Which Programming Language Should I Learn First? A Comprehensive Guide for Beginners
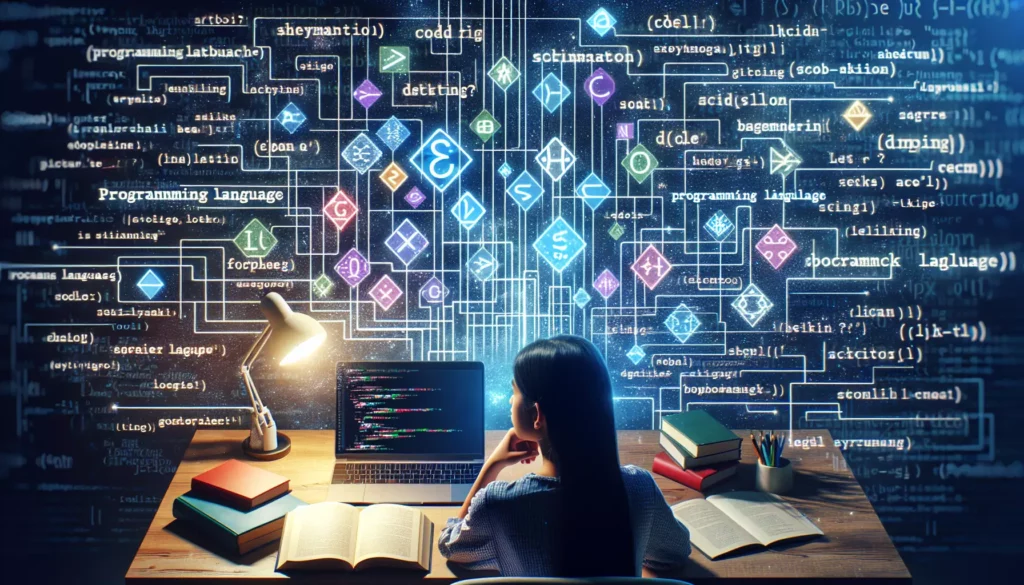
Embarking on your coding journey is an exciting adventure, but it often starts with a crucial question: “Which programming language should I learn first?” This decision can feel overwhelming, given the vast array of options available. In this comprehensive guide, we’ll explore the factors to consider when choosing your first programming language and provide insights into some of the most popular languages for beginners.
Table of Contents
- Why Your First Programming Language is Important
- Factors to Consider When Choosing
- Popular Programming Languages for Beginners
- Python: The Versatile Choice
- JavaScript: The Web Development Powerhouse
- Java: The Enterprise-Level Language
- C#: The Microsoft Ecosystem Language
- Ruby: The Developer-Friendly Language
- Swift: The Apple Ecosystem Language
- Go: The Modern Systems Programming Language
- Comparison of Beginner-Friendly Languages
- Learning Resources and Platforms
- Conclusion: Making Your Choice
Why Your First Programming Language is Important
Your first programming language serves as the foundation for your coding journey. It shapes your understanding of programming concepts, problem-solving approaches, and even your future career path. While experienced programmers can easily switch between languages, your initial choice can significantly impact your learning curve and early successes.
Remember, though, that the principles of programming are universal. Once you grasp the fundamentals in one language, transitioning to others becomes much easier. The key is to start with a language that aligns with your goals and learning style.
Factors to Consider When Choosing
Before diving into specific languages, let’s explore the key factors you should consider when making your choice:
- Your Goals: Are you interested in web development, mobile apps, data science, or game development? Different languages are better suited for different purposes.
- Job Market Demand: Consider which languages are in high demand in your target job market or industry.
- Learning Curve: Some languages are more beginner-friendly than others. Consider starting with a language known for its readability and simplicity.
- Community and Resources: A large, active community means more tutorials, forums, and resources to help you learn.
- Versatility: Some languages are more versatile and can be used across various domains, which can be beneficial for beginners.
- Future Prospects: Consider the language’s growth trajectory and its potential relevance in the coming years.
Popular Programming Languages for Beginners
Now, let’s explore some of the most popular programming languages for beginners, their strengths, and ideal use cases.
Python: The Versatile Choice
Python has become increasingly popular as a first programming language, and for good reason. Its simple, readable syntax makes it an excellent choice for beginners.
Pros of Python:
- Easy to read and write, with a syntax that resembles plain English
- Versatile, used in web development, data science, AI, machine learning, and more
- Large, supportive community with abundant learning resources
- Extensive library of pre-built modules and frameworks
Cons of Python:
- Slower execution speed compared to compiled languages
- Not ideal for mobile app development
Example Python Code:
# A simple Python program to calculate the factorial of a number
def factorial(n):
if n == 0 or n == 1:
return 1
else:
return n * factorial(n-1)
number = 5
result = factorial(number)
print(f"The factorial of {number} is {result}")
JavaScript: The Web Development Powerhouse
JavaScript is the language of the web and an excellent choice for those interested in front-end or full-stack web development.
Pros of JavaScript:
- Essential for web development, both front-end and back-end (Node.js)
- Can be used for mobile app development (React Native, Ionic)
- Huge ecosystem with numerous libraries and frameworks
- Immediate visual feedback when working on web projects
Cons of JavaScript:
- Can be quirky with some counterintuitive behaviors
- Frequent updates and changes in best practices
Example JavaScript Code:
// A simple JavaScript program to calculate the factorial of a number
function factorial(n) {
if (n === 0 || n === 1) {
return 1;
} else {
return n * factorial(n - 1);
}
}
const number = 5;
const result = factorial(number);
console.log(`The factorial of ${number} is ${result}`);
Java: The Enterprise-Level Language
Java is a popular choice for large-scale enterprise applications and Android app development.
Pros of Java:
- Platform independence (“Write Once, Run Anywhere”)
- Strong typing helps catch errors early
- Extensive libraries and frameworks
- High demand in enterprise environments
Cons of Java:
- More verbose syntax compared to Python or JavaScript
- Steeper learning curve for beginners
Example Java Code:
// A simple Java program to calculate the factorial of a number
public class Factorial {
public static int factorial(int n) {
if (n == 0 || n == 1) {
return 1;
} else {
return n * factorial(n - 1);
}
}
public static void main(String[] args) {
int number = 5;
int result = factorial(number);
System.out.println("The factorial of " + number + " is " + result);
}
}
C#: The Microsoft Ecosystem Language
C# (pronounced “C-sharp”) is a versatile language developed by Microsoft, primarily used for Windows desktop applications and game development with Unity.
Pros of C#:
- Versatile, used for desktop, web, and game development
- Strong integration with the Microsoft ecosystem
- Cleaner and more modern syntax compared to C++
- Popular for game development with Unity
Cons of C#:
- Primarily Windows-focused, though cross-platform development is possible
- Steeper learning curve compared to Python or JavaScript
Example C# Code:
// A simple C# program to calculate the factorial of a number
using System;
class Factorial
{
static int CalculateFactorial(int n)
{
if (n == 0 || n == 1)
return 1;
else
return n * CalculateFactorial(n - 1);
}
static void Main()
{
int number = 5;
int result = CalculateFactorial(number);
Console.WriteLine($"The factorial of {number} is {result}");
}
}
Ruby: The Developer-Friendly Language
Ruby is known for its simplicity and readability, making it a popular choice for beginners and experienced developers alike.
Pros of Ruby:
- Clean, readable syntax that’s easy for beginners to understand
- Strong focus on developer happiness and productivity
- Popular web development framework (Ruby on Rails)
- Active and supportive community
Cons of Ruby:
- Slower performance compared to some other languages
- Less popular for areas outside of web development
Example Ruby Code:
# A simple Ruby program to calculate the factorial of a number
def factorial(n)
if n == 0 || n == 1
1
else
n * factorial(n - 1)
end
end
number = 5
result = factorial(number)
puts "The factorial of #{number} is #{result}"
Swift: The Apple Ecosystem Language
Swift is Apple’s programming language for iOS, macOS, watchOS, and tvOS app development.
Pros of Swift:
- Modern, safe, and fast language design
- Essential for iOS and macOS app development
- Strong support from Apple and growing community
- Interoperable with Objective-C
Cons of Swift:
- Limited to Apple’s ecosystem (though server-side Swift is emerging)
- Frequent language updates can lead to compatibility issues
Example Swift Code:
// A simple Swift program to calculate the factorial of a number
func factorial(_ n: Int) -> Int {
if n == 0 || n == 1 {
return 1
} else {
return n * factorial(n - 1)
}
}
let number = 5
let result = factorial(number)
print("The factorial of \(number) is \(result)")
Go: The Modern Systems Programming Language
Go, developed by Google, is gaining popularity for its simplicity and efficiency in building scalable network applications and microservices.
Pros of Go:
- Simple and easy to learn, especially for those with C-family language experience
- Excellent for concurrent and parallel programming
- Fast compilation and execution
- Growing popularity in cloud and network services
Cons of Go:
- Less versatile compared to languages like Python or JavaScript
- Smaller ecosystem compared to more established languages
Example Go Code:
// A simple Go program to calculate the factorial of a number
package main
import "fmt"
func factorial(n int) int {
if n == 0 || n == 1 {
return 1
}
return n * factorial(n-1)
}
func main() {
number := 5
result := factorial(number)
fmt.Printf("The factorial of %d is %d\n", number, result)
}
Comparison of Beginner-Friendly Languages
Language | Ease of Learning | Versatility | Job Market Demand | Community Support |
---|---|---|---|---|
Python | High | High | High | Excellent |
JavaScript | Medium | High | High | Excellent |
Java | Medium | High | High | Excellent |
C# | Medium | High | High | Good |
Ruby | High | Medium | Medium | Good |
Swift | Medium | Medium | Medium | Good |
Go | Medium | Medium | Growing | Good |
Learning Resources and Platforms
Regardless of the language you choose, there are numerous resources available to help you learn. Here are some popular platforms and resources:
- Online Coding Platforms:
- Codecademy: Interactive coding lessons for various languages
- freeCodeCamp: Free, comprehensive web development courses
- LeetCode: Platform for practicing coding problems and preparing for technical interviews
- HackerRank: Coding challenges and competitions
- Video Courses:
- Coursera: University-level courses on various programming topics
- edX: Courses from top institutions worldwide
- Udemy: Wide range of affordable programming courses
- Books and Documentation:
- Official language documentation
- “Head First” series for various programming languages
- “Learn Python the Hard Way” by Zed Shaw (for Python learners)
- Community Resources:
- Stack Overflow: Q&A platform for programming-related questions
- GitHub: Platform for sharing and collaborating on code
- Reddit programming communities (e.g., r/learnprogramming, r/python, r/javascript)
Conclusion: Making Your Choice
Choosing your first programming language is an important decision, but remember that it’s just the beginning of your coding journey. Here are some final tips to help you make your choice:
- Align with Your Goals: Choose a language that aligns with your immediate goals and interests. If web development excites you, JavaScript might be the way to go. If you’re interested in data science or AI, Python could be your best bet.
- Consider Job Prospects: If you’re learning to enhance your career, research the job market in your area or desired industry to see which languages are in demand.
- Start with Something Approachable: For absolute beginners, starting with a language known for its simplicity (like Python or Ruby) can help build confidence and fundamental programming concepts.
- Leverage Available Resources: Consider the learning resources available to you. Some languages have more comprehensive, beginner-friendly resources than others.
- Don’t Overthink It: While your first language is important, remember that many programmers learn multiple languages throughout their careers. The most important thing is to start learning and building projects.
Ultimately, the best programming language to learn first is the one that you’ll stick with and enjoy learning. Each language has its strengths, and there’s no one-size-fits-all answer. Whether you choose Python for its versatility, JavaScript for web development, Java for enterprise applications, or any other language, the key is to start coding, practice regularly, and build projects that interest you.
Remember, programming is a journey of continuous learning. As you progress, you’ll likely explore multiple languages and find the ones that best suit your evolving interests and career goals. The most important step is to begin your coding adventure with enthusiasm and persistence. Happy coding!