Why Algorithmic Thinking is Like Cooking: Follow a Recipe, Then Experiment
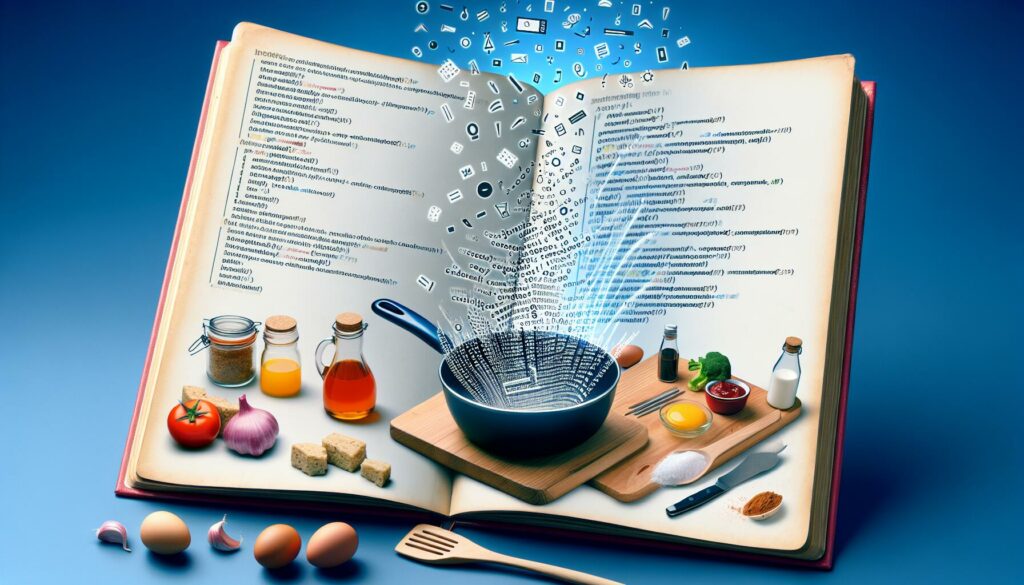
In the world of programming and computer science, algorithmic thinking is a fundamental skill that sets apart great developers from the rest. But for many beginners, the concept can seem daunting and abstract. What if we told you that algorithmic thinking is a lot like something you probably do every week – cooking? In this article, we’ll explore the fascinating parallels between algorithmic thinking and culinary arts, and how understanding this connection can help you become a better programmer.
The Recipe: Your First Algorithm
When you’re learning to cook a new dish, you typically start with a recipe. This step-by-step guide tells you exactly what ingredients you need and how to combine them to create the desired outcome. Similarly, when you’re learning a new algorithm, you often begin with a set of instructions or pseudocode that outlines the steps needed to solve a particular problem.
Let’s look at a simple example. Imagine you’re cooking spaghetti bolognese for the first time. Your recipe might look something like this:
- Boil water in a large pot
- Add spaghetti and cook for 8-10 minutes
- Meanwhile, brown ground beef in a pan
- Add chopped onions and garlic to the beef
- Pour in tomato sauce and simmer for 15 minutes
- Drain the spaghetti
- Serve the spaghetti with the sauce on top
Now, let’s look at a simple algorithm for finding the maximum number in an array:
1. Set max_number to the first element of the array
2. For each element in the array:
a. If the current element is greater than max_number:
i. Set max_number to the current element
3. Return max_number
Notice how both the cooking recipe and the algorithm provide a clear, step-by-step process to achieve a specific goal. They both start with initial conditions (ingredients or input), provide a series of actions to take, and result in a final output (a meal or a solution to a problem).
Understanding the Ingredients: Variables and Data Types
In cooking, understanding your ingredients is crucial. You need to know what each ingredient does, how it interacts with others, and how it contributes to the final dish. Similarly, in programming, understanding variables and data types is fundamental to algorithmic thinking.
Let’s break this down:
- Ingredients in cooking = Variables in programming
- Types of ingredients (e.g., protein, carbohydrate, vegetable) = Data types (e.g., integer, string, boolean)
- Quantity of ingredients = Value assigned to variables
Just as you wouldn’t use sugar instead of salt in a savory dish, you wouldn’t use a string variable where an integer is needed in your code. Understanding the ‘ingredients’ of your algorithm is crucial for its success.
The Cooking Process: Control Flow in Algorithms
The process of cooking often involves a series of steps that must be performed in a specific order. This is very similar to the control flow in algorithms. Let’s explore some common programming constructs and their culinary counterparts:
1. Sequencing
In cooking, you often need to perform steps in a specific order. For example, you need to boil the water before adding pasta. In programming, this is known as sequencing – executing instructions in a specific order.
// Sequencing in code
boil_water();
add_pasta();
cook_for_8_minutes();
2. Conditionals (If-Else Statements)
In cooking, you might have instructions like “If the sauce is too thick, add more water.” This is similar to if-else statements in programming.
// Conditional in code
if (sauce_consistency == "too thick") {
add_water();
} else {
continue_cooking();
}
3. Loops
When a recipe says “Stir continuously for 5 minutes,” that’s essentially a loop. In programming, we use loops to repeat actions.
// Loop in code
for (int i = 0; i < 5; i++) {
stir_sauce();
wait_one_minute();
}
4. Functions
In cooking, you might have sub-recipes or common techniques that you use across different dishes. In programming, we call these functions – reusable blocks of code that perform specific tasks.
// Function in code
function chop_vegetables() {
chop_onions();
chop_garlic();
chop_tomatoes();
}
Experimentation: Optimizing and Improving Algorithms
Once you’ve mastered the basic recipe, both in cooking and in algorithmic thinking, it’s time to experiment and optimize. This is where the real creativity and problem-solving skills come into play.
Tweaking the Recipe
In cooking, you might experiment by adding new spices, changing cooking times, or substituting ingredients. Similarly, in algorithmic thinking, you can optimize your code by:
- Improving time complexity (making your algorithm faster)
- Reducing space complexity (using less memory)
- Enhancing readability and maintainability
- Adding new features or functionality
Let’s look at an example. Remember our simple algorithm for finding the maximum number in an array? We could optimize it like this:
// Original algorithm
function findMax(arr) {
let max_number = arr[0];
for (let i = 1; i < arr.length; i++) {
if (arr[i] > max_number) {
max_number = arr[i];
}
}
return max_number;
}
// Optimized algorithm
function findMaxOptimized(arr) {
return Math.max(...arr);
}
The optimized version uses JavaScript’s built-in Math.max() function with the spread operator, which is both more concise and potentially more efficient for large arrays.
Handling Edge Cases
In cooking, you might need to adjust your recipe for different dietary requirements or available ingredients. Similarly, in algorithmic thinking, you need to consider edge cases – unusual or extreme inputs that could cause your algorithm to fail.
For our maximum number algorithm, some edge cases to consider might be:
- An empty array
- An array with only one element
- An array with all elements being the same
- An array with very large numbers that might cause overflow
Here’s how we might handle these edge cases:
function findMaxRobust(arr) {
if (arr.length === 0) {
throw new Error("Cannot find maximum of an empty array");
}
return Math.max(...arr);
}
The Importance of Practice
Just as you become a better cook through practice, you become better at algorithmic thinking by solving many problems. Platforms like AlgoCademy provide a wealth of coding challenges and tutorials to help you hone your skills.
Here are some tips for effective practice:
- Start simple: Begin with basic algorithms and gradually increase complexity.
- Understand before optimizing: Make sure you understand how an algorithm works before trying to optimize it.
- Learn from others: Study different solutions to the same problem. There’s often more than one way to solve a problem efficiently.
- Apply to real-world problems: Try to relate algorithms to real-world scenarios. This can help in understanding their practical applications.
- Reflect on your process: After solving a problem, think about how you approached it. What worked well? What could be improved?
Beyond the Recipe: Creative Problem-Solving
While following recipes (or established algorithms) is a great way to start, the real power of both cooking and algorithmic thinking lies in creative problem-solving. Once you understand the basic principles, you can start to innovate and create your own solutions.
In cooking, this might mean creating a fusion dish that combines elements from different cuisines. In algorithmic thinking, it could involve combining different algorithmic techniques to solve a complex problem.
For example, let’s say you’re tasked with creating an algorithm to find the most frequent item in a very large dataset. You might combine a hash table for counting occurrences with a max heap for efficiently tracking the most frequent items. This kind of creative combination of techniques is what sets apart great problem-solvers.
The Role of Tools and Techniques
Both cooking and algorithmic thinking benefit from a range of tools and techniques that can make the process more efficient and effective.
Cooking Tools and Programming Tools
In cooking, you might use tools like:
- A sharp knife for efficient chopping
- A food processor for quick preparation
- A pressure cooker for faster cooking
Similarly, in programming, you have tools like:
- Integrated Development Environments (IDEs) for efficient coding
- Debuggers for finding and fixing errors
- Version control systems for managing code changes
Just as a good chef knows when to use each kitchen tool, a skilled programmer knows which programming tools to use in different situations.
Cooking Techniques and Algorithm Techniques
Cooking techniques like sautéing, braising, or roasting each have their place in creating different dishes. Similarly, algorithmic techniques like divide-and-conquer, dynamic programming, or greedy algorithms are suited to different types of problems.
For instance:
- Divide-and-conquer is like breaking down a complex dish into simpler components. Think of making a multi-course meal where each course is prepared separately.
- Dynamic programming is similar to meal prep, where you prepare components in advance to save time later.
- Greedy algorithms are like making quick decisions in the kitchen based on what’s immediately available, without considering all possible options.
The Importance of Efficiency
In both cooking and algorithmic thinking, efficiency is key. A professional chef can prepare a complex dish much faster than a novice, not because they’re rushing, but because they’ve optimized their process. Similarly, an experienced programmer can write more efficient algorithms that solve problems faster and use fewer resources.
In algorithmic thinking, we often talk about time complexity (how long an algorithm takes to run) and space complexity (how much memory it uses). These concepts are crucial for writing efficient code, especially when dealing with large datasets.
Let’s look at an example. Suppose we want to find if a number exists in a sorted array. A simple approach would be to check every element:
function linearSearch(arr, target) {
for (let i = 0; i < arr.length; i++) {
if (arr[i] === target) return true;
}
return false;
}
This has a time complexity of O(n), where n is the number of elements in the array. But since we know the array is sorted, we can use a more efficient binary search algorithm:
function binarySearch(arr, target) {
let left = 0;
let right = arr.length - 1;
while (left <= right) {
let mid = Math.floor((left + right) / 2);
if (arr[mid] === target) return true;
if (arr[mid] < target) left = mid + 1;
else right = mid - 1;
}
return false;
}
This binary search algorithm has a time complexity of O(log n), which is much more efficient for large arrays.
The Joy of Problem-Solving
Perhaps the most important parallel between cooking and algorithmic thinking is the joy of problem-solving. Just as a chef feels satisfaction in creating a delicious meal from raw ingredients, a programmer experiences a sense of accomplishment in solving a complex problem with elegant code.
Both activities involve creativity, analytical thinking, and a dash of intuition. They require you to break down complex problems into manageable steps, experiment with different approaches, and continuously learn and improve your skills.
Conclusion: From Kitchen to Keyboard
As we’ve explored in this article, algorithmic thinking shares many similarities with cooking. Both start with following recipes or established algorithms, then progress to experimentation and creative problem-solving. Both require an understanding of your tools and ingredients, a grasp of fundamental techniques, and a focus on efficiency and optimization.
By understanding these parallels, you can approach algorithmic thinking with a familiar mindset. Just as you might experiment in the kitchen to create new and exciting dishes, don’t be afraid to experiment with your code. Try different approaches, combine various techniques, and always be open to learning and improving.
Remember, becoming proficient in algorithmic thinking, like becoming a great cook, takes time and practice. But with persistence and the right approach, you can develop this crucial skill and become a more effective problem-solver and programmer.
So the next time you’re struggling with a coding problem, take a step back and think about how you might approach a similar challenge in the kitchen. You might just find that your culinary intuition can guide your algorithmic thinking in surprising and effective ways.
Happy coding, and bon appétit!