the core principles of effective coding education, aligning perfectly with the goals of platforms like AlgoCademy.
By taking on this challenge, you’re not just learning to code; you’re learning to think like a software engineer. You’re developing the problem-solving skills, creativity, and technical depth that are highly valued in the industry, especially by top tech companies.
Remember, the goal isn’t necessarily to create the next Vue.js or Lodash (although that would be great!). The real value lies in the learning process itself. Each bug you fix, each design decision you make, and each optimization you implement contributes to your growth as a developer.
So, whether you’re a beginner looking to deepen your understanding of programming concepts or an experienced developer aiming to sharpen your skills for technical interviews, consider taking on the challenge of building your own framework or tool. It’s a path that leads to true mastery of coding, preparing you for whatever challenges your programming career may bring.
As you embark on this journey, remember that resources like AlgoCademy are there to support you. Use their interactive tutorials, AI-powered assistance, and problem-solving exercises to complement your project work. With dedication, curiosity, and hands-on practice, you’ll not only learn to code but truly master the art of programming.