When to Use a Min/Max Heap in Your Solution
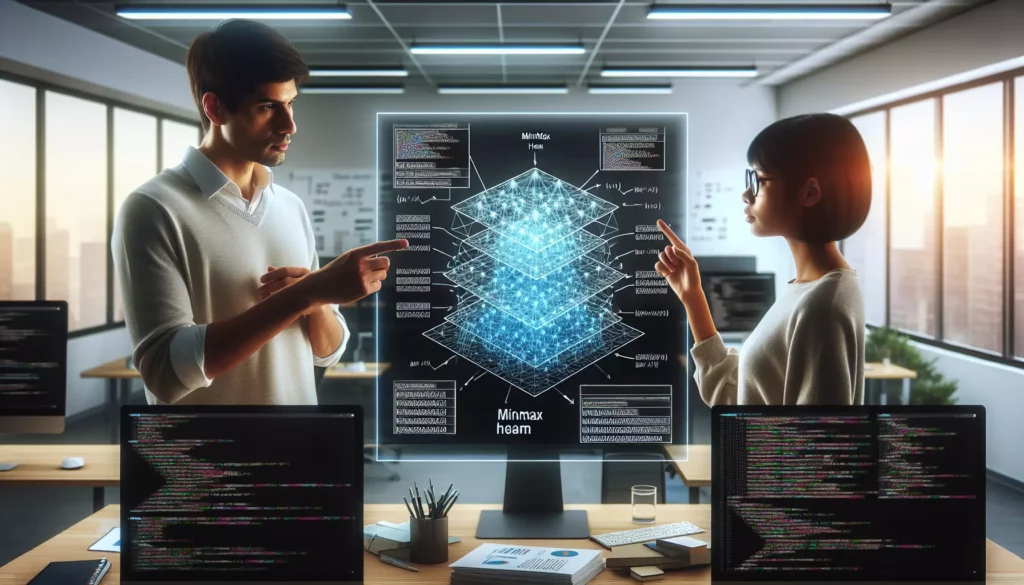
As you progress in your coding journey and prepare for technical interviews, particularly for major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), you’ll encounter various data structures and algorithms. One such essential data structure is the heap, specifically the min heap and max heap. Understanding when and how to use these structures can significantly improve your problem-solving skills and algorithm efficiency. In this comprehensive guide, we’ll explore the intricacies of min and max heaps, their applications, and when to leverage them in your coding solutions.
What are Min and Max Heaps?
Before diving into when to use min and max heaps, let’s briefly review what they are:
Min Heap
A min heap is a binary tree-based data structure where the parent node is always smaller than or equal to its children. The root node contains the minimum element in the entire heap.
Max Heap
Conversely, a max heap is a binary tree-based structure where the parent node is always larger than or equal to its children. The root node contains the maximum element in the entire heap.
Both min and max heaps are complete binary trees, meaning all levels are filled except possibly the last level, which is filled from left to right.
Key Properties of Heaps
Understanding the properties of heaps is crucial for determining when to use them in your solutions:
- Efficient insertion and deletion: Both operations take O(log n) time complexity.
- Quick access to the minimum/maximum element: The root node always contains the min/max element, accessible in O(1) time.
- Space efficiency: Heaps can be implemented using arrays, making them memory-efficient.
- Self-balancing: Heaps maintain their structure automatically after insertions and deletions.
When to Use a Min Heap
Min heaps are particularly useful in scenarios where you need quick access to the smallest element in a dataset. Here are some common use cases:
1. Priority Queues with Smallest Element Priority
When implementing a priority queue where the smallest element has the highest priority, a min heap is an excellent choice. This is useful in scenarios like:
- Task scheduling based on priority (lower number = higher priority)
- Implementing Dijkstra’s algorithm for finding the shortest path in a graph
- Managing network packet routing based on packet size or priority
2. K Smallest Elements Problems
When you need to find the k smallest elements in an array or stream of data, a min heap can be very efficient. Here’s a common interview question that can be solved using a min heap:
Problem: Find the kth smallest element in an unsorted array.
Solution approach:
- Create a max heap of size k
- Iterate through the array, adding elements to the heap
- If the heap size exceeds k, remove the largest element
- After processing all elements, the root of the heap will be the kth smallest element
import heapq
def kth_smallest(arr, k):
heap = []
for num in arr:
if len(heap) < k:
heapq.heappush(heap, -num) # Use negative for max heap behavior
elif -num > heap[0]:
heapq.heappushpop(heap, -num)
return -heap[0]
# Example usage
arr = [7, 10, 4, 3, 20, 15]
k = 3
print(f"The {k}th smallest element is: {kth_smallest(arr, k)}")
3. Merge K Sorted Arrays or Lists
When you need to merge multiple sorted arrays or lists, a min heap can be very useful. This problem often appears in system design interviews or when dealing with large datasets that can’t fit into memory.
Problem: Merge k sorted arrays into a single sorted array.
Solution approach:
- Create a min heap to store the smallest element from each array along with its array index and element index
- Initialize the heap with the first element from each array
- While the heap is not empty:
- Pop the smallest element and add it to the result
- If there are more elements in the array this element came from, push the next element onto the heap
import heapq
def merge_k_sorted_arrays(arrays):
result = []
heap = []
# Initialize the heap with the first element from each array
for i, arr in enumerate(arrays):
if arr:
heapq.heappush(heap, (arr[0], i, 0))
while heap:
val, array_index, element_index = heapq.heappop(heap)
result.append(val)
if element_index + 1 < len(arrays[array_index]):
next_element = arrays[array_index][element_index + 1]
heapq.heappush(heap, (next_element, array_index, element_index + 1))
return result
# Example usage
arrays = [
[1, 4, 7],
[2, 5, 8],
[3, 6, 9]
]
print("Merged sorted array:", merge_k_sorted_arrays(arrays))
When to Use a Max Heap
Max heaps are useful when you need quick access to the largest element in a dataset. Here are some common scenarios:
1. Priority Queues with Largest Element Priority
When implementing a priority queue where the largest element has the highest priority, a max heap is the go-to choice. This is useful in scenarios like:
- CPU scheduling in operating systems (highest priority process first)
- Auction systems where the highest bid gets priority
- Emergency room triage systems (most critical patients first)
2. K Largest Elements Problems
Similar to the k smallest elements problem, max heaps are excellent for finding the k largest elements in an array or stream of data.
Problem: Find the kth largest element in an unsorted array.
Solution approach:
- Create a min heap of size k
- Iterate through the array, adding elements to the heap
- If the heap size exceeds k, remove the smallest element
- After processing all elements, the root of the heap will be the kth largest element
import heapq
def kth_largest(arr, k):
heap = []
for num in arr:
if len(heap) < k:
heapq.heappush(heap, num)
elif num > heap[0]:
heapq.heappushpop(heap, num)
return heap[0]
# Example usage
arr = [3, 2, 1, 5, 6, 4]
k = 2
print(f"The {k}th largest element is: {kth_largest(arr, k)}")
3. Heap Sort
While not as commonly used as quicksort or mergesort, heapsort is an efficient sorting algorithm that uses a max heap to sort elements in ascending order. It has a time complexity of O(n log n) and is an in-place sorting algorithm, which means it doesn’t require extra space proportional to the input size.
Here’s a basic implementation of heapsort:
def heapify(arr, n, i):
largest = i
left = 2 * i + 1
right = 2 * i + 2
if left < n and arr[left] > arr[largest]:
largest = left
if right < n and arr[right] > arr[largest]:
largest = right
if largest != i:
arr[i], arr[largest] = arr[largest], arr[i]
heapify(arr, n, largest)
def heap_sort(arr):
n = len(arr)
# Build a max heap
for i in range(n // 2 - 1, -1, -1):
heapify(arr, n, i)
# Extract elements from the heap one by one
for i in range(n - 1, 0, -1):
arr[0], arr[i] = arr[i], arr[0]
heapify(arr, i, 0)
return arr
# Example usage
arr = [12, 11, 13, 5, 6, 7]
sorted_arr = heap_sort(arr)
print("Sorted array:", sorted_arr)
Implementing Heaps in Different Programming Languages
Many programming languages provide built-in implementations or libraries for heaps. Here’s how you can work with heaps in some popular languages:
Python
Python’s heapq
module provides an implementation of the heap queue algorithm. By default, it’s a min heap.
import heapq
# Min heap
min_heap = []
heapq.heappush(min_heap, 3)
heapq.heappush(min_heap, 1)
heapq.heappush(min_heap, 4)
print(heapq.heappop(min_heap)) # Output: 1
# Max heap (use negative values)
max_heap = []
heapq.heappush(max_heap, -3)
heapq.heappush(max_heap, -1)
heapq.heappush(max_heap, -4)
print(-heapq.heappop(max_heap)) # Output: 4
Java
Java provides the PriorityQueue
class, which is an implementation of a min heap. For a max heap, you can use a custom comparator.
import java.util.PriorityQueue;
import java.util.Collections;
public class HeapExample {
public static void main(String[] args) {
// Min heap
PriorityQueue<Integer> minHeap = new PriorityQueue<>();
minHeap.add(3);
minHeap.add(1);
minHeap.add(4);
System.out.println(minHeap.poll()); // Output: 1
// Max heap
PriorityQueue<Integer> maxHeap = new PriorityQueue<>(Collections.reverseOrder());
maxHeap.add(3);
maxHeap.add(1);
maxHeap.add(4);
System.out.println(maxHeap.poll()); // Output: 4
}
}
C++
C++ provides the priority_queue
container adapter, which is by default a max heap. For a min heap, you can use greater<int>
as the comparison function.
#include <iostream>
#include <queue>
int main() {
// Max heap
std::priority_queue<int> maxHeap;
maxHeap.push(3);
maxHeap.push(1);
maxHeap.push(4);
std::cout << maxHeap.top() << std::endl; // Output: 4
// Min heap
std::priority_queue<int, std::vector<int>, std::greater<int>> minHeap;
minHeap.push(3);
minHeap.push(1);
minHeap.push(4);
std::cout << minHeap.top() << std::endl; // Output: 1
return 0;
}
Common Pitfalls and Best Practices
When working with heaps, keep these points in mind:
- Time complexity awareness: While insertion and deletion are O(log n), building a heap from an array is O(n). Be mindful of this when analyzing your algorithm’s overall time complexity.
- Space complexity: Heaps typically require O(n) space. If memory is a constraint, consider alternative solutions or in-place heap implementations.
- Choosing between min and max heaps: Always consider whether you need quick access to the minimum or maximum element. Using the wrong type can lead to inefficient solutions.
- Heap size management: When solving k-th element problems, maintain the heap size at k to ensure optimal performance.
- Custom comparators: For complex objects, remember to implement custom comparators to define the heap order correctly.
Conclusion
Min and max heaps are powerful data structures that can significantly improve the efficiency of your algorithms in various scenarios. They are particularly useful for problems involving priority queues, k-th element selection, and certain sorting algorithms. As you prepare for technical interviews and tackle complex coding challenges, keep heaps in your problem-solving toolkit.
Remember, the key to mastering heaps and other data structures is practice. Implement them from scratch, solve problems using them, and analyze their performance in different scenarios. This hands-on experience will not only prepare you for interviews but also make you a more effective programmer in real-world situations.
As you continue your journey with AlgoCademy, take advantage of our interactive coding tutorials and AI-powered assistance to deepen your understanding of heaps and other essential data structures and algorithms. Happy coding!