When to Use a Prefix Sum Array: Recognition Patterns
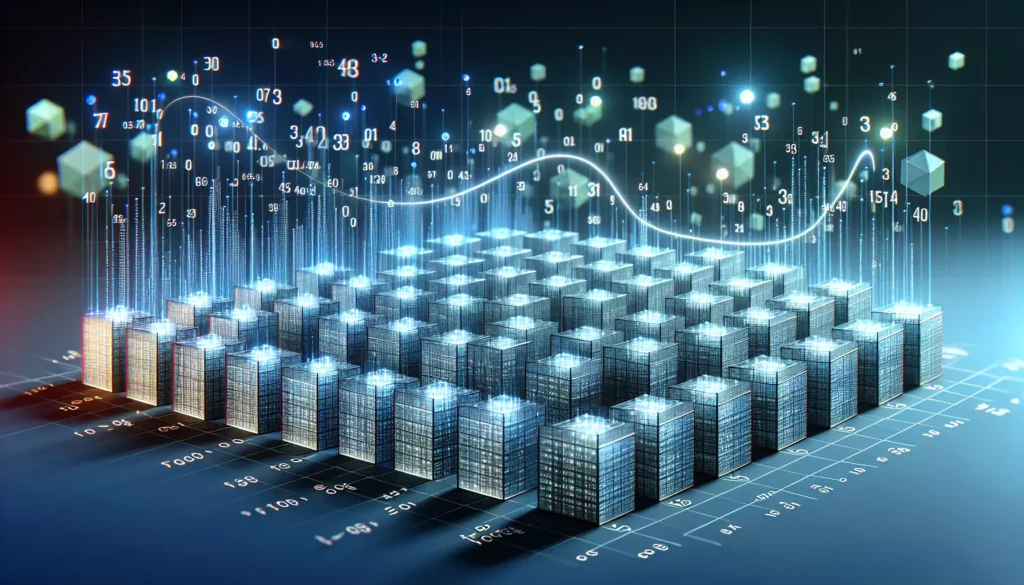
In the world of algorithmic problem-solving, efficiency is key. One powerful technique that often comes to the rescue is the prefix sum array. This data structure can significantly optimize certain types of queries and calculations, especially when dealing with cumulative sums over ranges in an array. But how do you know when to reach for this tool in your algorithmic toolbox? In this comprehensive guide, we’ll explore the recognition patterns that signal when a prefix sum array might be the perfect solution to your problem.
What is a Prefix Sum Array?
Before we dive into the recognition patterns, let’s quickly refresh our understanding of what a prefix sum array is. A prefix sum array, also known as a cumulative sum array, is a data structure where each element is the sum of all previous elements in the original array, including itself.
For example, given an array [3, 1, 4, 1, 5, 9]
, its prefix sum array would be [3, 4, 8, 9, 14, 23]
.
The power of a prefix sum array lies in its ability to calculate the sum of any contiguous subarray in constant time, once the prefix sum array is constructed.
Recognition Pattern 1: Range Sum Queries
The most common and straightforward pattern that calls for a prefix sum array is when you need to perform multiple range sum queries on a static array.
Problem Characteristics:
- You have a fixed array of numbers.
- You need to calculate the sum of elements between two indices multiple times.
- The array doesn’t change between queries.
Example Problem:
Given an array of integers, answer multiple queries asking for the sum of elements between index i and j (inclusive).
Solution Approach:
Construct a prefix sum array once, then use it to answer each query in O(1) time by subtracting the prefix sum at (i-1) from the prefix sum at j.
Code Example:
class PrefixSum {
private int[] prefixSum;
public PrefixSum(int[] nums) {
prefixSum = new int[nums.length + 1];
for (int i = 0; i < nums.length; i++) {
prefixSum[i + 1] = prefixSum[i] + nums[i];
}
}
public int rangeSum(int left, int right) {
return prefixSum[right + 1] - prefixSum[left];
}
}
Recognition Pattern 2: Cumulative Property Calculations
Another pattern where prefix sum arrays shine is when you need to calculate cumulative properties of an array, especially when these properties have an additive nature.
Problem Characteristics:
- You need to track a cumulative property across an array.
- The property is additive (can be summed).
- You may need to query this cumulative property for different ranges.
Example Problem:
Given an array representing daily temperatures, find the number of days in any given range where the temperature was above average.
Solution Approach:
Create a binary array where 1 represents above average and 0 represents below or at average. Then create a prefix sum of this binary array. The difference between two prefix sums will give the count of above-average days in that range.
Code Example:
class TemperatureAnalyzer {
private int[] prefixSum;
public TemperatureAnalyzer(int[] temperatures) {
int avg = calculateAverage(temperatures);
int[] aboveAverage = new int[temperatures.length];
for (int i = 0; i < temperatures.length; i++) {
aboveAverage[i] = temperatures[i] > avg ? 1 : 0;
}
prefixSum = new int[aboveAverage.length + 1];
for (int i = 0; i < aboveAverage.length; i++) {
prefixSum[i + 1] = prefixSum[i] + aboveAverage[i];
}
}
public int countAboveAverageDays(int start, int end) {
return prefixSum[end + 1] - prefixSum[start];
}
private int calculateAverage(int[] arr) {
int sum = 0;
for (int num : arr) sum += num;
return sum / arr.length;
}
}
Recognition Pattern 3: Equilibrium Index Problems
Prefix sum arrays are particularly useful in problems involving finding an equilibrium index or a pivot point in an array where certain conditions are met on both sides.
Problem Characteristics:
- You need to find a point in the array where the sum of elements on one side equals the sum on the other side.
- You may need to find multiple such points or the count of such points.
Example Problem:
Find the index in an array where the sum of all elements to the left is equal to the sum of all elements to the right.
Solution Approach:
Construct a prefix sum array. Then, for each index, compare the sum to its left (which is the prefix sum at that index) with the sum to its right (which is the total sum minus the prefix sum at that index).
Code Example:
class EquilibriumFinder {
public int findEquilibriumIndex(int[] nums) {
int[] prefixSum = new int[nums.length + 1];
for (int i = 0; i < nums.length; i++) {
prefixSum[i + 1] = prefixSum[i] + nums[i];
}
int totalSum = prefixSum[nums.length];
for (int i = 0; i < nums.length; i++) {
int leftSum = prefixSum[i];
int rightSum = totalSum - prefixSum[i + 1];
if (leftSum == rightSum) {
return i;
}
}
return -1; // No equilibrium index found
}
}
Recognition Pattern 4: Subarray Sum Problems
Prefix sum arrays are invaluable when dealing with problems that involve finding subarrays with a particular sum or counting such subarrays.
Problem Characteristics:
- You need to find or count subarrays with a specific sum.
- You’re dealing with cumulative effects of elements in subarrays.
Example Problem:
Count the number of subarrays in an array that sum to a given target value.
Solution Approach:
Construct a prefix sum array and use a hash map to keep track of the frequency of each prefix sum. As you iterate through the prefix sums, check if (current prefix sum – target) exists in the map, which would indicate a subarray with the target sum.
Code Example:
import java.util.HashMap;
class SubarraySumCounter {
public int countSubarrays(int[] nums, int k) {
HashMap<Integer, Integer> sumFrequency = new HashMap<>();
sumFrequency.put(0, 1);
int count = 0;
int currentSum = 0;
for (int num : nums) {
currentSum += num;
count += sumFrequency.getOrDefault(currentSum - k, 0);
sumFrequency.put(currentSum, sumFrequency.getOrDefault(currentSum, 0) + 1);
}
return count;
}
}
Recognition Pattern 5: Difference Array (Variant of Prefix Sum)
While not strictly a prefix sum, the difference array (or prefix sum of differences) is a closely related concept that’s useful for range update queries.
Problem Characteristics:
- You need to perform multiple range updates (add a value to all elements in a range).
- After updates, you need to query the final state of individual elements or ranges.
Example Problem:
Given an array initially filled with zeros, perform multiple range update operations where you add a value to all elements in a given range. After all updates, return the final array.
Solution Approach:
Use a difference array to mark the start and end of each update range. After all updates, the prefix sum of the difference array gives the final state of the original array.
Code Example:
class RangeUpdate {
public int[] getModifiedArray(int length, int[][] updates) {
int[] diff = new int[length + 1];
for (int[] update : updates) {
int start = update[0];
int end = update[1];
int val = update[2];
diff[start] += val;
diff[end + 1] -= val;
}
int[] result = new int[length];
int prefixSum = 0;
for (int i = 0; i < length; i++) {
prefixSum += diff[i];
result[i] = prefixSum;
}
return result;
}
}
When Not to Use Prefix Sum Arrays
While prefix sum arrays are powerful, they’re not always the best solution. Here are some situations where you might want to consider alternative approaches:
- Frequently Changing Arrays: If the underlying array is frequently modified, maintaining a prefix sum array becomes costly.
- Space Constraints: If memory is a significant constraint and the original array is very large, the additional space for a prefix sum array might be prohibitive.
- Single Query Scenarios: If you only need to perform a single range sum query, it might be more efficient to simply iterate through the range rather than constructing a prefix sum array.
- Non-Additive Properties: Prefix sum arrays work well with additive properties. For non-additive properties (like finding the maximum in a range), other data structures like segment trees might be more appropriate.
Conclusion
Recognizing when to use a prefix sum array is a valuable skill in algorithmic problem-solving. By identifying patterns such as range sum queries, cumulative property calculations, equilibrium index problems, subarray sum problems, and scenarios requiring difference arrays, you can quickly determine if a prefix sum approach will lead to an efficient solution.
Remember, the key advantages of prefix sum arrays are:
- O(1) time complexity for range sum queries after O(n) preprocessing
- Ability to handle multiple queries efficiently on static arrays
- Simplification of cumulative calculations and subarray-related problems
As you encounter more problems, you’ll develop an intuition for when to apply this technique. Practice is key – try implementing prefix sum solutions for various problems to reinforce your understanding and recognition of these patterns.
By mastering the use of prefix sum arrays, you’ll add a powerful tool to your algorithmic toolkit, enabling you to solve a wide range of problems with improved efficiency and elegance. Happy coding!