When to Use a Divide and Conquer Approach in Programming
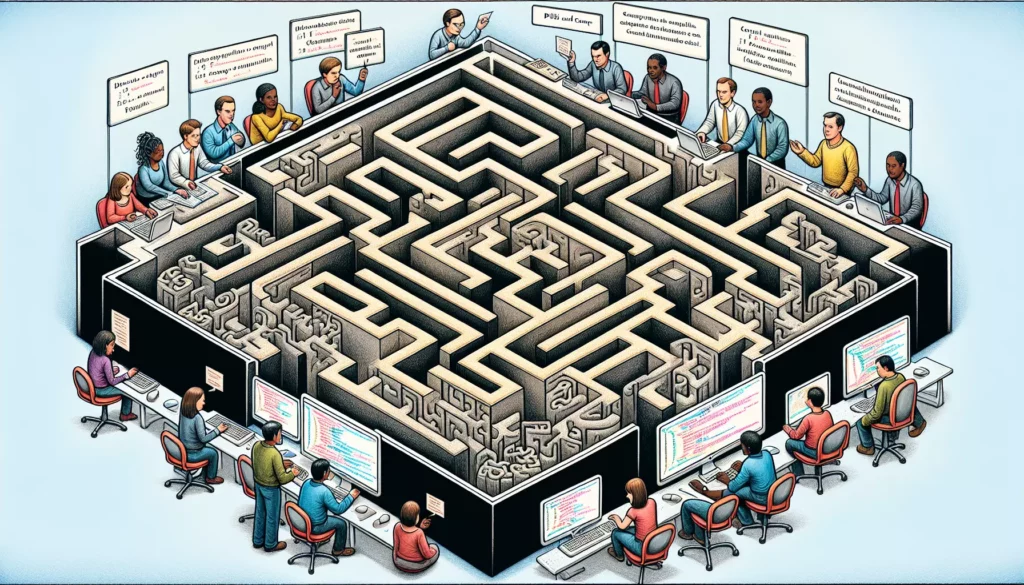
In the world of algorithmic problem-solving and efficient programming, the divide and conquer approach stands out as a powerful technique. This strategy, which involves breaking down complex problems into smaller, more manageable subproblems, solving them independently, and then combining the results, has proven invaluable in numerous applications. But when exactly should you reach for this tool in your coding toolkit? In this comprehensive guide, we’ll explore the scenarios where divide and conquer shines, its benefits, and how to recognize when it’s the right choice for your programming challenges.
Understanding Divide and Conquer
Before diving into when to use divide and conquer, let’s briefly recap what this approach entails:
- Divide: Break the original problem into smaller subproblems.
- Conquer: Recursively solve these subproblems.
- Combine: Merge the solutions of the subproblems to create a solution to the original problem.
This paradigm is the foundation for many efficient algorithms and is particularly useful when dealing with problems that exhibit certain characteristics.
Key Indicators for Using Divide and Conquer
Here are some telltale signs that a problem might be well-suited for a divide and conquer approach:
1. The Problem Can Be Broken Down into Similar Subproblems
If you can decompose your problem into smaller instances of the same problem, divide and conquer might be appropriate. For example, sorting algorithms like Merge Sort and Quick Sort use this principle effectively.
2. Subproblems Are Independent
When the subproblems can be solved independently without affecting each other, divide and conquer becomes particularly powerful. This independence allows for potential parallelization, which can lead to significant performance improvements.
3. Solutions to Subproblems Can Be Efficiently Combined
The ability to merge solutions quickly is crucial. If combining solutions takes longer than solving the problem directly, the divide and conquer approach may not be beneficial.
4. The Problem Size Reduces Significantly with Each Division
Effective divide and conquer algorithms typically reduce the problem size by a constant factor with each recursive step. This leads to logarithmic time complexity in many cases.
5. There’s a Clear Base Case
A well-defined base case that can be solved directly is essential for any recursive algorithm, including divide and conquer methods.
Common Scenarios for Divide and Conquer
Let’s explore some typical scenarios where divide and conquer is particularly effective:
Sorting Algorithms
Sorting is perhaps the most classic application of divide and conquer. Algorithms like Merge Sort and Quick Sort exemplify this approach:
Merge Sort
Merge Sort divides the array into two halves, recursively sorts them, and then merges the sorted halves. Here’s a simple implementation in Python:
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = merge_sort(arr[:mid])
right = merge_sort(arr[mid:])
return merge(left, right)
def merge(left, right):
result = []
i, j = 0, 0
while i < len(left) and j < len(right):
if left[i] <= right[j]:
result.append(left[i])
i += 1
else:
result.append(right[j])
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
Quick Sort
Quick Sort selects a ‘pivot’ element and partitions the array around it, recursively sorting the subarrays on either side of the pivot:
def quick_sort(arr):
if len(arr) <= 1:
return arr
pivot = arr[len(arr) // 2]
left = [x for x in arr if x < pivot]
middle = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
return quick_sort(left) + middle + quick_sort(right)
Binary Search
Binary search is another classic divide and conquer algorithm. It’s used to find an element in a sorted array by repeatedly dividing the search interval in half:
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1 # Element not found
Matrix Multiplication
Strassen’s algorithm for matrix multiplication is a sophisticated application of divide and conquer. It reduces the number of recursive calls compared to the naive approach, resulting in better performance for large matrices.
Closest Pair of Points
This geometric problem involves finding the two closest points in a set. A divide and conquer approach can solve this in O(n log n) time, which is more efficient than the naive O(n^2) solution.
Fast Fourier Transform (FFT)
The FFT, crucial in signal processing and polynomial multiplication, uses divide and conquer to compute the Discrete Fourier Transform efficiently.
Benefits of Using Divide and Conquer
When applied appropriately, divide and conquer offers several advantages:
1. Improved Efficiency
Many divide and conquer algorithms achieve logarithmic or linearithmic time complexity, making them highly efficient for large datasets.
2. Parallelization Potential
The independent nature of subproblems in divide and conquer algorithms often allows for effective parallelization, which can significantly speed up execution on multi-core processors.
3. Simplification of Complex Problems
Breaking down a complex problem into smaller, more manageable parts can make the overall solution easier to conceptualize and implement.
4. Reusability
The recursive nature of divide and conquer often leads to elegant, reusable code structures.
Challenges and Considerations
While divide and conquer is powerful, it’s not always the best choice. Consider these factors:
1. Overhead of Recursion
The recursive nature of many divide and conquer algorithms can lead to stack overflow for very large inputs or consume excessive memory.
2. Complexity of Implementation
Some divide and conquer algorithms can be more complex to implement correctly compared to iterative solutions.
3. Not Always the Most Efficient
For smaller datasets or certain problem types, simpler algorithms might outperform divide and conquer approaches.
Real-World Applications
Divide and conquer isn’t just a theoretical concept; it has numerous practical applications:
1. Database Systems
Many database operations, including indexing and query optimization, utilize divide and conquer principles.
2. Image Processing
Algorithms for image compression, such as those used in JPEG encoding, often employ divide and conquer strategies.
3. Machine Learning
Some clustering algorithms and decision tree construction methods in machine learning use divide and conquer approaches.
4. Big Data Processing
MapReduce, a programming model for processing large datasets, is fundamentally based on the divide and conquer paradigm.
When Not to Use Divide and Conquer
While powerful, divide and conquer isn’t always the best choice. Here are some scenarios where you might want to consider alternative approaches:
1. Simple, Linear Problems
For straightforward problems that can be solved efficiently with a single pass through the data, a simple iterative approach is often more appropriate.
2. Problems with Interdependent Subproblems
If the subproblems are not truly independent and require significant communication or shared state, divide and conquer may not be efficient.
3. Memory-Constrained Environments
In situations where memory is severely limited, the recursive nature of many divide and conquer algorithms might cause issues.
4. When Combining Solutions is Costly
If merging the solutions to subproblems is computationally expensive, the benefits of divide and conquer might be negated.
Implementing Divide and Conquer: Best Practices
When you decide to use a divide and conquer approach, keep these best practices in mind:
1. Identify the Base Case
Clearly define the simplest form of the problem that can be solved directly. This is crucial for terminating the recursion.
2. Ensure Proper Division
The division step should significantly reduce the problem size, ideally by a constant factor.
3. Optimize the Combine Step
The efficiency of combining solutions often determines the overall performance of the algorithm.
4. Consider Tail Recursion
Where possible, structure your recursion to be tail-recursive, as this can be optimized by many compilers.
5. Test with Various Input Sizes
Ensure your implementation performs well with both small and large inputs.
Advanced Divide and Conquer Techniques
As you become more comfortable with basic divide and conquer strategies, consider exploring these advanced techniques:
1. Multi-way Divide and Conquer
Instead of dividing into two subproblems, some algorithms divide into multiple subproblems. For example, the Fast Fourier Transform (FFT) often uses a radix-2 approach, dividing the problem into two, but can be generalized to higher radixes.
2. Hybrid Algorithms
Some algorithms combine divide and conquer with other techniques. For instance, Introsort, used in many standard library implementations of quicksort, switches to heapsort if the recursion depth becomes too large.
3. Parallel Divide and Conquer
Leveraging multi-core processors or distributed systems to solve subproblems in parallel can lead to significant speedups. Here’s a simple example using Python’s concurrent.futures module:
import concurrent.futures
def parallel_divide_and_conquer(problem):
if is_base_case(problem):
return solve_base_case(problem)
subproblems = divide(problem)
with concurrent.futures.ThreadPoolExecutor() as executor:
futures = [executor.submit(parallel_divide_and_conquer, subproblem) for subproblem in subproblems]
results = [future.result() for future in concurrent.futures.as_completed(futures)]
return combine(results)
4. Dynamic Programming with Divide and Conquer
Some problems can benefit from a combination of divide and conquer and dynamic programming. This approach, sometimes called “divide and conquer DP,” can be particularly effective for optimization problems.
Conclusion
The divide and conquer approach is a fundamental paradigm in computer science and algorithm design. Its ability to break down complex problems into manageable pieces makes it an invaluable tool in a programmer’s arsenal. By understanding when to apply divide and conquer, you can tackle a wide range of problems more efficiently and elegantly.
Remember, the key to mastering divide and conquer is practice. Start with classic problems like sorting and searching, then gradually move on to more complex applications. As you gain experience, you’ll develop an intuition for when divide and conquer is the right choice and how to implement it effectively.
Whether you’re preparing for technical interviews, working on large-scale software projects, or simply looking to improve your problem-solving skills, a solid grasp of divide and conquer techniques will serve you well. So, the next time you face a challenging programming problem, ask yourself: Can I divide this problem into smaller, similar subproblems? If the answer is yes, you might just have found the perfect opportunity to apply the powerful divide and conquer approach.