Why Brute Force Solutions Can Be a Good Starting Point
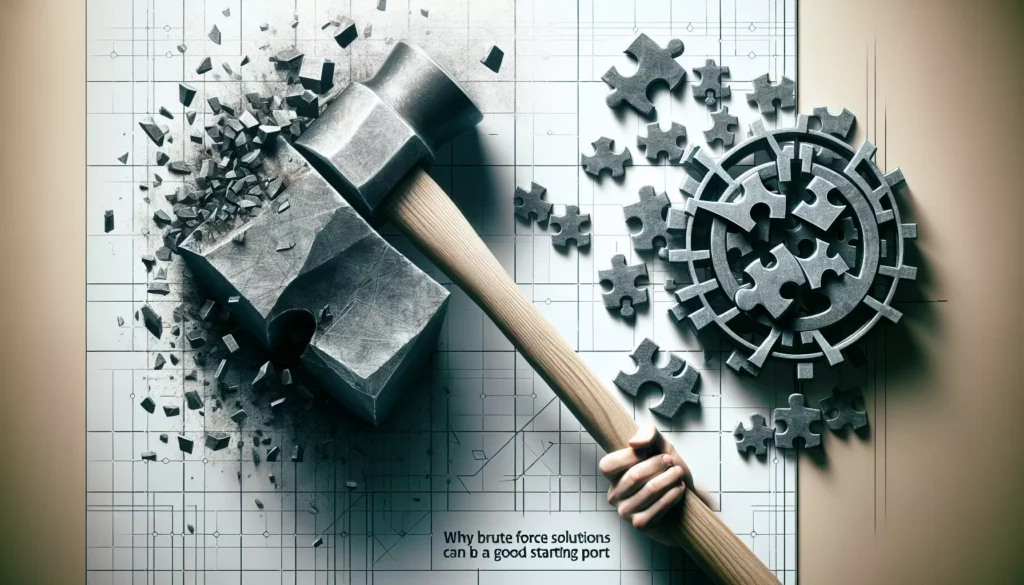
In the world of programming and algorithm design, we often hear about the importance of efficiency and optimization. While these are undoubtedly crucial aspects of writing good code, there’s a powerful argument to be made for starting with brute force solutions. This approach, often overlooked or dismissed as naive, can actually be an excellent starting point for problem-solving, especially for those learning to code or tackling new types of challenges.
What is a Brute Force Solution?
Before we dive into the benefits of brute force solutions, let’s clarify what we mean by the term. A brute force solution is an approach to problem-solving that involves systematically enumerating all possible candidates for the solution and checking whether each candidate satisfies the problem’s statement.
In simpler terms, it’s a straightforward method that tries all possibilities until a satisfactory solution is found. While this approach can be computationally expensive and time-consuming for complex problems, it has several advantages, especially in the learning and development process.
The Benefits of Starting with Brute Force
1. Simplicity and Clarity
One of the primary advantages of brute force solutions is their simplicity. They are often the most intuitive and straightforward way to approach a problem. This simplicity makes them easier to understand, implement, and debug, which is particularly beneficial for beginners or when tackling a new type of problem.
For example, consider the problem of finding the maximum element in an array. A brute force solution might look like this:
def find_max(arr):
max_element = arr[0]
for i in range(1, len(arr)):
if arr[i] > max_element:
max_element = arr[i]
return max_element
This solution is clear, easy to understand, and directly translates the problem statement into code.
2. Guaranteed Correctness
Brute force solutions, by their nature, explore all possible options. This exhaustive search guarantees that if a solution exists, it will be found. This certainty can be incredibly valuable, especially when you’re still grappling with the problem and aren’t sure about the correctness of more optimized approaches.
3. Baseline for Optimization
Starting with a brute force solution provides a solid baseline for further optimization. Once you have a working (albeit potentially slow) solution, you can measure its performance and identify bottlenecks. This information is crucial for understanding where and how to optimize.
For instance, if you’re solving a sorting problem, you might start with a simple bubble sort (a brute force approach). While inefficient for large datasets, it gives you a working solution and helps you understand the problem better. From there, you can explore more efficient algorithms like quicksort or mergesort.
4. Problem Understanding
Implementing a brute force solution often requires a thorough understanding of the problem. As you work through all possible cases, you gain insights into the problem’s structure and constraints. This deep understanding is invaluable when it comes to developing more sophisticated solutions later.
5. Debugging and Testing
Brute force solutions, due to their simplicity, are often easier to debug. When something goes wrong, it’s usually straightforward to trace the execution and find the issue. Additionally, these solutions can serve as excellent tools for generating test cases and verifying the correctness of more optimized algorithms.
When to Use Brute Force Approaches
While brute force solutions have their advantages, it’s important to recognize when they are appropriate. Here are some scenarios where starting with a brute force approach can be particularly beneficial:
1. Learning and Practice
For those learning to code or practicing problem-solving skills, brute force solutions are an excellent starting point. They allow you to focus on translating the problem into code without getting bogged down in complex optimizations.
2. Small Input Sizes
When dealing with small input sizes, the performance difference between a brute force solution and an optimized one might be negligible. In such cases, the simplicity of the brute force approach can be preferable.
3. Prototyping
In the early stages of development or when exploring a new problem domain, brute force solutions can help you quickly prototype and validate your ideas.
4. Verification
Brute force solutions can serve as a reference implementation to verify the correctness of more complex, optimized algorithms.
Moving Beyond Brute Force
While brute force solutions are a great starting point, it’s important to recognize their limitations and know when to move beyond them. Here are some steps to transition from brute force to more optimized solutions:
1. Analyze Time and Space Complexity
Once you have a working brute force solution, analyze its time and space complexity. This analysis will help you understand the solution’s limitations and identify areas for improvement.
2. Identify Patterns and Redundancies
Look for patterns or redundant computations in your brute force solution. Often, these insights can lead to more efficient algorithms.
3. Apply Problem-Solving Techniques
Explore various problem-solving techniques such as dynamic programming, divide and conquer, or greedy algorithms to optimize your solution.
4. Use Data Structures
Consider how different data structures might help improve the efficiency of your solution. For example, using a hash table might significantly speed up lookup operations compared to a linear search.
5. Learn from Existing Algorithms
Study well-known algorithms and data structures. Understanding these can often provide insights into how to optimize your own solutions.
Case Study: The Two Sum Problem
Let’s look at a practical example to illustrate the progression from a brute force solution to an optimized one. Consider the Two Sum problem: given an array of integers and a target sum, find two numbers in the array that add up to the target.
Brute Force Solution
Here’s a brute force approach to the Two Sum problem:
def two_sum_brute_force(nums, target):
for i in range(len(nums)):
for j in range(i + 1, len(nums)):
if nums[i] + nums[j] == target:
return [i, j]
return []
This solution checks every possible pair of numbers in the array. It’s simple to understand and implement, but has a time complexity of O(n^2).
Optimized Solution
Now, let’s optimize this using a hash table:
def two_sum_optimized(nums, target):
num_dict = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_dict:
return [num_dict[complement], i]
num_dict[num] = i
return []
This optimized solution uses a hash table to store the numbers we’ve seen so far, allowing us to find complements in constant time. It reduces the time complexity to O(n).
The Learning Process
Starting with the brute force solution allowed us to:
- Understand the problem clearly
- Implement a correct (though inefficient) solution
- Identify the bottleneck (nested loops)
- Recognize the need for faster lookup
This understanding then guided us towards the optimized solution using a hash table.
Balancing Brute Force and Optimization in Coding Education
In the context of coding education and platforms like AlgoCademy, the role of brute force solutions is particularly significant. Here’s why:
1. Building Confidence
For beginners, being able to solve a problem, even with a brute force approach, builds confidence. It’s important for learners to know that they can solve problems, even if their initial solutions aren’t the most efficient.
2. Incremental Learning
Starting with brute force and then optimizing allows for incremental learning. Students can first focus on correctly translating problem statements into code, then learn about efficiency and optimization techniques.
3. Problem-Solving Skills
The process of moving from a brute force solution to an optimized one develops critical problem-solving skills. It teaches students to analyze their code, identify inefficiencies, and think creatively about improvements.
4. Interview Preparation
In technical interviews, especially for roles at major tech companies, it’s often valuable to start with a brute force solution. This demonstrates to the interviewer that you can quickly come up with a working solution, and provides a starting point for discussing optimizations.
Conclusion
While the ultimate goal in programming is often to create efficient, optimized solutions, the importance of brute force approaches shouldn’t be underestimated. They serve as an excellent starting point, particularly in the learning process and when tackling new problems.
Brute force solutions offer simplicity, guaranteed correctness, and a solid foundation for optimization. They help in building a deeper understanding of the problem and provide a clear baseline for improvement. For beginners and experienced programmers alike, starting with a brute force approach can be a valuable step in the problem-solving process.
As you continue your coding journey, remember that every complex algorithm started as a simpler idea. Embrace brute force solutions as a learning tool, a stepping stone to more sophisticated algorithms, and a way to build your problem-solving skills. With practice and persistence, you’ll develop the ability to both implement straightforward solutions and optimize them effectively.
In the world of coding education and platforms like AlgoCademy, the journey from brute force to optimization is not just about learning algorithms—it’s about developing a mindset. It’s about understanding problems deeply, approaching them systematically, and continuously improving your solutions. So the next time you face a challenging coding problem, don’t hesitate to start with a brute force approach. It might just be the first step towards a brilliant, optimized solution.