When to Use Memoization vs Tabulation: A Comprehensive Guide
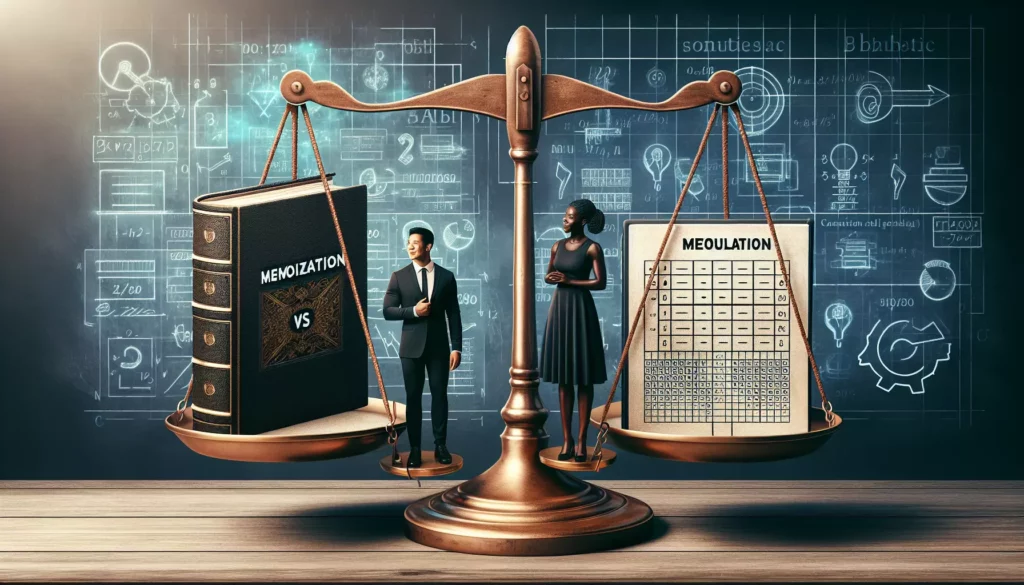
In the world of dynamic programming, two powerful techniques stand out: memoization and tabulation. Both are essential tools in a programmer’s arsenal, especially when tackling complex algorithmic problems. But when should you use one over the other? This comprehensive guide will dive deep into the nuances of memoization and tabulation, helping you make informed decisions in your coding journey.
Understanding Dynamic Programming
Before we delve into the specifics of memoization and tabulation, it’s crucial to understand the concept of dynamic programming (DP). Dynamic programming is a method for solving complex problems by breaking them down into simpler subproblems. It’s particularly useful when the subproblems overlap and when the problem has an optimal substructure.
The core idea behind dynamic programming is to store the results of expensive function calls and return the cached result when the same inputs occur again. This approach can significantly reduce the time complexity of algorithms, often from exponential to polynomial time.
Memoization: Top-Down Approach
Memoization is a top-down approach to dynamic programming. It involves caching the results of expensive function calls and returning the cached result when the same inputs occur again. This technique is particularly useful when you have a recursive solution to a problem and want to optimize it.
How Memoization Works
In memoization, you start with the top-level problem and break it down into subproblems. As you solve each subproblem, you store its result in a cache (usually a hash table or an array). Before solving any subproblem, you first check if its result is already in the cache. If it is, you return the cached result instead of recalculating it.
Example: Fibonacci Sequence with Memoization
Let’s look at a classic example of memoization: calculating the nth Fibonacci number.
def fibonacci(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fibonacci(n-1, memo) + fibonacci(n-2, memo)
return memo[n]
print(fibonacci(100)) # This would be extremely slow without memoization
In this example, we use a dictionary memo
to store the results of previously calculated Fibonacci numbers. This dramatically reduces the time complexity from O(2^n) to O(n).
Advantages of Memoization
- Intuitive implementation for recursive problems
- Only calculates needed values
- Easier to implement for complex problems with multiple parameters
- Can be more space-efficient for sparse problems
Disadvantages of Memoization
- Can lead to stack overflow for deeply recursive problems
- May have slightly higher overhead due to recursive calls
- Not as straightforward for problems that require all subproblems to be solved
Tabulation: Bottom-Up Approach
Tabulation is a bottom-up approach to dynamic programming. Instead of starting with the final problem and working backwards, tabulation begins by solving the smallest subproblems and uses their solutions to build up to the final problem.
How Tabulation Works
In tabulation, you typically use a table (usually an array) to store the results of subproblems. You start by filling in the solutions for the base cases, then use these to compute solutions for progressively larger subproblems until you reach the solution to the original problem.
Example: Fibonacci Sequence with Tabulation
Let’s implement the Fibonacci sequence using tabulation:
def fibonacci(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
print(fibonacci(100)) # This is very fast and doesn't risk stack overflow
In this implementation, we use an array dp
to store all Fibonacci numbers up to n. We start with the base cases and iteratively build up to the nth number.
Advantages of Tabulation
- Usually more efficient as it avoids recursive calls
- Eliminates the risk of stack overflow
- Often easier to analyze time and space complexity
- Better for problems that require computing all subproblems
Disadvantages of Tabulation
- May compute unnecessary subproblems
- Can be less intuitive to implement for some problems
- May use more space if many subproblems are unnecessary
When to Use Memoization
Memoization is often the preferred choice in the following scenarios:
- Recursive Problems: When you have a natural recursive solution to a problem, memoization can be an intuitive way to optimize it.
- Sparse Subproblems: If only a small subset of all possible subproblems needs to be solved, memoization can be more space-efficient.
- Top-Down Analysis: When it’s more natural to think about the problem from the top down, memoization can be easier to implement and understand.
- Multiple Parameters: For problems with multiple changing parameters, memoization can be easier to implement as you can use tuples as keys in your memoization cache.
- Quick Prototyping: Memoization often allows for quicker initial implementation, making it useful for prototyping or coding interviews.
Example: Longest Common Subsequence
The Longest Common Subsequence (LCS) problem is a classic example where memoization shines. Here’s an implementation:
def lcs(X, Y, m, n, memo={}):
if (m, n) in memo:
return memo[(m, n)]
if m == 0 or n == 0:
result = 0
elif X[m-1] == Y[n-1]:
result = 1 + lcs(X, Y, m-1, n-1, memo)
else:
result = max(lcs(X, Y, m, n-1, memo), lcs(X, Y, m-1, n, memo))
memo[(m, n)] = result
return result
X = "ABCDGH"
Y = "AEDFHR"
print(lcs(X, Y, len(X), len(Y))) # Output: 3
This problem naturally lends itself to a recursive solution, and memoization effectively optimizes it by caching results of subproblems.
When to Use Tabulation
Tabulation is often the better choice in these scenarios:
- Iterative Approach: When the problem can be easily solved iteratively from the bottom up, tabulation is often more straightforward.
- Avoiding Stack Overflow: For problems with a large input size that might cause stack overflow with recursion, tabulation is safer.
- Optimization: When you need to squeeze out every bit of performance, tabulation often has less overhead.
- All Subproblems Required: If you need to compute all subproblems anyway, tabulation ensures you do this in the most efficient order.
- Space Optimization: In some cases, tabulation allows for easy space optimization where you only need to keep track of a few previous states.
Example: Coin Change Problem
The Coin Change problem is a great example where tabulation is particularly effective. Here’s an implementation:
def coin_change(coins, amount):
dp = [float('inf')] * (amount + 1)
dp[0] = 0
for coin in coins:
for x in range(coin, amount + 1):
dp[x] = min(dp[x], dp[x - coin] + 1)
return dp[amount] if dp[amount] != float('inf') else -1
coins = [1, 2, 5]
amount = 11
print(coin_change(coins, amount)) # Output: 3
This problem is naturally solved from the bottom up, making tabulation an excellent choice. It also requires computing all subproblems, which tabulation handles efficiently.
Comparing Memoization and Tabulation
Let’s compare memoization and tabulation across several key factors:
Factor | Memoization | Tabulation |
---|---|---|
Approach | Top-down | Bottom-up |
Implementation | Recursive | Iterative |
Subproblem Solving | On-demand | Systematic |
Code Complexity | Often simpler for recursive problems | Can be complex for multi-dimensional problems |
Space Efficiency | Can be more efficient for sparse problems | Uses predetermined space |
Stack Overflow Risk | Higher risk for deep recursion | No risk |
Speed | Slightly slower due to recursive calls | Generally faster |
Hybrid Approaches
In some cases, you might find it beneficial to combine aspects of both memoization and tabulation. These hybrid approaches can leverage the strengths of both techniques.
Iterative Memoization
This approach uses an iterative structure like tabulation but only computes necessary values like memoization. It’s useful when you want to avoid recursion but still benefit from on-demand computation.
Example: Matrix Chain Multiplication
Here’s an example of a hybrid approach for the Matrix Chain Multiplication problem:
def matrix_chain_order(p):
n = len(p) - 1
m = [[0 for _ in range(n)] for _ in range(n)]
for L in range(2, n + 1):
for i in range(n - L + 1):
j = i + L - 1
m[i][j] = float('inf')
for k in range(i, j):
q = m[i][k] + m[k+1][j] + p[i] * p[k+1] * p[j+1]
if q < m[i][j]:
m[i][j] = q
return m[0][n-1]
arr = [40, 20, 30, 10, 30]
print(matrix_chain_order(arr)) # Output: 26000
This implementation uses a tabulation-like structure but computes values in a way similar to memoization, filling the table diagonally.
Real-World Applications
Understanding when to use memoization vs tabulation is crucial in many real-world scenarios:
1. Bioinformatics
In DNA sequence alignment, memoization is often used due to the sparse nature of the problem – not all subsequences need to be compared.
2. Financial Modeling
Option pricing models often use tabulation as they require computing all intermediate states.
3. Game Development
Pathfinding algorithms in games might use memoization to cache results of frequently queried paths.
4. Machine Learning
Dynamic programming techniques are used in various ML algorithms. For instance, Hidden Markov Models often employ tabulation for the Viterbi algorithm.
Best Practices and Tips
To effectively choose between memoization and tabulation, consider these best practices:
- Analyze the Problem Structure: Understand if the problem naturally lends itself to a top-down or bottom-up approach.
- Consider Space Complexity: If memory is a constraint, evaluate which approach will be more space-efficient for your specific problem.
- Evaluate Time Constraints: For time-critical applications, tabulation might offer better performance.
- Think About Maintainability: Choose the approach that results in more readable and maintainable code for your team.
- Profile Your Code: When in doubt, implement both approaches and profile them with real data to make an informed decision.
Conclusion
Choosing between memoization and tabulation is not always a clear-cut decision. It depends on the specific problem, the constraints of your environment, and sometimes personal or team preference. Memoization shines in recursive scenarios and when dealing with sparse subproblems, while tabulation excels in iterative approaches and when all subproblems need to be solved.
As you gain more experience with dynamic programming, you’ll develop an intuition for which approach to use. Remember, the goal is to write efficient, readable, and maintainable code. Sometimes, the best approach might even be a hybrid of the two techniques.
By mastering both memoization and tabulation, you’ll be well-equipped to tackle a wide range of algorithmic challenges, whether you’re preparing for technical interviews, optimizing critical systems, or solving complex real-world problems. Keep practicing, and don’t hesitate to experiment with both approaches to deepen your understanding of dynamic programming.