When to Use BFS vs DFS in Graph Problems: A Comprehensive Guide
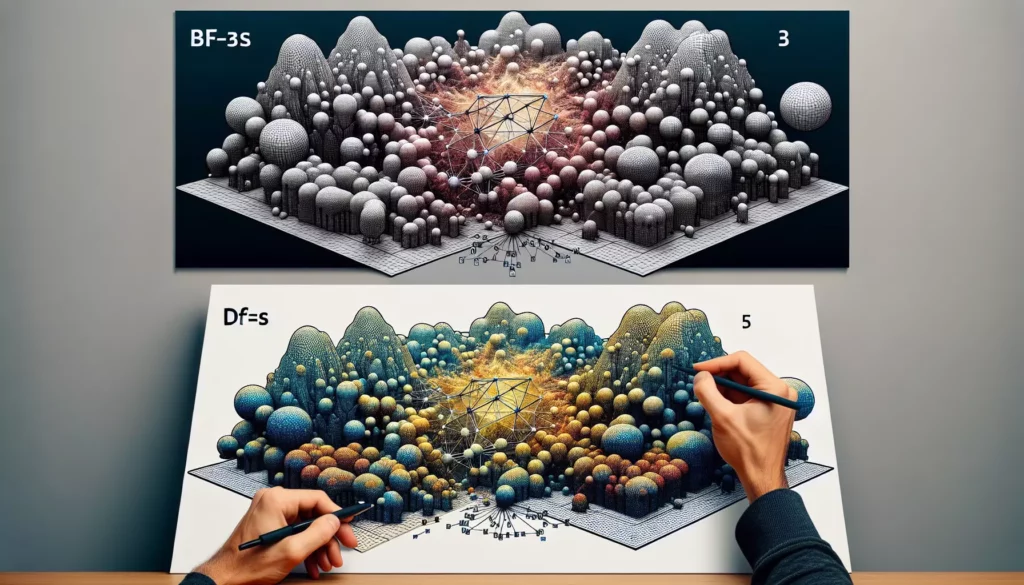
Graph traversal algorithms are fundamental tools in computer science and are extensively used in solving various real-world problems. Two of the most common graph traversal techniques are Breadth-First Search (BFS) and Depth-First Search (DFS). While both algorithms are designed to explore or search through a graph, they have distinct characteristics that make them more suitable for different types of problems. In this comprehensive guide, we’ll dive deep into when to use BFS versus DFS in graph problems, providing you with the knowledge to make informed decisions in your coding journey.
Understanding BFS and DFS
Before we delve into the specifics of when to use each algorithm, let’s briefly review what BFS and DFS are and how they work.
Breadth-First Search (BFS)
BFS is an algorithm that explores a graph level by level. It starts at a chosen node (often called the root) and explores all the neighboring nodes at the present depth before moving on to the nodes at the next depth level. This process continues until all nodes have been visited.
Key characteristics of BFS:
- Uses a queue data structure
- Explores nodes in order of their distance from the starting node
- Guarantees the shortest path in unweighted graphs
Depth-First Search (DFS)
DFS, on the other hand, explores as far as possible along each branch before backtracking. It starts at a chosen node and explores as far as possible along each branch before backtracking.
Key characteristics of DFS:
- Uses a stack data structure (or recursion)
- Explores nodes by going as deep as possible before backtracking
- Memory-efficient for certain types of graphs
When to Use BFS
BFS is particularly useful in several scenarios. Let’s explore some common situations where BFS is the preferred choice:
1. Finding the Shortest Path in Unweighted Graphs
One of the most common applications of BFS is finding the shortest path between two nodes in an unweighted graph. Since BFS explores nodes level by level, it guarantees that the first time a node is discovered during the traversal, it is reached by the shortest path from the source.
Example problem: Finding the shortest route between two stations in a metro network where all connections have equal length.
def bfs_shortest_path(graph, start, goal):
queue = [(start, [start])]
visited = set()
while queue:
(vertex, path) = queue.pop(0)
if vertex not in visited:
if vertex == goal:
return path
visited.add(vertex)
for neighbor in graph[vertex]:
if neighbor not in visited:
queue.append((neighbor, path + [neighbor]))
return None
# Example usage
graph = {
'A': ['B', 'C'],
'B': ['A', 'D', 'E'],
'C': ['A', 'F'],
'D': ['B'],
'E': ['B', 'F'],
'F': ['C', 'E']
}
print(bfs_shortest_path(graph, 'A', 'F')) # Output: ['A', 'C', 'F']
2. Level-Order Traversal of a Tree
BFS is naturally suited for level-order traversal of a tree, where you need to process all nodes at a given depth before moving to the next level. This is particularly useful in scenarios like:
- Printing a binary tree level by level
- Finding the minimum depth of a binary tree
- Serializing and deserializing a binary tree
from collections import deque
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def levelOrder(root):
if not root:
return []
result = []
queue = deque([root])
while queue:
level_size = len(queue)
current_level = []
for _ in range(level_size):
node = queue.popleft()
current_level.append(node.val)
if node.left:
queue.append(node.left)
if node.right:
queue.append(node.right)
result.append(current_level)
return result
# Example usage
root = TreeNode(3)
root.left = TreeNode(9)
root.right = TreeNode(20)
root.right.left = TreeNode(15)
root.right.right = TreeNode(7)
print(levelOrder(root)) # Output: [[3], [9, 20], [15, 7]]
3. Finding All Nodes at a Given Distance
BFS is efficient when you need to find all nodes at a certain distance from a given node. This is because BFS explores the graph in layers, making it easy to keep track of the distance from the starting node.
Example problem: In a social network, find all friends of friends (nodes at distance 2) for a given user.
from collections import deque
def friends_of_friends(graph, start):
queue = deque([(start, 0)])
visited = set([start])
friends_of_friends = set()
while queue:
person, distance = queue.popleft()
if distance == 2:
friends_of_friends.add(person)
elif distance < 2:
for friend in graph[person]:
if friend not in visited:
visited.add(friend)
queue.append((friend, distance + 1))
return friends_of_friends
# Example usage
social_network = {
'Alice': ['Bob', 'Charlie'],
'Bob': ['Alice', 'David'],
'Charlie': ['Alice', 'Eve'],
'David': ['Bob', 'Eve'],
'Eve': ['Charlie', 'David']
}
print(friends_of_friends(social_network, 'Alice')) # Output: {'David', 'Eve'}
4. Testing Graph Bipartiteness
BFS can be used to determine if a graph is bipartite (i.e., its vertices can be divided into two disjoint sets such that every edge connects a vertex in one set to a vertex in the other set). This is because BFS naturally divides the graph into levels, which can be used to assign colors to the nodes.
from collections import deque
def is_bipartite(graph):
color = {}
def bfs(start):
queue = deque([start])
color[start] = 0
while queue:
node = queue.popleft()
for neighbor in graph[node]:
if neighbor not in color:
color[neighbor] = 1 - color[node]
queue.append(neighbor)
elif color[neighbor] == color[node]:
return False
return True
for node in graph:
if node not in color:
if not bfs(node):
return False
return True
# Example usage
graph1 = {
0: [1, 3],
1: [0, 2],
2: [1, 3],
3: [0, 2]
}
print(is_bipartite(graph1)) # Output: True
graph2 = {
0: [1, 2],
1: [0, 2],
2: [0, 1]
}
print(is_bipartite(graph2)) # Output: False
When to Use DFS
DFS has its own set of strengths that make it the preferred choice in certain scenarios. Let’s explore when DFS shines:
1. Detecting Cycles in a Graph
DFS is particularly useful for detecting cycles in a graph. As it explores paths to their full depth, it can easily keep track of nodes in the current path and detect if a back edge exists (an edge that points to an ancestor in the DFS tree).
def has_cycle(graph):
visited = set()
rec_stack = set()
def dfs(node):
visited.add(node)
rec_stack.add(node)
for neighbor in graph[node]:
if neighbor not in visited:
if dfs(neighbor):
return True
elif neighbor in rec_stack:
return True
rec_stack.remove(node)
return False
for node in graph:
if node not in visited:
if dfs(node):
return True
return False
# Example usage
graph_with_cycle = {
0: [1, 2],
1: [2],
2: [0, 3],
3: [3]
}
print(has_cycle(graph_with_cycle)) # Output: True
graph_without_cycle = {
0: [1, 2],
1: [2],
2: [3],
3: []
}
print(has_cycle(graph_without_cycle)) # Output: False
2. Topological Sorting
DFS is commonly used for topological sorting of a directed acyclic graph (DAG). This is useful in scenarios where you need to order tasks with dependencies, such as in build systems or course scheduling.
from collections import defaultdict
def topological_sort(graph):
visited = set()
stack = []
def dfs(node):
visited.add(node)
for neighbor in graph[node]:
if neighbor not in visited:
dfs(neighbor)
stack.append(node)
for node in graph:
if node not in visited:
dfs(node)
return stack[::-1]
# Example usage
graph = {
'A': ['C'],
'B': ['C', 'D'],
'C': ['E'],
'D': ['F'],
'E': ['H', 'F'],
'F': ['G'],
'G': [],
'H': []
}
print(topological_sort(graph)) # Output: ['B', 'A', 'C', 'E', 'D', 'F', 'H', 'G']
3. Solving Mazes or Puzzles
DFS is often the algorithm of choice when solving mazes or similar puzzles. Its nature of exploring one path fully before backtracking makes it well-suited for finding a solution in these scenarios.
def solve_maze(maze, start, end):
def dfs(x, y, path):
if (x, y) == end:
return path
for dx, dy in [(0, 1), (1, 0), (0, -1), (-1, 0)]: # right, down, left, up
next_x, next_y = x + dx, y + dy
if 0 <= next_x < len(maze) and 0 <= next_y < len(maze[0]) and maze[next_x][next_y] == 0:
maze[next_x][next_y] = 2 # mark as visited
result = dfs(next_x, next_y, path + [(next_x, next_y)])
if result:
return result
maze[next_x][next_y] = 0 # backtrack
return None
maze[start[0]][start[1]] = 2 # mark start as visited
return dfs(start[0], start[1], [start])
# Example usage
maze = [
[0, 0, 0, 0, 0],
[1, 1, 0, 1, 0],
[0, 0, 0, 0, 0],
[0, 1, 1, 1, 0],
[0, 0, 0, 1, 0]
]
start = (0, 0)
end = (4, 4)
solution = solve_maze(maze, start, end)
print(solution) # Output: [(0, 0), (0, 1), (0, 2), (0, 3), (0, 4), (1, 4), (2, 4), (3, 4), (4, 4)]
4. Finding Strongly Connected Components
DFS is used in algorithms like Kosaraju’s algorithm to find strongly connected components in a directed graph. This is useful in various applications, such as analyzing the structure of websites or social networks.
from collections import defaultdict
def kosaraju(graph):
def dfs_first_pass(node):
visited.add(node)
for neighbor in graph[node]:
if neighbor not in visited:
dfs_first_pass(neighbor)
stack.append(node)
def dfs_second_pass(node):
visited.add(node)
component.append(node)
for neighbor in reversed_graph[node]:
if neighbor not in visited:
dfs_second_pass(neighbor)
stack = []
visited = set()
for node in graph:
if node not in visited:
dfs_first_pass(node)
reversed_graph = defaultdict(list)
for node in graph:
for neighbor in graph[node]:
reversed_graph[neighbor].append(node)
visited = set()
strongly_connected_components = []
while stack:
node = stack.pop()
if node not in visited:
component = []
dfs_second_pass(node)
strongly_connected_components.append(component)
return strongly_connected_components
# Example usage
graph = {
0: [1, 3],
1: [2],
2: [0],
3: [4],
4: [5],
5: [3]
}
print(kosaraju(graph)) # Output: [[0, 2, 1], [3, 5, 4]]
Comparing BFS and DFS
Now that we’ve explored when to use BFS and DFS, let’s compare them side by side to highlight their differences:
Aspect | BFS | DFS |
---|---|---|
Traversal Order | Level by level | Path by path |
Data Structure | Queue | Stack (or recursion) |
Space Complexity | O(bd) where b is branching factor and d is depth | O(h) where h is the height of the tree |
Completeness | Complete (finds all solutions) | Not complete (may not find all solutions in infinite graphs) |
Optimal for Unweighted Graphs | Yes | No |
Memory Usage | Higher (keeps all nodes at a level in memory) | Lower (keeps only nodes on a single path in memory) |
Conclusion
Choosing between BFS and DFS depends on the specific problem you’re trying to solve and the characteristics of your graph. Here’s a quick summary to help you decide:
- Use BFS when:
- Finding the shortest path in an unweighted graph
- Exploring nodes level by level is important
- The solution is not far from the root
- You need to find all nodes at a certain distance
- Use DFS when:
- Exploring paths to their full extent is important
- Memory is a constraint
- The solution is likely to be far from the root
- You’re dealing with problems like cycle detection, topological sorting, or maze solving
Remember, the choice between BFS and DFS isn’t always clear-cut. Sometimes, a problem can be solved efficiently with either approach, and the decision may come down to personal preference or specific implementation details. As you gain more experience with graph problems, you’ll develop an intuition for which algorithm to use in different scenarios.
Practice implementing both BFS and DFS for various graph problems to deepen your understanding and sharpen your problem-solving skills. This knowledge will be invaluable as you prepare for technical interviews and tackle real-world coding challenges.