When to Use a Two-Heap Approach: Mastering Advanced Data Structures
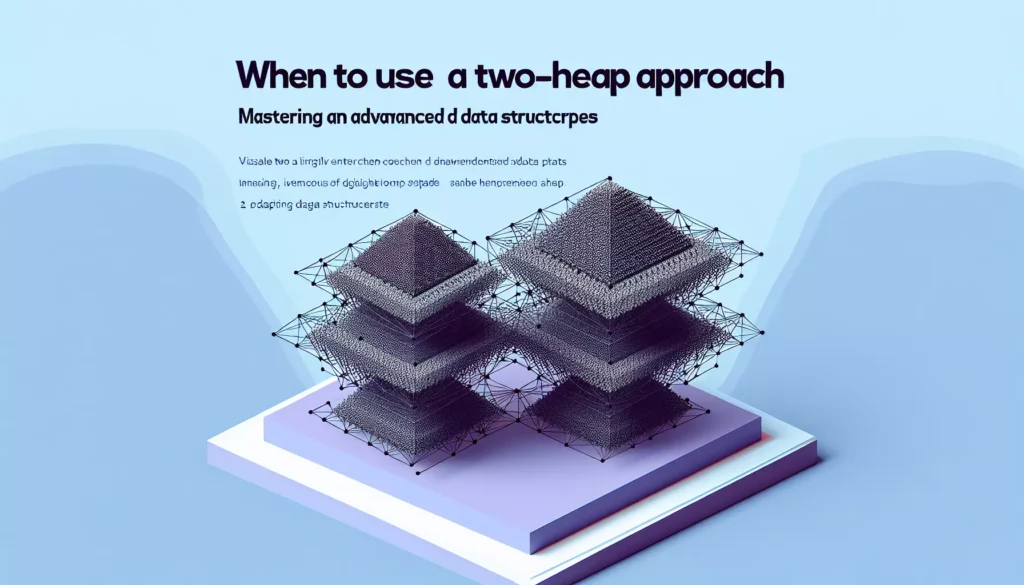
In the world of algorithmic problem-solving and data structure optimization, the two-heap approach stands out as a powerful technique that can significantly enhance the efficiency of certain operations. As aspiring software engineers and coding enthusiasts progress in their journey, understanding when and how to implement this strategy becomes crucial. In this comprehensive guide, we’ll explore the intricacies of the two-heap approach, its applications, and the scenarios where it shines brightest.
Understanding the Two-Heap Approach
Before diving into the specifics of when to use a two-heap approach, let’s establish a solid foundation by understanding what it entails.
What is a Heap?
A heap is a specialized tree-based data structure that satisfies the heap property. In a max heap, for any given node I, the value of I is greater than or equal to the values of its children. Conversely, in a min heap, the value of I is less than or equal to the values of its children.
The Two-Heap Concept
The two-heap approach involves using two heaps simultaneously to solve a problem efficiently. Typically, one heap is a max heap, and the other is a min heap. This setup allows for constant-time access to both the maximum and minimum elements of a dataset, as well as efficient insertion and deletion operations.
When to Consider Using a Two-Heap Approach
Now that we have a basic understanding of the two-heap concept, let’s explore the scenarios where this approach proves most beneficial.
1. Median Maintenance
One of the most common applications of the two-heap approach is in maintaining the median of a stream of numbers. This problem arises in various real-world scenarios, such as:
- Real-time analytics of financial data
- Processing sensor readings in IoT devices
- Monitoring network traffic patterns
In these cases, you need to efficiently keep track of the median as new numbers are added to the dataset. The two-heap approach allows for O(log n) insertion and O(1) median retrieval, making it an ideal solution.
Implementation for Median Maintenance
Here’s a basic implementation of the median maintenance problem using two heaps in Python:
import heapq
class MedianFinder:
def __init__(self):
self.small = [] # max heap
self.large = [] # min heap
def addNum(self, num: int) -> None:
if len(self.small) == len(self.large):
heapq.heappush(self.large, -heapq.heappushpop(self.small, -num))
else:
heapq.heappush(self.small, -heapq.heappushpop(self.large, num))
def findMedian(self) -> float:
if len(self.small) == len(self.large):
return (-self.small[0] + self.large[0]) / 2.0
else:
return float(self.large[0])
In this implementation, we maintain two heaps: small
(a max heap) and large
(a min heap). The small
heap contains the smaller half of the numbers, while the large
heap contains the larger half. By balancing these heaps, we can efficiently retrieve the median.
2. Sliding Window Problems
Another scenario where the two-heap approach proves valuable is in solving sliding window problems, particularly when you need to maintain the maximum or minimum element within a dynamic range. Some examples include:
- Finding the maximum/minimum element in a sliding window of fixed size
- Maintaining a running median in a sliding window
- Implementing priority queues with expiration
The two-heap approach allows for efficient updates as elements enter and leave the window, making it an excellent choice for these types of problems.
Example: Sliding Window Median
Let’s look at an example of how to use the two-heap approach to solve the sliding window median problem:
from heapq import *
class Solution:
def medianSlidingWindow(self, nums: List[int], k: int) -> List[float]:
def move(h1, h2):
x, i = heappop(h1)
heappush(h2, (-x, i))
def get_med(h1, h2, k):
return h2[0][0] * 1. if k & 1 else (h2[0][0] - h1[0][0]) / 2.
small, large = [], []
for i, x in enumerate(nums[:k]):
heappush(small, (-x, i))
for _ in range(k - (k >> 1)):
move(small, large)
ans = [get_med(small, large, k)]
for i, x in enumerate(nums[k:]):
if x >= large[0][0]:
heappush(large, (x, i + k))
if nums[i] <= large[0][0]:
move(large, small)
else:
heappush(small, (-x, i + k))
if nums[i] >= large[0][0]:
move(small, large)
while small and small[0][1] <= i: heappop(small)
while large and large[0][1] <= i: heappop(large)
ans.append(get_med(small, large, k))
return ans
This solution efficiently maintains the median within a sliding window using two heaps, demonstrating the power of the two-heap approach in handling dynamic range queries.
3. K-th Largest/Smallest Element
While a single heap can be used to find the k-th largest or smallest element, the two-heap approach can be beneficial when you need to maintain both the k-th largest and k-th smallest elements simultaneously. This scenario might arise in:
- Ranking systems that track both top and bottom performers
- Data streaming applications that need to monitor extremes
- Anomaly detection systems that identify outliers in both directions
By using a max heap for the smaller elements and a min heap for the larger elements, you can efficiently maintain and update these k-th elements as new data arrives.
Implementation for K-th Largest/Smallest
Here’s a basic implementation that maintains both the k-th largest and k-th smallest elements:
import heapq
class KthLargestSmallest:
def __init__(self, k: int):
self.k = k
self.small = [] # max heap for smallest elements
self.large = [] # min heap for largest elements
def add(self, num: int) -> Tuple[int, int]:
if len(self.small) < self.k:
heapq.heappush(self.small, -num)
elif num > -self.small[0]:
heapq.heappush(self.large, num)
if len(self.large) > self.k:
heapq.heappush(self.small, -heapq.heappop(self.large))
else:
heapq.heappush(self.small, -num)
heapq.heappush(self.large, -heapq.heappop(self.small))
while len(self.small) > self.k:
heapq.heappush(self.large, -heapq.heappop(self.small))
kth_smallest = -self.small[0] if len(self.small) == self.k else float('inf')
kth_largest = self.large[0] if len(self.large) >= self.k else float('-inf')
return kth_smallest, kth_largest
This implementation maintains two heaps: small
for the k smallest elements and large
for the rest. It efficiently updates both heaps as new elements are added, allowing for quick retrieval of both the k-th largest and k-th smallest elements.
Advantages of the Two-Heap Approach
Now that we’ve explored some scenarios where the two-heap approach is applicable, let’s discuss its advantages:
1. Efficient Operations
The two-heap approach offers O(log n) time complexity for insertions and deletions, and O(1) for accessing the maximum or minimum elements. This efficiency is crucial when dealing with large datasets or real-time data streams.
2. Balanced Data Distribution
By maintaining two heaps, you can effectively balance the distribution of data, which is particularly useful in problems like median finding or maintaining a specific range of elements.
3. Flexibility
The two-heap approach can be adapted to various problems beyond just finding medians or extremes. It’s a versatile technique that can be applied creatively to solve complex data management issues.
4. Space Efficiency
Compared to other data structures that might require storing all elements in sorted order, the two-heap approach often requires less memory, as it only needs to maintain a portion of the data in each heap.
Considerations and Limitations
While the two-heap approach is powerful, it’s important to be aware of its limitations and considerations:
1. Implementation Complexity
Implementing and maintaining two heaps can be more complex than using a single data structure, especially when ensuring proper balance between the heaps.
2. Not Always the Best Solution
For some problems, other data structures like balanced binary search trees or segment trees might be more appropriate, especially if you need more complex range queries.
3. Memory Usage
While generally space-efficient, the two-heap approach still requires storing elements in memory. For extremely large datasets, you might need to consider external memory algorithms.
4. Handling Duplicates
When dealing with duplicate elements, special care must be taken to ensure the heaps remain balanced and that the logic for moving elements between heaps remains correct.
Real-World Applications
The two-heap approach finds applications in various real-world scenarios:
1. Stock Market Analysis
In financial markets, maintaining a running median of stock prices can help identify trends and anomalies. The two-heap approach allows for efficient updates as new price data streams in.
2. Load Balancing in Distributed Systems
Two heaps can be used to efficiently distribute tasks or data across servers, ensuring a balanced workload by always having access to both the most and least loaded servers.
3. Anomaly Detection in IoT Devices
For IoT systems collecting sensor data, the two-heap approach can help in real-time identification of outliers by maintaining both the highest and lowest ranges of normal readings.
4. Social Media Trending Topics
Platforms can use two heaps to efficiently track both the most and least popular topics, allowing for quick updates as post engagements change over time.
Implementing the Two-Heap Approach: Best Practices
When implementing the two-heap approach, consider the following best practices:
1. Choose the Right Heap Implementation
Most programming languages offer heap implementations. In Python, you can use the heapq
module. In Java, consider PriorityQueue
. Choose the implementation that best fits your language and performance requirements.
2. Balance the Heaps
Ensure that the two heaps remain balanced or nearly balanced. The size difference between the heaps should typically not exceed 1.
3. Handle Edge Cases
Be prepared to handle edge cases, such as when the dataset is empty or when there are duplicate elements.
4. Optimize for Your Specific Use Case
Depending on your problem, you might need to customize the heap comparison function or add additional metadata to the heap elements.
5. Consider Lazy Deletion
In scenarios where elements might become invalid (like in sliding window problems), consider implementing lazy deletion to avoid frequent rebalancing of the heaps.
Conclusion
The two-heap approach is a powerful technique in the toolkit of any proficient programmer or software engineer. Its ability to efficiently manage datasets while providing quick access to extremes makes it invaluable in scenarios ranging from median maintenance to complex data streaming problems.
As you progress in your coding journey, mastering the two-heap approach will not only enhance your problem-solving skills but also prepare you for tackling advanced algorithmic challenges often encountered in technical interviews at top tech companies.
Remember, the key to mastering this technique lies in practice and application. Try implementing the two-heap approach in various scenarios, experiment with different problem types, and always be on the lookout for situations where this elegant solution can be applied.
By understanding when and how to use the two-heap approach, you’re taking a significant step towards becoming a more versatile and efficient programmer, ready to tackle complex data management challenges in the ever-evolving world of software development.