Why Building Todo Apps Isn’t Teaching You Real Programming
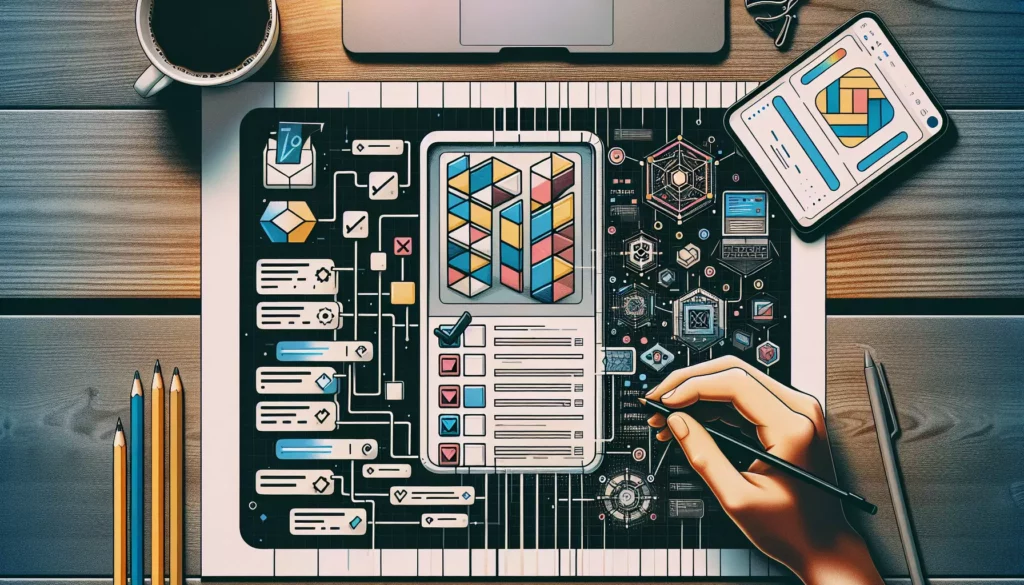
If you’ve ever tried to learn programming, you’ve probably been instructed to build a todo app. It’s practically a rite of passage in the coding world. “Create a simple todo list to understand the basics,” they say. But here’s a controversial take: building todo apps might not be teaching you real programming at all.
Todo apps have become the “Hello World” of modern web development tutorials. They’re seemingly simple enough for beginners but complex enough to demonstrate core concepts. However, the skills you develop while creating these applications often fall short of what you’ll need in professional environments or when tackling real world problems.
In this article, we’ll explore why the ubiquitous todo app might be limiting your growth as a programmer and what alternatives might better prepare you for actual software engineering challenges.
The Limitations of Todo App Tutorials
1. They Focus on UI, Not Logic
Most todo app tutorials spend disproportionate time on styling buttons and positioning elements rather than developing complex logic. While understanding UI is important, real programming challenges often involve deeper algorithmic thinking, data structures, and system design considerations.
Consider this common code snippet from a todo app:
function addTodo() {
const todoText = document.getElementById("todoInput").value;
if(todoText === "") return;
const todoItem = document.createElement("li");
todoItem.innerText = todoText;
todoList.appendChild(todoItem);
document.getElementById("todoInput").value = "";
}
This code handles a basic UI interaction but doesn’t teach you about computational complexity, memory management, or optimizing algorithms—skills that differentiate novice coders from professional engineers.
2. They Oversimplify State Management
In a typical todo app, state management is often trivial. Add an item, delete an item, mark as complete. But real applications deal with complex, interconnected states that require thoughtful architecture to prevent bugs and maintain performance.
Professional applications might need to:
- Handle concurrent state updates from multiple users
- Implement optimistic UI updates with fallback patterns
- Manage deeply nested state objects with careful consideration for immutability
- Implement state machines for complex workflows
These concepts rarely appear in todo app tutorials but are essential for building robust applications.
3. They Rarely Cover Error Handling
Most todo tutorials gloss over proper error handling. In the real world, errors are inevitable, and handling them gracefully is a critical skill. Experienced programmers spend significant time planning for edge cases and failure scenarios.
A more realistic approach would include:
async function fetchTodos() {
try {
const response = await fetch("/api/todos");
if (!response.ok) {
throw new Error(`HTTP error! Status: ${response.status}`);
}
const data = await response.json();
return data;
} catch (error) {
console.error("Failed to fetch todos:", error);
notifyUser("Unable to load your todos. Please try again later.");
return [];
} finally {
hideLoadingIndicator();
}
}
This comprehensive error handling approach is rarely taught in basic tutorials but is standard practice in production code.
What Todo Apps Don’t Teach You
1. Algorithmic Thinking and Problem Solving
The heart of programming isn’t memorizing syntax or frameworks—it’s developing the ability to break down complex problems into solvable parts. Todo apps rarely challenge your algorithmic thinking or require you to optimize for performance.
Consider sorting algorithms. In a professional setting, choosing the right sorting algorithm can make the difference between an application that scales to millions of users and one that crashes under load. Yet todo apps typically use simple array methods without exploring the implications:
// Common todo app approach
todos.sort((a, b) => a.priority - b.priority);
// What you should be learning
function mergeSort(arr, compareFn) {
if (arr.length <= 1) return arr;
const mid = Math.floor(arr.length / 2);
const left = mergeSort(arr.slice(0, mid), compareFn);
const right = mergeSort(arr.slice(mid), compareFn);
return merge(left, right, compareFn);
}
function merge(left, right, compareFn) {
let result = [];
let leftIndex = 0;
let rightIndex = 0;
while (leftIndex < left.length && rightIndex < right.length) {
if (compareFn(left[leftIndex], right[rightIndex]) <= 0) {
result.push(left[leftIndex]);
leftIndex++;
} else {
result.push(right[rightIndex]);
rightIndex++;
}
}
return result.concat(left.slice(leftIndex)).concat(right.slice(rightIndex));
}
Understanding algorithm complexity and when to apply different algorithms is crucial for real world programming but rarely addressed in basic tutorials.
2. System Design and Architecture
Todo apps typically exist as standalone applications with minimal architecture. Real world applications require thoughtful system design, considering factors like:
- Scalability across multiple servers
- Database design and query optimization
- Caching strategies
- Service oriented architecture
- API design principles
These considerations are fundamental to professional software development but rarely appear in beginner tutorials.
3. Testing Strategies
Professional code requires comprehensive testing strategies. Todo app tutorials might introduce basic unit tests, but they rarely cover:
- Integration testing
- End to end testing
- Performance testing
- Mocking and test doubles
- Test driven development workflows
A more realistic testing approach might look like:
// Unit test
describe("Todo Item Component", () => {
it("should toggle completion status when clicked", () => {
const { getByText } = render(<TodoItem text="Test todo" completed={false} onToggle={jest.fn()} />);
const todoElement = getByText("Test todo");
expect(todoElement).not.toHaveClass("completed");
fireEvent.click(todoElement);
expect(onToggle).toHaveBeenCalledTimes(1);
});
it("should apply completed class when todo is completed", () => {
const { getByText } = render(<TodoItem text="Test todo" completed={true} onToggle={jest.fn()} />);
expect(getByText("Test todo")).toHaveClass("completed");
});
});
// Integration test
describe("Todo List Integration", () => {
it("should add new todos and display them", async () => {
const { getByPlaceholderText, getByText, findByText } = render(<TodoApp />);
const input = getByPlaceholderText("Add a todo");
const addButton = getByText("Add");
fireEvent.change(input, { target: { value: "New test todo" } });
fireEvent.click(addButton);
const newTodo = await findByText("New test todo");
expect(newTodo).toBeInTheDocument();
});
});
Comprehensive testing is a critical professional skill that beginners should be exposed to early.
4. Performance Optimization
Todo apps rarely require performance optimization, but real applications need to be fast and efficient. Professional developers regularly consider:
- Memoization and caching
- Virtual DOM optimization
- Code splitting and lazy loading
- Bundle size optimization
- Network request batching
These optimizations can dramatically improve user experience but are rarely covered in beginner tutorials.
Real World Programming Skills You Should Be Learning
1. Data Structures Beyond Arrays
Todo apps typically use simple arrays to store items. In professional development, choosing the right data structure can significantly impact performance and code clarity.
Consider this more advanced approach using a Map for O(1) access time:
class TodoManager {
constructor() {
// Using Map for O(1) access by ID
this.todos = new Map();
this.nextId = 1;
}
addTodo(text, priority = 1) {
const id = this.nextId++;
this.todos.set(id, {
id,
text,
priority,
completed: false,
createdAt: new Date()
});
return id;
}
getTodo(id) {
return this.todos.get(id);
}
updateTodo(id, updates) {
if (!this.todos.has(id)) {
throw new Error(`Todo with id ${id} not found`);
}
const todo = this.todos.get(id);
this.todos.set(id, { ...todo, ...updates });
return this.todos.get(id);
}
deleteTodo(id) {
return this.todos.delete(id);
}
getAllTodos() {
return Array.from(this.todos.values());
}
getCompletedTodos() {
return this.getAllTodos().filter(todo => todo.completed);
}
getPendingTodos() {
return this.getAllTodos().filter(todo => !todo.completed);
}
// Advanced functionality
searchTodos(query) {
const lowercaseQuery = query.toLowerCase();
return this.getAllTodos()
.filter(todo => todo.text.toLowerCase().includes(lowercaseQuery));
}
sortTodosByPriority() {
return this.getAllTodos().sort((a, b) => b.priority - a.priority);
}
}
This implementation demonstrates proper encapsulation and more sophisticated data handling than typical todo app tutorials.
2. Asynchronous Programming Patterns
Basic todo apps might introduce simple callbacks or promises, but professional development requires a deeper understanding of asynchronous patterns:
// Advanced asynchronous patterns
class TodoService {
constructor(apiClient) {
this.apiClient = apiClient;
this.cache = new Map();
}
async getTodos() {
// Implementing caching
if (this.cache.has('todos')) {
console.log('Returning cached todos');
return this.cache.get('todos');
}
try {
const todos = await this.apiClient.get('/todos');
this.cache.set('todos', todos);
return todos;
} catch (error) {
console.error('Failed to fetch todos:', error);
throw error;
}
}
async addTodo(todo) {
try {
// Optimistic update
const optimisticId = `temp-${Date.now()}`;
const optimisticTodo = { ...todo, id: optimisticId, status: 'pending' };
// Update local cache immediately
const currentTodos = this.cache.get('todos') || [];
this.cache.set('todos', [...currentTodos, optimisticTodo]);
// Notify subscribers about the optimistic update
this.notifySubscribers();
// Perform actual API call
const savedTodo = await this.apiClient.post('/todos', todo);
// Replace the optimistic todo with the real one
const updatedTodos = this.cache.get('todos').map(t =>
t.id === optimisticId ? savedTodo : t
);
this.cache.set('todos', updatedTodos);
// Notify subscribers about the confirmed update
this.notifySubscribers();
return savedTodo;
} catch (error) {
// Rollback the optimistic update
const currentTodos = this.cache.get('todos');
this.cache.set('todos', currentTodos.filter(t => !t.id.startsWith('temp-')));
// Notify subscribers about the rollback
this.notifySubscribers();
console.error('Failed to add todo:', error);
throw error;
}
}
}
This implementation demonstrates professional patterns like optimistic updates, caching, and proper error handling that go far beyond basic tutorials.
3. Security Considerations
Professional developers must consider security at every level of application development, something rarely covered in todo app tutorials:
// Security considerations in a todo API
const express = require('express');
const helmet = require('helmet');
const rateLimit = require('express-rate-limit');
const xss = require('xss');
const { body, validationResult } = require('express-validator');
const app = express();
// Basic security headers
app.use(helmet());
// Rate limiting to prevent brute force attacks
const apiLimiter = rateLimit({
windowMs: 15 * 60 * 1000, // 15 minutes
max: 100, // limit each IP to 100 requests per windowMs
message: 'Too many requests from this IP, please try again later'
});
app.use('/api/', apiLimiter);
// Parse JSON bodies
app.use(express.json());
// Create a new todo with input validation
app.post('/api/todos', [
// Validate and sanitize input
body('title').trim().isLength({ min: 1, max: 100 }).escape(),
body('description').optional().trim().escape(),
body('completed').isBoolean(),
], (req, res) => {
// Check for validation errors
const errors = validationResult(req);
if (!errors.isEmpty()) {
return res.status(400).json({ errors: errors.array() });
}
// Additional XSS protection
const sanitizedTitle = xss(req.body.title);
const sanitizedDescription = req.body.description ? xss(req.body.description) : '';
// Process the todo...
res.status(201).json({ message: 'Todo created successfully' });
});
Security considerations like these are essential in professional applications but rarely taught in beginner tutorials.
Better Alternatives to Todo Apps for Learning
1. Build a Data Structure Visualizer
Instead of a todo app, consider building a tool that visualizes common data structures like linked lists, trees, or graphs. This project would require:
- Understanding complex data structures and their operations
- Implementing rendering algorithms
- Handling user interactions to modify the structures
- Animating transitions between states
This type of project forces you to understand both the theoretical aspects of data structures and practical implementation details.
2. Create a Simplified Database
Building a simplified database system teaches fundamental concepts used in professional programming:
- File system operations and persistent storage
- Indexing and query optimization
- Transaction management
- Concurrency control
Even a basic implementation would cover far more real world programming concepts than a todo app.
3. Develop a Real Time Collaboration Tool
Building a simple collaborative editor or whiteboard teaches critical concepts:
- WebSockets and real time communication
- Conflict resolution algorithms
- State synchronization across clients
- Operational transformation or CRDT algorithms
These concepts appear frequently in professional applications but are rarely covered in beginner tutorials.
4. Build a Custom State Management Library
Instead of using existing state management solutions, try building your own simplified version. This project would teach:
- Reactive programming patterns
- Event emitter implementation
- Immutability and state tracking
- Time travel debugging
Understanding how these tools work under the hood will make you a much stronger developer than simply using them in a todo app.
How AlgoCademy Approaches Coding Education Differently
At AlgoCademy, we believe learning to code should go beyond creating simple CRUD applications. Our approach focuses on developing fundamental algorithmic thinking and problem solving skills that translate to real world engineering challenges.
Emphasis on Algorithmic Thinking
Instead of memorizing syntax or following cookie cutter tutorials, AlgoCademy teaches you how to think like a programmer. Our curriculum includes:
- Deep dives into essential data structures and algorithms
- Problem decomposition techniques
- Complexity analysis and optimization strategies
- Pattern recognition in programming problems
These skills form the foundation of software engineering and prepare you for challenges beyond basic web development.
Interactive Problem Solving
Our platform provides interactive coding challenges that progress from fundamental concepts to complex problems similar to those used in technical interviews at major tech companies.
Each challenge is designed to:
- Reinforce specific algorithms or data structures
- Provide immediate feedback on your solution
- Offer hints and guidance when you get stuck
- Show optimized solutions after you successfully solve a problem
This approach builds confidence through incremental progress while ensuring you develop robust problem solving skills.
AI Powered Assistance
Our AI assistant provides personalized guidance as you work through problems, offering:
- Targeted hints based on your specific approach
- Identification of logical errors in your code
- Suggestions for optimization when your solution works but could be improved
- Explanations of complex concepts tailored to your current understanding
This personalized approach ensures you're never completely stuck while still allowing you to develop independent problem solving skills.
Real World System Design
Beyond algorithms, we teach system design principles that prepare you for building complex, scalable applications:
- Distributed systems architecture
- Database design and query optimization
- Caching strategies and performance optimization
- API design and microservices architecture
These topics bridge the gap between academic computer science and practical software engineering.
Conclusion: Beyond Todo Apps
While todo apps can introduce basic programming concepts, they fall short of teaching the skills needed for professional software development. Real programming involves complex problem solving, algorithmic thinking, and system design considerations that go far beyond simple CRUD operations.
If you're serious about becoming a proficient programmer, challenge yourself with projects that:
- Force you to understand and implement complex algorithms
- Require thoughtful data structure selection
- Present non trivial architectural challenges
- Deal with concurrent or distributed systems
At AlgoCademy, we've designed our curriculum to build these exact skills, preparing you not just to pass coding interviews but to excel as a software engineer in real world environments.
Remember, the goal isn't to memorize syntax or follow tutorials—it's to develop the problem solving mindset that allows you to tackle novel challenges confidently. By focusing on algorithmic thinking and fundamental computer science principles, you'll build a foundation that will serve you throughout your programming career, regardless of which languages or frameworks you ultimately use.
So the next time someone suggests building a todo app as a learning exercise, consider whether there might be a more challenging project that would better develop your programming skills. Your future self will thank you for it.