When to Use a Visited Set in Graph Problems: A Comprehensive Guide
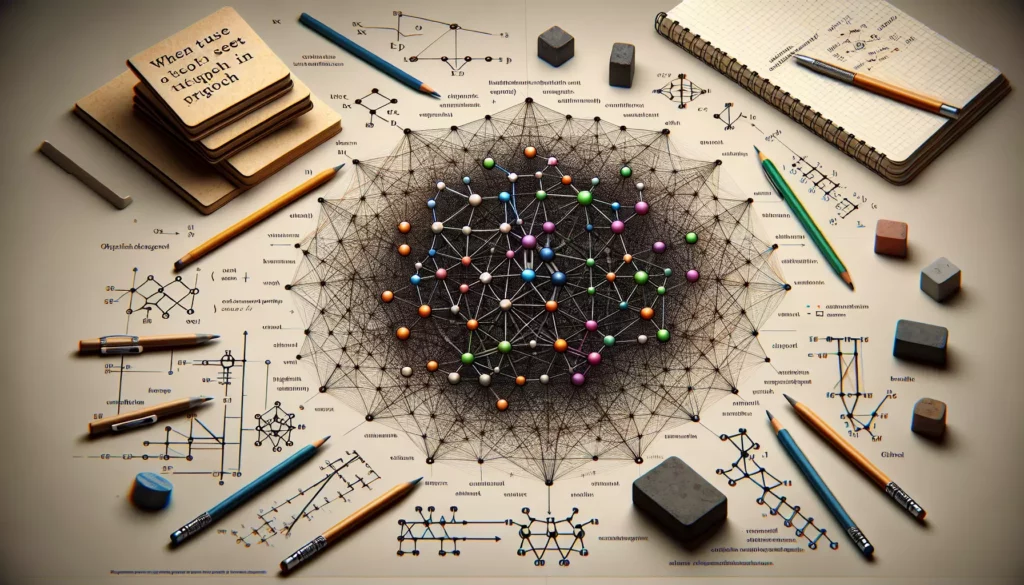
Graph problems are a fundamental part of computer science and a common feature in coding interviews, especially for major tech companies. One crucial concept that often comes up when dealing with graph traversals is the use of a “visited” set. In this comprehensive guide, we’ll explore when and why you should use a visited set in graph problems, providing you with the knowledge to tackle these challenges confidently.
Understanding Graph Traversals
Before we dive into the specifics of using a visited set, let’s briefly review what graph traversals are and why they’re important.
Graph traversals are algorithms used to visit all the vertices in a graph. The two primary types of graph traversals are:
- Depth-First Search (DFS)
- Breadth-First Search (BFS)
These traversal methods are crucial for solving various graph problems, such as finding the shortest path, detecting cycles, or identifying connected components.
What is a Visited Set?
A visited set is a data structure used to keep track of the vertices that have already been explored during a graph traversal. It’s typically implemented as a set or a boolean array, where each element corresponds to a vertex in the graph.
Here’s a simple example of how a visited set might be initialized in Python:
def initialize_visited(num_vertices):
return set() # For a set implementation
# OR
# return [False] * num_vertices # For a boolean array implementation
When to Use a Visited Set
Now, let’s explore the scenarios where using a visited set is crucial:
1. Preventing Infinite Loops in Cyclic Graphs
One of the primary reasons to use a visited set is to prevent infinite loops when traversing cyclic graphs. Without a visited set, your algorithm might keep revisiting the same vertices indefinitely.
Consider this simple undirected graph with a cycle:
A --- B
| |
C --- D
Without a visited set, a DFS starting from A might follow the path A -> B -> D -> C -> A -> B -> D -> C -> A, and so on, never terminating.
Here’s how you might implement a DFS with a visited set to avoid this issue:
def dfs(graph, start, visited=None):
if visited is None:
visited = set()
visited.add(start)
print(start) # Process the current vertex
for neighbor in graph[start]:
if neighbor not in visited:
dfs(graph, neighbor, visited)
# Example usage
graph = {
'A': ['B', 'C'],
'B': ['A', 'D'],
'C': ['A', 'D'],
'D': ['B', 'C']
}
dfs(graph, 'A')
2. Ensuring Efficiency in Large Graphs
For large graphs, using a visited set can significantly improve the efficiency of your traversal. Without it, you might end up processing the same vertices multiple times, leading to unnecessary computations.
This is particularly important in problems where you need to find the shortest path or perform any kind of optimization. By marking vertices as visited, you ensure that each vertex is processed only once, reducing the time complexity of your algorithm.
3. Detecting Cycles
A visited set is crucial when you need to detect cycles in a graph. By keeping track of visited vertices, you can identify when you’ve returned to a vertex that’s already been explored in the current traversal path.
Here’s a simple example of cycle detection using DFS and a visited set:
def has_cycle(graph):
visited = set()
rec_stack = set()
def dfs(vertex):
visited.add(vertex)
rec_stack.add(vertex)
for neighbor in graph[vertex]:
if neighbor not in visited:
if dfs(neighbor):
return True
elif neighbor in rec_stack:
return True
rec_stack.remove(vertex)
return False
for vertex in graph:
if vertex not in visited:
if dfs(vertex):
return True
return False
# Example usage
graph = {
'A': ['B', 'C'],
'B': ['D'],
'C': ['D'],
'D': ['A']
}
print(has_cycle(graph)) # Output: True
4. Finding Connected Components
When you need to identify connected components in an undirected graph, a visited set is essential. It helps you keep track of which vertices have been explored and which belong to the current component.
Here’s an example of how to find connected components using a visited set:
def find_connected_components(graph):
visited = set()
components = []
def dfs(vertex, component):
visited.add(vertex)
component.append(vertex)
for neighbor in graph[vertex]:
if neighbor not in visited:
dfs(neighbor, component)
for vertex in graph:
if vertex not in visited:
component = []
dfs(vertex, component)
components.append(component)
return components
# Example usage
graph = {
'A': ['B'],
'B': ['A', 'C'],
'C': ['B'],
'D': ['E'],
'E': ['D'],
'F': []
}
print(find_connected_components(graph))
# Output: [['A', 'B', 'C'], ['D', 'E'], ['F']]
5. Topological Sorting
In directed acyclic graphs (DAGs), topological sorting is a common operation. A visited set is crucial for implementing this algorithm efficiently. It helps in keeping track of vertices that have already been processed and ensures that each vertex is visited only once.
Here’s an example of topological sorting using DFS and a visited set:
from collections import defaultdict
def topological_sort(graph):
visited = set()
stack = []
def dfs(vertex):
visited.add(vertex)
for neighbor in graph[vertex]:
if neighbor not in visited:
dfs(neighbor)
stack.append(vertex)
for vertex in graph:
if vertex not in visited:
dfs(vertex)
return stack[::-1]
# Example usage
graph = defaultdict(list)
graph[5].append(2)
graph[5].append(0)
graph[4].append(0)
graph[4].append(1)
graph[2].append(3)
graph[3].append(1)
print(topological_sort(graph))
# Output: [5, 4, 2, 3, 1, 0]
When Not to Use a Visited Set
While a visited set is crucial in many graph problems, there are situations where it might not be necessary or even desirable:
1. Tree Traversals
In a tree (which is a special type of graph), you don’t need a visited set for basic traversals. This is because trees don’t have cycles, so you won’t risk infinite loops. However, if you’re treating the tree as a general graph and don’t know it’s a tree beforehand, using a visited set is still a safe approach.
2. Problems Requiring Multiple Visits
Some graph problems might require you to visit vertices multiple times. For example, if you’re trying to find all possible paths between two vertices, you wouldn’t want to mark vertices as visited and prevent revisiting them.
3. Memory Constraints
In some cases, especially with extremely large graphs, the memory overhead of maintaining a visited set might be prohibitive. In such situations, you might need to explore alternative approaches or use more memory-efficient data structures.
Best Practices for Using Visited Sets
To make the most of visited sets in your graph algorithms, consider these best practices:
1. Choose the Right Data Structure
Depending on your graph representation and the problem at hand, you might choose different data structures for your visited set:
- Set: Offers O(1) average time complexity for insertions and lookups. Good for graphs with string or object vertex identifiers.
- Boolean Array: If your vertices are numbered from 0 to n-1, a boolean array can be more memory-efficient and offer faster access times.
- Bitset: For even more memory efficiency, especially with large graphs, consider using a bitset.
2. Initialize Properly
Make sure to initialize your visited set or array correctly at the start of your algorithm. For sets, this means creating an empty set. For arrays, initialize all elements to False or 0.
3. Clear Between Runs
If you’re running multiple traversals on the same graph, remember to clear or reinitialize your visited set between runs.
4. Consider Problem-Specific Variations
Sometimes, you might need more than just a binary visited/not visited state. For example, in detecting cycles in directed graphs, you might need to distinguish between vertices in the current recursion stack and those that have been completely processed.
Common Pitfalls and How to Avoid Them
When working with visited sets in graph problems, be aware of these common pitfalls:
1. Forgetting to Mark as Visited
One of the most common mistakes is forgetting to mark a vertex as visited before exploring its neighbors. This can lead to unnecessary repeated explorations and potential infinite loops in cyclic graphs.
To avoid this, always mark a vertex as visited as soon as you start processing it:
def dfs(graph, vertex, visited):
visited.add(vertex) # Mark as visited immediately
# Process vertex
for neighbor in graph[vertex]:
if neighbor not in visited:
dfs(graph, neighbor, visited)
2. Incorrect Initialization
Another common mistake is incorrectly initializing the visited set or array. For example, if you’re using a boolean array, make sure it’s the correct size and all elements are initially set to False.
def initialize_visited(num_vertices):
return [False] * num_vertices # Correct initialization for boolean array
# NOT: return [False] # This would be incorrect!
3. Not Clearing Between Multiple Runs
If you’re running multiple traversals on the same graph (e.g., finding paths from different start points), remember to clear or reinitialize your visited set between runs. Otherwise, you might incorrectly think a vertex has been visited in the current traversal when it was actually visited in a previous one.
def multiple_traversals(graph, start_points):
for start in start_points:
visited = set() # Reinitialize for each traversal
dfs(graph, start, visited)
4. Misusing in Bidirectional Search
In bidirectional search algorithms, you typically need two separate visited sets – one for the forward search and one for the backward search. Mixing these up can lead to incorrect results.
def bidirectional_search(graph, start, end):
forward_visited = set([start])
backward_visited = set([end])
# ... rest of the algorithm
Advanced Topics: Variations on the Visited Set
As you become more proficient with graph algorithms, you’ll encounter situations where a simple binary visited/not visited state isn’t sufficient. Here are some advanced variations on the visited set concept:
1. Multi-State Visited Sets
In some problems, you might need to track multiple states for each vertex. For example, in cycle detection for directed graphs, you often need to distinguish between vertices that are currently in the recursion stack and those that have been fully processed.
def has_cycle(graph):
WHITE, GRAY, BLACK = 0, 1, 2
colors = {v: WHITE for v in graph}
def dfs(vertex):
colors[vertex] = GRAY
for neighbor in graph[vertex]:
if colors[neighbor] == WHITE:
if dfs(neighbor):
return True
elif colors[neighbor] == GRAY:
return True
colors[vertex] = BLACK
return False
for vertex in graph:
if colors[vertex] == WHITE:
if dfs(vertex):
return True
return False
2. Distance-Aware Visited Sets
In problems where you need to keep track of the distance from a starting point, you can use a dictionary instead of a set, where the keys are vertices and the values are distances.
from collections import deque
def bfs_with_distance(graph, start):
visited = {start: 0}
queue = deque([(start, 0)])
while queue:
vertex, distance = queue.popleft()
for neighbor in graph[vertex]:
if neighbor not in visited:
visited[neighbor] = distance + 1
queue.append((neighbor, distance + 1))
return visited
3. Path-Aware Visited Sets
When you need to reconstruct the path taken during a traversal, you can modify your visited structure to keep track of the parent of each visited vertex.
def bfs_with_path(graph, start, end):
visited = {start: None}
queue = deque([start])
while queue:
vertex = queue.popleft()
if vertex == end:
path = []
while vertex is not None:
path.append(vertex)
vertex = visited[vertex]
return path[::-1]
for neighbor in graph[vertex]:
if neighbor not in visited:
visited[neighbor] = vertex
queue.append(neighbor)
return None # Path not found
Conclusion
Understanding when and how to use a visited set is crucial for solving graph problems efficiently. Whether you’re preparing for coding interviews at major tech companies or working on real-world graph algorithms, mastering this concept will significantly improve your problem-solving skills.
Remember, the key points are:
- Use visited sets to prevent infinite loops in cyclic graphs
- Improve efficiency by ensuring each vertex is processed only once
- Utilize visited sets for cycle detection, finding connected components, and topological sorting
- Be aware of situations where a visited set might not be necessary
- Choose the appropriate data structure for your visited set based on your specific problem
- Be mindful of common pitfalls like forgetting to mark vertices as visited or incorrect initialization
As you practice more graph problems, you’ll develop an intuition for when and how to use visited sets effectively. Keep exploring, and don’t hesitate to experiment with different approaches to deepen your understanding of this fundamental concept in graph algorithms.