Why Am I Failing Coding Interviews Even After Preparing? A Comprehensive Guide to Success
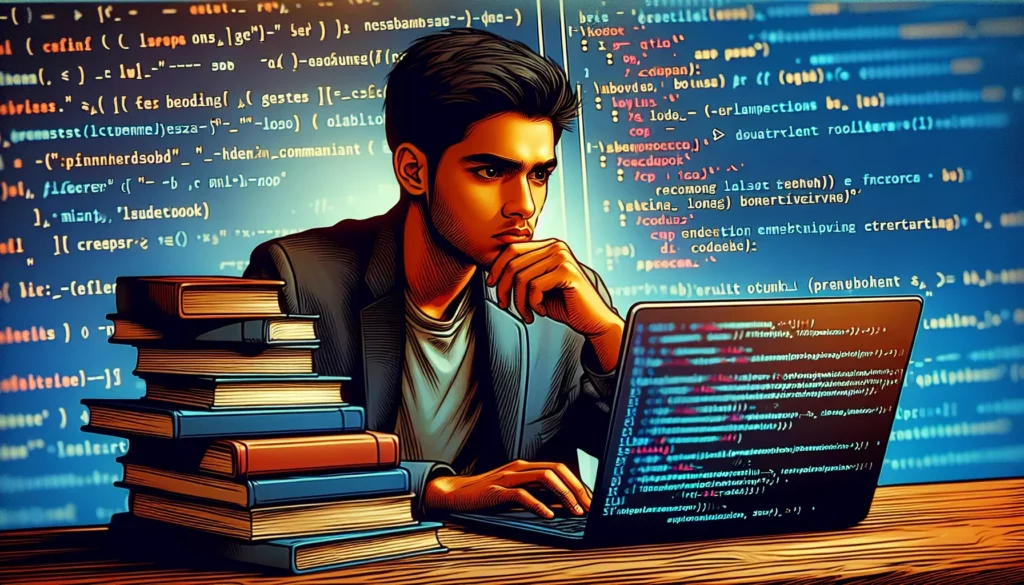
Preparing for coding interviews can be an arduous and time-consuming process. You’ve spent countless hours studying data structures, algorithms, and solving LeetCode problems. Yet, when it comes to the actual interview, you find yourself stumbling and ultimately not getting the job offer. If this sounds familiar, you’re not alone. Many aspiring developers face this frustrating situation, but there’s hope. In this comprehensive guide, we’ll explore the reasons why you might be failing coding interviews despite your preparation and provide actionable strategies to improve your performance.
Understanding the Root Causes
Before we dive into solutions, it’s essential to identify the potential reasons for your interview struggles. Here are some common factors that might be holding you back:
- Insufficient breadth of knowledge
- Lack of deep understanding
- Poor problem-solving approach
- Weak communication skills
- Interview anxiety and nervousness
- Inadequate real-world coding experience
- Inability to handle edge cases and optimize solutions
- Lack of familiarity with the specific company’s interview process
Now that we’ve identified potential issues, let’s explore each one in detail and discuss strategies to overcome them.
1. Insufficient Breadth of Knowledge
While you may have focused on certain areas of computer science, coding interviews often cover a wide range of topics. Ensure you have a solid foundation in the following areas:
- Data Structures: Arrays, Linked Lists, Stacks, Queues, Trees, Graphs, Hash Tables
- Algorithms: Sorting, Searching, Dynamic Programming, Greedy Algorithms
- Time and Space Complexity Analysis
- Object-Oriented Programming concepts
- Database fundamentals and SQL
- System Design basics
- Operating Systems concepts
- Networking fundamentals
Action Plan:
- Create a study schedule that covers all these topics
- Use resources like AlgoCademy, LeetCode, HackerRank, and Coursera to learn and practice
- Read books like “Cracking the Coding Interview” and “Introduction to Algorithms”
- Join online communities and forums to discuss and learn from others
2. Lack of Deep Understanding
Memorizing solutions to common problems is not enough. Interviewers want to see that you truly understand the underlying concepts and can apply them to new situations.
Action Plan:
- Focus on understanding the “why” behind each solution
- Implement data structures and algorithms from scratch
- Explain concepts to others (use the Feynman Technique)
- Solve variations of problems you’ve already tackled
- Analyze multiple solutions for each problem and understand their trade-offs
3. Poor Problem-Solving Approach
Your approach to solving problems is just as important as arriving at the correct solution. Interviewers want to see your thought process and how you break down complex problems.
Action Plan:
- Practice the following problem-solving steps:
- Understand the problem thoroughly
- Clarify any ambiguities
- Identify the inputs and outputs
- Consider edge cases and constraints
- Brainstorm potential approaches
- Choose the most appropriate solution
- Implement the solution step-by-step
- Test and optimize your code
- Use platforms like AlgoCademy that provide interactive coding tutorials and AI-powered assistance to improve your problem-solving skills
- Practice thinking out loud while solving problems
- Time yourself to simulate interview conditions
4. Weak Communication Skills
Technical skills alone are not enough. Your ability to communicate your thoughts clearly and collaborate with the interviewer is crucial.
Action Plan:
- Practice explaining your approach before writing any code
- Use clear and concise language
- Ask clarifying questions when needed
- Engage with the interviewer and be open to feedback
- Practice mock interviews with friends or mentors
- Record yourself solving problems and review your communication
5. Interview Anxiety and Nervousness
Nervousness can significantly impact your performance during an interview. It’s essential to develop strategies to manage stress and stay calm under pressure.
Action Plan:
- Practice relaxation techniques like deep breathing and meditation
- Familiarize yourself with common interview formats and questions
- Conduct mock interviews in a simulated environment
- Focus on your strengths and past successes
- Prepare a pre-interview routine to help you feel grounded
- Remember that it’s okay to take a moment to collect your thoughts during the interview
6. Inadequate Real-World Coding Experience
While solving algorithm problems is important, having practical coding experience is equally valuable. Many interviewers look for candidates who can apply their skills to real-world scenarios.
Action Plan:
- Work on personal projects or contribute to open-source projects
- Participate in hackathons or coding competitions
- Build a portfolio showcasing your projects
- Practice coding in a professional IDE or text editor
- Familiarize yourself with version control systems like Git
- Learn about software development best practices and design patterns
7. Inability to Handle Edge Cases and Optimize Solutions
Interviewers often look for candidates who can not only solve the problem but also consider edge cases and optimize their solutions for better performance.
Action Plan:
- Always consider edge cases when solving problems (e.g., empty inputs, large inputs, negative numbers)
- Practice analyzing time and space complexity of your solutions
- Learn common optimization techniques (e.g., memoization, two-pointer approach)
- Challenge yourself to improve the efficiency of your initial solutions
- Study and implement different data structures to understand their performance characteristics
8. Lack of Familiarity with the Specific Company’s Interview Process
Each company has its own unique interview process and culture. Being familiar with these can give you a significant advantage.
Action Plan:
- Research the company’s interview process on sites like Glassdoor and LeetCode
- Practice problems that are frequently asked by the company
- Understand the company’s values and culture
- Prepare relevant questions to ask your interviewers
- Review the job description thoroughly and align your preparation accordingly
Leveraging AlgoCademy for Interview Success
AlgoCademy is an excellent resource for improving your coding skills and preparing for technical interviews. Here’s how you can make the most of it:
- Interactive Tutorials: Take advantage of AlgoCademy’s step-by-step coding tutorials to reinforce your understanding of key concepts and algorithms.
- AI-Powered Assistance: Utilize the platform’s AI features to get personalized feedback on your code and problem-solving approach.
- Comprehensive Curriculum: Follow AlgoCademy’s structured learning path to ensure you cover all essential topics for coding interviews.
- Practice Problems: Solve a variety of coding challenges on the platform to improve your problem-solving skills and familiarize yourself with different question types.
- Progress Tracking: Use AlgoCademy’s progress tracking features to identify areas where you need improvement and focus your study efforts accordingly.
Implementing a Structured Preparation Plan
To maximize your chances of success in coding interviews, it’s crucial to have a well-structured preparation plan. Here’s a sample 12-week plan to help you get started:
Weeks 1-4: Building a Strong Foundation
- Review and implement basic data structures (Arrays, Linked Lists, Stacks, Queues)
- Study and practice fundamental algorithms (Sorting, Searching)
- Solve easy to medium difficulty problems on AlgoCademy and LeetCode
- Start reading “Cracking the Coding Interview”
Weeks 5-8: Advancing Your Skills
- Deep dive into advanced data structures (Trees, Graphs, Hash Tables)
- Learn and implement more complex algorithms (Dynamic Programming, Greedy Algorithms)
- Practice medium to hard difficulty problems
- Begin studying system design concepts
- Start working on a personal project to gain practical experience
Weeks 9-12: Interview Preparation and Practice
- Conduct regular mock interviews with friends or online platforms
- Focus on communicating your thought process clearly
- Review and optimize solutions to previously solved problems
- Study company-specific interview questions and processes
- Practice solving problems under timed conditions
Common Pitfalls to Avoid
As you prepare for coding interviews, be aware of these common mistakes:
- Overlooking the importance of communication: Remember that your ability to explain your thought process is just as important as solving the problem.
- Neglecting to practice on a whiteboard or shared doc: Many interviews require coding without the help of an IDE. Practice writing code by hand or in a simple text editor.
- Focusing solely on problem-solving: While important, don’t forget to brush up on computer science fundamentals and system design concepts.
- Memorizing solutions without understanding: Aim to understand the underlying principles rather than memorizing specific solutions.
- Neglecting behavioral interview preparation: Many companies include behavioral questions. Prepare stories that highlight your skills and experiences.
- Not asking for clarification: Don’t hesitate to ask questions to fully understand the problem before diving into a solution.
- Giving up too quickly: If you’re stuck, try to work through the problem step-by-step. Interviewers often want to see how you handle challenges.
The Importance of Continuous Learning and Improvement
Remember that becoming proficient at coding interviews is a journey, not a destination. Even if you face setbacks, each interview is an opportunity to learn and improve. Here are some tips for continuous improvement:
- Reflect on each interview experience: After each interview, take time to reflect on what went well and what could be improved.
- Seek feedback: Whenever possible, ask for feedback from your interviewers or recruiters.
- Stay updated with industry trends: The tech industry evolves rapidly. Stay informed about new technologies and best practices.
- Engage with the developer community: Participate in coding forums, attend meetups, or contribute to open-source projects to expand your network and knowledge.
- Teach others: Explaining concepts to others can deepen your own understanding and highlight areas where you need more study.
Conclusion
Failing coding interviews can be disheartening, but it’s important to remember that even experienced developers face challenges in the interview process. By identifying your weaknesses, implementing a structured preparation plan, and continuously refining your skills, you can significantly improve your chances of success.
Leverage resources like AlgoCademy to guide your learning journey, practice consistently, and approach each interview as an opportunity to showcase your skills and learn something new. With persistence and the right strategies, you can overcome your interview struggles and land your dream job in the tech industry.
Remember, the key to success lies not just in what you know, but in how you apply that knowledge and communicate your thought process. Stay motivated, keep learning, and approach each interview with confidence. Your dedication will pay off, and you’ll find yourself better equipped to tackle even the most challenging coding interviews.