Why Binary Search is Important for Problem Solving in Coding Interviews
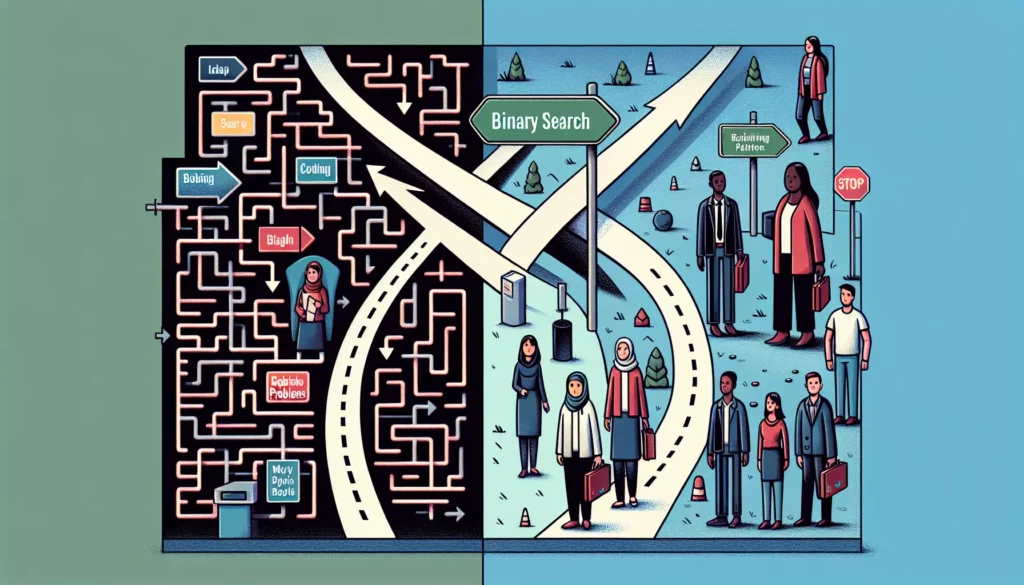
When it comes to coding interviews, particularly those at prestigious tech companies like FAANG (Facebook, Amazon, Apple, Netflix, and Google), having a strong grasp of fundamental algorithms is crucial. Among these algorithms, binary search stands out as a particularly important concept. In this comprehensive guide, we’ll explore why binary search is so vital for problem-solving in coding interviews and how mastering this algorithm can significantly boost your chances of success.
What is Binary Search?
Before diving into the importance of binary search, let’s briefly review what it is. Binary search is an efficient algorithm for finding an item from a sorted list of items. It works by repeatedly dividing the search interval in half. If the value of the searched item is less than the item in the middle of the interval, narrow the interval to the lower half. Otherwise, narrow it to the upper half. Repeatedly check until the value is found or the interval is empty.
Here’s a simple implementation of binary search in Python:
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1 # Target not found
Why is Binary Search Important in Coding Interviews?
Now that we’ve refreshed our understanding of binary search, let’s explore why it’s so crucial for coding interviews:
1. Efficiency and Time Complexity
One of the primary reasons binary search is essential in coding interviews is its efficiency. Binary search has a time complexity of O(log n), which is significantly faster than linear search’s O(n) for large datasets. In interviews, you’re often asked to optimize solutions, and knowing when and how to apply binary search can dramatically improve your algorithm’s performance.
For example, consider a problem where you need to find a specific element in a sorted array of 1 million elements:
- Linear search: In the worst case, it might take 1 million comparisons.
- Binary search: It would take at most 20 comparisons (log₂ 1,000,000 ≈ 19.93).
This efficiency becomes even more critical when dealing with large datasets or when your algorithm needs to perform multiple searches.
2. Versatility in Problem Solving
While the basic binary search algorithm is straightforward, its concept can be applied to solve a wide variety of problems. Many interview questions can be solved or optimized using binary search or its variations. Some examples include:
- Finding the first or last occurrence of an element in a sorted array
- Searching in a rotated sorted array
- Finding the peak element in a bitonic array
- Minimizing the maximum or maximizing the minimum in certain optimization problems
Understanding binary search deeply allows you to recognize these patterns and apply the concept creatively to solve complex problems.
3. Demonstrating Algorithmic Thinking
Using binary search in your solutions demonstrates to interviewers that you understand fundamental algorithmic concepts and can apply them effectively. It shows that you’re thinking about efficiency and can optimize your code, which are crucial skills for any software engineer.
4. Foundation for More Advanced Algorithms
Binary search serves as a foundation for more advanced algorithms and data structures. Understanding binary search well makes it easier to grasp concepts like:
- Binary Search Trees
- Divide and Conquer algorithms
- Logarithmic time complexity analysis
These advanced topics often come up in more challenging interview questions, especially at top tech companies.
5. Handling Edge Cases
Implementing binary search correctly requires careful consideration of edge cases, such as:
- Empty arrays
- Arrays with one element
- Searching for elements not present in the array
- Handling integer overflow in the mid-point calculation
Demonstrating your ability to handle these edge cases shows attention to detail and robust coding practices, which are highly valued in coding interviews.
Common Binary Search Variations in Interviews
To further illustrate the importance of binary search, let’s look at some common variations and problems that often appear in coding interviews:
1. Finding the First and Last Occurrence
This problem involves finding the first and last occurrence of a target element in a sorted array. It’s a slight modification of the standard binary search but requires careful handling of the boundaries.
def find_first_occurrence(arr, target):
left, right = 0, len(arr) - 1
result = -1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
result = mid
right = mid - 1 # Continue searching in the left half
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return result
def find_last_occurrence(arr, target):
left, right = 0, len(arr) - 1
result = -1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
result = mid
left = mid + 1 # Continue searching in the right half
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return result
2. Search in Rotated Sorted Array
This problem involves searching for a target value in a sorted array that has been rotated at some pivot point. It’s a classic example of how binary search can be adapted to more complex scenarios.
def search_rotated(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
# Check which half is sorted
if arr[left] <= arr[mid]:
if arr[left] <= target < arr[mid]:
right = mid - 1
else:
left = mid + 1
else:
if arr[mid] < target <= arr[right]:
left = mid + 1
else:
right = mid - 1
return -1 # Target not found
3. Finding the Peak Element
A peak element is an element that is greater than its neighbors. This problem demonstrates how binary search can be applied to problems that aren’t strictly about searching for a specific value.
def find_peak_element(arr):
left, right = 0, len(arr) - 1
while left < right:
mid = (left + right) // 2
if arr[mid] < arr[mid + 1]:
left = mid + 1
else:
right = mid
return left # Peak element index
Tips for Mastering Binary Search for Interviews
Now that we’ve seen the importance and versatility of binary search, here are some tips to help you master this algorithm for your coding interviews:
1. Practice Implementation
Implement binary search from scratch multiple times. Make sure you can write the basic algorithm without referring to any resources. Practice both recursive and iterative implementations.
2. Understand the Invariants
Binary search relies on maintaining certain invariants (conditions that remain true throughout the algorithm). Understanding these invariants is crucial for correctly implementing and modifying binary search. The key invariant in binary search is that the target, if it exists, is always within the current search range.
3. Master the Edge Cases
Pay special attention to edge cases. Practice with arrays of different sizes, including empty arrays and arrays with one or two elements. Also, consider cases where the target element is not present in the array.
4. Learn Common Variations
Familiarize yourself with common variations of binary search, such as finding the first or last occurrence, searching in a rotated array, or finding a peak element. Understanding these variations will help you recognize when and how to apply binary search in more complex problems.
5. Analyze Time and Space Complexity
Be prepared to discuss the time and space complexity of your binary search implementation. Understand why binary search has a time complexity of O(log n) and a space complexity of O(1) for iterative implementation or O(log n) for recursive implementation due to the call stack.
6. Practice Problem Recognition
Train yourself to recognize problems where binary search can be applied, even when it’s not immediately obvious. Look for sorted data or problems involving searching or optimizing over a range of values.
7. Implement in Multiple Languages
If you’re comfortable with multiple programming languages, practice implementing binary search in each of them. This will help you become more versatile and prepared for interviews that may require coding in a specific language.
8. Understand the Limitations
While binary search is powerful, it’s important to understand its limitations. It requires sorted data and random access to elements (which rules out certain data structures like linked lists). Being aware of when binary search is not applicable is just as important as knowing when to use it.
Real-world Applications of Binary Search
Understanding the real-world applications of binary search can help you appreciate its importance beyond coding interviews. Here are some practical uses of binary search:
1. Database Systems
Binary search is fundamental in database indexing. When you query a database for a specific record, the database often uses a binary search tree or a variation of binary search to quickly locate the desired information.
2. Version Control Systems
Git’s bisect command, which helps find the commit that introduced a bug, uses a binary search algorithm to efficiently narrow down the problematic commit.
3. Computer Networking
In computer networking, binary search is used in routing tables to efficiently look up the best route for a packet to reach its destination.
4. Machine Learning
Some machine learning algorithms, like decision trees, use binary search-like approaches to make decisions and classifications.
5. Image Processing
In image processing and computer vision, binary search can be used in algorithms for edge detection or image segmentation.
6. Operating Systems
Operating systems use binary search in memory management, particularly in free space management algorithms.
Conclusion
Binary search is a fundamental algorithm that plays a crucial role in problem-solving, particularly in coding interviews. Its efficiency, versatility, and wide range of applications make it an essential tool in any programmer’s toolkit. By mastering binary search and its variations, you’ll not only be better prepared for coding interviews but also equipped to solve real-world problems more efficiently.
Remember, the key to mastering binary search is consistent practice and application. Challenge yourself with various problems, implement different variations, and always strive to recognize scenarios where binary search can be applied. With dedication and practice, you’ll find that binary search becomes an intuitive and powerful tool in your problem-solving arsenal.
As you prepare for your coding interviews, especially for top tech companies, make sure to give binary search the attention it deserves. It’s not just about memorizing the algorithm; it’s about understanding its underlying principles and learning to apply it creatively to solve complex problems. With a solid grasp of binary search, you’ll be well on your way to impressing interviewers and tackling challenging coding problems with confidence.